mirror of
https://gitea.com/Lydanne/buildx.git
synced 2025-07-01 08:58:36 +08:00
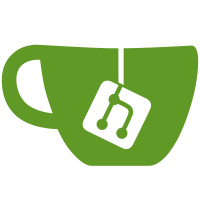
This adds a build duration metric for the build command with attributes related to the buildx driver, the error type (if any), and which options were used to perform the build from a subset of the options. This also refactors some of the utility methods used by the git tool to determine filepaths into its own separate package so they can be reused in another place. Also adds a test to ensure the resource is initialized correctly and doesn't error. The otel handler logging message is suppressed on buildx invocations so we never see the error if there's a problem with the schema url. It's so easy to mess up the schema url when upgrading OTEL that we need a proper test to make sure we haven't broken the functionality. Signed-off-by: Jonathan A. Sternberg <jonathan.sternberg@docker.com>
43 lines
928 B
Go
43 lines
928 B
Go
//go:build !windows
|
|
// +build !windows
|
|
|
|
package gitutil
|
|
|
|
import (
|
|
"os"
|
|
"os/exec"
|
|
"path/filepath"
|
|
|
|
"github.com/moby/sys/mountinfo"
|
|
)
|
|
|
|
func gitPath(wd string) (string, error) {
|
|
// On WSL2 we need to check if the current working directory is mounted on
|
|
// a Windows drive and if so, we need to use the Windows git executable.
|
|
if os.Getenv("WSL_DISTRO_NAME") != "" && wd != "" {
|
|
// ensure any symlinks are resolved
|
|
wdPath, err := filepath.EvalSymlinks(wd)
|
|
if err != nil {
|
|
return "", err
|
|
}
|
|
mi, err := mountinfo.GetMounts(mountinfo.ParentsFilter(wdPath))
|
|
if err != nil {
|
|
return "", err
|
|
}
|
|
// find the longest mount point
|
|
var idx, maxlen int
|
|
for i := range mi {
|
|
if len(mi[i].Mountpoint) > maxlen {
|
|
maxlen = len(mi[i].Mountpoint)
|
|
idx = i
|
|
}
|
|
}
|
|
if mi[idx].FSType == "9p" {
|
|
if p, err := exec.LookPath("git.exe"); err == nil {
|
|
return p, nil
|
|
}
|
|
}
|
|
}
|
|
return exec.LookPath("git")
|
|
}
|