mirror of
https://gitea.com/Lydanne/buildx.git
synced 2025-05-18 00:47:48 +08:00
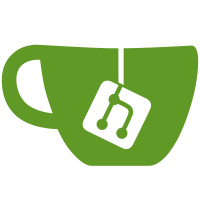
Removes gogo/protobuf from buildx and updates to a version of moby/buildkit where gogo is removed. This also changes how the proto files are generated. This is because newer versions of protobuf are more strict about name conflicts. If two files have the same name (even if they are relative paths) and are used in different protoc commands, they'll conflict in the registry. Since protobuf file generation doesn't work very well with `paths=source_relative`, this removes the `go:generate` expression and just relies on the dockerfile to perform the generation. Signed-off-by: Jonathan A. Sternberg <jonathan.sternberg@docker.com>
81 lines
2.1 KiB
Go
81 lines
2.1 KiB
Go
// Copyright 2019 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package term
|
|
|
|
import (
|
|
"os"
|
|
|
|
"golang.org/x/sys/windows"
|
|
)
|
|
|
|
type state struct {
|
|
mode uint32
|
|
}
|
|
|
|
func isTerminal(fd int) bool {
|
|
var st uint32
|
|
err := windows.GetConsoleMode(windows.Handle(fd), &st)
|
|
return err == nil
|
|
}
|
|
|
|
func makeRaw(fd int) (*State, error) {
|
|
var st uint32
|
|
if err := windows.GetConsoleMode(windows.Handle(fd), &st); err != nil {
|
|
return nil, err
|
|
}
|
|
raw := st &^ (windows.ENABLE_ECHO_INPUT | windows.ENABLE_PROCESSED_INPUT | windows.ENABLE_LINE_INPUT | windows.ENABLE_PROCESSED_OUTPUT)
|
|
raw |= windows.ENABLE_VIRTUAL_TERMINAL_INPUT
|
|
if err := windows.SetConsoleMode(windows.Handle(fd), raw); err != nil {
|
|
return nil, err
|
|
}
|
|
return &State{state{st}}, nil
|
|
}
|
|
|
|
func getState(fd int) (*State, error) {
|
|
var st uint32
|
|
if err := windows.GetConsoleMode(windows.Handle(fd), &st); err != nil {
|
|
return nil, err
|
|
}
|
|
return &State{state{st}}, nil
|
|
}
|
|
|
|
func restore(fd int, state *State) error {
|
|
return windows.SetConsoleMode(windows.Handle(fd), state.mode)
|
|
}
|
|
|
|
func getSize(fd int) (width, height int, err error) {
|
|
var info windows.ConsoleScreenBufferInfo
|
|
if err := windows.GetConsoleScreenBufferInfo(windows.Handle(fd), &info); err != nil {
|
|
return 0, 0, err
|
|
}
|
|
return int(info.Window.Right - info.Window.Left + 1), int(info.Window.Bottom - info.Window.Top + 1), nil
|
|
}
|
|
|
|
func readPassword(fd int) ([]byte, error) {
|
|
var st uint32
|
|
if err := windows.GetConsoleMode(windows.Handle(fd), &st); err != nil {
|
|
return nil, err
|
|
}
|
|
old := st
|
|
|
|
st &^= (windows.ENABLE_ECHO_INPUT | windows.ENABLE_LINE_INPUT)
|
|
st |= (windows.ENABLE_PROCESSED_OUTPUT | windows.ENABLE_PROCESSED_INPUT)
|
|
if err := windows.SetConsoleMode(windows.Handle(fd), st); err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
defer windows.SetConsoleMode(windows.Handle(fd), old)
|
|
|
|
var h windows.Handle
|
|
p, _ := windows.GetCurrentProcess()
|
|
if err := windows.DuplicateHandle(p, windows.Handle(fd), p, &h, 0, false, windows.DUPLICATE_SAME_ACCESS); err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
f := os.NewFile(uintptr(h), "stdin")
|
|
defer f.Close()
|
|
return readPasswordLine(f)
|
|
}
|