mirror of
https://gitea.com/Lydanne/buildx.git
synced 2025-05-19 01:47:43 +08:00
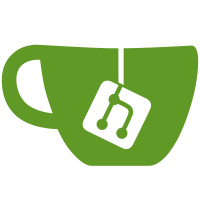
Removes gogo/protobuf from buildx and updates to a version of moby/buildkit where gogo is removed. This also changes how the proto files are generated. This is because newer versions of protobuf are more strict about name conflicts. If two files have the same name (even if they are relative paths) and are used in different protoc commands, they'll conflict in the registry. Since protobuf file generation doesn't work very well with `paths=source_relative`, this removes the `go:generate` expression and just relies on the dockerfile to perform the generation. Signed-off-by: Jonathan A. Sternberg <jonathan.sternberg@docker.com>
69 lines
1.7 KiB
Go
69 lines
1.7 KiB
Go
// Copyright 2018 The Go Authors. All rights reserved.
|
|
// Use of this source code is governed by a BSD-style
|
|
// license that can be found in the LICENSE file.
|
|
|
|
package packages
|
|
|
|
import (
|
|
"fmt"
|
|
"os"
|
|
"sort"
|
|
)
|
|
|
|
// Visit visits all the packages in the import graph whose roots are
|
|
// pkgs, calling the optional pre function the first time each package
|
|
// is encountered (preorder), and the optional post function after a
|
|
// package's dependencies have been visited (postorder).
|
|
// The boolean result of pre(pkg) determines whether
|
|
// the imports of package pkg are visited.
|
|
func Visit(pkgs []*Package, pre func(*Package) bool, post func(*Package)) {
|
|
seen := make(map[*Package]bool)
|
|
var visit func(*Package)
|
|
visit = func(pkg *Package) {
|
|
if !seen[pkg] {
|
|
seen[pkg] = true
|
|
|
|
if pre == nil || pre(pkg) {
|
|
paths := make([]string, 0, len(pkg.Imports))
|
|
for path := range pkg.Imports {
|
|
paths = append(paths, path)
|
|
}
|
|
sort.Strings(paths) // Imports is a map, this makes visit stable
|
|
for _, path := range paths {
|
|
visit(pkg.Imports[path])
|
|
}
|
|
}
|
|
|
|
if post != nil {
|
|
post(pkg)
|
|
}
|
|
}
|
|
}
|
|
for _, pkg := range pkgs {
|
|
visit(pkg)
|
|
}
|
|
}
|
|
|
|
// PrintErrors prints to os.Stderr the accumulated errors of all
|
|
// packages in the import graph rooted at pkgs, dependencies first.
|
|
// PrintErrors returns the number of errors printed.
|
|
func PrintErrors(pkgs []*Package) int {
|
|
var n int
|
|
errModules := make(map[*Module]bool)
|
|
Visit(pkgs, nil, func(pkg *Package) {
|
|
for _, err := range pkg.Errors {
|
|
fmt.Fprintln(os.Stderr, err)
|
|
n++
|
|
}
|
|
|
|
// Print pkg.Module.Error once if present.
|
|
mod := pkg.Module
|
|
if mod != nil && mod.Error != nil && !errModules[mod] {
|
|
errModules[mod] = true
|
|
fmt.Fprintln(os.Stderr, mod.Error.Err)
|
|
n++
|
|
}
|
|
})
|
|
return n
|
|
}
|