mirror of
https://gitea.com/Lydanne/buildx.git
synced 2025-05-19 01:47:43 +08:00
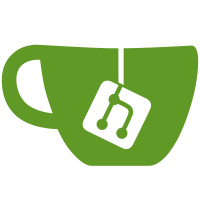
Integrates vtproto into buildx. The generated files dockerfile has been modified to copy the buildkit equivalent file to ensure files are laid out in the appropriate way for imports. An import has also been included to change the grpc codec to the version in buildkit that supports vtproto. This will allow buildx to utilize the speed and memory improvements from that. Also updates the gc control options for prune. Signed-off-by: Jonathan A. Sternberg <jonathan.sternberg@docker.com>
11431 lines
246 KiB
Go
11431 lines
246 KiB
Go
// Code generated by protoc-gen-go-vtproto. DO NOT EDIT.
|
|
// protoc-gen-go-vtproto version: v0.6.1-0.20240319094008-0393e58bdf10
|
|
// source: github.com/docker/buildx/controller/pb/controller.proto
|
|
|
|
package pb
|
|
|
|
import (
|
|
fmt "fmt"
|
|
control "github.com/moby/buildkit/api/services/control"
|
|
pb "github.com/moby/buildkit/sourcepolicy/pb"
|
|
protohelpers "github.com/planetscale/vtprotobuf/protohelpers"
|
|
proto "google.golang.org/protobuf/proto"
|
|
protoimpl "google.golang.org/protobuf/runtime/protoimpl"
|
|
io "io"
|
|
)
|
|
|
|
const (
|
|
// Verify that this generated code is sufficiently up-to-date.
|
|
_ = protoimpl.EnforceVersion(20 - protoimpl.MinVersion)
|
|
// Verify that runtime/protoimpl is sufficiently up-to-date.
|
|
_ = protoimpl.EnforceVersion(protoimpl.MaxVersion - 20)
|
|
)
|
|
|
|
func (m *ListProcessesRequest) CloneVT() *ListProcessesRequest {
|
|
if m == nil {
|
|
return (*ListProcessesRequest)(nil)
|
|
}
|
|
r := new(ListProcessesRequest)
|
|
r.Ref = m.Ref
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *ListProcessesRequest) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *ListProcessesResponse) CloneVT() *ListProcessesResponse {
|
|
if m == nil {
|
|
return (*ListProcessesResponse)(nil)
|
|
}
|
|
r := new(ListProcessesResponse)
|
|
if rhs := m.Infos; rhs != nil {
|
|
tmpContainer := make([]*ProcessInfo, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpContainer[k] = v.CloneVT()
|
|
}
|
|
r.Infos = tmpContainer
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *ListProcessesResponse) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *ProcessInfo) CloneVT() *ProcessInfo {
|
|
if m == nil {
|
|
return (*ProcessInfo)(nil)
|
|
}
|
|
r := new(ProcessInfo)
|
|
r.ProcessID = m.ProcessID
|
|
r.InvokeConfig = m.InvokeConfig.CloneVT()
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *ProcessInfo) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *DisconnectProcessRequest) CloneVT() *DisconnectProcessRequest {
|
|
if m == nil {
|
|
return (*DisconnectProcessRequest)(nil)
|
|
}
|
|
r := new(DisconnectProcessRequest)
|
|
r.Ref = m.Ref
|
|
r.ProcessID = m.ProcessID
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *DisconnectProcessRequest) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *DisconnectProcessResponse) CloneVT() *DisconnectProcessResponse {
|
|
if m == nil {
|
|
return (*DisconnectProcessResponse)(nil)
|
|
}
|
|
r := new(DisconnectProcessResponse)
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *DisconnectProcessResponse) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *BuildRequest) CloneVT() *BuildRequest {
|
|
if m == nil {
|
|
return (*BuildRequest)(nil)
|
|
}
|
|
r := new(BuildRequest)
|
|
r.Ref = m.Ref
|
|
r.Options = m.Options.CloneVT()
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *BuildRequest) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *BuildOptions) CloneVT() *BuildOptions {
|
|
if m == nil {
|
|
return (*BuildOptions)(nil)
|
|
}
|
|
r := new(BuildOptions)
|
|
r.ContextPath = m.ContextPath
|
|
r.DockerfileName = m.DockerfileName
|
|
r.CallFunc = m.CallFunc.CloneVT()
|
|
r.CgroupParent = m.CgroupParent
|
|
r.NetworkMode = m.NetworkMode
|
|
r.ShmSize = m.ShmSize
|
|
r.Target = m.Target
|
|
r.Ulimits = m.Ulimits.CloneVT()
|
|
r.Builder = m.Builder
|
|
r.NoCache = m.NoCache
|
|
r.Pull = m.Pull
|
|
r.ExportPush = m.ExportPush
|
|
r.ExportLoad = m.ExportLoad
|
|
r.Ref = m.Ref
|
|
r.GroupRef = m.GroupRef
|
|
r.ProvenanceResponseMode = m.ProvenanceResponseMode
|
|
if rhs := m.NamedContexts; rhs != nil {
|
|
tmpContainer := make(map[string]string, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpContainer[k] = v
|
|
}
|
|
r.NamedContexts = tmpContainer
|
|
}
|
|
if rhs := m.Allow; rhs != nil {
|
|
tmpContainer := make([]string, len(rhs))
|
|
copy(tmpContainer, rhs)
|
|
r.Allow = tmpContainer
|
|
}
|
|
if rhs := m.Attests; rhs != nil {
|
|
tmpContainer := make([]*Attest, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpContainer[k] = v.CloneVT()
|
|
}
|
|
r.Attests = tmpContainer
|
|
}
|
|
if rhs := m.BuildArgs; rhs != nil {
|
|
tmpContainer := make(map[string]string, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpContainer[k] = v
|
|
}
|
|
r.BuildArgs = tmpContainer
|
|
}
|
|
if rhs := m.CacheFrom; rhs != nil {
|
|
tmpContainer := make([]*CacheOptionsEntry, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpContainer[k] = v.CloneVT()
|
|
}
|
|
r.CacheFrom = tmpContainer
|
|
}
|
|
if rhs := m.CacheTo; rhs != nil {
|
|
tmpContainer := make([]*CacheOptionsEntry, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpContainer[k] = v.CloneVT()
|
|
}
|
|
r.CacheTo = tmpContainer
|
|
}
|
|
if rhs := m.Exports; rhs != nil {
|
|
tmpContainer := make([]*ExportEntry, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpContainer[k] = v.CloneVT()
|
|
}
|
|
r.Exports = tmpContainer
|
|
}
|
|
if rhs := m.ExtraHosts; rhs != nil {
|
|
tmpContainer := make([]string, len(rhs))
|
|
copy(tmpContainer, rhs)
|
|
r.ExtraHosts = tmpContainer
|
|
}
|
|
if rhs := m.Labels; rhs != nil {
|
|
tmpContainer := make(map[string]string, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpContainer[k] = v
|
|
}
|
|
r.Labels = tmpContainer
|
|
}
|
|
if rhs := m.NoCacheFilter; rhs != nil {
|
|
tmpContainer := make([]string, len(rhs))
|
|
copy(tmpContainer, rhs)
|
|
r.NoCacheFilter = tmpContainer
|
|
}
|
|
if rhs := m.Platforms; rhs != nil {
|
|
tmpContainer := make([]string, len(rhs))
|
|
copy(tmpContainer, rhs)
|
|
r.Platforms = tmpContainer
|
|
}
|
|
if rhs := m.Secrets; rhs != nil {
|
|
tmpContainer := make([]*Secret, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpContainer[k] = v.CloneVT()
|
|
}
|
|
r.Secrets = tmpContainer
|
|
}
|
|
if rhs := m.SSH; rhs != nil {
|
|
tmpContainer := make([]*SSH, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpContainer[k] = v.CloneVT()
|
|
}
|
|
r.SSH = tmpContainer
|
|
}
|
|
if rhs := m.Tags; rhs != nil {
|
|
tmpContainer := make([]string, len(rhs))
|
|
copy(tmpContainer, rhs)
|
|
r.Tags = tmpContainer
|
|
}
|
|
if rhs := m.SourcePolicy; rhs != nil {
|
|
if vtpb, ok := interface{}(rhs).(interface{ CloneVT() *pb.Policy }); ok {
|
|
r.SourcePolicy = vtpb.CloneVT()
|
|
} else {
|
|
r.SourcePolicy = proto.Clone(rhs).(*pb.Policy)
|
|
}
|
|
}
|
|
if rhs := m.Annotations; rhs != nil {
|
|
tmpContainer := make([]string, len(rhs))
|
|
copy(tmpContainer, rhs)
|
|
r.Annotations = tmpContainer
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *BuildOptions) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *ExportEntry) CloneVT() *ExportEntry {
|
|
if m == nil {
|
|
return (*ExportEntry)(nil)
|
|
}
|
|
r := new(ExportEntry)
|
|
r.Type = m.Type
|
|
r.Destination = m.Destination
|
|
if rhs := m.Attrs; rhs != nil {
|
|
tmpContainer := make(map[string]string, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpContainer[k] = v
|
|
}
|
|
r.Attrs = tmpContainer
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *ExportEntry) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *CacheOptionsEntry) CloneVT() *CacheOptionsEntry {
|
|
if m == nil {
|
|
return (*CacheOptionsEntry)(nil)
|
|
}
|
|
r := new(CacheOptionsEntry)
|
|
r.Type = m.Type
|
|
if rhs := m.Attrs; rhs != nil {
|
|
tmpContainer := make(map[string]string, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpContainer[k] = v
|
|
}
|
|
r.Attrs = tmpContainer
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *CacheOptionsEntry) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *Attest) CloneVT() *Attest {
|
|
if m == nil {
|
|
return (*Attest)(nil)
|
|
}
|
|
r := new(Attest)
|
|
r.Type = m.Type
|
|
r.Disabled = m.Disabled
|
|
r.Attrs = m.Attrs
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *Attest) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *SSH) CloneVT() *SSH {
|
|
if m == nil {
|
|
return (*SSH)(nil)
|
|
}
|
|
r := new(SSH)
|
|
r.ID = m.ID
|
|
if rhs := m.Paths; rhs != nil {
|
|
tmpContainer := make([]string, len(rhs))
|
|
copy(tmpContainer, rhs)
|
|
r.Paths = tmpContainer
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *SSH) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *Secret) CloneVT() *Secret {
|
|
if m == nil {
|
|
return (*Secret)(nil)
|
|
}
|
|
r := new(Secret)
|
|
r.ID = m.ID
|
|
r.FilePath = m.FilePath
|
|
r.Env = m.Env
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *Secret) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *CallFunc) CloneVT() *CallFunc {
|
|
if m == nil {
|
|
return (*CallFunc)(nil)
|
|
}
|
|
r := new(CallFunc)
|
|
r.Name = m.Name
|
|
r.Format = m.Format
|
|
r.IgnoreStatus = m.IgnoreStatus
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *CallFunc) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *InspectRequest) CloneVT() *InspectRequest {
|
|
if m == nil {
|
|
return (*InspectRequest)(nil)
|
|
}
|
|
r := new(InspectRequest)
|
|
r.Ref = m.Ref
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *InspectRequest) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *InspectResponse) CloneVT() *InspectResponse {
|
|
if m == nil {
|
|
return (*InspectResponse)(nil)
|
|
}
|
|
r := new(InspectResponse)
|
|
r.Options = m.Options.CloneVT()
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *InspectResponse) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *UlimitOpt) CloneVT() *UlimitOpt {
|
|
if m == nil {
|
|
return (*UlimitOpt)(nil)
|
|
}
|
|
r := new(UlimitOpt)
|
|
if rhs := m.Values; rhs != nil {
|
|
tmpContainer := make(map[string]*Ulimit, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpContainer[k] = v.CloneVT()
|
|
}
|
|
r.Values = tmpContainer
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *UlimitOpt) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *Ulimit) CloneVT() *Ulimit {
|
|
if m == nil {
|
|
return (*Ulimit)(nil)
|
|
}
|
|
r := new(Ulimit)
|
|
r.Name = m.Name
|
|
r.Hard = m.Hard
|
|
r.Soft = m.Soft
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *Ulimit) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *BuildResponse) CloneVT() *BuildResponse {
|
|
if m == nil {
|
|
return (*BuildResponse)(nil)
|
|
}
|
|
r := new(BuildResponse)
|
|
if rhs := m.ExporterResponse; rhs != nil {
|
|
tmpContainer := make(map[string]string, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpContainer[k] = v
|
|
}
|
|
r.ExporterResponse = tmpContainer
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *BuildResponse) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *DisconnectRequest) CloneVT() *DisconnectRequest {
|
|
if m == nil {
|
|
return (*DisconnectRequest)(nil)
|
|
}
|
|
r := new(DisconnectRequest)
|
|
r.Ref = m.Ref
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *DisconnectRequest) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *DisconnectResponse) CloneVT() *DisconnectResponse {
|
|
if m == nil {
|
|
return (*DisconnectResponse)(nil)
|
|
}
|
|
r := new(DisconnectResponse)
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *DisconnectResponse) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *ListRequest) CloneVT() *ListRequest {
|
|
if m == nil {
|
|
return (*ListRequest)(nil)
|
|
}
|
|
r := new(ListRequest)
|
|
r.Ref = m.Ref
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *ListRequest) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *ListResponse) CloneVT() *ListResponse {
|
|
if m == nil {
|
|
return (*ListResponse)(nil)
|
|
}
|
|
r := new(ListResponse)
|
|
if rhs := m.Keys; rhs != nil {
|
|
tmpContainer := make([]string, len(rhs))
|
|
copy(tmpContainer, rhs)
|
|
r.Keys = tmpContainer
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *ListResponse) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *InputMessage) CloneVT() *InputMessage {
|
|
if m == nil {
|
|
return (*InputMessage)(nil)
|
|
}
|
|
r := new(InputMessage)
|
|
if m.Input != nil {
|
|
r.Input = m.Input.(interface{ CloneVT() isInputMessage_Input }).CloneVT()
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *InputMessage) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *InputMessage_Init) CloneVT() isInputMessage_Input {
|
|
if m == nil {
|
|
return (*InputMessage_Init)(nil)
|
|
}
|
|
r := new(InputMessage_Init)
|
|
r.Init = m.Init.CloneVT()
|
|
return r
|
|
}
|
|
|
|
func (m *InputMessage_Data) CloneVT() isInputMessage_Input {
|
|
if m == nil {
|
|
return (*InputMessage_Data)(nil)
|
|
}
|
|
r := new(InputMessage_Data)
|
|
r.Data = m.Data.CloneVT()
|
|
return r
|
|
}
|
|
|
|
func (m *InputInitMessage) CloneVT() *InputInitMessage {
|
|
if m == nil {
|
|
return (*InputInitMessage)(nil)
|
|
}
|
|
r := new(InputInitMessage)
|
|
r.Ref = m.Ref
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *InputInitMessage) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *DataMessage) CloneVT() *DataMessage {
|
|
if m == nil {
|
|
return (*DataMessage)(nil)
|
|
}
|
|
r := new(DataMessage)
|
|
r.EOF = m.EOF
|
|
if rhs := m.Data; rhs != nil {
|
|
tmpBytes := make([]byte, len(rhs))
|
|
copy(tmpBytes, rhs)
|
|
r.Data = tmpBytes
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *DataMessage) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *InputResponse) CloneVT() *InputResponse {
|
|
if m == nil {
|
|
return (*InputResponse)(nil)
|
|
}
|
|
r := new(InputResponse)
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *InputResponse) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *Message) CloneVT() *Message {
|
|
if m == nil {
|
|
return (*Message)(nil)
|
|
}
|
|
r := new(Message)
|
|
if m.Input != nil {
|
|
r.Input = m.Input.(interface{ CloneVT() isMessage_Input }).CloneVT()
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *Message) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *Message_Init) CloneVT() isMessage_Input {
|
|
if m == nil {
|
|
return (*Message_Init)(nil)
|
|
}
|
|
r := new(Message_Init)
|
|
r.Init = m.Init.CloneVT()
|
|
return r
|
|
}
|
|
|
|
func (m *Message_File) CloneVT() isMessage_Input {
|
|
if m == nil {
|
|
return (*Message_File)(nil)
|
|
}
|
|
r := new(Message_File)
|
|
r.File = m.File.CloneVT()
|
|
return r
|
|
}
|
|
|
|
func (m *Message_Resize) CloneVT() isMessage_Input {
|
|
if m == nil {
|
|
return (*Message_Resize)(nil)
|
|
}
|
|
r := new(Message_Resize)
|
|
r.Resize = m.Resize.CloneVT()
|
|
return r
|
|
}
|
|
|
|
func (m *Message_Signal) CloneVT() isMessage_Input {
|
|
if m == nil {
|
|
return (*Message_Signal)(nil)
|
|
}
|
|
r := new(Message_Signal)
|
|
r.Signal = m.Signal.CloneVT()
|
|
return r
|
|
}
|
|
|
|
func (m *InitMessage) CloneVT() *InitMessage {
|
|
if m == nil {
|
|
return (*InitMessage)(nil)
|
|
}
|
|
r := new(InitMessage)
|
|
r.Ref = m.Ref
|
|
r.ProcessID = m.ProcessID
|
|
r.InvokeConfig = m.InvokeConfig.CloneVT()
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *InitMessage) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *InvokeConfig) CloneVT() *InvokeConfig {
|
|
if m == nil {
|
|
return (*InvokeConfig)(nil)
|
|
}
|
|
r := new(InvokeConfig)
|
|
r.NoCmd = m.NoCmd
|
|
r.User = m.User
|
|
r.NoUser = m.NoUser
|
|
r.Cwd = m.Cwd
|
|
r.NoCwd = m.NoCwd
|
|
r.Tty = m.Tty
|
|
r.Rollback = m.Rollback
|
|
r.Initial = m.Initial
|
|
if rhs := m.Entrypoint; rhs != nil {
|
|
tmpContainer := make([]string, len(rhs))
|
|
copy(tmpContainer, rhs)
|
|
r.Entrypoint = tmpContainer
|
|
}
|
|
if rhs := m.Cmd; rhs != nil {
|
|
tmpContainer := make([]string, len(rhs))
|
|
copy(tmpContainer, rhs)
|
|
r.Cmd = tmpContainer
|
|
}
|
|
if rhs := m.Env; rhs != nil {
|
|
tmpContainer := make([]string, len(rhs))
|
|
copy(tmpContainer, rhs)
|
|
r.Env = tmpContainer
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *InvokeConfig) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *FdMessage) CloneVT() *FdMessage {
|
|
if m == nil {
|
|
return (*FdMessage)(nil)
|
|
}
|
|
r := new(FdMessage)
|
|
r.Fd = m.Fd
|
|
r.EOF = m.EOF
|
|
if rhs := m.Data; rhs != nil {
|
|
tmpBytes := make([]byte, len(rhs))
|
|
copy(tmpBytes, rhs)
|
|
r.Data = tmpBytes
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *FdMessage) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *ResizeMessage) CloneVT() *ResizeMessage {
|
|
if m == nil {
|
|
return (*ResizeMessage)(nil)
|
|
}
|
|
r := new(ResizeMessage)
|
|
r.Rows = m.Rows
|
|
r.Cols = m.Cols
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *ResizeMessage) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *SignalMessage) CloneVT() *SignalMessage {
|
|
if m == nil {
|
|
return (*SignalMessage)(nil)
|
|
}
|
|
r := new(SignalMessage)
|
|
r.Name = m.Name
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *SignalMessage) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *StatusRequest) CloneVT() *StatusRequest {
|
|
if m == nil {
|
|
return (*StatusRequest)(nil)
|
|
}
|
|
r := new(StatusRequest)
|
|
r.Ref = m.Ref
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *StatusRequest) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *StatusResponse) CloneVT() *StatusResponse {
|
|
if m == nil {
|
|
return (*StatusResponse)(nil)
|
|
}
|
|
r := new(StatusResponse)
|
|
if rhs := m.Vertexes; rhs != nil {
|
|
tmpContainer := make([]*control.Vertex, len(rhs))
|
|
for k, v := range rhs {
|
|
if vtpb, ok := interface{}(v).(interface{ CloneVT() *control.Vertex }); ok {
|
|
tmpContainer[k] = vtpb.CloneVT()
|
|
} else {
|
|
tmpContainer[k] = proto.Clone(v).(*control.Vertex)
|
|
}
|
|
}
|
|
r.Vertexes = tmpContainer
|
|
}
|
|
if rhs := m.Statuses; rhs != nil {
|
|
tmpContainer := make([]*control.VertexStatus, len(rhs))
|
|
for k, v := range rhs {
|
|
if vtpb, ok := interface{}(v).(interface{ CloneVT() *control.VertexStatus }); ok {
|
|
tmpContainer[k] = vtpb.CloneVT()
|
|
} else {
|
|
tmpContainer[k] = proto.Clone(v).(*control.VertexStatus)
|
|
}
|
|
}
|
|
r.Statuses = tmpContainer
|
|
}
|
|
if rhs := m.Logs; rhs != nil {
|
|
tmpContainer := make([]*control.VertexLog, len(rhs))
|
|
for k, v := range rhs {
|
|
if vtpb, ok := interface{}(v).(interface{ CloneVT() *control.VertexLog }); ok {
|
|
tmpContainer[k] = vtpb.CloneVT()
|
|
} else {
|
|
tmpContainer[k] = proto.Clone(v).(*control.VertexLog)
|
|
}
|
|
}
|
|
r.Logs = tmpContainer
|
|
}
|
|
if rhs := m.Warnings; rhs != nil {
|
|
tmpContainer := make([]*control.VertexWarning, len(rhs))
|
|
for k, v := range rhs {
|
|
if vtpb, ok := interface{}(v).(interface{ CloneVT() *control.VertexWarning }); ok {
|
|
tmpContainer[k] = vtpb.CloneVT()
|
|
} else {
|
|
tmpContainer[k] = proto.Clone(v).(*control.VertexWarning)
|
|
}
|
|
}
|
|
r.Warnings = tmpContainer
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *StatusResponse) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *InfoRequest) CloneVT() *InfoRequest {
|
|
if m == nil {
|
|
return (*InfoRequest)(nil)
|
|
}
|
|
r := new(InfoRequest)
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *InfoRequest) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *InfoResponse) CloneVT() *InfoResponse {
|
|
if m == nil {
|
|
return (*InfoResponse)(nil)
|
|
}
|
|
r := new(InfoResponse)
|
|
r.BuildxVersion = m.BuildxVersion.CloneVT()
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *InfoResponse) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *BuildxVersion) CloneVT() *BuildxVersion {
|
|
if m == nil {
|
|
return (*BuildxVersion)(nil)
|
|
}
|
|
r := new(BuildxVersion)
|
|
r.Package = m.Package
|
|
r.Version = m.Version
|
|
r.Revision = m.Revision
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *BuildxVersion) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (this *ListProcessesRequest) EqualVT(that *ListProcessesRequest) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Ref != that.Ref {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *ListProcessesRequest) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*ListProcessesRequest)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *ListProcessesResponse) EqualVT(that *ListProcessesResponse) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if len(this.Infos) != len(that.Infos) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Infos {
|
|
vy := that.Infos[i]
|
|
if p, q := vx, vy; p != q {
|
|
if p == nil {
|
|
p = &ProcessInfo{}
|
|
}
|
|
if q == nil {
|
|
q = &ProcessInfo{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *ListProcessesResponse) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*ListProcessesResponse)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *ProcessInfo) EqualVT(that *ProcessInfo) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.ProcessID != that.ProcessID {
|
|
return false
|
|
}
|
|
if !this.InvokeConfig.EqualVT(that.InvokeConfig) {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *ProcessInfo) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*ProcessInfo)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *DisconnectProcessRequest) EqualVT(that *DisconnectProcessRequest) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Ref != that.Ref {
|
|
return false
|
|
}
|
|
if this.ProcessID != that.ProcessID {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *DisconnectProcessRequest) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*DisconnectProcessRequest)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *DisconnectProcessResponse) EqualVT(that *DisconnectProcessResponse) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *DisconnectProcessResponse) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*DisconnectProcessResponse)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *BuildRequest) EqualVT(that *BuildRequest) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Ref != that.Ref {
|
|
return false
|
|
}
|
|
if !this.Options.EqualVT(that.Options) {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *BuildRequest) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*BuildRequest)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *BuildOptions) EqualVT(that *BuildOptions) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.ContextPath != that.ContextPath {
|
|
return false
|
|
}
|
|
if this.DockerfileName != that.DockerfileName {
|
|
return false
|
|
}
|
|
if !this.CallFunc.EqualVT(that.CallFunc) {
|
|
return false
|
|
}
|
|
if len(this.NamedContexts) != len(that.NamedContexts) {
|
|
return false
|
|
}
|
|
for i, vx := range this.NamedContexts {
|
|
vy, ok := that.NamedContexts[i]
|
|
if !ok {
|
|
return false
|
|
}
|
|
if vx != vy {
|
|
return false
|
|
}
|
|
}
|
|
if len(this.Allow) != len(that.Allow) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Allow {
|
|
vy := that.Allow[i]
|
|
if vx != vy {
|
|
return false
|
|
}
|
|
}
|
|
if len(this.Attests) != len(that.Attests) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Attests {
|
|
vy := that.Attests[i]
|
|
if p, q := vx, vy; p != q {
|
|
if p == nil {
|
|
p = &Attest{}
|
|
}
|
|
if q == nil {
|
|
q = &Attest{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
}
|
|
if len(this.BuildArgs) != len(that.BuildArgs) {
|
|
return false
|
|
}
|
|
for i, vx := range this.BuildArgs {
|
|
vy, ok := that.BuildArgs[i]
|
|
if !ok {
|
|
return false
|
|
}
|
|
if vx != vy {
|
|
return false
|
|
}
|
|
}
|
|
if len(this.CacheFrom) != len(that.CacheFrom) {
|
|
return false
|
|
}
|
|
for i, vx := range this.CacheFrom {
|
|
vy := that.CacheFrom[i]
|
|
if p, q := vx, vy; p != q {
|
|
if p == nil {
|
|
p = &CacheOptionsEntry{}
|
|
}
|
|
if q == nil {
|
|
q = &CacheOptionsEntry{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
}
|
|
if len(this.CacheTo) != len(that.CacheTo) {
|
|
return false
|
|
}
|
|
for i, vx := range this.CacheTo {
|
|
vy := that.CacheTo[i]
|
|
if p, q := vx, vy; p != q {
|
|
if p == nil {
|
|
p = &CacheOptionsEntry{}
|
|
}
|
|
if q == nil {
|
|
q = &CacheOptionsEntry{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
}
|
|
if this.CgroupParent != that.CgroupParent {
|
|
return false
|
|
}
|
|
if len(this.Exports) != len(that.Exports) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Exports {
|
|
vy := that.Exports[i]
|
|
if p, q := vx, vy; p != q {
|
|
if p == nil {
|
|
p = &ExportEntry{}
|
|
}
|
|
if q == nil {
|
|
q = &ExportEntry{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
}
|
|
if len(this.ExtraHosts) != len(that.ExtraHosts) {
|
|
return false
|
|
}
|
|
for i, vx := range this.ExtraHosts {
|
|
vy := that.ExtraHosts[i]
|
|
if vx != vy {
|
|
return false
|
|
}
|
|
}
|
|
if len(this.Labels) != len(that.Labels) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Labels {
|
|
vy, ok := that.Labels[i]
|
|
if !ok {
|
|
return false
|
|
}
|
|
if vx != vy {
|
|
return false
|
|
}
|
|
}
|
|
if this.NetworkMode != that.NetworkMode {
|
|
return false
|
|
}
|
|
if len(this.NoCacheFilter) != len(that.NoCacheFilter) {
|
|
return false
|
|
}
|
|
for i, vx := range this.NoCacheFilter {
|
|
vy := that.NoCacheFilter[i]
|
|
if vx != vy {
|
|
return false
|
|
}
|
|
}
|
|
if len(this.Platforms) != len(that.Platforms) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Platforms {
|
|
vy := that.Platforms[i]
|
|
if vx != vy {
|
|
return false
|
|
}
|
|
}
|
|
if len(this.Secrets) != len(that.Secrets) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Secrets {
|
|
vy := that.Secrets[i]
|
|
if p, q := vx, vy; p != q {
|
|
if p == nil {
|
|
p = &Secret{}
|
|
}
|
|
if q == nil {
|
|
q = &Secret{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
}
|
|
if this.ShmSize != that.ShmSize {
|
|
return false
|
|
}
|
|
if len(this.SSH) != len(that.SSH) {
|
|
return false
|
|
}
|
|
for i, vx := range this.SSH {
|
|
vy := that.SSH[i]
|
|
if p, q := vx, vy; p != q {
|
|
if p == nil {
|
|
p = &SSH{}
|
|
}
|
|
if q == nil {
|
|
q = &SSH{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
}
|
|
if len(this.Tags) != len(that.Tags) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Tags {
|
|
vy := that.Tags[i]
|
|
if vx != vy {
|
|
return false
|
|
}
|
|
}
|
|
if this.Target != that.Target {
|
|
return false
|
|
}
|
|
if !this.Ulimits.EqualVT(that.Ulimits) {
|
|
return false
|
|
}
|
|
if this.Builder != that.Builder {
|
|
return false
|
|
}
|
|
if this.NoCache != that.NoCache {
|
|
return false
|
|
}
|
|
if this.Pull != that.Pull {
|
|
return false
|
|
}
|
|
if this.ExportPush != that.ExportPush {
|
|
return false
|
|
}
|
|
if this.ExportLoad != that.ExportLoad {
|
|
return false
|
|
}
|
|
if equal, ok := interface{}(this.SourcePolicy).(interface{ EqualVT(*pb.Policy) bool }); ok {
|
|
if !equal.EqualVT(that.SourcePolicy) {
|
|
return false
|
|
}
|
|
} else if !proto.Equal(this.SourcePolicy, that.SourcePolicy) {
|
|
return false
|
|
}
|
|
if this.Ref != that.Ref {
|
|
return false
|
|
}
|
|
if this.GroupRef != that.GroupRef {
|
|
return false
|
|
}
|
|
if len(this.Annotations) != len(that.Annotations) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Annotations {
|
|
vy := that.Annotations[i]
|
|
if vx != vy {
|
|
return false
|
|
}
|
|
}
|
|
if this.ProvenanceResponseMode != that.ProvenanceResponseMode {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *BuildOptions) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*BuildOptions)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *ExportEntry) EqualVT(that *ExportEntry) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Type != that.Type {
|
|
return false
|
|
}
|
|
if len(this.Attrs) != len(that.Attrs) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Attrs {
|
|
vy, ok := that.Attrs[i]
|
|
if !ok {
|
|
return false
|
|
}
|
|
if vx != vy {
|
|
return false
|
|
}
|
|
}
|
|
if this.Destination != that.Destination {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *ExportEntry) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*ExportEntry)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *CacheOptionsEntry) EqualVT(that *CacheOptionsEntry) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Type != that.Type {
|
|
return false
|
|
}
|
|
if len(this.Attrs) != len(that.Attrs) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Attrs {
|
|
vy, ok := that.Attrs[i]
|
|
if !ok {
|
|
return false
|
|
}
|
|
if vx != vy {
|
|
return false
|
|
}
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *CacheOptionsEntry) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*CacheOptionsEntry)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *Attest) EqualVT(that *Attest) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Type != that.Type {
|
|
return false
|
|
}
|
|
if this.Disabled != that.Disabled {
|
|
return false
|
|
}
|
|
if this.Attrs != that.Attrs {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *Attest) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*Attest)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *SSH) EqualVT(that *SSH) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.ID != that.ID {
|
|
return false
|
|
}
|
|
if len(this.Paths) != len(that.Paths) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Paths {
|
|
vy := that.Paths[i]
|
|
if vx != vy {
|
|
return false
|
|
}
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *SSH) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*SSH)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *Secret) EqualVT(that *Secret) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.ID != that.ID {
|
|
return false
|
|
}
|
|
if this.FilePath != that.FilePath {
|
|
return false
|
|
}
|
|
if this.Env != that.Env {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *Secret) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*Secret)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *CallFunc) EqualVT(that *CallFunc) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Name != that.Name {
|
|
return false
|
|
}
|
|
if this.Format != that.Format {
|
|
return false
|
|
}
|
|
if this.IgnoreStatus != that.IgnoreStatus {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *CallFunc) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*CallFunc)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *InspectRequest) EqualVT(that *InspectRequest) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Ref != that.Ref {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *InspectRequest) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*InspectRequest)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *InspectResponse) EqualVT(that *InspectResponse) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if !this.Options.EqualVT(that.Options) {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *InspectResponse) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*InspectResponse)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *UlimitOpt) EqualVT(that *UlimitOpt) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if len(this.Values) != len(that.Values) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Values {
|
|
vy, ok := that.Values[i]
|
|
if !ok {
|
|
return false
|
|
}
|
|
if p, q := vx, vy; p != q {
|
|
if p == nil {
|
|
p = &Ulimit{}
|
|
}
|
|
if q == nil {
|
|
q = &Ulimit{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *UlimitOpt) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*UlimitOpt)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *Ulimit) EqualVT(that *Ulimit) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Name != that.Name {
|
|
return false
|
|
}
|
|
if this.Hard != that.Hard {
|
|
return false
|
|
}
|
|
if this.Soft != that.Soft {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *Ulimit) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*Ulimit)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *BuildResponse) EqualVT(that *BuildResponse) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if len(this.ExporterResponse) != len(that.ExporterResponse) {
|
|
return false
|
|
}
|
|
for i, vx := range this.ExporterResponse {
|
|
vy, ok := that.ExporterResponse[i]
|
|
if !ok {
|
|
return false
|
|
}
|
|
if vx != vy {
|
|
return false
|
|
}
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *BuildResponse) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*BuildResponse)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *DisconnectRequest) EqualVT(that *DisconnectRequest) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Ref != that.Ref {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *DisconnectRequest) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*DisconnectRequest)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *DisconnectResponse) EqualVT(that *DisconnectResponse) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *DisconnectResponse) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*DisconnectResponse)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *ListRequest) EqualVT(that *ListRequest) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Ref != that.Ref {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *ListRequest) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*ListRequest)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *ListResponse) EqualVT(that *ListResponse) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if len(this.Keys) != len(that.Keys) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Keys {
|
|
vy := that.Keys[i]
|
|
if vx != vy {
|
|
return false
|
|
}
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *ListResponse) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*ListResponse)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *InputMessage) EqualVT(that *InputMessage) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Input == nil && that.Input != nil {
|
|
return false
|
|
} else if this.Input != nil {
|
|
if that.Input == nil {
|
|
return false
|
|
}
|
|
if !this.Input.(interface {
|
|
EqualVT(isInputMessage_Input) bool
|
|
}).EqualVT(that.Input) {
|
|
return false
|
|
}
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *InputMessage) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*InputMessage)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *InputMessage_Init) EqualVT(thatIface isInputMessage_Input) bool {
|
|
that, ok := thatIface.(*InputMessage_Init)
|
|
if !ok {
|
|
return false
|
|
}
|
|
if this == that {
|
|
return true
|
|
}
|
|
if this == nil && that != nil || this != nil && that == nil {
|
|
return false
|
|
}
|
|
if p, q := this.Init, that.Init; p != q {
|
|
if p == nil {
|
|
p = &InputInitMessage{}
|
|
}
|
|
if q == nil {
|
|
q = &InputInitMessage{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (this *InputMessage_Data) EqualVT(thatIface isInputMessage_Input) bool {
|
|
that, ok := thatIface.(*InputMessage_Data)
|
|
if !ok {
|
|
return false
|
|
}
|
|
if this == that {
|
|
return true
|
|
}
|
|
if this == nil && that != nil || this != nil && that == nil {
|
|
return false
|
|
}
|
|
if p, q := this.Data, that.Data; p != q {
|
|
if p == nil {
|
|
p = &DataMessage{}
|
|
}
|
|
if q == nil {
|
|
q = &DataMessage{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (this *InputInitMessage) EqualVT(that *InputInitMessage) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Ref != that.Ref {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *InputInitMessage) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*InputInitMessage)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *DataMessage) EqualVT(that *DataMessage) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.EOF != that.EOF {
|
|
return false
|
|
}
|
|
if string(this.Data) != string(that.Data) {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *DataMessage) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*DataMessage)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *InputResponse) EqualVT(that *InputResponse) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *InputResponse) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*InputResponse)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *Message) EqualVT(that *Message) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Input == nil && that.Input != nil {
|
|
return false
|
|
} else if this.Input != nil {
|
|
if that.Input == nil {
|
|
return false
|
|
}
|
|
if !this.Input.(interface{ EqualVT(isMessage_Input) bool }).EqualVT(that.Input) {
|
|
return false
|
|
}
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *Message) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*Message)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *Message_Init) EqualVT(thatIface isMessage_Input) bool {
|
|
that, ok := thatIface.(*Message_Init)
|
|
if !ok {
|
|
return false
|
|
}
|
|
if this == that {
|
|
return true
|
|
}
|
|
if this == nil && that != nil || this != nil && that == nil {
|
|
return false
|
|
}
|
|
if p, q := this.Init, that.Init; p != q {
|
|
if p == nil {
|
|
p = &InitMessage{}
|
|
}
|
|
if q == nil {
|
|
q = &InitMessage{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (this *Message_File) EqualVT(thatIface isMessage_Input) bool {
|
|
that, ok := thatIface.(*Message_File)
|
|
if !ok {
|
|
return false
|
|
}
|
|
if this == that {
|
|
return true
|
|
}
|
|
if this == nil && that != nil || this != nil && that == nil {
|
|
return false
|
|
}
|
|
if p, q := this.File, that.File; p != q {
|
|
if p == nil {
|
|
p = &FdMessage{}
|
|
}
|
|
if q == nil {
|
|
q = &FdMessage{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (this *Message_Resize) EqualVT(thatIface isMessage_Input) bool {
|
|
that, ok := thatIface.(*Message_Resize)
|
|
if !ok {
|
|
return false
|
|
}
|
|
if this == that {
|
|
return true
|
|
}
|
|
if this == nil && that != nil || this != nil && that == nil {
|
|
return false
|
|
}
|
|
if p, q := this.Resize, that.Resize; p != q {
|
|
if p == nil {
|
|
p = &ResizeMessage{}
|
|
}
|
|
if q == nil {
|
|
q = &ResizeMessage{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (this *Message_Signal) EqualVT(thatIface isMessage_Input) bool {
|
|
that, ok := thatIface.(*Message_Signal)
|
|
if !ok {
|
|
return false
|
|
}
|
|
if this == that {
|
|
return true
|
|
}
|
|
if this == nil && that != nil || this != nil && that == nil {
|
|
return false
|
|
}
|
|
if p, q := this.Signal, that.Signal; p != q {
|
|
if p == nil {
|
|
p = &SignalMessage{}
|
|
}
|
|
if q == nil {
|
|
q = &SignalMessage{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (this *InitMessage) EqualVT(that *InitMessage) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Ref != that.Ref {
|
|
return false
|
|
}
|
|
if this.ProcessID != that.ProcessID {
|
|
return false
|
|
}
|
|
if !this.InvokeConfig.EqualVT(that.InvokeConfig) {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *InitMessage) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*InitMessage)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *InvokeConfig) EqualVT(that *InvokeConfig) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if len(this.Entrypoint) != len(that.Entrypoint) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Entrypoint {
|
|
vy := that.Entrypoint[i]
|
|
if vx != vy {
|
|
return false
|
|
}
|
|
}
|
|
if len(this.Cmd) != len(that.Cmd) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Cmd {
|
|
vy := that.Cmd[i]
|
|
if vx != vy {
|
|
return false
|
|
}
|
|
}
|
|
if len(this.Env) != len(that.Env) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Env {
|
|
vy := that.Env[i]
|
|
if vx != vy {
|
|
return false
|
|
}
|
|
}
|
|
if this.User != that.User {
|
|
return false
|
|
}
|
|
if this.NoUser != that.NoUser {
|
|
return false
|
|
}
|
|
if this.Cwd != that.Cwd {
|
|
return false
|
|
}
|
|
if this.NoCwd != that.NoCwd {
|
|
return false
|
|
}
|
|
if this.Tty != that.Tty {
|
|
return false
|
|
}
|
|
if this.Rollback != that.Rollback {
|
|
return false
|
|
}
|
|
if this.Initial != that.Initial {
|
|
return false
|
|
}
|
|
if this.NoCmd != that.NoCmd {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *InvokeConfig) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*InvokeConfig)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *FdMessage) EqualVT(that *FdMessage) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Fd != that.Fd {
|
|
return false
|
|
}
|
|
if this.EOF != that.EOF {
|
|
return false
|
|
}
|
|
if string(this.Data) != string(that.Data) {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *FdMessage) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*FdMessage)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *ResizeMessage) EqualVT(that *ResizeMessage) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Rows != that.Rows {
|
|
return false
|
|
}
|
|
if this.Cols != that.Cols {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *ResizeMessage) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*ResizeMessage)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *SignalMessage) EqualVT(that *SignalMessage) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Name != that.Name {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *SignalMessage) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*SignalMessage)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *StatusRequest) EqualVT(that *StatusRequest) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Ref != that.Ref {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *StatusRequest) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*StatusRequest)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *StatusResponse) EqualVT(that *StatusResponse) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if len(this.Vertexes) != len(that.Vertexes) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Vertexes {
|
|
vy := that.Vertexes[i]
|
|
if p, q := vx, vy; p != q {
|
|
if p == nil {
|
|
p = &control.Vertex{}
|
|
}
|
|
if q == nil {
|
|
q = &control.Vertex{}
|
|
}
|
|
if equal, ok := interface{}(p).(interface{ EqualVT(*control.Vertex) bool }); ok {
|
|
if !equal.EqualVT(q) {
|
|
return false
|
|
}
|
|
} else if !proto.Equal(p, q) {
|
|
return false
|
|
}
|
|
}
|
|
}
|
|
if len(this.Statuses) != len(that.Statuses) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Statuses {
|
|
vy := that.Statuses[i]
|
|
if p, q := vx, vy; p != q {
|
|
if p == nil {
|
|
p = &control.VertexStatus{}
|
|
}
|
|
if q == nil {
|
|
q = &control.VertexStatus{}
|
|
}
|
|
if equal, ok := interface{}(p).(interface {
|
|
EqualVT(*control.VertexStatus) bool
|
|
}); ok {
|
|
if !equal.EqualVT(q) {
|
|
return false
|
|
}
|
|
} else if !proto.Equal(p, q) {
|
|
return false
|
|
}
|
|
}
|
|
}
|
|
if len(this.Logs) != len(that.Logs) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Logs {
|
|
vy := that.Logs[i]
|
|
if p, q := vx, vy; p != q {
|
|
if p == nil {
|
|
p = &control.VertexLog{}
|
|
}
|
|
if q == nil {
|
|
q = &control.VertexLog{}
|
|
}
|
|
if equal, ok := interface{}(p).(interface{ EqualVT(*control.VertexLog) bool }); ok {
|
|
if !equal.EqualVT(q) {
|
|
return false
|
|
}
|
|
} else if !proto.Equal(p, q) {
|
|
return false
|
|
}
|
|
}
|
|
}
|
|
if len(this.Warnings) != len(that.Warnings) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Warnings {
|
|
vy := that.Warnings[i]
|
|
if p, q := vx, vy; p != q {
|
|
if p == nil {
|
|
p = &control.VertexWarning{}
|
|
}
|
|
if q == nil {
|
|
q = &control.VertexWarning{}
|
|
}
|
|
if equal, ok := interface{}(p).(interface {
|
|
EqualVT(*control.VertexWarning) bool
|
|
}); ok {
|
|
if !equal.EqualVT(q) {
|
|
return false
|
|
}
|
|
} else if !proto.Equal(p, q) {
|
|
return false
|
|
}
|
|
}
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *StatusResponse) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*StatusResponse)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *InfoRequest) EqualVT(that *InfoRequest) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *InfoRequest) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*InfoRequest)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *InfoResponse) EqualVT(that *InfoResponse) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if !this.BuildxVersion.EqualVT(that.BuildxVersion) {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *InfoResponse) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*InfoResponse)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *BuildxVersion) EqualVT(that *BuildxVersion) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Package != that.Package {
|
|
return false
|
|
}
|
|
if this.Version != that.Version {
|
|
return false
|
|
}
|
|
if this.Revision != that.Revision {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *BuildxVersion) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*BuildxVersion)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (m *ListProcessesRequest) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *ListProcessesRequest) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *ListProcessesRequest) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.Ref) > 0 {
|
|
i -= len(m.Ref)
|
|
copy(dAtA[i:], m.Ref)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Ref)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *ListProcessesResponse) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *ListProcessesResponse) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *ListProcessesResponse) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.Infos) > 0 {
|
|
for iNdEx := len(m.Infos) - 1; iNdEx >= 0; iNdEx-- {
|
|
size, err := m.Infos[iNdEx].MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *ProcessInfo) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *ProcessInfo) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *ProcessInfo) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.InvokeConfig != nil {
|
|
size, err := m.InvokeConfig.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
if len(m.ProcessID) > 0 {
|
|
i -= len(m.ProcessID)
|
|
copy(dAtA[i:], m.ProcessID)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.ProcessID)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *DisconnectProcessRequest) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *DisconnectProcessRequest) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *DisconnectProcessRequest) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.ProcessID) > 0 {
|
|
i -= len(m.ProcessID)
|
|
copy(dAtA[i:], m.ProcessID)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.ProcessID)))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
if len(m.Ref) > 0 {
|
|
i -= len(m.Ref)
|
|
copy(dAtA[i:], m.Ref)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Ref)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *DisconnectProcessResponse) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *DisconnectProcessResponse) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *DisconnectProcessResponse) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *BuildRequest) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *BuildRequest) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *BuildRequest) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.Options != nil {
|
|
size, err := m.Options.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
if len(m.Ref) > 0 {
|
|
i -= len(m.Ref)
|
|
copy(dAtA[i:], m.Ref)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Ref)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *BuildOptions) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *BuildOptions) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *BuildOptions) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.ProvenanceResponseMode) > 0 {
|
|
i -= len(m.ProvenanceResponseMode)
|
|
copy(dAtA[i:], m.ProvenanceResponseMode)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.ProvenanceResponseMode)))
|
|
i--
|
|
dAtA[i] = 0x2
|
|
i--
|
|
dAtA[i] = 0x82
|
|
}
|
|
if len(m.Annotations) > 0 {
|
|
for iNdEx := len(m.Annotations) - 1; iNdEx >= 0; iNdEx-- {
|
|
i -= len(m.Annotations[iNdEx])
|
|
copy(dAtA[i:], m.Annotations[iNdEx])
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Annotations[iNdEx])))
|
|
i--
|
|
dAtA[i] = 0x1
|
|
i--
|
|
dAtA[i] = 0xfa
|
|
}
|
|
}
|
|
if len(m.GroupRef) > 0 {
|
|
i -= len(m.GroupRef)
|
|
copy(dAtA[i:], m.GroupRef)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.GroupRef)))
|
|
i--
|
|
dAtA[i] = 0x1
|
|
i--
|
|
dAtA[i] = 0xf2
|
|
}
|
|
if len(m.Ref) > 0 {
|
|
i -= len(m.Ref)
|
|
copy(dAtA[i:], m.Ref)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Ref)))
|
|
i--
|
|
dAtA[i] = 0x1
|
|
i--
|
|
dAtA[i] = 0xea
|
|
}
|
|
if m.SourcePolicy != nil {
|
|
if vtmsg, ok := interface{}(m.SourcePolicy).(interface {
|
|
MarshalToSizedBufferVT([]byte) (int, error)
|
|
}); ok {
|
|
size, err := vtmsg.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
} else {
|
|
encoded, err := proto.Marshal(m.SourcePolicy)
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= len(encoded)
|
|
copy(dAtA[i:], encoded)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(encoded)))
|
|
}
|
|
i--
|
|
dAtA[i] = 0x1
|
|
i--
|
|
dAtA[i] = 0xe2
|
|
}
|
|
if m.ExportLoad {
|
|
i--
|
|
if m.ExportLoad {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x1
|
|
i--
|
|
dAtA[i] = 0xd8
|
|
}
|
|
if m.ExportPush {
|
|
i--
|
|
if m.ExportPush {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x1
|
|
i--
|
|
dAtA[i] = 0xd0
|
|
}
|
|
if m.Pull {
|
|
i--
|
|
if m.Pull {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x1
|
|
i--
|
|
dAtA[i] = 0xc8
|
|
}
|
|
if m.NoCache {
|
|
i--
|
|
if m.NoCache {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x1
|
|
i--
|
|
dAtA[i] = 0xc0
|
|
}
|
|
if len(m.Builder) > 0 {
|
|
i -= len(m.Builder)
|
|
copy(dAtA[i:], m.Builder)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Builder)))
|
|
i--
|
|
dAtA[i] = 0x1
|
|
i--
|
|
dAtA[i] = 0xba
|
|
}
|
|
if m.Ulimits != nil {
|
|
size, err := m.Ulimits.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x1
|
|
i--
|
|
dAtA[i] = 0xb2
|
|
}
|
|
if len(m.Target) > 0 {
|
|
i -= len(m.Target)
|
|
copy(dAtA[i:], m.Target)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Target)))
|
|
i--
|
|
dAtA[i] = 0x1
|
|
i--
|
|
dAtA[i] = 0xaa
|
|
}
|
|
if len(m.Tags) > 0 {
|
|
for iNdEx := len(m.Tags) - 1; iNdEx >= 0; iNdEx-- {
|
|
i -= len(m.Tags[iNdEx])
|
|
copy(dAtA[i:], m.Tags[iNdEx])
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Tags[iNdEx])))
|
|
i--
|
|
dAtA[i] = 0x1
|
|
i--
|
|
dAtA[i] = 0xa2
|
|
}
|
|
}
|
|
if len(m.SSH) > 0 {
|
|
for iNdEx := len(m.SSH) - 1; iNdEx >= 0; iNdEx-- {
|
|
size, err := m.SSH[iNdEx].MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x1
|
|
i--
|
|
dAtA[i] = 0x9a
|
|
}
|
|
}
|
|
if m.ShmSize != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.ShmSize))
|
|
i--
|
|
dAtA[i] = 0x1
|
|
i--
|
|
dAtA[i] = 0x90
|
|
}
|
|
if len(m.Secrets) > 0 {
|
|
for iNdEx := len(m.Secrets) - 1; iNdEx >= 0; iNdEx-- {
|
|
size, err := m.Secrets[iNdEx].MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x1
|
|
i--
|
|
dAtA[i] = 0x8a
|
|
}
|
|
}
|
|
if len(m.Platforms) > 0 {
|
|
for iNdEx := len(m.Platforms) - 1; iNdEx >= 0; iNdEx-- {
|
|
i -= len(m.Platforms[iNdEx])
|
|
copy(dAtA[i:], m.Platforms[iNdEx])
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Platforms[iNdEx])))
|
|
i--
|
|
dAtA[i] = 0x1
|
|
i--
|
|
dAtA[i] = 0x82
|
|
}
|
|
}
|
|
if len(m.NoCacheFilter) > 0 {
|
|
for iNdEx := len(m.NoCacheFilter) - 1; iNdEx >= 0; iNdEx-- {
|
|
i -= len(m.NoCacheFilter[iNdEx])
|
|
copy(dAtA[i:], m.NoCacheFilter[iNdEx])
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.NoCacheFilter[iNdEx])))
|
|
i--
|
|
dAtA[i] = 0x7a
|
|
}
|
|
}
|
|
if len(m.NetworkMode) > 0 {
|
|
i -= len(m.NetworkMode)
|
|
copy(dAtA[i:], m.NetworkMode)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.NetworkMode)))
|
|
i--
|
|
dAtA[i] = 0x72
|
|
}
|
|
if len(m.Labels) > 0 {
|
|
for k := range m.Labels {
|
|
v := m.Labels[k]
|
|
baseI := i
|
|
i -= len(v)
|
|
copy(dAtA[i:], v)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(v)))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
i -= len(k)
|
|
copy(dAtA[i:], k)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(k)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(baseI-i))
|
|
i--
|
|
dAtA[i] = 0x6a
|
|
}
|
|
}
|
|
if len(m.ExtraHosts) > 0 {
|
|
for iNdEx := len(m.ExtraHosts) - 1; iNdEx >= 0; iNdEx-- {
|
|
i -= len(m.ExtraHosts[iNdEx])
|
|
copy(dAtA[i:], m.ExtraHosts[iNdEx])
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.ExtraHosts[iNdEx])))
|
|
i--
|
|
dAtA[i] = 0x62
|
|
}
|
|
}
|
|
if len(m.Exports) > 0 {
|
|
for iNdEx := len(m.Exports) - 1; iNdEx >= 0; iNdEx-- {
|
|
size, err := m.Exports[iNdEx].MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x5a
|
|
}
|
|
}
|
|
if len(m.CgroupParent) > 0 {
|
|
i -= len(m.CgroupParent)
|
|
copy(dAtA[i:], m.CgroupParent)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.CgroupParent)))
|
|
i--
|
|
dAtA[i] = 0x52
|
|
}
|
|
if len(m.CacheTo) > 0 {
|
|
for iNdEx := len(m.CacheTo) - 1; iNdEx >= 0; iNdEx-- {
|
|
size, err := m.CacheTo[iNdEx].MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x4a
|
|
}
|
|
}
|
|
if len(m.CacheFrom) > 0 {
|
|
for iNdEx := len(m.CacheFrom) - 1; iNdEx >= 0; iNdEx-- {
|
|
size, err := m.CacheFrom[iNdEx].MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x42
|
|
}
|
|
}
|
|
if len(m.BuildArgs) > 0 {
|
|
for k := range m.BuildArgs {
|
|
v := m.BuildArgs[k]
|
|
baseI := i
|
|
i -= len(v)
|
|
copy(dAtA[i:], v)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(v)))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
i -= len(k)
|
|
copy(dAtA[i:], k)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(k)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(baseI-i))
|
|
i--
|
|
dAtA[i] = 0x3a
|
|
}
|
|
}
|
|
if len(m.Attests) > 0 {
|
|
for iNdEx := len(m.Attests) - 1; iNdEx >= 0; iNdEx-- {
|
|
size, err := m.Attests[iNdEx].MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x32
|
|
}
|
|
}
|
|
if len(m.Allow) > 0 {
|
|
for iNdEx := len(m.Allow) - 1; iNdEx >= 0; iNdEx-- {
|
|
i -= len(m.Allow[iNdEx])
|
|
copy(dAtA[i:], m.Allow[iNdEx])
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Allow[iNdEx])))
|
|
i--
|
|
dAtA[i] = 0x2a
|
|
}
|
|
}
|
|
if len(m.NamedContexts) > 0 {
|
|
for k := range m.NamedContexts {
|
|
v := m.NamedContexts[k]
|
|
baseI := i
|
|
i -= len(v)
|
|
copy(dAtA[i:], v)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(v)))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
i -= len(k)
|
|
copy(dAtA[i:], k)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(k)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(baseI-i))
|
|
i--
|
|
dAtA[i] = 0x22
|
|
}
|
|
}
|
|
if m.CallFunc != nil {
|
|
size, err := m.CallFunc.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x1a
|
|
}
|
|
if len(m.DockerfileName) > 0 {
|
|
i -= len(m.DockerfileName)
|
|
copy(dAtA[i:], m.DockerfileName)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.DockerfileName)))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
if len(m.ContextPath) > 0 {
|
|
i -= len(m.ContextPath)
|
|
copy(dAtA[i:], m.ContextPath)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.ContextPath)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *ExportEntry) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *ExportEntry) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *ExportEntry) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.Destination) > 0 {
|
|
i -= len(m.Destination)
|
|
copy(dAtA[i:], m.Destination)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Destination)))
|
|
i--
|
|
dAtA[i] = 0x1a
|
|
}
|
|
if len(m.Attrs) > 0 {
|
|
for k := range m.Attrs {
|
|
v := m.Attrs[k]
|
|
baseI := i
|
|
i -= len(v)
|
|
copy(dAtA[i:], v)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(v)))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
i -= len(k)
|
|
copy(dAtA[i:], k)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(k)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(baseI-i))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
}
|
|
if len(m.Type) > 0 {
|
|
i -= len(m.Type)
|
|
copy(dAtA[i:], m.Type)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Type)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *CacheOptionsEntry) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *CacheOptionsEntry) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *CacheOptionsEntry) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.Attrs) > 0 {
|
|
for k := range m.Attrs {
|
|
v := m.Attrs[k]
|
|
baseI := i
|
|
i -= len(v)
|
|
copy(dAtA[i:], v)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(v)))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
i -= len(k)
|
|
copy(dAtA[i:], k)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(k)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(baseI-i))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
}
|
|
if len(m.Type) > 0 {
|
|
i -= len(m.Type)
|
|
copy(dAtA[i:], m.Type)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Type)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *Attest) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *Attest) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *Attest) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.Attrs) > 0 {
|
|
i -= len(m.Attrs)
|
|
copy(dAtA[i:], m.Attrs)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Attrs)))
|
|
i--
|
|
dAtA[i] = 0x1a
|
|
}
|
|
if m.Disabled {
|
|
i--
|
|
if m.Disabled {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x10
|
|
}
|
|
if len(m.Type) > 0 {
|
|
i -= len(m.Type)
|
|
copy(dAtA[i:], m.Type)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Type)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *SSH) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *SSH) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *SSH) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.Paths) > 0 {
|
|
for iNdEx := len(m.Paths) - 1; iNdEx >= 0; iNdEx-- {
|
|
i -= len(m.Paths[iNdEx])
|
|
copy(dAtA[i:], m.Paths[iNdEx])
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Paths[iNdEx])))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
}
|
|
if len(m.ID) > 0 {
|
|
i -= len(m.ID)
|
|
copy(dAtA[i:], m.ID)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.ID)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *Secret) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *Secret) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *Secret) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.Env) > 0 {
|
|
i -= len(m.Env)
|
|
copy(dAtA[i:], m.Env)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Env)))
|
|
i--
|
|
dAtA[i] = 0x1a
|
|
}
|
|
if len(m.FilePath) > 0 {
|
|
i -= len(m.FilePath)
|
|
copy(dAtA[i:], m.FilePath)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.FilePath)))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
if len(m.ID) > 0 {
|
|
i -= len(m.ID)
|
|
copy(dAtA[i:], m.ID)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.ID)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *CallFunc) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *CallFunc) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *CallFunc) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.IgnoreStatus {
|
|
i--
|
|
if m.IgnoreStatus {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x18
|
|
}
|
|
if len(m.Format) > 0 {
|
|
i -= len(m.Format)
|
|
copy(dAtA[i:], m.Format)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Format)))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
if len(m.Name) > 0 {
|
|
i -= len(m.Name)
|
|
copy(dAtA[i:], m.Name)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Name)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *InspectRequest) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *InspectRequest) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *InspectRequest) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.Ref) > 0 {
|
|
i -= len(m.Ref)
|
|
copy(dAtA[i:], m.Ref)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Ref)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *InspectResponse) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *InspectResponse) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *InspectResponse) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.Options != nil {
|
|
size, err := m.Options.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *UlimitOpt) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *UlimitOpt) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *UlimitOpt) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.Values) > 0 {
|
|
for k := range m.Values {
|
|
v := m.Values[k]
|
|
baseI := i
|
|
size, err := v.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
i -= len(k)
|
|
copy(dAtA[i:], k)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(k)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(baseI-i))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *Ulimit) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *Ulimit) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *Ulimit) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.Soft != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Soft))
|
|
i--
|
|
dAtA[i] = 0x18
|
|
}
|
|
if m.Hard != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Hard))
|
|
i--
|
|
dAtA[i] = 0x10
|
|
}
|
|
if len(m.Name) > 0 {
|
|
i -= len(m.Name)
|
|
copy(dAtA[i:], m.Name)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Name)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *BuildResponse) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *BuildResponse) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *BuildResponse) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.ExporterResponse) > 0 {
|
|
for k := range m.ExporterResponse {
|
|
v := m.ExporterResponse[k]
|
|
baseI := i
|
|
i -= len(v)
|
|
copy(dAtA[i:], v)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(v)))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
i -= len(k)
|
|
copy(dAtA[i:], k)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(k)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(baseI-i))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *DisconnectRequest) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *DisconnectRequest) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *DisconnectRequest) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.Ref) > 0 {
|
|
i -= len(m.Ref)
|
|
copy(dAtA[i:], m.Ref)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Ref)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *DisconnectResponse) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *DisconnectResponse) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *DisconnectResponse) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *ListRequest) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *ListRequest) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *ListRequest) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.Ref) > 0 {
|
|
i -= len(m.Ref)
|
|
copy(dAtA[i:], m.Ref)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Ref)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *ListResponse) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *ListResponse) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *ListResponse) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.Keys) > 0 {
|
|
for iNdEx := len(m.Keys) - 1; iNdEx >= 0; iNdEx-- {
|
|
i -= len(m.Keys[iNdEx])
|
|
copy(dAtA[i:], m.Keys[iNdEx])
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Keys[iNdEx])))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *InputMessage) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *InputMessage) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *InputMessage) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if vtmsg, ok := m.Input.(interface {
|
|
MarshalToSizedBufferVT([]byte) (int, error)
|
|
}); ok {
|
|
size, err := vtmsg.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *InputMessage_Init) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *InputMessage_Init) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
i := len(dAtA)
|
|
if m.Init != nil {
|
|
size, err := m.Init.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
} else {
|
|
i = protohelpers.EncodeVarint(dAtA, i, 0)
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
func (m *InputMessage_Data) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *InputMessage_Data) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
i := len(dAtA)
|
|
if m.Data != nil {
|
|
size, err := m.Data.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
} else {
|
|
i = protohelpers.EncodeVarint(dAtA, i, 0)
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
func (m *InputInitMessage) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *InputInitMessage) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *InputInitMessage) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.Ref) > 0 {
|
|
i -= len(m.Ref)
|
|
copy(dAtA[i:], m.Ref)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Ref)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *DataMessage) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *DataMessage) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *DataMessage) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.Data) > 0 {
|
|
i -= len(m.Data)
|
|
copy(dAtA[i:], m.Data)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Data)))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
if m.EOF {
|
|
i--
|
|
if m.EOF {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x8
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *InputResponse) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *InputResponse) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *InputResponse) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *Message) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *Message) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *Message) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if vtmsg, ok := m.Input.(interface {
|
|
MarshalToSizedBufferVT([]byte) (int, error)
|
|
}); ok {
|
|
size, err := vtmsg.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *Message_Init) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *Message_Init) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
i := len(dAtA)
|
|
if m.Init != nil {
|
|
size, err := m.Init.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
} else {
|
|
i = protohelpers.EncodeVarint(dAtA, i, 0)
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
func (m *Message_File) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *Message_File) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
i := len(dAtA)
|
|
if m.File != nil {
|
|
size, err := m.File.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
} else {
|
|
i = protohelpers.EncodeVarint(dAtA, i, 0)
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
func (m *Message_Resize) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *Message_Resize) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
i := len(dAtA)
|
|
if m.Resize != nil {
|
|
size, err := m.Resize.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x1a
|
|
} else {
|
|
i = protohelpers.EncodeVarint(dAtA, i, 0)
|
|
i--
|
|
dAtA[i] = 0x1a
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
func (m *Message_Signal) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *Message_Signal) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
i := len(dAtA)
|
|
if m.Signal != nil {
|
|
size, err := m.Signal.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x22
|
|
} else {
|
|
i = protohelpers.EncodeVarint(dAtA, i, 0)
|
|
i--
|
|
dAtA[i] = 0x22
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
func (m *InitMessage) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *InitMessage) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *InitMessage) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.InvokeConfig != nil {
|
|
size, err := m.InvokeConfig.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x1a
|
|
}
|
|
if len(m.ProcessID) > 0 {
|
|
i -= len(m.ProcessID)
|
|
copy(dAtA[i:], m.ProcessID)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.ProcessID)))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
if len(m.Ref) > 0 {
|
|
i -= len(m.Ref)
|
|
copy(dAtA[i:], m.Ref)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Ref)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *InvokeConfig) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *InvokeConfig) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *InvokeConfig) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.NoCmd {
|
|
i--
|
|
if m.NoCmd {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x58
|
|
}
|
|
if m.Initial {
|
|
i--
|
|
if m.Initial {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x50
|
|
}
|
|
if m.Rollback {
|
|
i--
|
|
if m.Rollback {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x48
|
|
}
|
|
if m.Tty {
|
|
i--
|
|
if m.Tty {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x40
|
|
}
|
|
if m.NoCwd {
|
|
i--
|
|
if m.NoCwd {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x38
|
|
}
|
|
if len(m.Cwd) > 0 {
|
|
i -= len(m.Cwd)
|
|
copy(dAtA[i:], m.Cwd)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Cwd)))
|
|
i--
|
|
dAtA[i] = 0x32
|
|
}
|
|
if m.NoUser {
|
|
i--
|
|
if m.NoUser {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x28
|
|
}
|
|
if len(m.User) > 0 {
|
|
i -= len(m.User)
|
|
copy(dAtA[i:], m.User)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.User)))
|
|
i--
|
|
dAtA[i] = 0x22
|
|
}
|
|
if len(m.Env) > 0 {
|
|
for iNdEx := len(m.Env) - 1; iNdEx >= 0; iNdEx-- {
|
|
i -= len(m.Env[iNdEx])
|
|
copy(dAtA[i:], m.Env[iNdEx])
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Env[iNdEx])))
|
|
i--
|
|
dAtA[i] = 0x1a
|
|
}
|
|
}
|
|
if len(m.Cmd) > 0 {
|
|
for iNdEx := len(m.Cmd) - 1; iNdEx >= 0; iNdEx-- {
|
|
i -= len(m.Cmd[iNdEx])
|
|
copy(dAtA[i:], m.Cmd[iNdEx])
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Cmd[iNdEx])))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
}
|
|
if len(m.Entrypoint) > 0 {
|
|
for iNdEx := len(m.Entrypoint) - 1; iNdEx >= 0; iNdEx-- {
|
|
i -= len(m.Entrypoint[iNdEx])
|
|
copy(dAtA[i:], m.Entrypoint[iNdEx])
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Entrypoint[iNdEx])))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *FdMessage) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *FdMessage) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *FdMessage) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.Data) > 0 {
|
|
i -= len(m.Data)
|
|
copy(dAtA[i:], m.Data)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Data)))
|
|
i--
|
|
dAtA[i] = 0x1a
|
|
}
|
|
if m.EOF {
|
|
i--
|
|
if m.EOF {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x10
|
|
}
|
|
if m.Fd != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Fd))
|
|
i--
|
|
dAtA[i] = 0x8
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *ResizeMessage) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *ResizeMessage) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *ResizeMessage) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.Cols != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Cols))
|
|
i--
|
|
dAtA[i] = 0x10
|
|
}
|
|
if m.Rows != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Rows))
|
|
i--
|
|
dAtA[i] = 0x8
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *SignalMessage) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *SignalMessage) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *SignalMessage) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.Name) > 0 {
|
|
i -= len(m.Name)
|
|
copy(dAtA[i:], m.Name)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Name)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *StatusRequest) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *StatusRequest) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *StatusRequest) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.Ref) > 0 {
|
|
i -= len(m.Ref)
|
|
copy(dAtA[i:], m.Ref)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Ref)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *StatusResponse) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *StatusResponse) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *StatusResponse) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.Warnings) > 0 {
|
|
for iNdEx := len(m.Warnings) - 1; iNdEx >= 0; iNdEx-- {
|
|
if vtmsg, ok := interface{}(m.Warnings[iNdEx]).(interface {
|
|
MarshalToSizedBufferVT([]byte) (int, error)
|
|
}); ok {
|
|
size, err := vtmsg.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
} else {
|
|
encoded, err := proto.Marshal(m.Warnings[iNdEx])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= len(encoded)
|
|
copy(dAtA[i:], encoded)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(encoded)))
|
|
}
|
|
i--
|
|
dAtA[i] = 0x22
|
|
}
|
|
}
|
|
if len(m.Logs) > 0 {
|
|
for iNdEx := len(m.Logs) - 1; iNdEx >= 0; iNdEx-- {
|
|
if vtmsg, ok := interface{}(m.Logs[iNdEx]).(interface {
|
|
MarshalToSizedBufferVT([]byte) (int, error)
|
|
}); ok {
|
|
size, err := vtmsg.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
} else {
|
|
encoded, err := proto.Marshal(m.Logs[iNdEx])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= len(encoded)
|
|
copy(dAtA[i:], encoded)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(encoded)))
|
|
}
|
|
i--
|
|
dAtA[i] = 0x1a
|
|
}
|
|
}
|
|
if len(m.Statuses) > 0 {
|
|
for iNdEx := len(m.Statuses) - 1; iNdEx >= 0; iNdEx-- {
|
|
if vtmsg, ok := interface{}(m.Statuses[iNdEx]).(interface {
|
|
MarshalToSizedBufferVT([]byte) (int, error)
|
|
}); ok {
|
|
size, err := vtmsg.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
} else {
|
|
encoded, err := proto.Marshal(m.Statuses[iNdEx])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= len(encoded)
|
|
copy(dAtA[i:], encoded)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(encoded)))
|
|
}
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
}
|
|
if len(m.Vertexes) > 0 {
|
|
for iNdEx := len(m.Vertexes) - 1; iNdEx >= 0; iNdEx-- {
|
|
if vtmsg, ok := interface{}(m.Vertexes[iNdEx]).(interface {
|
|
MarshalToSizedBufferVT([]byte) (int, error)
|
|
}); ok {
|
|
size, err := vtmsg.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
} else {
|
|
encoded, err := proto.Marshal(m.Vertexes[iNdEx])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= len(encoded)
|
|
copy(dAtA[i:], encoded)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(encoded)))
|
|
}
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *InfoRequest) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *InfoRequest) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *InfoRequest) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *InfoResponse) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *InfoResponse) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *InfoResponse) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.BuildxVersion != nil {
|
|
size, err := m.BuildxVersion.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *BuildxVersion) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *BuildxVersion) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *BuildxVersion) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.Revision) > 0 {
|
|
i -= len(m.Revision)
|
|
copy(dAtA[i:], m.Revision)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Revision)))
|
|
i--
|
|
dAtA[i] = 0x1a
|
|
}
|
|
if len(m.Version) > 0 {
|
|
i -= len(m.Version)
|
|
copy(dAtA[i:], m.Version)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Version)))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
if len(m.Package) > 0 {
|
|
i -= len(m.Package)
|
|
copy(dAtA[i:], m.Package)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Package)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *ListProcessesRequest) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.Ref)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *ListProcessesResponse) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if len(m.Infos) > 0 {
|
|
for _, e := range m.Infos {
|
|
l = e.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *ProcessInfo) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.ProcessID)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.InvokeConfig != nil {
|
|
l = m.InvokeConfig.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *DisconnectProcessRequest) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.Ref)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
l = len(m.ProcessID)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *DisconnectProcessResponse) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *BuildRequest) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.Ref)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.Options != nil {
|
|
l = m.Options.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *BuildOptions) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.ContextPath)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
l = len(m.DockerfileName)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.CallFunc != nil {
|
|
l = m.CallFunc.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if len(m.NamedContexts) > 0 {
|
|
for k, v := range m.NamedContexts {
|
|
_ = k
|
|
_ = v
|
|
mapEntrySize := 1 + len(k) + protohelpers.SizeOfVarint(uint64(len(k))) + 1 + len(v) + protohelpers.SizeOfVarint(uint64(len(v)))
|
|
n += mapEntrySize + 1 + protohelpers.SizeOfVarint(uint64(mapEntrySize))
|
|
}
|
|
}
|
|
if len(m.Allow) > 0 {
|
|
for _, s := range m.Allow {
|
|
l = len(s)
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
if len(m.Attests) > 0 {
|
|
for _, e := range m.Attests {
|
|
l = e.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
if len(m.BuildArgs) > 0 {
|
|
for k, v := range m.BuildArgs {
|
|
_ = k
|
|
_ = v
|
|
mapEntrySize := 1 + len(k) + protohelpers.SizeOfVarint(uint64(len(k))) + 1 + len(v) + protohelpers.SizeOfVarint(uint64(len(v)))
|
|
n += mapEntrySize + 1 + protohelpers.SizeOfVarint(uint64(mapEntrySize))
|
|
}
|
|
}
|
|
if len(m.CacheFrom) > 0 {
|
|
for _, e := range m.CacheFrom {
|
|
l = e.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
if len(m.CacheTo) > 0 {
|
|
for _, e := range m.CacheTo {
|
|
l = e.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
l = len(m.CgroupParent)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if len(m.Exports) > 0 {
|
|
for _, e := range m.Exports {
|
|
l = e.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
if len(m.ExtraHosts) > 0 {
|
|
for _, s := range m.ExtraHosts {
|
|
l = len(s)
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
if len(m.Labels) > 0 {
|
|
for k, v := range m.Labels {
|
|
_ = k
|
|
_ = v
|
|
mapEntrySize := 1 + len(k) + protohelpers.SizeOfVarint(uint64(len(k))) + 1 + len(v) + protohelpers.SizeOfVarint(uint64(len(v)))
|
|
n += mapEntrySize + 1 + protohelpers.SizeOfVarint(uint64(mapEntrySize))
|
|
}
|
|
}
|
|
l = len(m.NetworkMode)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if len(m.NoCacheFilter) > 0 {
|
|
for _, s := range m.NoCacheFilter {
|
|
l = len(s)
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
if len(m.Platforms) > 0 {
|
|
for _, s := range m.Platforms {
|
|
l = len(s)
|
|
n += 2 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
if len(m.Secrets) > 0 {
|
|
for _, e := range m.Secrets {
|
|
l = e.SizeVT()
|
|
n += 2 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
if m.ShmSize != 0 {
|
|
n += 2 + protohelpers.SizeOfVarint(uint64(m.ShmSize))
|
|
}
|
|
if len(m.SSH) > 0 {
|
|
for _, e := range m.SSH {
|
|
l = e.SizeVT()
|
|
n += 2 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
if len(m.Tags) > 0 {
|
|
for _, s := range m.Tags {
|
|
l = len(s)
|
|
n += 2 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
l = len(m.Target)
|
|
if l > 0 {
|
|
n += 2 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.Ulimits != nil {
|
|
l = m.Ulimits.SizeVT()
|
|
n += 2 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
l = len(m.Builder)
|
|
if l > 0 {
|
|
n += 2 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.NoCache {
|
|
n += 3
|
|
}
|
|
if m.Pull {
|
|
n += 3
|
|
}
|
|
if m.ExportPush {
|
|
n += 3
|
|
}
|
|
if m.ExportLoad {
|
|
n += 3
|
|
}
|
|
if m.SourcePolicy != nil {
|
|
if size, ok := interface{}(m.SourcePolicy).(interface {
|
|
SizeVT() int
|
|
}); ok {
|
|
l = size.SizeVT()
|
|
} else {
|
|
l = proto.Size(m.SourcePolicy)
|
|
}
|
|
n += 2 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
l = len(m.Ref)
|
|
if l > 0 {
|
|
n += 2 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
l = len(m.GroupRef)
|
|
if l > 0 {
|
|
n += 2 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if len(m.Annotations) > 0 {
|
|
for _, s := range m.Annotations {
|
|
l = len(s)
|
|
n += 2 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
l = len(m.ProvenanceResponseMode)
|
|
if l > 0 {
|
|
n += 2 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *ExportEntry) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.Type)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if len(m.Attrs) > 0 {
|
|
for k, v := range m.Attrs {
|
|
_ = k
|
|
_ = v
|
|
mapEntrySize := 1 + len(k) + protohelpers.SizeOfVarint(uint64(len(k))) + 1 + len(v) + protohelpers.SizeOfVarint(uint64(len(v)))
|
|
n += mapEntrySize + 1 + protohelpers.SizeOfVarint(uint64(mapEntrySize))
|
|
}
|
|
}
|
|
l = len(m.Destination)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *CacheOptionsEntry) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.Type)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if len(m.Attrs) > 0 {
|
|
for k, v := range m.Attrs {
|
|
_ = k
|
|
_ = v
|
|
mapEntrySize := 1 + len(k) + protohelpers.SizeOfVarint(uint64(len(k))) + 1 + len(v) + protohelpers.SizeOfVarint(uint64(len(v)))
|
|
n += mapEntrySize + 1 + protohelpers.SizeOfVarint(uint64(mapEntrySize))
|
|
}
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *Attest) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.Type)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.Disabled {
|
|
n += 2
|
|
}
|
|
l = len(m.Attrs)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *SSH) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.ID)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if len(m.Paths) > 0 {
|
|
for _, s := range m.Paths {
|
|
l = len(s)
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *Secret) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.ID)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
l = len(m.FilePath)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
l = len(m.Env)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *CallFunc) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.Name)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
l = len(m.Format)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.IgnoreStatus {
|
|
n += 2
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *InspectRequest) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.Ref)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *InspectResponse) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.Options != nil {
|
|
l = m.Options.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *UlimitOpt) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if len(m.Values) > 0 {
|
|
for k, v := range m.Values {
|
|
_ = k
|
|
_ = v
|
|
l = 0
|
|
if v != nil {
|
|
l = v.SizeVT()
|
|
}
|
|
l += 1 + protohelpers.SizeOfVarint(uint64(l))
|
|
mapEntrySize := 1 + len(k) + protohelpers.SizeOfVarint(uint64(len(k))) + l
|
|
n += mapEntrySize + 1 + protohelpers.SizeOfVarint(uint64(mapEntrySize))
|
|
}
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *Ulimit) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.Name)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.Hard != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Hard))
|
|
}
|
|
if m.Soft != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Soft))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *BuildResponse) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if len(m.ExporterResponse) > 0 {
|
|
for k, v := range m.ExporterResponse {
|
|
_ = k
|
|
_ = v
|
|
mapEntrySize := 1 + len(k) + protohelpers.SizeOfVarint(uint64(len(k))) + 1 + len(v) + protohelpers.SizeOfVarint(uint64(len(v)))
|
|
n += mapEntrySize + 1 + protohelpers.SizeOfVarint(uint64(mapEntrySize))
|
|
}
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *DisconnectRequest) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.Ref)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *DisconnectResponse) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *ListRequest) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.Ref)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *ListResponse) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if len(m.Keys) > 0 {
|
|
for _, s := range m.Keys {
|
|
l = len(s)
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *InputMessage) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if vtmsg, ok := m.Input.(interface{ SizeVT() int }); ok {
|
|
n += vtmsg.SizeVT()
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *InputMessage_Init) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.Init != nil {
|
|
l = m.Init.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
} else {
|
|
n += 3
|
|
}
|
|
return n
|
|
}
|
|
func (m *InputMessage_Data) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.Data != nil {
|
|
l = m.Data.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
} else {
|
|
n += 3
|
|
}
|
|
return n
|
|
}
|
|
func (m *InputInitMessage) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.Ref)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *DataMessage) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.EOF {
|
|
n += 2
|
|
}
|
|
l = len(m.Data)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *InputResponse) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *Message) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if vtmsg, ok := m.Input.(interface{ SizeVT() int }); ok {
|
|
n += vtmsg.SizeVT()
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *Message_Init) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.Init != nil {
|
|
l = m.Init.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
} else {
|
|
n += 3
|
|
}
|
|
return n
|
|
}
|
|
func (m *Message_File) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.File != nil {
|
|
l = m.File.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
} else {
|
|
n += 3
|
|
}
|
|
return n
|
|
}
|
|
func (m *Message_Resize) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.Resize != nil {
|
|
l = m.Resize.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
} else {
|
|
n += 3
|
|
}
|
|
return n
|
|
}
|
|
func (m *Message_Signal) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.Signal != nil {
|
|
l = m.Signal.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
} else {
|
|
n += 3
|
|
}
|
|
return n
|
|
}
|
|
func (m *InitMessage) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.Ref)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
l = len(m.ProcessID)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.InvokeConfig != nil {
|
|
l = m.InvokeConfig.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *InvokeConfig) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if len(m.Entrypoint) > 0 {
|
|
for _, s := range m.Entrypoint {
|
|
l = len(s)
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
if len(m.Cmd) > 0 {
|
|
for _, s := range m.Cmd {
|
|
l = len(s)
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
if len(m.Env) > 0 {
|
|
for _, s := range m.Env {
|
|
l = len(s)
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
l = len(m.User)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.NoUser {
|
|
n += 2
|
|
}
|
|
l = len(m.Cwd)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.NoCwd {
|
|
n += 2
|
|
}
|
|
if m.Tty {
|
|
n += 2
|
|
}
|
|
if m.Rollback {
|
|
n += 2
|
|
}
|
|
if m.Initial {
|
|
n += 2
|
|
}
|
|
if m.NoCmd {
|
|
n += 2
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *FdMessage) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.Fd != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Fd))
|
|
}
|
|
if m.EOF {
|
|
n += 2
|
|
}
|
|
l = len(m.Data)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *ResizeMessage) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.Rows != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Rows))
|
|
}
|
|
if m.Cols != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Cols))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *SignalMessage) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.Name)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *StatusRequest) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.Ref)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *StatusResponse) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if len(m.Vertexes) > 0 {
|
|
for _, e := range m.Vertexes {
|
|
if size, ok := interface{}(e).(interface {
|
|
SizeVT() int
|
|
}); ok {
|
|
l = size.SizeVT()
|
|
} else {
|
|
l = proto.Size(e)
|
|
}
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
if len(m.Statuses) > 0 {
|
|
for _, e := range m.Statuses {
|
|
if size, ok := interface{}(e).(interface {
|
|
SizeVT() int
|
|
}); ok {
|
|
l = size.SizeVT()
|
|
} else {
|
|
l = proto.Size(e)
|
|
}
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
if len(m.Logs) > 0 {
|
|
for _, e := range m.Logs {
|
|
if size, ok := interface{}(e).(interface {
|
|
SizeVT() int
|
|
}); ok {
|
|
l = size.SizeVT()
|
|
} else {
|
|
l = proto.Size(e)
|
|
}
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
if len(m.Warnings) > 0 {
|
|
for _, e := range m.Warnings {
|
|
if size, ok := interface{}(e).(interface {
|
|
SizeVT() int
|
|
}); ok {
|
|
l = size.SizeVT()
|
|
} else {
|
|
l = proto.Size(e)
|
|
}
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *InfoRequest) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *InfoResponse) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.BuildxVersion != nil {
|
|
l = m.BuildxVersion.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *BuildxVersion) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.Package)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
l = len(m.Version)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
l = len(m.Revision)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *ListProcessesRequest) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: ListProcessesRequest: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: ListProcessesRequest: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Ref", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Ref = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *ListProcessesResponse) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: ListProcessesResponse: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: ListProcessesResponse: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Infos", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Infos = append(m.Infos, &ProcessInfo{})
|
|
if err := m.Infos[len(m.Infos)-1].UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *ProcessInfo) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: ProcessInfo: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: ProcessInfo: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ProcessID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ProcessID = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field InvokeConfig", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.InvokeConfig == nil {
|
|
m.InvokeConfig = &InvokeConfig{}
|
|
}
|
|
if err := m.InvokeConfig.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *DisconnectProcessRequest) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: DisconnectProcessRequest: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: DisconnectProcessRequest: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Ref", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Ref = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ProcessID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ProcessID = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *DisconnectProcessResponse) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: DisconnectProcessResponse: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: DisconnectProcessResponse: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *BuildRequest) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: BuildRequest: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: BuildRequest: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Ref", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Ref = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Options", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Options == nil {
|
|
m.Options = &BuildOptions{}
|
|
}
|
|
if err := m.Options.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *BuildOptions) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: BuildOptions: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: BuildOptions: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ContextPath", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ContextPath = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field DockerfileName", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.DockerfileName = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field CallFunc", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.CallFunc == nil {
|
|
m.CallFunc = &CallFunc{}
|
|
}
|
|
if err := m.CallFunc.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field NamedContexts", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.NamedContexts == nil {
|
|
m.NamedContexts = make(map[string]string)
|
|
}
|
|
var mapkey string
|
|
var mapvalue string
|
|
for iNdEx < postIndex {
|
|
entryPreIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
if fieldNum == 1 {
|
|
var stringLenmapkey uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLenmapkey |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLenmapkey := int(stringLenmapkey)
|
|
if intStringLenmapkey < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postStringIndexmapkey := iNdEx + intStringLenmapkey
|
|
if postStringIndexmapkey < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postStringIndexmapkey > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapkey = string(dAtA[iNdEx:postStringIndexmapkey])
|
|
iNdEx = postStringIndexmapkey
|
|
} else if fieldNum == 2 {
|
|
var stringLenmapvalue uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLenmapvalue |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLenmapvalue := int(stringLenmapvalue)
|
|
if intStringLenmapvalue < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postStringIndexmapvalue := iNdEx + intStringLenmapvalue
|
|
if postStringIndexmapvalue < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postStringIndexmapvalue > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapvalue = string(dAtA[iNdEx:postStringIndexmapvalue])
|
|
iNdEx = postStringIndexmapvalue
|
|
} else {
|
|
iNdEx = entryPreIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > postIndex {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
m.NamedContexts[mapkey] = mapvalue
|
|
iNdEx = postIndex
|
|
case 5:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Allow", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Allow = append(m.Allow, string(dAtA[iNdEx:postIndex]))
|
|
iNdEx = postIndex
|
|
case 6:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Attests", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Attests = append(m.Attests, &Attest{})
|
|
if err := m.Attests[len(m.Attests)-1].UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 7:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field BuildArgs", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.BuildArgs == nil {
|
|
m.BuildArgs = make(map[string]string)
|
|
}
|
|
var mapkey string
|
|
var mapvalue string
|
|
for iNdEx < postIndex {
|
|
entryPreIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
if fieldNum == 1 {
|
|
var stringLenmapkey uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLenmapkey |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLenmapkey := int(stringLenmapkey)
|
|
if intStringLenmapkey < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postStringIndexmapkey := iNdEx + intStringLenmapkey
|
|
if postStringIndexmapkey < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postStringIndexmapkey > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapkey = string(dAtA[iNdEx:postStringIndexmapkey])
|
|
iNdEx = postStringIndexmapkey
|
|
} else if fieldNum == 2 {
|
|
var stringLenmapvalue uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLenmapvalue |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLenmapvalue := int(stringLenmapvalue)
|
|
if intStringLenmapvalue < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postStringIndexmapvalue := iNdEx + intStringLenmapvalue
|
|
if postStringIndexmapvalue < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postStringIndexmapvalue > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapvalue = string(dAtA[iNdEx:postStringIndexmapvalue])
|
|
iNdEx = postStringIndexmapvalue
|
|
} else {
|
|
iNdEx = entryPreIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > postIndex {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
m.BuildArgs[mapkey] = mapvalue
|
|
iNdEx = postIndex
|
|
case 8:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field CacheFrom", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.CacheFrom = append(m.CacheFrom, &CacheOptionsEntry{})
|
|
if err := m.CacheFrom[len(m.CacheFrom)-1].UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 9:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field CacheTo", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.CacheTo = append(m.CacheTo, &CacheOptionsEntry{})
|
|
if err := m.CacheTo[len(m.CacheTo)-1].UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 10:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field CgroupParent", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.CgroupParent = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 11:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Exports", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Exports = append(m.Exports, &ExportEntry{})
|
|
if err := m.Exports[len(m.Exports)-1].UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 12:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ExtraHosts", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ExtraHosts = append(m.ExtraHosts, string(dAtA[iNdEx:postIndex]))
|
|
iNdEx = postIndex
|
|
case 13:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Labels", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Labels == nil {
|
|
m.Labels = make(map[string]string)
|
|
}
|
|
var mapkey string
|
|
var mapvalue string
|
|
for iNdEx < postIndex {
|
|
entryPreIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
if fieldNum == 1 {
|
|
var stringLenmapkey uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLenmapkey |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLenmapkey := int(stringLenmapkey)
|
|
if intStringLenmapkey < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postStringIndexmapkey := iNdEx + intStringLenmapkey
|
|
if postStringIndexmapkey < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postStringIndexmapkey > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapkey = string(dAtA[iNdEx:postStringIndexmapkey])
|
|
iNdEx = postStringIndexmapkey
|
|
} else if fieldNum == 2 {
|
|
var stringLenmapvalue uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLenmapvalue |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLenmapvalue := int(stringLenmapvalue)
|
|
if intStringLenmapvalue < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postStringIndexmapvalue := iNdEx + intStringLenmapvalue
|
|
if postStringIndexmapvalue < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postStringIndexmapvalue > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapvalue = string(dAtA[iNdEx:postStringIndexmapvalue])
|
|
iNdEx = postStringIndexmapvalue
|
|
} else {
|
|
iNdEx = entryPreIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > postIndex {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
m.Labels[mapkey] = mapvalue
|
|
iNdEx = postIndex
|
|
case 14:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field NetworkMode", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.NetworkMode = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 15:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field NoCacheFilter", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.NoCacheFilter = append(m.NoCacheFilter, string(dAtA[iNdEx:postIndex]))
|
|
iNdEx = postIndex
|
|
case 16:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Platforms", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Platforms = append(m.Platforms, string(dAtA[iNdEx:postIndex]))
|
|
iNdEx = postIndex
|
|
case 17:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Secrets", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Secrets = append(m.Secrets, &Secret{})
|
|
if err := m.Secrets[len(m.Secrets)-1].UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 18:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ShmSize", wireType)
|
|
}
|
|
m.ShmSize = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.ShmSize |= int64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 19:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field SSH", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.SSH = append(m.SSH, &SSH{})
|
|
if err := m.SSH[len(m.SSH)-1].UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 20:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Tags", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Tags = append(m.Tags, string(dAtA[iNdEx:postIndex]))
|
|
iNdEx = postIndex
|
|
case 21:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Target", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Target = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 22:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Ulimits", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Ulimits == nil {
|
|
m.Ulimits = &UlimitOpt{}
|
|
}
|
|
if err := m.Ulimits.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 23:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Builder", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Builder = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 24:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field NoCache", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.NoCache = bool(v != 0)
|
|
case 25:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Pull", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.Pull = bool(v != 0)
|
|
case 26:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ExportPush", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.ExportPush = bool(v != 0)
|
|
case 27:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ExportLoad", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.ExportLoad = bool(v != 0)
|
|
case 28:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field SourcePolicy", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.SourcePolicy == nil {
|
|
m.SourcePolicy = &pb.Policy{}
|
|
}
|
|
if unmarshal, ok := interface{}(m.SourcePolicy).(interface {
|
|
UnmarshalVT([]byte) error
|
|
}); ok {
|
|
if err := unmarshal.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
} else {
|
|
if err := proto.Unmarshal(dAtA[iNdEx:postIndex], m.SourcePolicy); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
iNdEx = postIndex
|
|
case 29:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Ref", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Ref = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 30:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field GroupRef", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.GroupRef = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 31:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Annotations", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Annotations = append(m.Annotations, string(dAtA[iNdEx:postIndex]))
|
|
iNdEx = postIndex
|
|
case 32:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ProvenanceResponseMode", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ProvenanceResponseMode = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *ExportEntry) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: ExportEntry: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: ExportEntry: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Type", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Type = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Attrs", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Attrs == nil {
|
|
m.Attrs = make(map[string]string)
|
|
}
|
|
var mapkey string
|
|
var mapvalue string
|
|
for iNdEx < postIndex {
|
|
entryPreIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
if fieldNum == 1 {
|
|
var stringLenmapkey uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLenmapkey |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLenmapkey := int(stringLenmapkey)
|
|
if intStringLenmapkey < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postStringIndexmapkey := iNdEx + intStringLenmapkey
|
|
if postStringIndexmapkey < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postStringIndexmapkey > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapkey = string(dAtA[iNdEx:postStringIndexmapkey])
|
|
iNdEx = postStringIndexmapkey
|
|
} else if fieldNum == 2 {
|
|
var stringLenmapvalue uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLenmapvalue |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLenmapvalue := int(stringLenmapvalue)
|
|
if intStringLenmapvalue < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postStringIndexmapvalue := iNdEx + intStringLenmapvalue
|
|
if postStringIndexmapvalue < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postStringIndexmapvalue > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapvalue = string(dAtA[iNdEx:postStringIndexmapvalue])
|
|
iNdEx = postStringIndexmapvalue
|
|
} else {
|
|
iNdEx = entryPreIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > postIndex {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
m.Attrs[mapkey] = mapvalue
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Destination", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Destination = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *CacheOptionsEntry) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: CacheOptionsEntry: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: CacheOptionsEntry: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Type", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Type = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Attrs", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Attrs == nil {
|
|
m.Attrs = make(map[string]string)
|
|
}
|
|
var mapkey string
|
|
var mapvalue string
|
|
for iNdEx < postIndex {
|
|
entryPreIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
if fieldNum == 1 {
|
|
var stringLenmapkey uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLenmapkey |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLenmapkey := int(stringLenmapkey)
|
|
if intStringLenmapkey < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postStringIndexmapkey := iNdEx + intStringLenmapkey
|
|
if postStringIndexmapkey < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postStringIndexmapkey > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapkey = string(dAtA[iNdEx:postStringIndexmapkey])
|
|
iNdEx = postStringIndexmapkey
|
|
} else if fieldNum == 2 {
|
|
var stringLenmapvalue uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLenmapvalue |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLenmapvalue := int(stringLenmapvalue)
|
|
if intStringLenmapvalue < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postStringIndexmapvalue := iNdEx + intStringLenmapvalue
|
|
if postStringIndexmapvalue < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postStringIndexmapvalue > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapvalue = string(dAtA[iNdEx:postStringIndexmapvalue])
|
|
iNdEx = postStringIndexmapvalue
|
|
} else {
|
|
iNdEx = entryPreIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > postIndex {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
m.Attrs[mapkey] = mapvalue
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Attest) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Attest: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Attest: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Type", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Type = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Disabled", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.Disabled = bool(v != 0)
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Attrs", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Attrs = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *SSH) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: SSH: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: SSH: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ID = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Paths", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Paths = append(m.Paths, string(dAtA[iNdEx:postIndex]))
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Secret) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Secret: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Secret: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ID = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field FilePath", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.FilePath = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Env", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Env = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *CallFunc) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: CallFunc: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: CallFunc: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Name", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Name = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Format", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Format = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field IgnoreStatus", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.IgnoreStatus = bool(v != 0)
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *InspectRequest) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: InspectRequest: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: InspectRequest: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Ref", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Ref = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *InspectResponse) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: InspectResponse: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: InspectResponse: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Options", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Options == nil {
|
|
m.Options = &BuildOptions{}
|
|
}
|
|
if err := m.Options.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *UlimitOpt) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: UlimitOpt: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: UlimitOpt: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Values", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Values == nil {
|
|
m.Values = make(map[string]*Ulimit)
|
|
}
|
|
var mapkey string
|
|
var mapvalue *Ulimit
|
|
for iNdEx < postIndex {
|
|
entryPreIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
if fieldNum == 1 {
|
|
var stringLenmapkey uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLenmapkey |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLenmapkey := int(stringLenmapkey)
|
|
if intStringLenmapkey < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postStringIndexmapkey := iNdEx + intStringLenmapkey
|
|
if postStringIndexmapkey < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postStringIndexmapkey > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapkey = string(dAtA[iNdEx:postStringIndexmapkey])
|
|
iNdEx = postStringIndexmapkey
|
|
} else if fieldNum == 2 {
|
|
var mapmsglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
mapmsglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if mapmsglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postmsgIndex := iNdEx + mapmsglen
|
|
if postmsgIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postmsgIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapvalue = &Ulimit{}
|
|
if err := mapvalue.UnmarshalVT(dAtA[iNdEx:postmsgIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postmsgIndex
|
|
} else {
|
|
iNdEx = entryPreIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > postIndex {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
m.Values[mapkey] = mapvalue
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Ulimit) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Ulimit: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Ulimit: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Name", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Name = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Hard", wireType)
|
|
}
|
|
m.Hard = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Hard |= int64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 3:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Soft", wireType)
|
|
}
|
|
m.Soft = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Soft |= int64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *BuildResponse) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: BuildResponse: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: BuildResponse: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ExporterResponse", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.ExporterResponse == nil {
|
|
m.ExporterResponse = make(map[string]string)
|
|
}
|
|
var mapkey string
|
|
var mapvalue string
|
|
for iNdEx < postIndex {
|
|
entryPreIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
if fieldNum == 1 {
|
|
var stringLenmapkey uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLenmapkey |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLenmapkey := int(stringLenmapkey)
|
|
if intStringLenmapkey < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postStringIndexmapkey := iNdEx + intStringLenmapkey
|
|
if postStringIndexmapkey < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postStringIndexmapkey > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapkey = string(dAtA[iNdEx:postStringIndexmapkey])
|
|
iNdEx = postStringIndexmapkey
|
|
} else if fieldNum == 2 {
|
|
var stringLenmapvalue uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLenmapvalue |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLenmapvalue := int(stringLenmapvalue)
|
|
if intStringLenmapvalue < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postStringIndexmapvalue := iNdEx + intStringLenmapvalue
|
|
if postStringIndexmapvalue < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postStringIndexmapvalue > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapvalue = string(dAtA[iNdEx:postStringIndexmapvalue])
|
|
iNdEx = postStringIndexmapvalue
|
|
} else {
|
|
iNdEx = entryPreIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > postIndex {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
m.ExporterResponse[mapkey] = mapvalue
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *DisconnectRequest) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: DisconnectRequest: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: DisconnectRequest: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Ref", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Ref = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *DisconnectResponse) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: DisconnectResponse: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: DisconnectResponse: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *ListRequest) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: ListRequest: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: ListRequest: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Ref", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Ref = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *ListResponse) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: ListResponse: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: ListResponse: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Keys", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Keys = append(m.Keys, string(dAtA[iNdEx:postIndex]))
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *InputMessage) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: InputMessage: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: InputMessage: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Init", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if oneof, ok := m.Input.(*InputMessage_Init); ok {
|
|
if err := oneof.Init.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
} else {
|
|
v := &InputInitMessage{}
|
|
if err := v.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
m.Input = &InputMessage_Init{Init: v}
|
|
}
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Data", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if oneof, ok := m.Input.(*InputMessage_Data); ok {
|
|
if err := oneof.Data.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
} else {
|
|
v := &DataMessage{}
|
|
if err := v.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
m.Input = &InputMessage_Data{Data: v}
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *InputInitMessage) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: InputInitMessage: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: InputInitMessage: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Ref", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Ref = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *DataMessage) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: DataMessage: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: DataMessage: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field EOF", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.EOF = bool(v != 0)
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Data", wireType)
|
|
}
|
|
var byteLen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
byteLen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if byteLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + byteLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Data = append(m.Data[:0], dAtA[iNdEx:postIndex]...)
|
|
if m.Data == nil {
|
|
m.Data = []byte{}
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *InputResponse) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: InputResponse: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: InputResponse: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Message) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Message: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Message: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Init", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if oneof, ok := m.Input.(*Message_Init); ok {
|
|
if err := oneof.Init.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
} else {
|
|
v := &InitMessage{}
|
|
if err := v.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
m.Input = &Message_Init{Init: v}
|
|
}
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field File", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if oneof, ok := m.Input.(*Message_File); ok {
|
|
if err := oneof.File.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
} else {
|
|
v := &FdMessage{}
|
|
if err := v.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
m.Input = &Message_File{File: v}
|
|
}
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Resize", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if oneof, ok := m.Input.(*Message_Resize); ok {
|
|
if err := oneof.Resize.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
} else {
|
|
v := &ResizeMessage{}
|
|
if err := v.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
m.Input = &Message_Resize{Resize: v}
|
|
}
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Signal", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if oneof, ok := m.Input.(*Message_Signal); ok {
|
|
if err := oneof.Signal.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
} else {
|
|
v := &SignalMessage{}
|
|
if err := v.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
m.Input = &Message_Signal{Signal: v}
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *InitMessage) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: InitMessage: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: InitMessage: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Ref", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Ref = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ProcessID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ProcessID = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field InvokeConfig", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.InvokeConfig == nil {
|
|
m.InvokeConfig = &InvokeConfig{}
|
|
}
|
|
if err := m.InvokeConfig.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *InvokeConfig) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: InvokeConfig: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: InvokeConfig: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Entrypoint", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Entrypoint = append(m.Entrypoint, string(dAtA[iNdEx:postIndex]))
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Cmd", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Cmd = append(m.Cmd, string(dAtA[iNdEx:postIndex]))
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Env", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Env = append(m.Env, string(dAtA[iNdEx:postIndex]))
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field User", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.User = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 5:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field NoUser", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.NoUser = bool(v != 0)
|
|
case 6:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Cwd", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Cwd = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 7:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field NoCwd", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.NoCwd = bool(v != 0)
|
|
case 8:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Tty", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.Tty = bool(v != 0)
|
|
case 9:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Rollback", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.Rollback = bool(v != 0)
|
|
case 10:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Initial", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.Initial = bool(v != 0)
|
|
case 11:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field NoCmd", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.NoCmd = bool(v != 0)
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *FdMessage) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: FdMessage: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: FdMessage: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Fd", wireType)
|
|
}
|
|
m.Fd = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Fd |= uint32(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 2:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field EOF", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.EOF = bool(v != 0)
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Data", wireType)
|
|
}
|
|
var byteLen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
byteLen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if byteLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + byteLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Data = append(m.Data[:0], dAtA[iNdEx:postIndex]...)
|
|
if m.Data == nil {
|
|
m.Data = []byte{}
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *ResizeMessage) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: ResizeMessage: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: ResizeMessage: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Rows", wireType)
|
|
}
|
|
m.Rows = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Rows |= uint32(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 2:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Cols", wireType)
|
|
}
|
|
m.Cols = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Cols |= uint32(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *SignalMessage) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: SignalMessage: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: SignalMessage: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Name", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Name = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *StatusRequest) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: StatusRequest: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: StatusRequest: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Ref", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Ref = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *StatusResponse) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: StatusResponse: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: StatusResponse: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Vertexes", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Vertexes = append(m.Vertexes, &control.Vertex{})
|
|
if unmarshal, ok := interface{}(m.Vertexes[len(m.Vertexes)-1]).(interface {
|
|
UnmarshalVT([]byte) error
|
|
}); ok {
|
|
if err := unmarshal.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
} else {
|
|
if err := proto.Unmarshal(dAtA[iNdEx:postIndex], m.Vertexes[len(m.Vertexes)-1]); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Statuses", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Statuses = append(m.Statuses, &control.VertexStatus{})
|
|
if unmarshal, ok := interface{}(m.Statuses[len(m.Statuses)-1]).(interface {
|
|
UnmarshalVT([]byte) error
|
|
}); ok {
|
|
if err := unmarshal.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
} else {
|
|
if err := proto.Unmarshal(dAtA[iNdEx:postIndex], m.Statuses[len(m.Statuses)-1]); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Logs", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Logs = append(m.Logs, &control.VertexLog{})
|
|
if unmarshal, ok := interface{}(m.Logs[len(m.Logs)-1]).(interface {
|
|
UnmarshalVT([]byte) error
|
|
}); ok {
|
|
if err := unmarshal.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
} else {
|
|
if err := proto.Unmarshal(dAtA[iNdEx:postIndex], m.Logs[len(m.Logs)-1]); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Warnings", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Warnings = append(m.Warnings, &control.VertexWarning{})
|
|
if unmarshal, ok := interface{}(m.Warnings[len(m.Warnings)-1]).(interface {
|
|
UnmarshalVT([]byte) error
|
|
}); ok {
|
|
if err := unmarshal.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
} else {
|
|
if err := proto.Unmarshal(dAtA[iNdEx:postIndex], m.Warnings[len(m.Warnings)-1]); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *InfoRequest) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: InfoRequest: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: InfoRequest: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *InfoResponse) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: InfoResponse: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: InfoResponse: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field BuildxVersion", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.BuildxVersion == nil {
|
|
m.BuildxVersion = &BuildxVersion{}
|
|
}
|
|
if err := m.BuildxVersion.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *BuildxVersion) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: BuildxVersion: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: BuildxVersion: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Package", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Package = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Version", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Version = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Revision", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Revision = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|