mirror of
https://gitea.com/Lydanne/buildx.git
synced 2025-05-19 09:57:45 +08:00
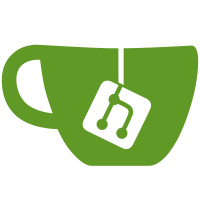
Removes gogo/protobuf from buildx and updates to a version of moby/buildkit where gogo is removed. This also changes how the proto files are generated. This is because newer versions of protobuf are more strict about name conflicts. If two files have the same name (even if they are relative paths) and are used in different protoc commands, they'll conflict in the registry. Since protobuf file generation doesn't work very well with `paths=source_relative`, this removes the `go:generate` expression and just relies on the dockerfile to perform the generation. Signed-off-by: Jonathan A. Sternberg <jonathan.sternberg@docker.com>
328 lines
7.3 KiB
Protocol Buffer
328 lines
7.3 KiB
Protocol Buffer
syntax = "proto3";
|
|
|
|
package moby.buildkit.v1.frontend;
|
|
|
|
option go_package = "github.com/moby/buildkit/frontend/gateway/pb;moby_buildkit_v1_frontend";
|
|
|
|
import "github.com/moby/buildkit/api/types/worker.proto";
|
|
import "github.com/moby/buildkit/solver/pb/ops.proto";
|
|
import "github.com/moby/buildkit/sourcepolicy/pb/policy.proto";
|
|
import "github.com/moby/buildkit/util/apicaps/pb/caps.proto";
|
|
import "github.com/tonistiigi/fsutil/types/stat.proto";
|
|
import "google/rpc/status.proto";
|
|
|
|
service LLBBridge {
|
|
// apicaps:CapResolveImage
|
|
rpc ResolveImageConfig(ResolveImageConfigRequest) returns (ResolveImageConfigResponse);
|
|
// apicaps:CapSourceMetaResolver
|
|
rpc ResolveSourceMeta(ResolveSourceMetaRequest) returns (ResolveSourceMetaResponse);
|
|
// apicaps:CapSolveBase
|
|
rpc Solve(SolveRequest) returns (SolveResponse);
|
|
// apicaps:CapReadFile
|
|
rpc ReadFile(ReadFileRequest) returns (ReadFileResponse);
|
|
// apicaps:CapReadDir
|
|
rpc ReadDir(ReadDirRequest) returns (ReadDirResponse);
|
|
// apicaps:CapStatFile
|
|
rpc StatFile(StatFileRequest) returns (StatFileResponse);
|
|
// apicaps:CapGatewayEvaluate
|
|
rpc Evaluate(EvaluateRequest) returns (EvaluateResponse);
|
|
rpc Ping(PingRequest) returns (PongResponse);
|
|
rpc Return(ReturnRequest) returns (ReturnResponse);
|
|
// apicaps:CapFrontendInputs
|
|
rpc Inputs(InputsRequest) returns (InputsResponse);
|
|
|
|
rpc NewContainer(NewContainerRequest) returns (NewContainerResponse);
|
|
rpc ReleaseContainer(ReleaseContainerRequest) returns (ReleaseContainerResponse);
|
|
rpc ExecProcess(stream ExecMessage) returns (stream ExecMessage);
|
|
|
|
// apicaps:CapGatewayWarnings
|
|
rpc Warn(WarnRequest) returns (WarnResponse);
|
|
}
|
|
|
|
message Result {
|
|
oneof result {
|
|
// Deprecated non-array refs.
|
|
string refDeprecated = 1;
|
|
RefMapDeprecated refsDeprecated = 2;
|
|
|
|
Ref ref = 3;
|
|
RefMap refs = 4;
|
|
}
|
|
map<string, bytes> metadata = 10;
|
|
// 11 was used during development and is reserved for old attestation format
|
|
map<string, Attestations> attestations = 12;
|
|
}
|
|
|
|
message RefMapDeprecated {
|
|
map<string, string> refs = 1;
|
|
}
|
|
|
|
message Ref {
|
|
string id = 1;
|
|
pb.Definition def = 2;
|
|
}
|
|
|
|
message RefMap {
|
|
map<string, Ref> refs = 1;
|
|
}
|
|
|
|
message Attestations {
|
|
repeated Attestation attestation = 1;
|
|
}
|
|
|
|
message Attestation {
|
|
AttestationKind kind = 1;
|
|
map<string, bytes> metadata = 2;
|
|
|
|
Ref ref = 3;
|
|
string path = 4;
|
|
string inTotoPredicateType = 5;
|
|
repeated InTotoSubject inTotoSubjects = 6;
|
|
}
|
|
|
|
enum AttestationKind {
|
|
InToto = 0;
|
|
Bundle = 1;
|
|
}
|
|
|
|
message InTotoSubject {
|
|
InTotoSubjectKind kind = 1;
|
|
|
|
repeated string digest = 2;
|
|
string name = 3;
|
|
}
|
|
|
|
enum InTotoSubjectKind {
|
|
Self = 0;
|
|
Raw = 1;
|
|
}
|
|
|
|
message ReturnRequest {
|
|
Result result = 1;
|
|
google.rpc.Status error = 2;
|
|
}
|
|
|
|
message ReturnResponse {
|
|
}
|
|
|
|
message InputsRequest {
|
|
}
|
|
|
|
message InputsResponse {
|
|
map<string, pb.Definition> Definitions = 1;
|
|
}
|
|
|
|
message ResolveImageConfigRequest {
|
|
string Ref = 1;
|
|
pb.Platform Platform = 2;
|
|
string ResolveMode = 3;
|
|
string LogName = 4;
|
|
int32 ResolverType = 5;
|
|
string SessionID = 6;
|
|
string StoreID = 7;
|
|
repeated moby.buildkit.v1.sourcepolicy.Policy SourcePolicies = 8;
|
|
}
|
|
|
|
message ResolveImageConfigResponse {
|
|
string Digest = 1;
|
|
bytes Config = 2;
|
|
string Ref = 3;
|
|
}
|
|
|
|
message ResolveSourceMetaRequest {
|
|
pb.SourceOp Source = 1;
|
|
pb.Platform Platform = 2;
|
|
string LogName = 3;
|
|
string ResolveMode = 4;
|
|
repeated moby.buildkit.v1.sourcepolicy.Policy SourcePolicies = 8;
|
|
}
|
|
|
|
message ResolveSourceMetaResponse {
|
|
pb.SourceOp Source = 1;
|
|
ResolveSourceImageResponse Image = 2;
|
|
}
|
|
|
|
message ResolveSourceImageResponse {
|
|
string Digest = 1;
|
|
bytes Config = 2;
|
|
}
|
|
|
|
message SolveRequest {
|
|
pb.Definition Definition = 1;
|
|
string Frontend = 2;
|
|
map<string, string> FrontendOpt = 3;
|
|
// 4 was removed in BuildKit v0.11.0.
|
|
bool allowResultReturn = 5;
|
|
bool allowResultArrayRef = 6;
|
|
|
|
// apicaps.CapSolveInlineReturn deprecated
|
|
bool Final = 10;
|
|
bytes ExporterAttr = 11;
|
|
// CacheImports was added in BuildKit v0.4.0.
|
|
// apicaps:CapImportCaches
|
|
repeated CacheOptionsEntry CacheImports = 12;
|
|
|
|
// apicaps:CapFrontendInputs
|
|
map<string, pb.Definition> FrontendInputs = 13;
|
|
|
|
bool Evaluate = 14;
|
|
|
|
repeated moby.buildkit.v1.sourcepolicy.Policy SourcePolicies = 15;
|
|
}
|
|
|
|
// CacheOptionsEntry corresponds to the control.CacheOptionsEntry
|
|
message CacheOptionsEntry {
|
|
string Type = 1;
|
|
map<string, string> Attrs = 2;
|
|
}
|
|
|
|
message SolveResponse {
|
|
// deprecated
|
|
string ref = 1; // can be used by readfile request
|
|
// deprecated
|
|
// bytes ExporterAttr = 2;
|
|
|
|
// these fields are returned when allowMapReturn was set
|
|
Result result = 3;
|
|
}
|
|
|
|
message ReadFileRequest {
|
|
string Ref = 1;
|
|
string FilePath = 2;
|
|
FileRange Range = 3;
|
|
}
|
|
|
|
message FileRange {
|
|
int64 Offset = 1;
|
|
int64 Length = 2;
|
|
}
|
|
|
|
message ReadFileResponse {
|
|
bytes Data = 1;
|
|
}
|
|
|
|
message ReadDirRequest {
|
|
string Ref = 1;
|
|
string DirPath = 2;
|
|
string IncludePattern = 3;
|
|
}
|
|
|
|
message ReadDirResponse {
|
|
repeated fsutil.types.Stat entries = 1;
|
|
}
|
|
|
|
message StatFileRequest {
|
|
string Ref = 1;
|
|
string Path = 2;
|
|
}
|
|
|
|
message StatFileResponse {
|
|
fsutil.types.Stat stat = 1;
|
|
}
|
|
|
|
message EvaluateRequest {
|
|
string Ref = 1;
|
|
}
|
|
|
|
message EvaluateResponse {
|
|
}
|
|
|
|
message PingRequest{
|
|
}
|
|
message PongResponse{
|
|
repeated moby.buildkit.v1.apicaps.APICap FrontendAPICaps = 1;
|
|
repeated moby.buildkit.v1.apicaps.APICap LLBCaps = 2;
|
|
repeated moby.buildkit.v1.types.WorkerRecord Workers = 3;
|
|
}
|
|
|
|
message WarnRequest {
|
|
string digest = 1;
|
|
int64 level = 2;
|
|
bytes short = 3;
|
|
repeated bytes detail = 4;
|
|
string url = 5;
|
|
pb.SourceInfo info = 6;
|
|
repeated pb.Range ranges = 7;
|
|
}
|
|
|
|
message WarnResponse{}
|
|
|
|
message NewContainerRequest {
|
|
string ContainerID = 1;
|
|
// For mount input values we can use random identifiers passed with ref
|
|
repeated pb.Mount Mounts = 2;
|
|
pb.NetMode Network = 3;
|
|
pb.Platform platform = 4;
|
|
pb.WorkerConstraints constraints = 5;
|
|
repeated pb.HostIP extraHosts = 6;
|
|
string hostname = 7;
|
|
}
|
|
|
|
message NewContainerResponse{}
|
|
|
|
message ReleaseContainerRequest {
|
|
string ContainerID = 1;
|
|
}
|
|
|
|
message ReleaseContainerResponse{}
|
|
|
|
message ExecMessage {
|
|
string ProcessID = 1;
|
|
oneof Input {
|
|
// InitMessage sent from client to server will start a new process in a
|
|
// container
|
|
InitMessage Init = 2;
|
|
// FdMessage used from client to server for input (stdin) and
|
|
// from server to client for output (stdout, stderr)
|
|
FdMessage File = 3;
|
|
// ResizeMessage used from client to server for terminal resize events
|
|
ResizeMessage Resize = 4;
|
|
// StartedMessage sent from server to client after InitMessage to
|
|
// indicate the process has started.
|
|
StartedMessage Started = 5;
|
|
// ExitMessage sent from server to client will contain the exit code
|
|
// when the process ends.
|
|
ExitMessage Exit = 6;
|
|
// DoneMessage from server to client will be the last message for any
|
|
// process. Note that FdMessage might be sent after ExitMessage.
|
|
DoneMessage Done = 7;
|
|
// SignalMessage is used from client to server to send signal events
|
|
SignalMessage Signal = 8;
|
|
}
|
|
}
|
|
|
|
message InitMessage{
|
|
string ContainerID = 1;
|
|
pb.Meta Meta = 2;
|
|
repeated uint32 Fds = 3;
|
|
bool Tty = 4;
|
|
pb.SecurityMode Security = 5;
|
|
repeated pb.SecretEnv secretenv = 6;
|
|
}
|
|
|
|
message ExitMessage {
|
|
uint32 Code = 1;
|
|
google.rpc.Status Error = 2;
|
|
}
|
|
|
|
message StartedMessage{}
|
|
|
|
message DoneMessage{}
|
|
|
|
message FdMessage{
|
|
uint32 Fd = 1; // what fd the data was from
|
|
bool EOF = 2; // true if eof was reached
|
|
bytes Data = 3;
|
|
}
|
|
|
|
message ResizeMessage{
|
|
uint32 Rows = 1;
|
|
uint32 Cols = 2;
|
|
}
|
|
|
|
message SignalMessage {
|
|
// we only send name (ie HUP, INT) because the int values
|
|
// are platform dependent.
|
|
string Name = 1;
|
|
}
|