mirror of
https://gitea.com/Lydanne/buildx.git
synced 2025-05-18 17:37:46 +08:00
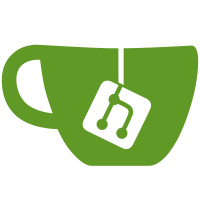
Integrates vtproto into buildx. The generated files dockerfile has been modified to copy the buildkit equivalent file to ensure files are laid out in the appropriate way for imports. An import has also been included to change the grpc codec to the version in buildkit that supports vtproto. This will allow buildx to utilize the speed and memory improvements from that. Also updates the gc control options for prune. Signed-off-by: Jonathan A. Sternberg <jonathan.sternberg@docker.com>
266 lines
8.8 KiB
Go
266 lines
8.8 KiB
Go
// Code generated by protoc-gen-go. DO NOT EDIT.
|
|
// versions:
|
|
// protoc-gen-go v1.34.1
|
|
// protoc v3.11.4
|
|
// source: github.com/moby/buildkit/util/stack/stack.proto
|
|
|
|
package stack
|
|
|
|
import (
|
|
protoreflect "google.golang.org/protobuf/reflect/protoreflect"
|
|
protoimpl "google.golang.org/protobuf/runtime/protoimpl"
|
|
reflect "reflect"
|
|
sync "sync"
|
|
)
|
|
|
|
const (
|
|
// Verify that this generated code is sufficiently up-to-date.
|
|
_ = protoimpl.EnforceVersion(20 - protoimpl.MinVersion)
|
|
// Verify that runtime/protoimpl is sufficiently up-to-date.
|
|
_ = protoimpl.EnforceVersion(protoimpl.MaxVersion - 20)
|
|
)
|
|
|
|
type Stack struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Frames []*Frame `protobuf:"bytes,1,rep,name=frames,proto3" json:"frames,omitempty"`
|
|
Cmdline []string `protobuf:"bytes,2,rep,name=cmdline,proto3" json:"cmdline,omitempty"`
|
|
Pid int32 `protobuf:"varint,3,opt,name=pid,proto3" json:"pid,omitempty"`
|
|
Version string `protobuf:"bytes,4,opt,name=version,proto3" json:"version,omitempty"`
|
|
Revision string `protobuf:"bytes,5,opt,name=revision,proto3" json:"revision,omitempty"`
|
|
}
|
|
|
|
func (x *Stack) Reset() {
|
|
*x = Stack{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_moby_buildkit_util_stack_stack_proto_msgTypes[0]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *Stack) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*Stack) ProtoMessage() {}
|
|
|
|
func (x *Stack) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_moby_buildkit_util_stack_stack_proto_msgTypes[0]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use Stack.ProtoReflect.Descriptor instead.
|
|
func (*Stack) Descriptor() ([]byte, []int) {
|
|
return file_github_com_moby_buildkit_util_stack_stack_proto_rawDescGZIP(), []int{0}
|
|
}
|
|
|
|
func (x *Stack) GetFrames() []*Frame {
|
|
if x != nil {
|
|
return x.Frames
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *Stack) GetCmdline() []string {
|
|
if x != nil {
|
|
return x.Cmdline
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *Stack) GetPid() int32 {
|
|
if x != nil {
|
|
return x.Pid
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *Stack) GetVersion() string {
|
|
if x != nil {
|
|
return x.Version
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *Stack) GetRevision() string {
|
|
if x != nil {
|
|
return x.Revision
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type Frame struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Name string `protobuf:"bytes,1,opt,name=Name,proto3" json:"Name,omitempty"`
|
|
File string `protobuf:"bytes,2,opt,name=File,proto3" json:"File,omitempty"`
|
|
Line int32 `protobuf:"varint,3,opt,name=Line,proto3" json:"Line,omitempty"`
|
|
}
|
|
|
|
func (x *Frame) Reset() {
|
|
*x = Frame{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_moby_buildkit_util_stack_stack_proto_msgTypes[1]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *Frame) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*Frame) ProtoMessage() {}
|
|
|
|
func (x *Frame) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_moby_buildkit_util_stack_stack_proto_msgTypes[1]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use Frame.ProtoReflect.Descriptor instead.
|
|
func (*Frame) Descriptor() ([]byte, []int) {
|
|
return file_github_com_moby_buildkit_util_stack_stack_proto_rawDescGZIP(), []int{1}
|
|
}
|
|
|
|
func (x *Frame) GetName() string {
|
|
if x != nil {
|
|
return x.Name
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *Frame) GetFile() string {
|
|
if x != nil {
|
|
return x.File
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *Frame) GetLine() int32 {
|
|
if x != nil {
|
|
return x.Line
|
|
}
|
|
return 0
|
|
}
|
|
|
|
var File_github_com_moby_buildkit_util_stack_stack_proto protoreflect.FileDescriptor
|
|
|
|
var file_github_com_moby_buildkit_util_stack_stack_proto_rawDesc = []byte{
|
|
0x0a, 0x2f, 0x67, 0x69, 0x74, 0x68, 0x75, 0x62, 0x2e, 0x63, 0x6f, 0x6d, 0x2f, 0x6d, 0x6f, 0x62,
|
|
0x79, 0x2f, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2f, 0x75, 0x74, 0x69, 0x6c, 0x2f,
|
|
0x73, 0x74, 0x61, 0x63, 0x6b, 0x2f, 0x73, 0x74, 0x61, 0x63, 0x6b, 0x2e, 0x70, 0x72, 0x6f, 0x74,
|
|
0x6f, 0x12, 0x05, 0x73, 0x74, 0x61, 0x63, 0x6b, 0x22, 0x8f, 0x01, 0x0a, 0x05, 0x53, 0x74, 0x61,
|
|
0x63, 0x6b, 0x12, 0x24, 0x0a, 0x06, 0x66, 0x72, 0x61, 0x6d, 0x65, 0x73, 0x18, 0x01, 0x20, 0x03,
|
|
0x28, 0x0b, 0x32, 0x0c, 0x2e, 0x73, 0x74, 0x61, 0x63, 0x6b, 0x2e, 0x46, 0x72, 0x61, 0x6d, 0x65,
|
|
0x52, 0x06, 0x66, 0x72, 0x61, 0x6d, 0x65, 0x73, 0x12, 0x18, 0x0a, 0x07, 0x63, 0x6d, 0x64, 0x6c,
|
|
0x69, 0x6e, 0x65, 0x18, 0x02, 0x20, 0x03, 0x28, 0x09, 0x52, 0x07, 0x63, 0x6d, 0x64, 0x6c, 0x69,
|
|
0x6e, 0x65, 0x12, 0x10, 0x0a, 0x03, 0x70, 0x69, 0x64, 0x18, 0x03, 0x20, 0x01, 0x28, 0x05, 0x52,
|
|
0x03, 0x70, 0x69, 0x64, 0x12, 0x18, 0x0a, 0x07, 0x76, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x18,
|
|
0x04, 0x20, 0x01, 0x28, 0x09, 0x52, 0x07, 0x76, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x12, 0x1a,
|
|
0x0a, 0x08, 0x72, 0x65, 0x76, 0x69, 0x73, 0x69, 0x6f, 0x6e, 0x18, 0x05, 0x20, 0x01, 0x28, 0x09,
|
|
0x52, 0x08, 0x72, 0x65, 0x76, 0x69, 0x73, 0x69, 0x6f, 0x6e, 0x22, 0x43, 0x0a, 0x05, 0x46, 0x72,
|
|
0x61, 0x6d, 0x65, 0x12, 0x12, 0x0a, 0x04, 0x4e, 0x61, 0x6d, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28,
|
|
0x09, 0x52, 0x04, 0x4e, 0x61, 0x6d, 0x65, 0x12, 0x12, 0x0a, 0x04, 0x46, 0x69, 0x6c, 0x65, 0x18,
|
|
0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x04, 0x46, 0x69, 0x6c, 0x65, 0x12, 0x12, 0x0a, 0x04, 0x4c,
|
|
0x69, 0x6e, 0x65, 0x18, 0x03, 0x20, 0x01, 0x28, 0x05, 0x52, 0x04, 0x4c, 0x69, 0x6e, 0x65, 0x42,
|
|
0x25, 0x5a, 0x23, 0x67, 0x69, 0x74, 0x68, 0x75, 0x62, 0x2e, 0x63, 0x6f, 0x6d, 0x2f, 0x6d, 0x6f,
|
|
0x62, 0x79, 0x2f, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2f, 0x75, 0x74, 0x69, 0x6c,
|
|
0x2f, 0x73, 0x74, 0x61, 0x63, 0x6b, 0x62, 0x06, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x33,
|
|
}
|
|
|
|
var (
|
|
file_github_com_moby_buildkit_util_stack_stack_proto_rawDescOnce sync.Once
|
|
file_github_com_moby_buildkit_util_stack_stack_proto_rawDescData = file_github_com_moby_buildkit_util_stack_stack_proto_rawDesc
|
|
)
|
|
|
|
func file_github_com_moby_buildkit_util_stack_stack_proto_rawDescGZIP() []byte {
|
|
file_github_com_moby_buildkit_util_stack_stack_proto_rawDescOnce.Do(func() {
|
|
file_github_com_moby_buildkit_util_stack_stack_proto_rawDescData = protoimpl.X.CompressGZIP(file_github_com_moby_buildkit_util_stack_stack_proto_rawDescData)
|
|
})
|
|
return file_github_com_moby_buildkit_util_stack_stack_proto_rawDescData
|
|
}
|
|
|
|
var file_github_com_moby_buildkit_util_stack_stack_proto_msgTypes = make([]protoimpl.MessageInfo, 2)
|
|
var file_github_com_moby_buildkit_util_stack_stack_proto_goTypes = []interface{}{
|
|
(*Stack)(nil), // 0: stack.Stack
|
|
(*Frame)(nil), // 1: stack.Frame
|
|
}
|
|
var file_github_com_moby_buildkit_util_stack_stack_proto_depIdxs = []int32{
|
|
1, // 0: stack.Stack.frames:type_name -> stack.Frame
|
|
1, // [1:1] is the sub-list for method output_type
|
|
1, // [1:1] is the sub-list for method input_type
|
|
1, // [1:1] is the sub-list for extension type_name
|
|
1, // [1:1] is the sub-list for extension extendee
|
|
0, // [0:1] is the sub-list for field type_name
|
|
}
|
|
|
|
func init() { file_github_com_moby_buildkit_util_stack_stack_proto_init() }
|
|
func file_github_com_moby_buildkit_util_stack_stack_proto_init() {
|
|
if File_github_com_moby_buildkit_util_stack_stack_proto != nil {
|
|
return
|
|
}
|
|
if !protoimpl.UnsafeEnabled {
|
|
file_github_com_moby_buildkit_util_stack_stack_proto_msgTypes[0].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*Stack); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_moby_buildkit_util_stack_stack_proto_msgTypes[1].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*Frame); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
}
|
|
type x struct{}
|
|
out := protoimpl.TypeBuilder{
|
|
File: protoimpl.DescBuilder{
|
|
GoPackagePath: reflect.TypeOf(x{}).PkgPath(),
|
|
RawDescriptor: file_github_com_moby_buildkit_util_stack_stack_proto_rawDesc,
|
|
NumEnums: 0,
|
|
NumMessages: 2,
|
|
NumExtensions: 0,
|
|
NumServices: 0,
|
|
},
|
|
GoTypes: file_github_com_moby_buildkit_util_stack_stack_proto_goTypes,
|
|
DependencyIndexes: file_github_com_moby_buildkit_util_stack_stack_proto_depIdxs,
|
|
MessageInfos: file_github_com_moby_buildkit_util_stack_stack_proto_msgTypes,
|
|
}.Build()
|
|
File_github_com_moby_buildkit_util_stack_stack_proto = out.File
|
|
file_github_com_moby_buildkit_util_stack_stack_proto_rawDesc = nil
|
|
file_github_com_moby_buildkit_util_stack_stack_proto_goTypes = nil
|
|
file_github_com_moby_buildkit_util_stack_stack_proto_depIdxs = nil
|
|
}
|