mirror of
https://gitea.com/Lydanne/buildx.git
synced 2025-05-18 00:47:48 +08:00
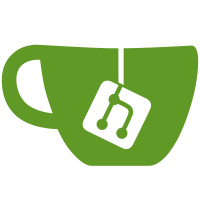
Introduce a meter provider to the buildx cli that will send metrics to the otel-collector included in docker desktop if enabled. This will send usage metrics to the desktop application but also send metrics to a user-provided otlp receiver endpoint through the standard environment variables. This introduces a single metric which is the cli count for build and bake along with the command name and a few additional attributes. Signed-off-by: Jonathan A. Sternberg <jonathan.sternberg@docker.com>
50 lines
1.5 KiB
Go
50 lines
1.5 KiB
Go
package metrics
|
|
|
|
import (
|
|
"context"
|
|
"os"
|
|
|
|
"github.com/pkg/errors"
|
|
"go.opentelemetry.io/otel/exporters/otlp/otlpmetric/otlpmetricgrpc"
|
|
"go.opentelemetry.io/otel/exporters/otlp/otlpmetric/otlpmetrichttp"
|
|
sdkmetric "go.opentelemetry.io/otel/sdk/metric"
|
|
)
|
|
|
|
// detectOtlpExporter configures a metrics exporter based on environment variables.
|
|
// This is similar to the version of this in buildkit, but we need direct access
|
|
// to the exporter and the prometheus exporter doesn't work at all in a CLI context.
|
|
//
|
|
// There's some duplication here which I hope to remove when the detect package
|
|
// is refactored or extracted from buildkit so it can be utilized here.
|
|
//
|
|
// This version of the exporter is public facing in contrast to the
|
|
// docker otel collector.
|
|
func detectOtlpExporter(ctx context.Context) (sdkmetric.Exporter, error) {
|
|
set := os.Getenv("OTEL_METRICS_EXPORTER") == "otlp" || os.Getenv("OTEL_EXPORTER_OTLP_ENDPOINT") != "" || os.Getenv("OTEL_EXPORTER_OTLP_METRICS_ENDPOINT") != ""
|
|
if !set {
|
|
return nil, nil
|
|
}
|
|
|
|
proto := os.Getenv("OTEL_EXPORTER_OTLP_METRICS_PROTOCOL")
|
|
if proto == "" {
|
|
proto = os.Getenv("OTEL_EXPORTER_OTLP_PROTOCOL")
|
|
}
|
|
if proto == "" {
|
|
proto = "grpc"
|
|
}
|
|
|
|
switch proto {
|
|
case "grpc":
|
|
return otlpmetricgrpc.New(ctx,
|
|
otlpmetricgrpc.WithTemporalitySelector(deltaTemporality),
|
|
)
|
|
case "http/protobuf":
|
|
return otlpmetrichttp.New(ctx,
|
|
otlpmetrichttp.WithTemporalitySelector(deltaTemporality),
|
|
)
|
|
// case "http/json": // unsupported by library
|
|
default:
|
|
return nil, errors.Errorf("unsupported otlp protocol %v", proto)
|
|
}
|
|
}
|