mirror of
https://gitea.com/Lydanne/buildx.git
synced 2025-07-02 09:28:36 +08:00
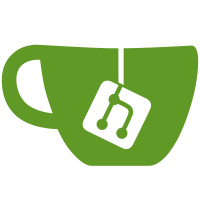
Remove the controller grpc service along with associated code related to sessions or remote controllers. Data types that are still used with complicated dependency chains have been kept in the same package for a future refactor. Signed-off-by: Jonathan A. Sternberg <jonathan.sternberg@docker.com>
45 lines
954 B
Go
45 lines
954 B
Go
package commands
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
"io"
|
|
"text/tabwriter"
|
|
|
|
"github.com/docker/buildx/monitor/types"
|
|
)
|
|
|
|
type PsCmd struct {
|
|
m types.Monitor
|
|
stdout io.WriteCloser
|
|
}
|
|
|
|
func NewPsCmd(m types.Monitor, stdout io.WriteCloser) types.Command {
|
|
return &PsCmd{m, stdout}
|
|
}
|
|
|
|
func (cm *PsCmd) Info() types.CommandInfo {
|
|
return types.CommandInfo{
|
|
Name: "ps",
|
|
HelpMessage: `list processes invoked by "exec". Use "attach" to attach IO to that process`,
|
|
HelpMessageLong: `
|
|
Usage:
|
|
ps
|
|
`,
|
|
}
|
|
}
|
|
|
|
func (cm *PsCmd) Exec(ctx context.Context, args []string) error {
|
|
plist, err := cm.m.ListProcesses(ctx)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
tw := tabwriter.NewWriter(cm.stdout, 1, 8, 1, '\t', 0)
|
|
fmt.Fprintln(tw, "PID\tCURRENT_SESSION\tCOMMAND")
|
|
for _, p := range plist {
|
|
fmt.Fprintf(tw, "%-20s\t%v\t%v\n", p.ProcessID, p.ProcessID == cm.m.AttachedPID(), append(p.InvokeConfig.Entrypoint, p.InvokeConfig.Cmd...))
|
|
}
|
|
tw.Flush()
|
|
return nil
|
|
}
|