mirror of
https://gitea.com/Lydanne/buildx.git
synced 2025-05-18 00:47:48 +08:00
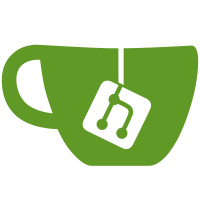
Update buildkit dependency to v0.14.0-rc1. Update the tracing infrastructure to use the new detect API which updates how the delegated exporter is configured. Signed-off-by: Jonathan A. Sternberg <jonathan.sternberg@docker.com>
59 lines
1.2 KiB
Go
59 lines
1.2 KiB
Go
package detect
|
|
|
|
import (
|
|
"context"
|
|
"os"
|
|
"path/filepath"
|
|
"sync"
|
|
|
|
"go.opentelemetry.io/otel"
|
|
"go.opentelemetry.io/otel/sdk/resource"
|
|
semconv "go.opentelemetry.io/otel/semconv/v1.21.0"
|
|
)
|
|
|
|
var (
|
|
ServiceName string
|
|
|
|
detectedResource *resource.Resource
|
|
detectedResourceOnce sync.Once
|
|
)
|
|
|
|
func Resource() *resource.Resource {
|
|
detectedResourceOnce.Do(func() {
|
|
res, err := resource.New(context.Background(),
|
|
resource.WithDetectors(serviceNameDetector{}),
|
|
resource.WithFromEnv(),
|
|
resource.WithTelemetrySDK(),
|
|
)
|
|
if err != nil {
|
|
otel.Handle(err)
|
|
}
|
|
detectedResource = res
|
|
})
|
|
return detectedResource
|
|
}
|
|
|
|
// OverrideResource overrides the resource returned from Resource.
|
|
//
|
|
// This must be invoked before Resource is called otherwise it is a no-op.
|
|
func OverrideResource(res *resource.Resource) {
|
|
detectedResourceOnce.Do(func() {
|
|
detectedResource = res
|
|
})
|
|
}
|
|
|
|
type serviceNameDetector struct{}
|
|
|
|
func (serviceNameDetector) Detect(ctx context.Context) (*resource.Resource, error) {
|
|
return resource.StringDetector(
|
|
semconv.SchemaURL,
|
|
semconv.ServiceNameKey,
|
|
func() (string, error) {
|
|
if ServiceName != "" {
|
|
return ServiceName, nil
|
|
}
|
|
return filepath.Base(os.Args[0]), nil
|
|
},
|
|
).Detect(ctx)
|
|
}
|