mirror of
https://gitea.com/Lydanne/buildx.git
synced 2025-07-04 10:27:43 +08:00
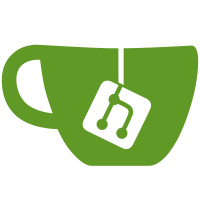
Removes gogo/protobuf from buildx and updates to a version of moby/buildkit where gogo is removed. This also changes how the proto files are generated. This is because newer versions of protobuf are more strict about name conflicts. If two files have the same name (even if they are relative paths) and are used in different protoc commands, they'll conflict in the registry. Since protobuf file generation doesn't work very well with `paths=source_relative`, this removes the `go:generate` expression and just relies on the dockerfile to perform the generation. Signed-off-by: Jonathan A. Sternberg <jonathan.sternberg@docker.com>
3360 lines
122 KiB
Go
3360 lines
122 KiB
Go
// Code generated by protoc-gen-go. DO NOT EDIT.
|
|
// versions:
|
|
// protoc-gen-go v1.34.1
|
|
// protoc v3.11.4
|
|
// source: github.com/docker/buildx/controller/pb/controller.proto
|
|
|
|
package pb
|
|
|
|
import (
|
|
control "github.com/moby/buildkit/api/services/control"
|
|
pb "github.com/moby/buildkit/sourcepolicy/pb"
|
|
protoreflect "google.golang.org/protobuf/reflect/protoreflect"
|
|
protoimpl "google.golang.org/protobuf/runtime/protoimpl"
|
|
reflect "reflect"
|
|
sync "sync"
|
|
)
|
|
|
|
const (
|
|
// Verify that this generated code is sufficiently up-to-date.
|
|
_ = protoimpl.EnforceVersion(20 - protoimpl.MinVersion)
|
|
// Verify that runtime/protoimpl is sufficiently up-to-date.
|
|
_ = protoimpl.EnforceVersion(protoimpl.MaxVersion - 20)
|
|
)
|
|
|
|
type ListProcessesRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Ref string `protobuf:"bytes,1,opt,name=Ref,proto3" json:"Ref,omitempty"`
|
|
}
|
|
|
|
func (x *ListProcessesRequest) Reset() {
|
|
*x = ListProcessesRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[0]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *ListProcessesRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*ListProcessesRequest) ProtoMessage() {}
|
|
|
|
func (x *ListProcessesRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[0]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use ListProcessesRequest.ProtoReflect.Descriptor instead.
|
|
func (*ListProcessesRequest) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{0}
|
|
}
|
|
|
|
func (x *ListProcessesRequest) GetRef() string {
|
|
if x != nil {
|
|
return x.Ref
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type ListProcessesResponse struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Infos []*ProcessInfo `protobuf:"bytes,1,rep,name=Infos,proto3" json:"Infos,omitempty"`
|
|
}
|
|
|
|
func (x *ListProcessesResponse) Reset() {
|
|
*x = ListProcessesResponse{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[1]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *ListProcessesResponse) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*ListProcessesResponse) ProtoMessage() {}
|
|
|
|
func (x *ListProcessesResponse) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[1]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use ListProcessesResponse.ProtoReflect.Descriptor instead.
|
|
func (*ListProcessesResponse) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{1}
|
|
}
|
|
|
|
func (x *ListProcessesResponse) GetInfos() []*ProcessInfo {
|
|
if x != nil {
|
|
return x.Infos
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type ProcessInfo struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
ProcessID string `protobuf:"bytes,1,opt,name=ProcessID,proto3" json:"ProcessID,omitempty"`
|
|
InvokeConfig *InvokeConfig `protobuf:"bytes,2,opt,name=InvokeConfig,proto3" json:"InvokeConfig,omitempty"`
|
|
}
|
|
|
|
func (x *ProcessInfo) Reset() {
|
|
*x = ProcessInfo{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[2]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *ProcessInfo) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*ProcessInfo) ProtoMessage() {}
|
|
|
|
func (x *ProcessInfo) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[2]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use ProcessInfo.ProtoReflect.Descriptor instead.
|
|
func (*ProcessInfo) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{2}
|
|
}
|
|
|
|
func (x *ProcessInfo) GetProcessID() string {
|
|
if x != nil {
|
|
return x.ProcessID
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *ProcessInfo) GetInvokeConfig() *InvokeConfig {
|
|
if x != nil {
|
|
return x.InvokeConfig
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type DisconnectProcessRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Ref string `protobuf:"bytes,1,opt,name=Ref,proto3" json:"Ref,omitempty"`
|
|
ProcessID string `protobuf:"bytes,2,opt,name=ProcessID,proto3" json:"ProcessID,omitempty"`
|
|
}
|
|
|
|
func (x *DisconnectProcessRequest) Reset() {
|
|
*x = DisconnectProcessRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[3]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *DisconnectProcessRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*DisconnectProcessRequest) ProtoMessage() {}
|
|
|
|
func (x *DisconnectProcessRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[3]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use DisconnectProcessRequest.ProtoReflect.Descriptor instead.
|
|
func (*DisconnectProcessRequest) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{3}
|
|
}
|
|
|
|
func (x *DisconnectProcessRequest) GetRef() string {
|
|
if x != nil {
|
|
return x.Ref
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *DisconnectProcessRequest) GetProcessID() string {
|
|
if x != nil {
|
|
return x.ProcessID
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type DisconnectProcessResponse struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
}
|
|
|
|
func (x *DisconnectProcessResponse) Reset() {
|
|
*x = DisconnectProcessResponse{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[4]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *DisconnectProcessResponse) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*DisconnectProcessResponse) ProtoMessage() {}
|
|
|
|
func (x *DisconnectProcessResponse) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[4]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use DisconnectProcessResponse.ProtoReflect.Descriptor instead.
|
|
func (*DisconnectProcessResponse) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{4}
|
|
}
|
|
|
|
type BuildRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Ref string `protobuf:"bytes,1,opt,name=Ref,proto3" json:"Ref,omitempty"`
|
|
Options *BuildOptions `protobuf:"bytes,2,opt,name=Options,proto3" json:"Options,omitempty"`
|
|
}
|
|
|
|
func (x *BuildRequest) Reset() {
|
|
*x = BuildRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[5]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *BuildRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*BuildRequest) ProtoMessage() {}
|
|
|
|
func (x *BuildRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[5]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use BuildRequest.ProtoReflect.Descriptor instead.
|
|
func (*BuildRequest) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{5}
|
|
}
|
|
|
|
func (x *BuildRequest) GetRef() string {
|
|
if x != nil {
|
|
return x.Ref
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *BuildRequest) GetOptions() *BuildOptions {
|
|
if x != nil {
|
|
return x.Options
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type BuildOptions struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
ContextPath string `protobuf:"bytes,1,opt,name=ContextPath,proto3" json:"ContextPath,omitempty"`
|
|
DockerfileName string `protobuf:"bytes,2,opt,name=DockerfileName,proto3" json:"DockerfileName,omitempty"`
|
|
CallFunc *CallFunc `protobuf:"bytes,3,opt,name=CallFunc,proto3" json:"CallFunc,omitempty"`
|
|
NamedContexts map[string]string `protobuf:"bytes,4,rep,name=NamedContexts,proto3" json:"NamedContexts,omitempty" protobuf_key:"bytes,1,opt,name=key,proto3" protobuf_val:"bytes,2,opt,name=value,proto3"`
|
|
Allow []string `protobuf:"bytes,5,rep,name=Allow,proto3" json:"Allow,omitempty"`
|
|
Attests []*Attest `protobuf:"bytes,6,rep,name=Attests,proto3" json:"Attests,omitempty"`
|
|
BuildArgs map[string]string `protobuf:"bytes,7,rep,name=BuildArgs,proto3" json:"BuildArgs,omitempty" protobuf_key:"bytes,1,opt,name=key,proto3" protobuf_val:"bytes,2,opt,name=value,proto3"`
|
|
CacheFrom []*CacheOptionsEntry `protobuf:"bytes,8,rep,name=CacheFrom,proto3" json:"CacheFrom,omitempty"`
|
|
CacheTo []*CacheOptionsEntry `protobuf:"bytes,9,rep,name=CacheTo,proto3" json:"CacheTo,omitempty"`
|
|
CgroupParent string `protobuf:"bytes,10,opt,name=CgroupParent,proto3" json:"CgroupParent,omitempty"`
|
|
Exports []*ExportEntry `protobuf:"bytes,11,rep,name=Exports,proto3" json:"Exports,omitempty"`
|
|
ExtraHosts []string `protobuf:"bytes,12,rep,name=ExtraHosts,proto3" json:"ExtraHosts,omitempty"`
|
|
Labels map[string]string `protobuf:"bytes,13,rep,name=Labels,proto3" json:"Labels,omitempty" protobuf_key:"bytes,1,opt,name=key,proto3" protobuf_val:"bytes,2,opt,name=value,proto3"`
|
|
NetworkMode string `protobuf:"bytes,14,opt,name=NetworkMode,proto3" json:"NetworkMode,omitempty"`
|
|
NoCacheFilter []string `protobuf:"bytes,15,rep,name=NoCacheFilter,proto3" json:"NoCacheFilter,omitempty"`
|
|
Platforms []string `protobuf:"bytes,16,rep,name=Platforms,proto3" json:"Platforms,omitempty"`
|
|
Secrets []*Secret `protobuf:"bytes,17,rep,name=Secrets,proto3" json:"Secrets,omitempty"`
|
|
ShmSize int64 `protobuf:"varint,18,opt,name=ShmSize,proto3" json:"ShmSize,omitempty"`
|
|
SSH []*SSH `protobuf:"bytes,19,rep,name=SSH,proto3" json:"SSH,omitempty"`
|
|
Tags []string `protobuf:"bytes,20,rep,name=Tags,proto3" json:"Tags,omitempty"`
|
|
Target string `protobuf:"bytes,21,opt,name=Target,proto3" json:"Target,omitempty"`
|
|
Ulimits *UlimitOpt `protobuf:"bytes,22,opt,name=Ulimits,proto3" json:"Ulimits,omitempty"`
|
|
Builder string `protobuf:"bytes,23,opt,name=Builder,proto3" json:"Builder,omitempty"`
|
|
NoCache bool `protobuf:"varint,24,opt,name=NoCache,proto3" json:"NoCache,omitempty"`
|
|
Pull bool `protobuf:"varint,25,opt,name=Pull,proto3" json:"Pull,omitempty"`
|
|
ExportPush bool `protobuf:"varint,26,opt,name=ExportPush,proto3" json:"ExportPush,omitempty"`
|
|
ExportLoad bool `protobuf:"varint,27,opt,name=ExportLoad,proto3" json:"ExportLoad,omitempty"`
|
|
SourcePolicy *pb.Policy `protobuf:"bytes,28,opt,name=SourcePolicy,proto3" json:"SourcePolicy,omitempty"`
|
|
Ref string `protobuf:"bytes,29,opt,name=Ref,proto3" json:"Ref,omitempty"`
|
|
GroupRef string `protobuf:"bytes,30,opt,name=GroupRef,proto3" json:"GroupRef,omitempty"`
|
|
Annotations []string `protobuf:"bytes,31,rep,name=Annotations,proto3" json:"Annotations,omitempty"`
|
|
ProvenanceResponseMode string `protobuf:"bytes,32,opt,name=ProvenanceResponseMode,proto3" json:"ProvenanceResponseMode,omitempty"`
|
|
}
|
|
|
|
func (x *BuildOptions) Reset() {
|
|
*x = BuildOptions{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[6]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *BuildOptions) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*BuildOptions) ProtoMessage() {}
|
|
|
|
func (x *BuildOptions) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[6]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use BuildOptions.ProtoReflect.Descriptor instead.
|
|
func (*BuildOptions) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{6}
|
|
}
|
|
|
|
func (x *BuildOptions) GetContextPath() string {
|
|
if x != nil {
|
|
return x.ContextPath
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *BuildOptions) GetDockerfileName() string {
|
|
if x != nil {
|
|
return x.DockerfileName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *BuildOptions) GetCallFunc() *CallFunc {
|
|
if x != nil {
|
|
return x.CallFunc
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BuildOptions) GetNamedContexts() map[string]string {
|
|
if x != nil {
|
|
return x.NamedContexts
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BuildOptions) GetAllow() []string {
|
|
if x != nil {
|
|
return x.Allow
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BuildOptions) GetAttests() []*Attest {
|
|
if x != nil {
|
|
return x.Attests
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BuildOptions) GetBuildArgs() map[string]string {
|
|
if x != nil {
|
|
return x.BuildArgs
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BuildOptions) GetCacheFrom() []*CacheOptionsEntry {
|
|
if x != nil {
|
|
return x.CacheFrom
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BuildOptions) GetCacheTo() []*CacheOptionsEntry {
|
|
if x != nil {
|
|
return x.CacheTo
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BuildOptions) GetCgroupParent() string {
|
|
if x != nil {
|
|
return x.CgroupParent
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *BuildOptions) GetExports() []*ExportEntry {
|
|
if x != nil {
|
|
return x.Exports
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BuildOptions) GetExtraHosts() []string {
|
|
if x != nil {
|
|
return x.ExtraHosts
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BuildOptions) GetLabels() map[string]string {
|
|
if x != nil {
|
|
return x.Labels
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BuildOptions) GetNetworkMode() string {
|
|
if x != nil {
|
|
return x.NetworkMode
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *BuildOptions) GetNoCacheFilter() []string {
|
|
if x != nil {
|
|
return x.NoCacheFilter
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BuildOptions) GetPlatforms() []string {
|
|
if x != nil {
|
|
return x.Platforms
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BuildOptions) GetSecrets() []*Secret {
|
|
if x != nil {
|
|
return x.Secrets
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BuildOptions) GetShmSize() int64 {
|
|
if x != nil {
|
|
return x.ShmSize
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *BuildOptions) GetSSH() []*SSH {
|
|
if x != nil {
|
|
return x.SSH
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BuildOptions) GetTags() []string {
|
|
if x != nil {
|
|
return x.Tags
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BuildOptions) GetTarget() string {
|
|
if x != nil {
|
|
return x.Target
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *BuildOptions) GetUlimits() *UlimitOpt {
|
|
if x != nil {
|
|
return x.Ulimits
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BuildOptions) GetBuilder() string {
|
|
if x != nil {
|
|
return x.Builder
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *BuildOptions) GetNoCache() bool {
|
|
if x != nil {
|
|
return x.NoCache
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *BuildOptions) GetPull() bool {
|
|
if x != nil {
|
|
return x.Pull
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *BuildOptions) GetExportPush() bool {
|
|
if x != nil {
|
|
return x.ExportPush
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *BuildOptions) GetExportLoad() bool {
|
|
if x != nil {
|
|
return x.ExportLoad
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *BuildOptions) GetSourcePolicy() *pb.Policy {
|
|
if x != nil {
|
|
return x.SourcePolicy
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BuildOptions) GetRef() string {
|
|
if x != nil {
|
|
return x.Ref
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *BuildOptions) GetGroupRef() string {
|
|
if x != nil {
|
|
return x.GroupRef
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *BuildOptions) GetAnnotations() []string {
|
|
if x != nil {
|
|
return x.Annotations
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BuildOptions) GetProvenanceResponseMode() string {
|
|
if x != nil {
|
|
return x.ProvenanceResponseMode
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type ExportEntry struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Type string `protobuf:"bytes,1,opt,name=Type,proto3" json:"Type,omitempty"`
|
|
Attrs map[string]string `protobuf:"bytes,2,rep,name=Attrs,proto3" json:"Attrs,omitempty" protobuf_key:"bytes,1,opt,name=key,proto3" protobuf_val:"bytes,2,opt,name=value,proto3"`
|
|
Destination string `protobuf:"bytes,3,opt,name=Destination,proto3" json:"Destination,omitempty"`
|
|
}
|
|
|
|
func (x *ExportEntry) Reset() {
|
|
*x = ExportEntry{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[7]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *ExportEntry) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*ExportEntry) ProtoMessage() {}
|
|
|
|
func (x *ExportEntry) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[7]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use ExportEntry.ProtoReflect.Descriptor instead.
|
|
func (*ExportEntry) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{7}
|
|
}
|
|
|
|
func (x *ExportEntry) GetType() string {
|
|
if x != nil {
|
|
return x.Type
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *ExportEntry) GetAttrs() map[string]string {
|
|
if x != nil {
|
|
return x.Attrs
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *ExportEntry) GetDestination() string {
|
|
if x != nil {
|
|
return x.Destination
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type CacheOptionsEntry struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Type string `protobuf:"bytes,1,opt,name=Type,proto3" json:"Type,omitempty"`
|
|
Attrs map[string]string `protobuf:"bytes,2,rep,name=Attrs,proto3" json:"Attrs,omitempty" protobuf_key:"bytes,1,opt,name=key,proto3" protobuf_val:"bytes,2,opt,name=value,proto3"`
|
|
}
|
|
|
|
func (x *CacheOptionsEntry) Reset() {
|
|
*x = CacheOptionsEntry{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[8]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *CacheOptionsEntry) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*CacheOptionsEntry) ProtoMessage() {}
|
|
|
|
func (x *CacheOptionsEntry) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[8]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use CacheOptionsEntry.ProtoReflect.Descriptor instead.
|
|
func (*CacheOptionsEntry) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{8}
|
|
}
|
|
|
|
func (x *CacheOptionsEntry) GetType() string {
|
|
if x != nil {
|
|
return x.Type
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *CacheOptionsEntry) GetAttrs() map[string]string {
|
|
if x != nil {
|
|
return x.Attrs
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type Attest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Type string `protobuf:"bytes,1,opt,name=Type,proto3" json:"Type,omitempty"`
|
|
Disabled bool `protobuf:"varint,2,opt,name=Disabled,proto3" json:"Disabled,omitempty"`
|
|
Attrs string `protobuf:"bytes,3,opt,name=Attrs,proto3" json:"Attrs,omitempty"`
|
|
}
|
|
|
|
func (x *Attest) Reset() {
|
|
*x = Attest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[9]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *Attest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*Attest) ProtoMessage() {}
|
|
|
|
func (x *Attest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[9]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use Attest.ProtoReflect.Descriptor instead.
|
|
func (*Attest) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{9}
|
|
}
|
|
|
|
func (x *Attest) GetType() string {
|
|
if x != nil {
|
|
return x.Type
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *Attest) GetDisabled() bool {
|
|
if x != nil {
|
|
return x.Disabled
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *Attest) GetAttrs() string {
|
|
if x != nil {
|
|
return x.Attrs
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type SSH struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
ID string `protobuf:"bytes,1,opt,name=ID,proto3" json:"ID,omitempty"`
|
|
Paths []string `protobuf:"bytes,2,rep,name=Paths,proto3" json:"Paths,omitempty"`
|
|
}
|
|
|
|
func (x *SSH) Reset() {
|
|
*x = SSH{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[10]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *SSH) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*SSH) ProtoMessage() {}
|
|
|
|
func (x *SSH) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[10]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use SSH.ProtoReflect.Descriptor instead.
|
|
func (*SSH) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{10}
|
|
}
|
|
|
|
func (x *SSH) GetID() string {
|
|
if x != nil {
|
|
return x.ID
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *SSH) GetPaths() []string {
|
|
if x != nil {
|
|
return x.Paths
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type Secret struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
ID string `protobuf:"bytes,1,opt,name=ID,proto3" json:"ID,omitempty"`
|
|
FilePath string `protobuf:"bytes,2,opt,name=FilePath,proto3" json:"FilePath,omitempty"`
|
|
Env string `protobuf:"bytes,3,opt,name=Env,proto3" json:"Env,omitempty"`
|
|
}
|
|
|
|
func (x *Secret) Reset() {
|
|
*x = Secret{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[11]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *Secret) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*Secret) ProtoMessage() {}
|
|
|
|
func (x *Secret) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[11]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use Secret.ProtoReflect.Descriptor instead.
|
|
func (*Secret) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{11}
|
|
}
|
|
|
|
func (x *Secret) GetID() string {
|
|
if x != nil {
|
|
return x.ID
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *Secret) GetFilePath() string {
|
|
if x != nil {
|
|
return x.FilePath
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *Secret) GetEnv() string {
|
|
if x != nil {
|
|
return x.Env
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type CallFunc struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Name string `protobuf:"bytes,1,opt,name=Name,proto3" json:"Name,omitempty"`
|
|
Format string `protobuf:"bytes,2,opt,name=Format,proto3" json:"Format,omitempty"`
|
|
IgnoreStatus bool `protobuf:"varint,3,opt,name=IgnoreStatus,proto3" json:"IgnoreStatus,omitempty"`
|
|
}
|
|
|
|
func (x *CallFunc) Reset() {
|
|
*x = CallFunc{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[12]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *CallFunc) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*CallFunc) ProtoMessage() {}
|
|
|
|
func (x *CallFunc) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[12]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use CallFunc.ProtoReflect.Descriptor instead.
|
|
func (*CallFunc) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{12}
|
|
}
|
|
|
|
func (x *CallFunc) GetName() string {
|
|
if x != nil {
|
|
return x.Name
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *CallFunc) GetFormat() string {
|
|
if x != nil {
|
|
return x.Format
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *CallFunc) GetIgnoreStatus() bool {
|
|
if x != nil {
|
|
return x.IgnoreStatus
|
|
}
|
|
return false
|
|
}
|
|
|
|
type InspectRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Ref string `protobuf:"bytes,1,opt,name=Ref,proto3" json:"Ref,omitempty"`
|
|
}
|
|
|
|
func (x *InspectRequest) Reset() {
|
|
*x = InspectRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[13]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *InspectRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*InspectRequest) ProtoMessage() {}
|
|
|
|
func (x *InspectRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[13]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use InspectRequest.ProtoReflect.Descriptor instead.
|
|
func (*InspectRequest) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{13}
|
|
}
|
|
|
|
func (x *InspectRequest) GetRef() string {
|
|
if x != nil {
|
|
return x.Ref
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type InspectResponse struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Options *BuildOptions `protobuf:"bytes,1,opt,name=Options,proto3" json:"Options,omitempty"`
|
|
}
|
|
|
|
func (x *InspectResponse) Reset() {
|
|
*x = InspectResponse{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[14]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *InspectResponse) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*InspectResponse) ProtoMessage() {}
|
|
|
|
func (x *InspectResponse) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[14]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use InspectResponse.ProtoReflect.Descriptor instead.
|
|
func (*InspectResponse) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{14}
|
|
}
|
|
|
|
func (x *InspectResponse) GetOptions() *BuildOptions {
|
|
if x != nil {
|
|
return x.Options
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type UlimitOpt struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Values map[string]*Ulimit `protobuf:"bytes,1,rep,name=values,proto3" json:"values,omitempty" protobuf_key:"bytes,1,opt,name=key,proto3" protobuf_val:"bytes,2,opt,name=value,proto3"`
|
|
}
|
|
|
|
func (x *UlimitOpt) Reset() {
|
|
*x = UlimitOpt{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[15]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *UlimitOpt) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*UlimitOpt) ProtoMessage() {}
|
|
|
|
func (x *UlimitOpt) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[15]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use UlimitOpt.ProtoReflect.Descriptor instead.
|
|
func (*UlimitOpt) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{15}
|
|
}
|
|
|
|
func (x *UlimitOpt) GetValues() map[string]*Ulimit {
|
|
if x != nil {
|
|
return x.Values
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type Ulimit struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Name string `protobuf:"bytes,1,opt,name=Name,proto3" json:"Name,omitempty"`
|
|
Hard int64 `protobuf:"varint,2,opt,name=Hard,proto3" json:"Hard,omitempty"`
|
|
Soft int64 `protobuf:"varint,3,opt,name=Soft,proto3" json:"Soft,omitempty"`
|
|
}
|
|
|
|
func (x *Ulimit) Reset() {
|
|
*x = Ulimit{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[16]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *Ulimit) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*Ulimit) ProtoMessage() {}
|
|
|
|
func (x *Ulimit) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[16]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use Ulimit.ProtoReflect.Descriptor instead.
|
|
func (*Ulimit) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{16}
|
|
}
|
|
|
|
func (x *Ulimit) GetName() string {
|
|
if x != nil {
|
|
return x.Name
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *Ulimit) GetHard() int64 {
|
|
if x != nil {
|
|
return x.Hard
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *Ulimit) GetSoft() int64 {
|
|
if x != nil {
|
|
return x.Soft
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type BuildResponse struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
ExporterResponse map[string]string `protobuf:"bytes,1,rep,name=ExporterResponse,proto3" json:"ExporterResponse,omitempty" protobuf_key:"bytes,1,opt,name=key,proto3" protobuf_val:"bytes,2,opt,name=value,proto3"`
|
|
}
|
|
|
|
func (x *BuildResponse) Reset() {
|
|
*x = BuildResponse{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[17]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *BuildResponse) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*BuildResponse) ProtoMessage() {}
|
|
|
|
func (x *BuildResponse) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[17]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use BuildResponse.ProtoReflect.Descriptor instead.
|
|
func (*BuildResponse) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{17}
|
|
}
|
|
|
|
func (x *BuildResponse) GetExporterResponse() map[string]string {
|
|
if x != nil {
|
|
return x.ExporterResponse
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type DisconnectRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Ref string `protobuf:"bytes,1,opt,name=Ref,proto3" json:"Ref,omitempty"`
|
|
}
|
|
|
|
func (x *DisconnectRequest) Reset() {
|
|
*x = DisconnectRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[18]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *DisconnectRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*DisconnectRequest) ProtoMessage() {}
|
|
|
|
func (x *DisconnectRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[18]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use DisconnectRequest.ProtoReflect.Descriptor instead.
|
|
func (*DisconnectRequest) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{18}
|
|
}
|
|
|
|
func (x *DisconnectRequest) GetRef() string {
|
|
if x != nil {
|
|
return x.Ref
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type DisconnectResponse struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
}
|
|
|
|
func (x *DisconnectResponse) Reset() {
|
|
*x = DisconnectResponse{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[19]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *DisconnectResponse) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*DisconnectResponse) ProtoMessage() {}
|
|
|
|
func (x *DisconnectResponse) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[19]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use DisconnectResponse.ProtoReflect.Descriptor instead.
|
|
func (*DisconnectResponse) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{19}
|
|
}
|
|
|
|
type ListRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Ref string `protobuf:"bytes,1,opt,name=Ref,proto3" json:"Ref,omitempty"`
|
|
}
|
|
|
|
func (x *ListRequest) Reset() {
|
|
*x = ListRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[20]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *ListRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*ListRequest) ProtoMessage() {}
|
|
|
|
func (x *ListRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[20]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use ListRequest.ProtoReflect.Descriptor instead.
|
|
func (*ListRequest) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{20}
|
|
}
|
|
|
|
func (x *ListRequest) GetRef() string {
|
|
if x != nil {
|
|
return x.Ref
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type ListResponse struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Keys []string `protobuf:"bytes,1,rep,name=keys,proto3" json:"keys,omitempty"`
|
|
}
|
|
|
|
func (x *ListResponse) Reset() {
|
|
*x = ListResponse{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[21]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *ListResponse) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*ListResponse) ProtoMessage() {}
|
|
|
|
func (x *ListResponse) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[21]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use ListResponse.ProtoReflect.Descriptor instead.
|
|
func (*ListResponse) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{21}
|
|
}
|
|
|
|
func (x *ListResponse) GetKeys() []string {
|
|
if x != nil {
|
|
return x.Keys
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type InputMessage struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
// Types that are assignable to Input:
|
|
//
|
|
// *InputMessage_Init
|
|
// *InputMessage_Data
|
|
Input isInputMessage_Input `protobuf_oneof:"Input"`
|
|
}
|
|
|
|
func (x *InputMessage) Reset() {
|
|
*x = InputMessage{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[22]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *InputMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*InputMessage) ProtoMessage() {}
|
|
|
|
func (x *InputMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[22]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use InputMessage.ProtoReflect.Descriptor instead.
|
|
func (*InputMessage) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{22}
|
|
}
|
|
|
|
func (m *InputMessage) GetInput() isInputMessage_Input {
|
|
if m != nil {
|
|
return m.Input
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *InputMessage) GetInit() *InputInitMessage {
|
|
if x, ok := x.GetInput().(*InputMessage_Init); ok {
|
|
return x.Init
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *InputMessage) GetData() *DataMessage {
|
|
if x, ok := x.GetInput().(*InputMessage_Data); ok {
|
|
return x.Data
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type isInputMessage_Input interface {
|
|
isInputMessage_Input()
|
|
}
|
|
|
|
type InputMessage_Init struct {
|
|
Init *InputInitMessage `protobuf:"bytes,1,opt,name=Init,proto3,oneof"`
|
|
}
|
|
|
|
type InputMessage_Data struct {
|
|
Data *DataMessage `protobuf:"bytes,2,opt,name=Data,proto3,oneof"`
|
|
}
|
|
|
|
func (*InputMessage_Init) isInputMessage_Input() {}
|
|
|
|
func (*InputMessage_Data) isInputMessage_Input() {}
|
|
|
|
type InputInitMessage struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Ref string `protobuf:"bytes,1,opt,name=Ref,proto3" json:"Ref,omitempty"`
|
|
}
|
|
|
|
func (x *InputInitMessage) Reset() {
|
|
*x = InputInitMessage{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[23]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *InputInitMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*InputInitMessage) ProtoMessage() {}
|
|
|
|
func (x *InputInitMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[23]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use InputInitMessage.ProtoReflect.Descriptor instead.
|
|
func (*InputInitMessage) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{23}
|
|
}
|
|
|
|
func (x *InputInitMessage) GetRef() string {
|
|
if x != nil {
|
|
return x.Ref
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type DataMessage struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
EOF bool `protobuf:"varint,1,opt,name=EOF,proto3" json:"EOF,omitempty"` // true if eof was reached
|
|
Data []byte `protobuf:"bytes,2,opt,name=Data,proto3" json:"Data,omitempty"` // should be chunked smaller than 4MB:
|
|
}
|
|
|
|
func (x *DataMessage) Reset() {
|
|
*x = DataMessage{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[24]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *DataMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*DataMessage) ProtoMessage() {}
|
|
|
|
func (x *DataMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[24]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use DataMessage.ProtoReflect.Descriptor instead.
|
|
func (*DataMessage) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{24}
|
|
}
|
|
|
|
func (x *DataMessage) GetEOF() bool {
|
|
if x != nil {
|
|
return x.EOF
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *DataMessage) GetData() []byte {
|
|
if x != nil {
|
|
return x.Data
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type InputResponse struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
}
|
|
|
|
func (x *InputResponse) Reset() {
|
|
*x = InputResponse{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[25]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *InputResponse) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*InputResponse) ProtoMessage() {}
|
|
|
|
func (x *InputResponse) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[25]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use InputResponse.ProtoReflect.Descriptor instead.
|
|
func (*InputResponse) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{25}
|
|
}
|
|
|
|
type Message struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
// Types that are assignable to Input:
|
|
//
|
|
// *Message_Init
|
|
// *Message_File
|
|
// *Message_Resize
|
|
// *Message_Signal
|
|
Input isMessage_Input `protobuf_oneof:"Input"`
|
|
}
|
|
|
|
func (x *Message) Reset() {
|
|
*x = Message{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[26]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *Message) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*Message) ProtoMessage() {}
|
|
|
|
func (x *Message) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[26]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use Message.ProtoReflect.Descriptor instead.
|
|
func (*Message) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{26}
|
|
}
|
|
|
|
func (m *Message) GetInput() isMessage_Input {
|
|
if m != nil {
|
|
return m.Input
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *Message) GetInit() *InitMessage {
|
|
if x, ok := x.GetInput().(*Message_Init); ok {
|
|
return x.Init
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *Message) GetFile() *FdMessage {
|
|
if x, ok := x.GetInput().(*Message_File); ok {
|
|
return x.File
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *Message) GetResize() *ResizeMessage {
|
|
if x, ok := x.GetInput().(*Message_Resize); ok {
|
|
return x.Resize
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *Message) GetSignal() *SignalMessage {
|
|
if x, ok := x.GetInput().(*Message_Signal); ok {
|
|
return x.Signal
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type isMessage_Input interface {
|
|
isMessage_Input()
|
|
}
|
|
|
|
type Message_Init struct {
|
|
Init *InitMessage `protobuf:"bytes,1,opt,name=Init,proto3,oneof"`
|
|
}
|
|
|
|
type Message_File struct {
|
|
// FdMessage used from client to server for input (stdin) and
|
|
// from server to client for output (stdout, stderr)
|
|
File *FdMessage `protobuf:"bytes,2,opt,name=File,proto3,oneof"`
|
|
}
|
|
|
|
type Message_Resize struct {
|
|
// ResizeMessage used from client to server for terminal resize events
|
|
Resize *ResizeMessage `protobuf:"bytes,3,opt,name=Resize,proto3,oneof"`
|
|
}
|
|
|
|
type Message_Signal struct {
|
|
// SignalMessage is used from client to server to send signal events
|
|
Signal *SignalMessage `protobuf:"bytes,4,opt,name=Signal,proto3,oneof"`
|
|
}
|
|
|
|
func (*Message_Init) isMessage_Input() {}
|
|
|
|
func (*Message_File) isMessage_Input() {}
|
|
|
|
func (*Message_Resize) isMessage_Input() {}
|
|
|
|
func (*Message_Signal) isMessage_Input() {}
|
|
|
|
type InitMessage struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Ref string `protobuf:"bytes,1,opt,name=Ref,proto3" json:"Ref,omitempty"`
|
|
// If ProcessID already exists in the server, it tries to connect to it
|
|
// instead of invoking the new one. In this case, InvokeConfig will be ignored.
|
|
ProcessID string `protobuf:"bytes,2,opt,name=ProcessID,proto3" json:"ProcessID,omitempty"`
|
|
InvokeConfig *InvokeConfig `protobuf:"bytes,3,opt,name=InvokeConfig,proto3" json:"InvokeConfig,omitempty"`
|
|
}
|
|
|
|
func (x *InitMessage) Reset() {
|
|
*x = InitMessage{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[27]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *InitMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*InitMessage) ProtoMessage() {}
|
|
|
|
func (x *InitMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[27]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use InitMessage.ProtoReflect.Descriptor instead.
|
|
func (*InitMessage) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{27}
|
|
}
|
|
|
|
func (x *InitMessage) GetRef() string {
|
|
if x != nil {
|
|
return x.Ref
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *InitMessage) GetProcessID() string {
|
|
if x != nil {
|
|
return x.ProcessID
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *InitMessage) GetInvokeConfig() *InvokeConfig {
|
|
if x != nil {
|
|
return x.InvokeConfig
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type InvokeConfig struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Entrypoint []string `protobuf:"bytes,1,rep,name=Entrypoint,proto3" json:"Entrypoint,omitempty"`
|
|
Cmd []string `protobuf:"bytes,2,rep,name=Cmd,proto3" json:"Cmd,omitempty"`
|
|
NoCmd bool `protobuf:"varint,11,opt,name=NoCmd,proto3" json:"NoCmd,omitempty"` // Do not set cmd but use the image's default
|
|
Env []string `protobuf:"bytes,3,rep,name=Env,proto3" json:"Env,omitempty"`
|
|
User string `protobuf:"bytes,4,opt,name=User,proto3" json:"User,omitempty"`
|
|
NoUser bool `protobuf:"varint,5,opt,name=NoUser,proto3" json:"NoUser,omitempty"` // Do not set user but use the image's default
|
|
Cwd string `protobuf:"bytes,6,opt,name=Cwd,proto3" json:"Cwd,omitempty"`
|
|
NoCwd bool `protobuf:"varint,7,opt,name=NoCwd,proto3" json:"NoCwd,omitempty"` // Do not set cwd but use the image's default
|
|
Tty bool `protobuf:"varint,8,opt,name=Tty,proto3" json:"Tty,omitempty"`
|
|
Rollback bool `protobuf:"varint,9,opt,name=Rollback,proto3" json:"Rollback,omitempty"` // Kill all process in the container and recreate it.
|
|
Initial bool `protobuf:"varint,10,opt,name=Initial,proto3" json:"Initial,omitempty"` // Run container from the initial state of that stage (supported only on the failed step)
|
|
}
|
|
|
|
func (x *InvokeConfig) Reset() {
|
|
*x = InvokeConfig{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[28]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *InvokeConfig) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*InvokeConfig) ProtoMessage() {}
|
|
|
|
func (x *InvokeConfig) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[28]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use InvokeConfig.ProtoReflect.Descriptor instead.
|
|
func (*InvokeConfig) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{28}
|
|
}
|
|
|
|
func (x *InvokeConfig) GetEntrypoint() []string {
|
|
if x != nil {
|
|
return x.Entrypoint
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *InvokeConfig) GetCmd() []string {
|
|
if x != nil {
|
|
return x.Cmd
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *InvokeConfig) GetNoCmd() bool {
|
|
if x != nil {
|
|
return x.NoCmd
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *InvokeConfig) GetEnv() []string {
|
|
if x != nil {
|
|
return x.Env
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *InvokeConfig) GetUser() string {
|
|
if x != nil {
|
|
return x.User
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *InvokeConfig) GetNoUser() bool {
|
|
if x != nil {
|
|
return x.NoUser
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *InvokeConfig) GetCwd() string {
|
|
if x != nil {
|
|
return x.Cwd
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *InvokeConfig) GetNoCwd() bool {
|
|
if x != nil {
|
|
return x.NoCwd
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *InvokeConfig) GetTty() bool {
|
|
if x != nil {
|
|
return x.Tty
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *InvokeConfig) GetRollback() bool {
|
|
if x != nil {
|
|
return x.Rollback
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *InvokeConfig) GetInitial() bool {
|
|
if x != nil {
|
|
return x.Initial
|
|
}
|
|
return false
|
|
}
|
|
|
|
type FdMessage struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Fd uint32 `protobuf:"varint,1,opt,name=Fd,proto3" json:"Fd,omitempty"` // what fd the data was from
|
|
EOF bool `protobuf:"varint,2,opt,name=EOF,proto3" json:"EOF,omitempty"` // true if eof was reached
|
|
Data []byte `protobuf:"bytes,3,opt,name=Data,proto3" json:"Data,omitempty"` // should be chunked smaller than 4MB:
|
|
}
|
|
|
|
func (x *FdMessage) Reset() {
|
|
*x = FdMessage{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[29]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *FdMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*FdMessage) ProtoMessage() {}
|
|
|
|
func (x *FdMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[29]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use FdMessage.ProtoReflect.Descriptor instead.
|
|
func (*FdMessage) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{29}
|
|
}
|
|
|
|
func (x *FdMessage) GetFd() uint32 {
|
|
if x != nil {
|
|
return x.Fd
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *FdMessage) GetEOF() bool {
|
|
if x != nil {
|
|
return x.EOF
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *FdMessage) GetData() []byte {
|
|
if x != nil {
|
|
return x.Data
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type ResizeMessage struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Rows uint32 `protobuf:"varint,1,opt,name=Rows,proto3" json:"Rows,omitempty"`
|
|
Cols uint32 `protobuf:"varint,2,opt,name=Cols,proto3" json:"Cols,omitempty"`
|
|
}
|
|
|
|
func (x *ResizeMessage) Reset() {
|
|
*x = ResizeMessage{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[30]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *ResizeMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*ResizeMessage) ProtoMessage() {}
|
|
|
|
func (x *ResizeMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[30]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use ResizeMessage.ProtoReflect.Descriptor instead.
|
|
func (*ResizeMessage) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{30}
|
|
}
|
|
|
|
func (x *ResizeMessage) GetRows() uint32 {
|
|
if x != nil {
|
|
return x.Rows
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *ResizeMessage) GetCols() uint32 {
|
|
if x != nil {
|
|
return x.Cols
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type SignalMessage struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
// we only send name (ie HUP, INT) because the int values
|
|
// are platform dependent.
|
|
Name string `protobuf:"bytes,1,opt,name=Name,proto3" json:"Name,omitempty"`
|
|
}
|
|
|
|
func (x *SignalMessage) Reset() {
|
|
*x = SignalMessage{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[31]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *SignalMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*SignalMessage) ProtoMessage() {}
|
|
|
|
func (x *SignalMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[31]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use SignalMessage.ProtoReflect.Descriptor instead.
|
|
func (*SignalMessage) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{31}
|
|
}
|
|
|
|
func (x *SignalMessage) GetName() string {
|
|
if x != nil {
|
|
return x.Name
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type StatusRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Ref string `protobuf:"bytes,1,opt,name=Ref,proto3" json:"Ref,omitempty"`
|
|
}
|
|
|
|
func (x *StatusRequest) Reset() {
|
|
*x = StatusRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[32]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *StatusRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*StatusRequest) ProtoMessage() {}
|
|
|
|
func (x *StatusRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[32]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use StatusRequest.ProtoReflect.Descriptor instead.
|
|
func (*StatusRequest) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{32}
|
|
}
|
|
|
|
func (x *StatusRequest) GetRef() string {
|
|
if x != nil {
|
|
return x.Ref
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type StatusResponse struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Vertexes []*control.Vertex `protobuf:"bytes,1,rep,name=vertexes,proto3" json:"vertexes,omitempty"`
|
|
Statuses []*control.VertexStatus `protobuf:"bytes,2,rep,name=statuses,proto3" json:"statuses,omitempty"`
|
|
Logs []*control.VertexLog `protobuf:"bytes,3,rep,name=logs,proto3" json:"logs,omitempty"`
|
|
Warnings []*control.VertexWarning `protobuf:"bytes,4,rep,name=warnings,proto3" json:"warnings,omitempty"`
|
|
}
|
|
|
|
func (x *StatusResponse) Reset() {
|
|
*x = StatusResponse{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[33]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *StatusResponse) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*StatusResponse) ProtoMessage() {}
|
|
|
|
func (x *StatusResponse) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[33]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use StatusResponse.ProtoReflect.Descriptor instead.
|
|
func (*StatusResponse) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{33}
|
|
}
|
|
|
|
func (x *StatusResponse) GetVertexes() []*control.Vertex {
|
|
if x != nil {
|
|
return x.Vertexes
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *StatusResponse) GetStatuses() []*control.VertexStatus {
|
|
if x != nil {
|
|
return x.Statuses
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *StatusResponse) GetLogs() []*control.VertexLog {
|
|
if x != nil {
|
|
return x.Logs
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *StatusResponse) GetWarnings() []*control.VertexWarning {
|
|
if x != nil {
|
|
return x.Warnings
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type InfoRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
}
|
|
|
|
func (x *InfoRequest) Reset() {
|
|
*x = InfoRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[34]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *InfoRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*InfoRequest) ProtoMessage() {}
|
|
|
|
func (x *InfoRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[34]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use InfoRequest.ProtoReflect.Descriptor instead.
|
|
func (*InfoRequest) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{34}
|
|
}
|
|
|
|
type InfoResponse struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
BuildxVersion *BuildxVersion `protobuf:"bytes,1,opt,name=buildxVersion,proto3" json:"buildxVersion,omitempty"`
|
|
}
|
|
|
|
func (x *InfoResponse) Reset() {
|
|
*x = InfoResponse{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[35]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *InfoResponse) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*InfoResponse) ProtoMessage() {}
|
|
|
|
func (x *InfoResponse) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[35]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use InfoResponse.ProtoReflect.Descriptor instead.
|
|
func (*InfoResponse) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{35}
|
|
}
|
|
|
|
func (x *InfoResponse) GetBuildxVersion() *BuildxVersion {
|
|
if x != nil {
|
|
return x.BuildxVersion
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type BuildxVersion struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Package string `protobuf:"bytes,1,opt,name=package,proto3" json:"package,omitempty"`
|
|
Version string `protobuf:"bytes,2,opt,name=version,proto3" json:"version,omitempty"`
|
|
Revision string `protobuf:"bytes,3,opt,name=revision,proto3" json:"revision,omitempty"`
|
|
}
|
|
|
|
func (x *BuildxVersion) Reset() {
|
|
*x = BuildxVersion{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[36]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *BuildxVersion) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*BuildxVersion) ProtoMessage() {}
|
|
|
|
func (x *BuildxVersion) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[36]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use BuildxVersion.ProtoReflect.Descriptor instead.
|
|
func (*BuildxVersion) Descriptor() ([]byte, []int) {
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP(), []int{36}
|
|
}
|
|
|
|
func (x *BuildxVersion) GetPackage() string {
|
|
if x != nil {
|
|
return x.Package
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *BuildxVersion) GetVersion() string {
|
|
if x != nil {
|
|
return x.Version
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *BuildxVersion) GetRevision() string {
|
|
if x != nil {
|
|
return x.Revision
|
|
}
|
|
return ""
|
|
}
|
|
|
|
var File_github_com_docker_buildx_controller_pb_controller_proto protoreflect.FileDescriptor
|
|
|
|
var file_github_com_docker_buildx_controller_pb_controller_proto_rawDesc = []byte{
|
|
0x0a, 0x37, 0x67, 0x69, 0x74, 0x68, 0x75, 0x62, 0x2e, 0x63, 0x6f, 0x6d, 0x2f, 0x64, 0x6f, 0x63,
|
|
0x6b, 0x65, 0x72, 0x2f, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x78, 0x2f, 0x63, 0x6f, 0x6e, 0x74, 0x72,
|
|
0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2f, 0x70, 0x62, 0x2f, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c,
|
|
0x6c, 0x65, 0x72, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x12, 0x14, 0x62, 0x75, 0x69, 0x6c, 0x64,
|
|
0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e, 0x76, 0x31, 0x1a,
|
|
0x3b, 0x67, 0x69, 0x74, 0x68, 0x75, 0x62, 0x2e, 0x63, 0x6f, 0x6d, 0x2f, 0x6d, 0x6f, 0x62, 0x79,
|
|
0x2f, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2f, 0x61, 0x70, 0x69, 0x2f, 0x73, 0x65,
|
|
0x72, 0x76, 0x69, 0x63, 0x65, 0x73, 0x2f, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x2f, 0x63,
|
|
0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x1a, 0x35, 0x67, 0x69,
|
|
0x74, 0x68, 0x75, 0x62, 0x2e, 0x63, 0x6f, 0x6d, 0x2f, 0x6d, 0x6f, 0x62, 0x79, 0x2f, 0x62, 0x75,
|
|
0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2f, 0x73, 0x6f, 0x75, 0x72, 0x63, 0x65, 0x70, 0x6f, 0x6c,
|
|
0x69, 0x63, 0x79, 0x2f, 0x70, 0x62, 0x2f, 0x70, 0x6f, 0x6c, 0x69, 0x63, 0x79, 0x2e, 0x70, 0x72,
|
|
0x6f, 0x74, 0x6f, 0x22, 0x28, 0x0a, 0x14, 0x4c, 0x69, 0x73, 0x74, 0x50, 0x72, 0x6f, 0x63, 0x65,
|
|
0x73, 0x73, 0x65, 0x73, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x12, 0x10, 0x0a, 0x03, 0x52,
|
|
0x65, 0x66, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x03, 0x52, 0x65, 0x66, 0x22, 0x50, 0x0a,
|
|
0x15, 0x4c, 0x69, 0x73, 0x74, 0x50, 0x72, 0x6f, 0x63, 0x65, 0x73, 0x73, 0x65, 0x73, 0x52, 0x65,
|
|
0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x37, 0x0a, 0x05, 0x49, 0x6e, 0x66, 0x6f, 0x73, 0x18,
|
|
0x01, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x21, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63,
|
|
0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e, 0x76, 0x31, 0x2e, 0x50, 0x72, 0x6f,
|
|
0x63, 0x65, 0x73, 0x73, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x05, 0x49, 0x6e, 0x66, 0x6f, 0x73, 0x22,
|
|
0x73, 0x0a, 0x0b, 0x50, 0x72, 0x6f, 0x63, 0x65, 0x73, 0x73, 0x49, 0x6e, 0x66, 0x6f, 0x12, 0x1c,
|
|
0x0a, 0x09, 0x50, 0x72, 0x6f, 0x63, 0x65, 0x73, 0x73, 0x49, 0x44, 0x18, 0x01, 0x20, 0x01, 0x28,
|
|
0x09, 0x52, 0x09, 0x50, 0x72, 0x6f, 0x63, 0x65, 0x73, 0x73, 0x49, 0x44, 0x12, 0x46, 0x0a, 0x0c,
|
|
0x49, 0x6e, 0x76, 0x6f, 0x6b, 0x65, 0x43, 0x6f, 0x6e, 0x66, 0x69, 0x67, 0x18, 0x02, 0x20, 0x01,
|
|
0x28, 0x0b, 0x32, 0x22, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74,
|
|
0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e, 0x76, 0x31, 0x2e, 0x49, 0x6e, 0x76, 0x6f, 0x6b, 0x65,
|
|
0x43, 0x6f, 0x6e, 0x66, 0x69, 0x67, 0x52, 0x0c, 0x49, 0x6e, 0x76, 0x6f, 0x6b, 0x65, 0x43, 0x6f,
|
|
0x6e, 0x66, 0x69, 0x67, 0x22, 0x4a, 0x0a, 0x18, 0x44, 0x69, 0x73, 0x63, 0x6f, 0x6e, 0x6e, 0x65,
|
|
0x63, 0x74, 0x50, 0x72, 0x6f, 0x63, 0x65, 0x73, 0x73, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74,
|
|
0x12, 0x10, 0x0a, 0x03, 0x52, 0x65, 0x66, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x03, 0x52,
|
|
0x65, 0x66, 0x12, 0x1c, 0x0a, 0x09, 0x50, 0x72, 0x6f, 0x63, 0x65, 0x73, 0x73, 0x49, 0x44, 0x18,
|
|
0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x09, 0x50, 0x72, 0x6f, 0x63, 0x65, 0x73, 0x73, 0x49, 0x44,
|
|
0x22, 0x1b, 0x0a, 0x19, 0x44, 0x69, 0x73, 0x63, 0x6f, 0x6e, 0x6e, 0x65, 0x63, 0x74, 0x50, 0x72,
|
|
0x6f, 0x63, 0x65, 0x73, 0x73, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x22, 0x5e, 0x0a,
|
|
0x0c, 0x42, 0x75, 0x69, 0x6c, 0x64, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x12, 0x10, 0x0a,
|
|
0x03, 0x52, 0x65, 0x66, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x03, 0x52, 0x65, 0x66, 0x12,
|
|
0x3c, 0x0a, 0x07, 0x4f, 0x70, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0b,
|
|
0x32, 0x22, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f,
|
|
0x6c, 0x6c, 0x65, 0x72, 0x2e, 0x76, 0x31, 0x2e, 0x42, 0x75, 0x69, 0x6c, 0x64, 0x4f, 0x70, 0x74,
|
|
0x69, 0x6f, 0x6e, 0x73, 0x52, 0x07, 0x4f, 0x70, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x22, 0xc5, 0x0c,
|
|
0x0a, 0x0c, 0x42, 0x75, 0x69, 0x6c, 0x64, 0x4f, 0x70, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x12, 0x20,
|
|
0x0a, 0x0b, 0x43, 0x6f, 0x6e, 0x74, 0x65, 0x78, 0x74, 0x50, 0x61, 0x74, 0x68, 0x18, 0x01, 0x20,
|
|
0x01, 0x28, 0x09, 0x52, 0x0b, 0x43, 0x6f, 0x6e, 0x74, 0x65, 0x78, 0x74, 0x50, 0x61, 0x74, 0x68,
|
|
0x12, 0x26, 0x0a, 0x0e, 0x44, 0x6f, 0x63, 0x6b, 0x65, 0x72, 0x66, 0x69, 0x6c, 0x65, 0x4e, 0x61,
|
|
0x6d, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0e, 0x44, 0x6f, 0x63, 0x6b, 0x65, 0x72,
|
|
0x66, 0x69, 0x6c, 0x65, 0x4e, 0x61, 0x6d, 0x65, 0x12, 0x3a, 0x0a, 0x08, 0x43, 0x61, 0x6c, 0x6c,
|
|
0x46, 0x75, 0x6e, 0x63, 0x18, 0x03, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1e, 0x2e, 0x62, 0x75, 0x69,
|
|
0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e, 0x76,
|
|
0x31, 0x2e, 0x43, 0x61, 0x6c, 0x6c, 0x46, 0x75, 0x6e, 0x63, 0x52, 0x08, 0x43, 0x61, 0x6c, 0x6c,
|
|
0x46, 0x75, 0x6e, 0x63, 0x12, 0x5b, 0x0a, 0x0d, 0x4e, 0x61, 0x6d, 0x65, 0x64, 0x43, 0x6f, 0x6e,
|
|
0x74, 0x65, 0x78, 0x74, 0x73, 0x18, 0x04, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x35, 0x2e, 0x62, 0x75,
|
|
0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e,
|
|
0x76, 0x31, 0x2e, 0x42, 0x75, 0x69, 0x6c, 0x64, 0x4f, 0x70, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x2e,
|
|
0x4e, 0x61, 0x6d, 0x65, 0x64, 0x43, 0x6f, 0x6e, 0x74, 0x65, 0x78, 0x74, 0x73, 0x45, 0x6e, 0x74,
|
|
0x72, 0x79, 0x52, 0x0d, 0x4e, 0x61, 0x6d, 0x65, 0x64, 0x43, 0x6f, 0x6e, 0x74, 0x65, 0x78, 0x74,
|
|
0x73, 0x12, 0x14, 0x0a, 0x05, 0x41, 0x6c, 0x6c, 0x6f, 0x77, 0x18, 0x05, 0x20, 0x03, 0x28, 0x09,
|
|
0x52, 0x05, 0x41, 0x6c, 0x6c, 0x6f, 0x77, 0x12, 0x36, 0x0a, 0x07, 0x41, 0x74, 0x74, 0x65, 0x73,
|
|
0x74, 0x73, 0x18, 0x06, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x1c, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64,
|
|
0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e, 0x76, 0x31, 0x2e,
|
|
0x41, 0x74, 0x74, 0x65, 0x73, 0x74, 0x52, 0x07, 0x41, 0x74, 0x74, 0x65, 0x73, 0x74, 0x73, 0x12,
|
|
0x4f, 0x0a, 0x09, 0x42, 0x75, 0x69, 0x6c, 0x64, 0x41, 0x72, 0x67, 0x73, 0x18, 0x07, 0x20, 0x03,
|
|
0x28, 0x0b, 0x32, 0x31, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74,
|
|
0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e, 0x76, 0x31, 0x2e, 0x42, 0x75, 0x69, 0x6c, 0x64, 0x4f,
|
|
0x70, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x2e, 0x42, 0x75, 0x69, 0x6c, 0x64, 0x41, 0x72, 0x67, 0x73,
|
|
0x45, 0x6e, 0x74, 0x72, 0x79, 0x52, 0x09, 0x42, 0x75, 0x69, 0x6c, 0x64, 0x41, 0x72, 0x67, 0x73,
|
|
0x12, 0x45, 0x0a, 0x09, 0x43, 0x61, 0x63, 0x68, 0x65, 0x46, 0x72, 0x6f, 0x6d, 0x18, 0x08, 0x20,
|
|
0x03, 0x28, 0x0b, 0x32, 0x27, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e,
|
|
0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e, 0x76, 0x31, 0x2e, 0x43, 0x61, 0x63, 0x68, 0x65,
|
|
0x4f, 0x70, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x52, 0x09, 0x43, 0x61,
|
|
0x63, 0x68, 0x65, 0x46, 0x72, 0x6f, 0x6d, 0x12, 0x41, 0x0a, 0x07, 0x43, 0x61, 0x63, 0x68, 0x65,
|
|
0x54, 0x6f, 0x18, 0x09, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x27, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64,
|
|
0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e, 0x76, 0x31, 0x2e,
|
|
0x43, 0x61, 0x63, 0x68, 0x65, 0x4f, 0x70, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x45, 0x6e, 0x74, 0x72,
|
|
0x79, 0x52, 0x07, 0x43, 0x61, 0x63, 0x68, 0x65, 0x54, 0x6f, 0x12, 0x22, 0x0a, 0x0c, 0x43, 0x67,
|
|
0x72, 0x6f, 0x75, 0x70, 0x50, 0x61, 0x72, 0x65, 0x6e, 0x74, 0x18, 0x0a, 0x20, 0x01, 0x28, 0x09,
|
|
0x52, 0x0c, 0x43, 0x67, 0x72, 0x6f, 0x75, 0x70, 0x50, 0x61, 0x72, 0x65, 0x6e, 0x74, 0x12, 0x3b,
|
|
0x0a, 0x07, 0x45, 0x78, 0x70, 0x6f, 0x72, 0x74, 0x73, 0x18, 0x0b, 0x20, 0x03, 0x28, 0x0b, 0x32,
|
|
0x21, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c,
|
|
0x6c, 0x65, 0x72, 0x2e, 0x76, 0x31, 0x2e, 0x45, 0x78, 0x70, 0x6f, 0x72, 0x74, 0x45, 0x6e, 0x74,
|
|
0x72, 0x79, 0x52, 0x07, 0x45, 0x78, 0x70, 0x6f, 0x72, 0x74, 0x73, 0x12, 0x1e, 0x0a, 0x0a, 0x45,
|
|
0x78, 0x74, 0x72, 0x61, 0x48, 0x6f, 0x73, 0x74, 0x73, 0x18, 0x0c, 0x20, 0x03, 0x28, 0x09, 0x52,
|
|
0x0a, 0x45, 0x78, 0x74, 0x72, 0x61, 0x48, 0x6f, 0x73, 0x74, 0x73, 0x12, 0x46, 0x0a, 0x06, 0x4c,
|
|
0x61, 0x62, 0x65, 0x6c, 0x73, 0x18, 0x0d, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x2e, 0x2e, 0x62, 0x75,
|
|
0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e,
|
|
0x76, 0x31, 0x2e, 0x42, 0x75, 0x69, 0x6c, 0x64, 0x4f, 0x70, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x2e,
|
|
0x4c, 0x61, 0x62, 0x65, 0x6c, 0x73, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x52, 0x06, 0x4c, 0x61, 0x62,
|
|
0x65, 0x6c, 0x73, 0x12, 0x20, 0x0a, 0x0b, 0x4e, 0x65, 0x74, 0x77, 0x6f, 0x72, 0x6b, 0x4d, 0x6f,
|
|
0x64, 0x65, 0x18, 0x0e, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0b, 0x4e, 0x65, 0x74, 0x77, 0x6f, 0x72,
|
|
0x6b, 0x4d, 0x6f, 0x64, 0x65, 0x12, 0x24, 0x0a, 0x0d, 0x4e, 0x6f, 0x43, 0x61, 0x63, 0x68, 0x65,
|
|
0x46, 0x69, 0x6c, 0x74, 0x65, 0x72, 0x18, 0x0f, 0x20, 0x03, 0x28, 0x09, 0x52, 0x0d, 0x4e, 0x6f,
|
|
0x43, 0x61, 0x63, 0x68, 0x65, 0x46, 0x69, 0x6c, 0x74, 0x65, 0x72, 0x12, 0x1c, 0x0a, 0x09, 0x50,
|
|
0x6c, 0x61, 0x74, 0x66, 0x6f, 0x72, 0x6d, 0x73, 0x18, 0x10, 0x20, 0x03, 0x28, 0x09, 0x52, 0x09,
|
|
0x50, 0x6c, 0x61, 0x74, 0x66, 0x6f, 0x72, 0x6d, 0x73, 0x12, 0x36, 0x0a, 0x07, 0x53, 0x65, 0x63,
|
|
0x72, 0x65, 0x74, 0x73, 0x18, 0x11, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x1c, 0x2e, 0x62, 0x75, 0x69,
|
|
0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e, 0x76,
|
|
0x31, 0x2e, 0x53, 0x65, 0x63, 0x72, 0x65, 0x74, 0x52, 0x07, 0x53, 0x65, 0x63, 0x72, 0x65, 0x74,
|
|
0x73, 0x12, 0x18, 0x0a, 0x07, 0x53, 0x68, 0x6d, 0x53, 0x69, 0x7a, 0x65, 0x18, 0x12, 0x20, 0x01,
|
|
0x28, 0x03, 0x52, 0x07, 0x53, 0x68, 0x6d, 0x53, 0x69, 0x7a, 0x65, 0x12, 0x2b, 0x0a, 0x03, 0x53,
|
|
0x53, 0x48, 0x18, 0x13, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x19, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64,
|
|
0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e, 0x76, 0x31, 0x2e,
|
|
0x53, 0x53, 0x48, 0x52, 0x03, 0x53, 0x53, 0x48, 0x12, 0x12, 0x0a, 0x04, 0x54, 0x61, 0x67, 0x73,
|
|
0x18, 0x14, 0x20, 0x03, 0x28, 0x09, 0x52, 0x04, 0x54, 0x61, 0x67, 0x73, 0x12, 0x16, 0x0a, 0x06,
|
|
0x54, 0x61, 0x72, 0x67, 0x65, 0x74, 0x18, 0x15, 0x20, 0x01, 0x28, 0x09, 0x52, 0x06, 0x54, 0x61,
|
|
0x72, 0x67, 0x65, 0x74, 0x12, 0x39, 0x0a, 0x07, 0x55, 0x6c, 0x69, 0x6d, 0x69, 0x74, 0x73, 0x18,
|
|
0x16, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1f, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63,
|
|
0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e, 0x76, 0x31, 0x2e, 0x55, 0x6c, 0x69,
|
|
0x6d, 0x69, 0x74, 0x4f, 0x70, 0x74, 0x52, 0x07, 0x55, 0x6c, 0x69, 0x6d, 0x69, 0x74, 0x73, 0x12,
|
|
0x18, 0x0a, 0x07, 0x42, 0x75, 0x69, 0x6c, 0x64, 0x65, 0x72, 0x18, 0x17, 0x20, 0x01, 0x28, 0x09,
|
|
0x52, 0x07, 0x42, 0x75, 0x69, 0x6c, 0x64, 0x65, 0x72, 0x12, 0x18, 0x0a, 0x07, 0x4e, 0x6f, 0x43,
|
|
0x61, 0x63, 0x68, 0x65, 0x18, 0x18, 0x20, 0x01, 0x28, 0x08, 0x52, 0x07, 0x4e, 0x6f, 0x43, 0x61,
|
|
0x63, 0x68, 0x65, 0x12, 0x12, 0x0a, 0x04, 0x50, 0x75, 0x6c, 0x6c, 0x18, 0x19, 0x20, 0x01, 0x28,
|
|
0x08, 0x52, 0x04, 0x50, 0x75, 0x6c, 0x6c, 0x12, 0x1e, 0x0a, 0x0a, 0x45, 0x78, 0x70, 0x6f, 0x72,
|
|
0x74, 0x50, 0x75, 0x73, 0x68, 0x18, 0x1a, 0x20, 0x01, 0x28, 0x08, 0x52, 0x0a, 0x45, 0x78, 0x70,
|
|
0x6f, 0x72, 0x74, 0x50, 0x75, 0x73, 0x68, 0x12, 0x1e, 0x0a, 0x0a, 0x45, 0x78, 0x70, 0x6f, 0x72,
|
|
0x74, 0x4c, 0x6f, 0x61, 0x64, 0x18, 0x1b, 0x20, 0x01, 0x28, 0x08, 0x52, 0x0a, 0x45, 0x78, 0x70,
|
|
0x6f, 0x72, 0x74, 0x4c, 0x6f, 0x61, 0x64, 0x12, 0x49, 0x0a, 0x0c, 0x53, 0x6f, 0x75, 0x72, 0x63,
|
|
0x65, 0x50, 0x6f, 0x6c, 0x69, 0x63, 0x79, 0x18, 0x1c, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x25, 0x2e,
|
|
0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31,
|
|
0x2e, 0x73, 0x6f, 0x75, 0x72, 0x63, 0x65, 0x70, 0x6f, 0x6c, 0x69, 0x63, 0x79, 0x2e, 0x50, 0x6f,
|
|
0x6c, 0x69, 0x63, 0x79, 0x52, 0x0c, 0x53, 0x6f, 0x75, 0x72, 0x63, 0x65, 0x50, 0x6f, 0x6c, 0x69,
|
|
0x63, 0x79, 0x12, 0x10, 0x0a, 0x03, 0x52, 0x65, 0x66, 0x18, 0x1d, 0x20, 0x01, 0x28, 0x09, 0x52,
|
|
0x03, 0x52, 0x65, 0x66, 0x12, 0x1a, 0x0a, 0x08, 0x47, 0x72, 0x6f, 0x75, 0x70, 0x52, 0x65, 0x66,
|
|
0x18, 0x1e, 0x20, 0x01, 0x28, 0x09, 0x52, 0x08, 0x47, 0x72, 0x6f, 0x75, 0x70, 0x52, 0x65, 0x66,
|
|
0x12, 0x20, 0x0a, 0x0b, 0x41, 0x6e, 0x6e, 0x6f, 0x74, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x18,
|
|
0x1f, 0x20, 0x03, 0x28, 0x09, 0x52, 0x0b, 0x41, 0x6e, 0x6e, 0x6f, 0x74, 0x61, 0x74, 0x69, 0x6f,
|
|
0x6e, 0x73, 0x12, 0x36, 0x0a, 0x16, 0x50, 0x72, 0x6f, 0x76, 0x65, 0x6e, 0x61, 0x6e, 0x63, 0x65,
|
|
0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x6f, 0x64, 0x65, 0x18, 0x20, 0x20, 0x01,
|
|
0x28, 0x09, 0x52, 0x16, 0x50, 0x72, 0x6f, 0x76, 0x65, 0x6e, 0x61, 0x6e, 0x63, 0x65, 0x52, 0x65,
|
|
0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x4d, 0x6f, 0x64, 0x65, 0x1a, 0x40, 0x0a, 0x12, 0x4e, 0x61,
|
|
0x6d, 0x65, 0x64, 0x43, 0x6f, 0x6e, 0x74, 0x65, 0x78, 0x74, 0x73, 0x45, 0x6e, 0x74, 0x72, 0x79,
|
|
0x12, 0x10, 0x0a, 0x03, 0x6b, 0x65, 0x79, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x03, 0x6b,
|
|
0x65, 0x79, 0x12, 0x14, 0x0a, 0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28,
|
|
0x09, 0x52, 0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x3a, 0x02, 0x38, 0x01, 0x1a, 0x3c, 0x0a, 0x0e,
|
|
0x42, 0x75, 0x69, 0x6c, 0x64, 0x41, 0x72, 0x67, 0x73, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x12, 0x10,
|
|
0x0a, 0x03, 0x6b, 0x65, 0x79, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x03, 0x6b, 0x65, 0x79,
|
|
0x12, 0x14, 0x0a, 0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52,
|
|
0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x3a, 0x02, 0x38, 0x01, 0x1a, 0x39, 0x0a, 0x0b, 0x4c, 0x61,
|
|
0x62, 0x65, 0x6c, 0x73, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x12, 0x10, 0x0a, 0x03, 0x6b, 0x65, 0x79,
|
|
0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x03, 0x6b, 0x65, 0x79, 0x12, 0x14, 0x0a, 0x05, 0x76,
|
|
0x61, 0x6c, 0x75, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x05, 0x76, 0x61, 0x6c, 0x75,
|
|
0x65, 0x3a, 0x02, 0x38, 0x01, 0x22, 0xc1, 0x01, 0x0a, 0x0b, 0x45, 0x78, 0x70, 0x6f, 0x72, 0x74,
|
|
0x45, 0x6e, 0x74, 0x72, 0x79, 0x12, 0x12, 0x0a, 0x04, 0x54, 0x79, 0x70, 0x65, 0x18, 0x01, 0x20,
|
|
0x01, 0x28, 0x09, 0x52, 0x04, 0x54, 0x79, 0x70, 0x65, 0x12, 0x42, 0x0a, 0x05, 0x41, 0x74, 0x74,
|
|
0x72, 0x73, 0x18, 0x02, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x2c, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64,
|
|
0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e, 0x76, 0x31, 0x2e,
|
|
0x45, 0x78, 0x70, 0x6f, 0x72, 0x74, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x2e, 0x41, 0x74, 0x74, 0x72,
|
|
0x73, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x52, 0x05, 0x41, 0x74, 0x74, 0x72, 0x73, 0x12, 0x20, 0x0a,
|
|
0x0b, 0x44, 0x65, 0x73, 0x74, 0x69, 0x6e, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x18, 0x03, 0x20, 0x01,
|
|
0x28, 0x09, 0x52, 0x0b, 0x44, 0x65, 0x73, 0x74, 0x69, 0x6e, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x1a,
|
|
0x38, 0x0a, 0x0a, 0x41, 0x74, 0x74, 0x72, 0x73, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x12, 0x10, 0x0a,
|
|
0x03, 0x6b, 0x65, 0x79, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x03, 0x6b, 0x65, 0x79, 0x12,
|
|
0x14, 0x0a, 0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x05,
|
|
0x76, 0x61, 0x6c, 0x75, 0x65, 0x3a, 0x02, 0x38, 0x01, 0x22, 0xab, 0x01, 0x0a, 0x11, 0x43, 0x61,
|
|
0x63, 0x68, 0x65, 0x4f, 0x70, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x12,
|
|
0x12, 0x0a, 0x04, 0x54, 0x79, 0x70, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x04, 0x54,
|
|
0x79, 0x70, 0x65, 0x12, 0x48, 0x0a, 0x05, 0x41, 0x74, 0x74, 0x72, 0x73, 0x18, 0x02, 0x20, 0x03,
|
|
0x28, 0x0b, 0x32, 0x32, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74,
|
|
0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e, 0x76, 0x31, 0x2e, 0x43, 0x61, 0x63, 0x68, 0x65, 0x4f,
|
|
0x70, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x2e, 0x41, 0x74, 0x74, 0x72,
|
|
0x73, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x52, 0x05, 0x41, 0x74, 0x74, 0x72, 0x73, 0x1a, 0x38, 0x0a,
|
|
0x0a, 0x41, 0x74, 0x74, 0x72, 0x73, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x12, 0x10, 0x0a, 0x03, 0x6b,
|
|
0x65, 0x79, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x03, 0x6b, 0x65, 0x79, 0x12, 0x14, 0x0a,
|
|
0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x05, 0x76, 0x61,
|
|
0x6c, 0x75, 0x65, 0x3a, 0x02, 0x38, 0x01, 0x22, 0x4e, 0x0a, 0x06, 0x41, 0x74, 0x74, 0x65, 0x73,
|
|
0x74, 0x12, 0x12, 0x0a, 0x04, 0x54, 0x79, 0x70, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52,
|
|
0x04, 0x54, 0x79, 0x70, 0x65, 0x12, 0x1a, 0x0a, 0x08, 0x44, 0x69, 0x73, 0x61, 0x62, 0x6c, 0x65,
|
|
0x64, 0x18, 0x02, 0x20, 0x01, 0x28, 0x08, 0x52, 0x08, 0x44, 0x69, 0x73, 0x61, 0x62, 0x6c, 0x65,
|
|
0x64, 0x12, 0x14, 0x0a, 0x05, 0x41, 0x74, 0x74, 0x72, 0x73, 0x18, 0x03, 0x20, 0x01, 0x28, 0x09,
|
|
0x52, 0x05, 0x41, 0x74, 0x74, 0x72, 0x73, 0x22, 0x2b, 0x0a, 0x03, 0x53, 0x53, 0x48, 0x12, 0x0e,
|
|
0x0a, 0x02, 0x49, 0x44, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x02, 0x49, 0x44, 0x12, 0x14,
|
|
0x0a, 0x05, 0x50, 0x61, 0x74, 0x68, 0x73, 0x18, 0x02, 0x20, 0x03, 0x28, 0x09, 0x52, 0x05, 0x50,
|
|
0x61, 0x74, 0x68, 0x73, 0x22, 0x46, 0x0a, 0x06, 0x53, 0x65, 0x63, 0x72, 0x65, 0x74, 0x12, 0x0e,
|
|
0x0a, 0x02, 0x49, 0x44, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x02, 0x49, 0x44, 0x12, 0x1a,
|
|
0x0a, 0x08, 0x46, 0x69, 0x6c, 0x65, 0x50, 0x61, 0x74, 0x68, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09,
|
|
0x52, 0x08, 0x46, 0x69, 0x6c, 0x65, 0x50, 0x61, 0x74, 0x68, 0x12, 0x10, 0x0a, 0x03, 0x45, 0x6e,
|
|
0x76, 0x18, 0x03, 0x20, 0x01, 0x28, 0x09, 0x52, 0x03, 0x45, 0x6e, 0x76, 0x22, 0x5a, 0x0a, 0x08,
|
|
0x43, 0x61, 0x6c, 0x6c, 0x46, 0x75, 0x6e, 0x63, 0x12, 0x12, 0x0a, 0x04, 0x4e, 0x61, 0x6d, 0x65,
|
|
0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x04, 0x4e, 0x61, 0x6d, 0x65, 0x12, 0x16, 0x0a, 0x06,
|
|
0x46, 0x6f, 0x72, 0x6d, 0x61, 0x74, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x06, 0x46, 0x6f,
|
|
0x72, 0x6d, 0x61, 0x74, 0x12, 0x22, 0x0a, 0x0c, 0x49, 0x67, 0x6e, 0x6f, 0x72, 0x65, 0x53, 0x74,
|
|
0x61, 0x74, 0x75, 0x73, 0x18, 0x03, 0x20, 0x01, 0x28, 0x08, 0x52, 0x0c, 0x49, 0x67, 0x6e, 0x6f,
|
|
0x72, 0x65, 0x53, 0x74, 0x61, 0x74, 0x75, 0x73, 0x22, 0x22, 0x0a, 0x0e, 0x49, 0x6e, 0x73, 0x70,
|
|
0x65, 0x63, 0x74, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x12, 0x10, 0x0a, 0x03, 0x52, 0x65,
|
|
0x66, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x03, 0x52, 0x65, 0x66, 0x22, 0x4f, 0x0a, 0x0f,
|
|
0x49, 0x6e, 0x73, 0x70, 0x65, 0x63, 0x74, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12,
|
|
0x3c, 0x0a, 0x07, 0x4f, 0x70, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0b,
|
|
0x32, 0x22, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f,
|
|
0x6c, 0x6c, 0x65, 0x72, 0x2e, 0x76, 0x31, 0x2e, 0x42, 0x75, 0x69, 0x6c, 0x64, 0x4f, 0x70, 0x74,
|
|
0x69, 0x6f, 0x6e, 0x73, 0x52, 0x07, 0x4f, 0x70, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x22, 0xa9, 0x01,
|
|
0x0a, 0x09, 0x55, 0x6c, 0x69, 0x6d, 0x69, 0x74, 0x4f, 0x70, 0x74, 0x12, 0x43, 0x0a, 0x06, 0x76,
|
|
0x61, 0x6c, 0x75, 0x65, 0x73, 0x18, 0x01, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x2b, 0x2e, 0x62, 0x75,
|
|
0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e,
|
|
0x76, 0x31, 0x2e, 0x55, 0x6c, 0x69, 0x6d, 0x69, 0x74, 0x4f, 0x70, 0x74, 0x2e, 0x56, 0x61, 0x6c,
|
|
0x75, 0x65, 0x73, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x52, 0x06, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x73,
|
|
0x1a, 0x57, 0x0a, 0x0b, 0x56, 0x61, 0x6c, 0x75, 0x65, 0x73, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x12,
|
|
0x10, 0x0a, 0x03, 0x6b, 0x65, 0x79, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x03, 0x6b, 0x65,
|
|
0x79, 0x12, 0x32, 0x0a, 0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0b,
|
|
0x32, 0x1c, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f,
|
|
0x6c, 0x6c, 0x65, 0x72, 0x2e, 0x76, 0x31, 0x2e, 0x55, 0x6c, 0x69, 0x6d, 0x69, 0x74, 0x52, 0x05,
|
|
0x76, 0x61, 0x6c, 0x75, 0x65, 0x3a, 0x02, 0x38, 0x01, 0x22, 0x44, 0x0a, 0x06, 0x55, 0x6c, 0x69,
|
|
0x6d, 0x69, 0x74, 0x12, 0x12, 0x0a, 0x04, 0x4e, 0x61, 0x6d, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28,
|
|
0x09, 0x52, 0x04, 0x4e, 0x61, 0x6d, 0x65, 0x12, 0x12, 0x0a, 0x04, 0x48, 0x61, 0x72, 0x64, 0x18,
|
|
0x02, 0x20, 0x01, 0x28, 0x03, 0x52, 0x04, 0x48, 0x61, 0x72, 0x64, 0x12, 0x12, 0x0a, 0x04, 0x53,
|
|
0x6f, 0x66, 0x74, 0x18, 0x03, 0x20, 0x01, 0x28, 0x03, 0x52, 0x04, 0x53, 0x6f, 0x66, 0x74, 0x22,
|
|
0xbb, 0x01, 0x0a, 0x0d, 0x42, 0x75, 0x69, 0x6c, 0x64, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73,
|
|
0x65, 0x12, 0x65, 0x0a, 0x10, 0x45, 0x78, 0x70, 0x6f, 0x72, 0x74, 0x65, 0x72, 0x52, 0x65, 0x73,
|
|
0x70, 0x6f, 0x6e, 0x73, 0x65, 0x18, 0x01, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x39, 0x2e, 0x62, 0x75,
|
|
0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e,
|
|
0x76, 0x31, 0x2e, 0x42, 0x75, 0x69, 0x6c, 0x64, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65,
|
|
0x2e, 0x45, 0x78, 0x70, 0x6f, 0x72, 0x74, 0x65, 0x72, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73,
|
|
0x65, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x52, 0x10, 0x45, 0x78, 0x70, 0x6f, 0x72, 0x74, 0x65, 0x72,
|
|
0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x1a, 0x43, 0x0a, 0x15, 0x45, 0x78, 0x70, 0x6f,
|
|
0x72, 0x74, 0x65, 0x72, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x45, 0x6e, 0x74, 0x72,
|
|
0x79, 0x12, 0x10, 0x0a, 0x03, 0x6b, 0x65, 0x79, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x03,
|
|
0x6b, 0x65, 0x79, 0x12, 0x14, 0x0a, 0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x18, 0x02, 0x20, 0x01,
|
|
0x28, 0x09, 0x52, 0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x3a, 0x02, 0x38, 0x01, 0x22, 0x25, 0x0a,
|
|
0x11, 0x44, 0x69, 0x73, 0x63, 0x6f, 0x6e, 0x6e, 0x65, 0x63, 0x74, 0x52, 0x65, 0x71, 0x75, 0x65,
|
|
0x73, 0x74, 0x12, 0x10, 0x0a, 0x03, 0x52, 0x65, 0x66, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52,
|
|
0x03, 0x52, 0x65, 0x66, 0x22, 0x14, 0x0a, 0x12, 0x44, 0x69, 0x73, 0x63, 0x6f, 0x6e, 0x6e, 0x65,
|
|
0x63, 0x74, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x22, 0x1f, 0x0a, 0x0b, 0x4c, 0x69,
|
|
0x73, 0x74, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x12, 0x10, 0x0a, 0x03, 0x52, 0x65, 0x66,
|
|
0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x03, 0x52, 0x65, 0x66, 0x22, 0x22, 0x0a, 0x0c, 0x4c,
|
|
0x69, 0x73, 0x74, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x12, 0x0a, 0x04, 0x6b,
|
|
0x65, 0x79, 0x73, 0x18, 0x01, 0x20, 0x03, 0x28, 0x09, 0x52, 0x04, 0x6b, 0x65, 0x79, 0x73, 0x22,
|
|
0x8e, 0x01, 0x0a, 0x0c, 0x49, 0x6e, 0x70, 0x75, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65,
|
|
0x12, 0x3c, 0x0a, 0x04, 0x49, 0x6e, 0x69, 0x74, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x26,
|
|
0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c,
|
|
0x65, 0x72, 0x2e, 0x76, 0x31, 0x2e, 0x49, 0x6e, 0x70, 0x75, 0x74, 0x49, 0x6e, 0x69, 0x74, 0x4d,
|
|
0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x48, 0x00, 0x52, 0x04, 0x49, 0x6e, 0x69, 0x74, 0x12, 0x37,
|
|
0x0a, 0x04, 0x44, 0x61, 0x74, 0x61, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x21, 0x2e, 0x62,
|
|
0x75, 0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72,
|
|
0x2e, 0x76, 0x31, 0x2e, 0x44, 0x61, 0x74, 0x61, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x48,
|
|
0x00, 0x52, 0x04, 0x44, 0x61, 0x74, 0x61, 0x42, 0x07, 0x0a, 0x05, 0x49, 0x6e, 0x70, 0x75, 0x74,
|
|
0x22, 0x24, 0x0a, 0x10, 0x49, 0x6e, 0x70, 0x75, 0x74, 0x49, 0x6e, 0x69, 0x74, 0x4d, 0x65, 0x73,
|
|
0x73, 0x61, 0x67, 0x65, 0x12, 0x10, 0x0a, 0x03, 0x52, 0x65, 0x66, 0x18, 0x01, 0x20, 0x01, 0x28,
|
|
0x09, 0x52, 0x03, 0x52, 0x65, 0x66, 0x22, 0x33, 0x0a, 0x0b, 0x44, 0x61, 0x74, 0x61, 0x4d, 0x65,
|
|
0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x10, 0x0a, 0x03, 0x45, 0x4f, 0x46, 0x18, 0x01, 0x20, 0x01,
|
|
0x28, 0x08, 0x52, 0x03, 0x45, 0x4f, 0x46, 0x12, 0x12, 0x0a, 0x04, 0x44, 0x61, 0x74, 0x61, 0x18,
|
|
0x02, 0x20, 0x01, 0x28, 0x0c, 0x52, 0x04, 0x44, 0x61, 0x74, 0x61, 0x22, 0x0f, 0x0a, 0x0d, 0x49,
|
|
0x6e, 0x70, 0x75, 0x74, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x22, 0x80, 0x02, 0x0a,
|
|
0x07, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x37, 0x0a, 0x04, 0x49, 0x6e, 0x69, 0x74,
|
|
0x18, 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x21, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x78, 0x2e,
|
|
0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e, 0x76, 0x31, 0x2e, 0x49, 0x6e,
|
|
0x69, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x48, 0x00, 0x52, 0x04, 0x49, 0x6e, 0x69,
|
|
0x74, 0x12, 0x35, 0x0a, 0x04, 0x46, 0x69, 0x6c, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0b, 0x32,
|
|
0x1f, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c,
|
|
0x6c, 0x65, 0x72, 0x2e, 0x76, 0x31, 0x2e, 0x46, 0x64, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65,
|
|
0x48, 0x00, 0x52, 0x04, 0x46, 0x69, 0x6c, 0x65, 0x12, 0x3d, 0x0a, 0x06, 0x52, 0x65, 0x73, 0x69,
|
|
0x7a, 0x65, 0x18, 0x03, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x23, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64,
|
|
0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e, 0x76, 0x31, 0x2e,
|
|
0x52, 0x65, 0x73, 0x69, 0x7a, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x48, 0x00, 0x52,
|
|
0x06, 0x52, 0x65, 0x73, 0x69, 0x7a, 0x65, 0x12, 0x3d, 0x0a, 0x06, 0x53, 0x69, 0x67, 0x6e, 0x61,
|
|
0x6c, 0x18, 0x04, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x23, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x78,
|
|
0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e, 0x76, 0x31, 0x2e, 0x53,
|
|
0x69, 0x67, 0x6e, 0x61, 0x6c, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x48, 0x00, 0x52, 0x06,
|
|
0x53, 0x69, 0x67, 0x6e, 0x61, 0x6c, 0x42, 0x07, 0x0a, 0x05, 0x49, 0x6e, 0x70, 0x75, 0x74, 0x22,
|
|
0x85, 0x01, 0x0a, 0x0b, 0x49, 0x6e, 0x69, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12,
|
|
0x10, 0x0a, 0x03, 0x52, 0x65, 0x66, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x03, 0x52, 0x65,
|
|
0x66, 0x12, 0x1c, 0x0a, 0x09, 0x50, 0x72, 0x6f, 0x63, 0x65, 0x73, 0x73, 0x49, 0x44, 0x18, 0x02,
|
|
0x20, 0x01, 0x28, 0x09, 0x52, 0x09, 0x50, 0x72, 0x6f, 0x63, 0x65, 0x73, 0x73, 0x49, 0x44, 0x12,
|
|
0x46, 0x0a, 0x0c, 0x49, 0x6e, 0x76, 0x6f, 0x6b, 0x65, 0x43, 0x6f, 0x6e, 0x66, 0x69, 0x67, 0x18,
|
|
0x03, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x22, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63,
|
|
0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e, 0x76, 0x31, 0x2e, 0x49, 0x6e, 0x76,
|
|
0x6f, 0x6b, 0x65, 0x43, 0x6f, 0x6e, 0x66, 0x69, 0x67, 0x52, 0x0c, 0x49, 0x6e, 0x76, 0x6f, 0x6b,
|
|
0x65, 0x43, 0x6f, 0x6e, 0x66, 0x69, 0x67, 0x22, 0x84, 0x02, 0x0a, 0x0c, 0x49, 0x6e, 0x76, 0x6f,
|
|
0x6b, 0x65, 0x43, 0x6f, 0x6e, 0x66, 0x69, 0x67, 0x12, 0x1e, 0x0a, 0x0a, 0x45, 0x6e, 0x74, 0x72,
|
|
0x79, 0x70, 0x6f, 0x69, 0x6e, 0x74, 0x18, 0x01, 0x20, 0x03, 0x28, 0x09, 0x52, 0x0a, 0x45, 0x6e,
|
|
0x74, 0x72, 0x79, 0x70, 0x6f, 0x69, 0x6e, 0x74, 0x12, 0x10, 0x0a, 0x03, 0x43, 0x6d, 0x64, 0x18,
|
|
0x02, 0x20, 0x03, 0x28, 0x09, 0x52, 0x03, 0x43, 0x6d, 0x64, 0x12, 0x14, 0x0a, 0x05, 0x4e, 0x6f,
|
|
0x43, 0x6d, 0x64, 0x18, 0x0b, 0x20, 0x01, 0x28, 0x08, 0x52, 0x05, 0x4e, 0x6f, 0x43, 0x6d, 0x64,
|
|
0x12, 0x10, 0x0a, 0x03, 0x45, 0x6e, 0x76, 0x18, 0x03, 0x20, 0x03, 0x28, 0x09, 0x52, 0x03, 0x45,
|
|
0x6e, 0x76, 0x12, 0x12, 0x0a, 0x04, 0x55, 0x73, 0x65, 0x72, 0x18, 0x04, 0x20, 0x01, 0x28, 0x09,
|
|
0x52, 0x04, 0x55, 0x73, 0x65, 0x72, 0x12, 0x16, 0x0a, 0x06, 0x4e, 0x6f, 0x55, 0x73, 0x65, 0x72,
|
|
0x18, 0x05, 0x20, 0x01, 0x28, 0x08, 0x52, 0x06, 0x4e, 0x6f, 0x55, 0x73, 0x65, 0x72, 0x12, 0x10,
|
|
0x0a, 0x03, 0x43, 0x77, 0x64, 0x18, 0x06, 0x20, 0x01, 0x28, 0x09, 0x52, 0x03, 0x43, 0x77, 0x64,
|
|
0x12, 0x14, 0x0a, 0x05, 0x4e, 0x6f, 0x43, 0x77, 0x64, 0x18, 0x07, 0x20, 0x01, 0x28, 0x08, 0x52,
|
|
0x05, 0x4e, 0x6f, 0x43, 0x77, 0x64, 0x12, 0x10, 0x0a, 0x03, 0x54, 0x74, 0x79, 0x18, 0x08, 0x20,
|
|
0x01, 0x28, 0x08, 0x52, 0x03, 0x54, 0x74, 0x79, 0x12, 0x1a, 0x0a, 0x08, 0x52, 0x6f, 0x6c, 0x6c,
|
|
0x62, 0x61, 0x63, 0x6b, 0x18, 0x09, 0x20, 0x01, 0x28, 0x08, 0x52, 0x08, 0x52, 0x6f, 0x6c, 0x6c,
|
|
0x62, 0x61, 0x63, 0x6b, 0x12, 0x18, 0x0a, 0x07, 0x49, 0x6e, 0x69, 0x74, 0x69, 0x61, 0x6c, 0x18,
|
|
0x0a, 0x20, 0x01, 0x28, 0x08, 0x52, 0x07, 0x49, 0x6e, 0x69, 0x74, 0x69, 0x61, 0x6c, 0x22, 0x41,
|
|
0x0a, 0x09, 0x46, 0x64, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x0e, 0x0a, 0x02, 0x46,
|
|
0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0d, 0x52, 0x02, 0x46, 0x64, 0x12, 0x10, 0x0a, 0x03, 0x45,
|
|
0x4f, 0x46, 0x18, 0x02, 0x20, 0x01, 0x28, 0x08, 0x52, 0x03, 0x45, 0x4f, 0x46, 0x12, 0x12, 0x0a,
|
|
0x04, 0x44, 0x61, 0x74, 0x61, 0x18, 0x03, 0x20, 0x01, 0x28, 0x0c, 0x52, 0x04, 0x44, 0x61, 0x74,
|
|
0x61, 0x22, 0x37, 0x0a, 0x0d, 0x52, 0x65, 0x73, 0x69, 0x7a, 0x65, 0x4d, 0x65, 0x73, 0x73, 0x61,
|
|
0x67, 0x65, 0x12, 0x12, 0x0a, 0x04, 0x52, 0x6f, 0x77, 0x73, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0d,
|
|
0x52, 0x04, 0x52, 0x6f, 0x77, 0x73, 0x12, 0x12, 0x0a, 0x04, 0x43, 0x6f, 0x6c, 0x73, 0x18, 0x02,
|
|
0x20, 0x01, 0x28, 0x0d, 0x52, 0x04, 0x43, 0x6f, 0x6c, 0x73, 0x22, 0x23, 0x0a, 0x0d, 0x53, 0x69,
|
|
0x67, 0x6e, 0x61, 0x6c, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12, 0x12, 0x0a, 0x04, 0x4e,
|
|
0x61, 0x6d, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x04, 0x4e, 0x61, 0x6d, 0x65, 0x22,
|
|
0x21, 0x0a, 0x0d, 0x53, 0x74, 0x61, 0x74, 0x75, 0x73, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74,
|
|
0x12, 0x10, 0x0a, 0x03, 0x52, 0x65, 0x66, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x03, 0x52,
|
|
0x65, 0x66, 0x22, 0xf0, 0x01, 0x0a, 0x0e, 0x53, 0x74, 0x61, 0x74, 0x75, 0x73, 0x52, 0x65, 0x73,
|
|
0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x34, 0x0a, 0x08, 0x76, 0x65, 0x72, 0x74, 0x65, 0x78, 0x65,
|
|
0x73, 0x18, 0x01, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x18, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62,
|
|
0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x56, 0x65, 0x72, 0x74, 0x65,
|
|
0x78, 0x52, 0x08, 0x76, 0x65, 0x72, 0x74, 0x65, 0x78, 0x65, 0x73, 0x12, 0x3a, 0x0a, 0x08, 0x73,
|
|
0x74, 0x61, 0x74, 0x75, 0x73, 0x65, 0x73, 0x18, 0x02, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x1e, 0x2e,
|
|
0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31,
|
|
0x2e, 0x56, 0x65, 0x72, 0x74, 0x65, 0x78, 0x53, 0x74, 0x61, 0x74, 0x75, 0x73, 0x52, 0x08, 0x73,
|
|
0x74, 0x61, 0x74, 0x75, 0x73, 0x65, 0x73, 0x12, 0x2f, 0x0a, 0x04, 0x6c, 0x6f, 0x67, 0x73, 0x18,
|
|
0x03, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x1b, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69,
|
|
0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x56, 0x65, 0x72, 0x74, 0x65, 0x78, 0x4c,
|
|
0x6f, 0x67, 0x52, 0x04, 0x6c, 0x6f, 0x67, 0x73, 0x12, 0x3b, 0x0a, 0x08, 0x77, 0x61, 0x72, 0x6e,
|
|
0x69, 0x6e, 0x67, 0x73, 0x18, 0x04, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x1f, 0x2e, 0x6d, 0x6f, 0x62,
|
|
0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x56, 0x65,
|
|
0x72, 0x74, 0x65, 0x78, 0x57, 0x61, 0x72, 0x6e, 0x69, 0x6e, 0x67, 0x52, 0x08, 0x77, 0x61, 0x72,
|
|
0x6e, 0x69, 0x6e, 0x67, 0x73, 0x22, 0x0d, 0x0a, 0x0b, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x71,
|
|
0x75, 0x65, 0x73, 0x74, 0x22, 0x59, 0x0a, 0x0c, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x73, 0x70,
|
|
0x6f, 0x6e, 0x73, 0x65, 0x12, 0x49, 0x0a, 0x0d, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x78, 0x56, 0x65,
|
|
0x72, 0x73, 0x69, 0x6f, 0x6e, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x23, 0x2e, 0x62, 0x75,
|
|
0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e,
|
|
0x76, 0x31, 0x2e, 0x42, 0x75, 0x69, 0x6c, 0x64, 0x78, 0x56, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e,
|
|
0x52, 0x0d, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x78, 0x56, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x22,
|
|
0x5f, 0x0a, 0x0d, 0x42, 0x75, 0x69, 0x6c, 0x64, 0x78, 0x56, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e,
|
|
0x12, 0x18, 0x0a, 0x07, 0x70, 0x61, 0x63, 0x6b, 0x61, 0x67, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28,
|
|
0x09, 0x52, 0x07, 0x70, 0x61, 0x63, 0x6b, 0x61, 0x67, 0x65, 0x12, 0x18, 0x0a, 0x07, 0x76, 0x65,
|
|
0x72, 0x73, 0x69, 0x6f, 0x6e, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x07, 0x76, 0x65, 0x72,
|
|
0x73, 0x69, 0x6f, 0x6e, 0x12, 0x1a, 0x0a, 0x08, 0x72, 0x65, 0x76, 0x69, 0x73, 0x69, 0x6f, 0x6e,
|
|
0x18, 0x03, 0x20, 0x01, 0x28, 0x09, 0x52, 0x08, 0x72, 0x65, 0x76, 0x69, 0x73, 0x69, 0x6f, 0x6e,
|
|
0x32, 0x8c, 0x07, 0x0a, 0x0a, 0x43, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x12,
|
|
0x50, 0x0a, 0x05, 0x42, 0x75, 0x69, 0x6c, 0x64, 0x12, 0x22, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64,
|
|
0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e, 0x76, 0x31, 0x2e,
|
|
0x42, 0x75, 0x69, 0x6c, 0x64, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x23, 0x2e, 0x62,
|
|
0x75, 0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72,
|
|
0x2e, 0x76, 0x31, 0x2e, 0x42, 0x75, 0x69, 0x6c, 0x64, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73,
|
|
0x65, 0x12, 0x56, 0x0a, 0x07, 0x49, 0x6e, 0x73, 0x70, 0x65, 0x63, 0x74, 0x12, 0x24, 0x2e, 0x62,
|
|
0x75, 0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72,
|
|
0x2e, 0x76, 0x31, 0x2e, 0x49, 0x6e, 0x73, 0x70, 0x65, 0x63, 0x74, 0x52, 0x65, 0x71, 0x75, 0x65,
|
|
0x73, 0x74, 0x1a, 0x25, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74,
|
|
0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e, 0x76, 0x31, 0x2e, 0x49, 0x6e, 0x73, 0x70, 0x65, 0x63,
|
|
0x74, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x55, 0x0a, 0x06, 0x53, 0x74, 0x61,
|
|
0x74, 0x75, 0x73, 0x12, 0x23, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e,
|
|
0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e, 0x76, 0x31, 0x2e, 0x53, 0x74, 0x61, 0x74, 0x75,
|
|
0x73, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x24, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64,
|
|
0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e, 0x76, 0x31, 0x2e,
|
|
0x53, 0x74, 0x61, 0x74, 0x75, 0x73, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x30, 0x01,
|
|
0x12, 0x52, 0x0a, 0x05, 0x49, 0x6e, 0x70, 0x75, 0x74, 0x12, 0x22, 0x2e, 0x62, 0x75, 0x69, 0x6c,
|
|
0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e, 0x76, 0x31,
|
|
0x2e, 0x49, 0x6e, 0x70, 0x75, 0x74, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x1a, 0x23, 0x2e,
|
|
0x62, 0x75, 0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65,
|
|
0x72, 0x2e, 0x76, 0x31, 0x2e, 0x49, 0x6e, 0x70, 0x75, 0x74, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e,
|
|
0x73, 0x65, 0x28, 0x01, 0x12, 0x4a, 0x0a, 0x06, 0x49, 0x6e, 0x76, 0x6f, 0x6b, 0x65, 0x12, 0x1d,
|
|
0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c,
|
|
0x65, 0x72, 0x2e, 0x76, 0x31, 0x2e, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x1a, 0x1d, 0x2e,
|
|
0x62, 0x75, 0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65,
|
|
0x72, 0x2e, 0x76, 0x31, 0x2e, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x28, 0x01, 0x30, 0x01,
|
|
0x12, 0x4d, 0x0a, 0x04, 0x4c, 0x69, 0x73, 0x74, 0x12, 0x21, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64,
|
|
0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e, 0x76, 0x31, 0x2e,
|
|
0x4c, 0x69, 0x73, 0x74, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x22, 0x2e, 0x62, 0x75,
|
|
0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e,
|
|
0x76, 0x31, 0x2e, 0x4c, 0x69, 0x73, 0x74, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12,
|
|
0x5f, 0x0a, 0x0a, 0x44, 0x69, 0x73, 0x63, 0x6f, 0x6e, 0x6e, 0x65, 0x63, 0x74, 0x12, 0x27, 0x2e,
|
|
0x62, 0x75, 0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65,
|
|
0x72, 0x2e, 0x76, 0x31, 0x2e, 0x44, 0x69, 0x73, 0x63, 0x6f, 0x6e, 0x6e, 0x65, 0x63, 0x74, 0x52,
|
|
0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x28, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x78, 0x2e,
|
|
0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e, 0x76, 0x31, 0x2e, 0x44, 0x69,
|
|
0x73, 0x63, 0x6f, 0x6e, 0x6e, 0x65, 0x63, 0x74, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65,
|
|
0x12, 0x4d, 0x0a, 0x04, 0x49, 0x6e, 0x66, 0x6f, 0x12, 0x21, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64,
|
|
0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e, 0x76, 0x31, 0x2e,
|
|
0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x22, 0x2e, 0x62, 0x75,
|
|
0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2e,
|
|
0x76, 0x31, 0x2e, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12,
|
|
0x68, 0x0a, 0x0d, 0x4c, 0x69, 0x73, 0x74, 0x50, 0x72, 0x6f, 0x63, 0x65, 0x73, 0x73, 0x65, 0x73,
|
|
0x12, 0x2a, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f,
|
|
0x6c, 0x6c, 0x65, 0x72, 0x2e, 0x76, 0x31, 0x2e, 0x4c, 0x69, 0x73, 0x74, 0x50, 0x72, 0x6f, 0x63,
|
|
0x65, 0x73, 0x73, 0x65, 0x73, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x2b, 0x2e, 0x62,
|
|
0x75, 0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72,
|
|
0x2e, 0x76, 0x31, 0x2e, 0x4c, 0x69, 0x73, 0x74, 0x50, 0x72, 0x6f, 0x63, 0x65, 0x73, 0x73, 0x65,
|
|
0x73, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x74, 0x0a, 0x11, 0x44, 0x69, 0x73,
|
|
0x63, 0x6f, 0x6e, 0x6e, 0x65, 0x63, 0x74, 0x50, 0x72, 0x6f, 0x63, 0x65, 0x73, 0x73, 0x12, 0x2e,
|
|
0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c,
|
|
0x65, 0x72, 0x2e, 0x76, 0x31, 0x2e, 0x44, 0x69, 0x73, 0x63, 0x6f, 0x6e, 0x6e, 0x65, 0x63, 0x74,
|
|
0x50, 0x72, 0x6f, 0x63, 0x65, 0x73, 0x73, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x2f,
|
|
0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x78, 0x2e, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x6c,
|
|
0x65, 0x72, 0x2e, 0x76, 0x31, 0x2e, 0x44, 0x69, 0x73, 0x63, 0x6f, 0x6e, 0x6e, 0x65, 0x63, 0x74,
|
|
0x50, 0x72, 0x6f, 0x63, 0x65, 0x73, 0x73, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x42,
|
|
0x28, 0x5a, 0x26, 0x67, 0x69, 0x74, 0x68, 0x75, 0x62, 0x2e, 0x63, 0x6f, 0x6d, 0x2f, 0x64, 0x6f,
|
|
0x63, 0x6b, 0x65, 0x72, 0x2f, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x78, 0x2f, 0x63, 0x6f, 0x6e, 0x74,
|
|
0x72, 0x6f, 0x6c, 0x6c, 0x65, 0x72, 0x2f, 0x70, 0x62, 0x62, 0x06, 0x70, 0x72, 0x6f, 0x74, 0x6f,
|
|
0x33,
|
|
}
|
|
|
|
var (
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_rawDescOnce sync.Once
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_rawDescData = file_github_com_docker_buildx_controller_pb_controller_proto_rawDesc
|
|
)
|
|
|
|
func file_github_com_docker_buildx_controller_pb_controller_proto_rawDescGZIP() []byte {
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_rawDescOnce.Do(func() {
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_rawDescData = protoimpl.X.CompressGZIP(file_github_com_docker_buildx_controller_pb_controller_proto_rawDescData)
|
|
})
|
|
return file_github_com_docker_buildx_controller_pb_controller_proto_rawDescData
|
|
}
|
|
|
|
var file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes = make([]protoimpl.MessageInfo, 44)
|
|
var file_github_com_docker_buildx_controller_pb_controller_proto_goTypes = []interface{}{
|
|
(*ListProcessesRequest)(nil), // 0: buildx.controller.v1.ListProcessesRequest
|
|
(*ListProcessesResponse)(nil), // 1: buildx.controller.v1.ListProcessesResponse
|
|
(*ProcessInfo)(nil), // 2: buildx.controller.v1.ProcessInfo
|
|
(*DisconnectProcessRequest)(nil), // 3: buildx.controller.v1.DisconnectProcessRequest
|
|
(*DisconnectProcessResponse)(nil), // 4: buildx.controller.v1.DisconnectProcessResponse
|
|
(*BuildRequest)(nil), // 5: buildx.controller.v1.BuildRequest
|
|
(*BuildOptions)(nil), // 6: buildx.controller.v1.BuildOptions
|
|
(*ExportEntry)(nil), // 7: buildx.controller.v1.ExportEntry
|
|
(*CacheOptionsEntry)(nil), // 8: buildx.controller.v1.CacheOptionsEntry
|
|
(*Attest)(nil), // 9: buildx.controller.v1.Attest
|
|
(*SSH)(nil), // 10: buildx.controller.v1.SSH
|
|
(*Secret)(nil), // 11: buildx.controller.v1.Secret
|
|
(*CallFunc)(nil), // 12: buildx.controller.v1.CallFunc
|
|
(*InspectRequest)(nil), // 13: buildx.controller.v1.InspectRequest
|
|
(*InspectResponse)(nil), // 14: buildx.controller.v1.InspectResponse
|
|
(*UlimitOpt)(nil), // 15: buildx.controller.v1.UlimitOpt
|
|
(*Ulimit)(nil), // 16: buildx.controller.v1.Ulimit
|
|
(*BuildResponse)(nil), // 17: buildx.controller.v1.BuildResponse
|
|
(*DisconnectRequest)(nil), // 18: buildx.controller.v1.DisconnectRequest
|
|
(*DisconnectResponse)(nil), // 19: buildx.controller.v1.DisconnectResponse
|
|
(*ListRequest)(nil), // 20: buildx.controller.v1.ListRequest
|
|
(*ListResponse)(nil), // 21: buildx.controller.v1.ListResponse
|
|
(*InputMessage)(nil), // 22: buildx.controller.v1.InputMessage
|
|
(*InputInitMessage)(nil), // 23: buildx.controller.v1.InputInitMessage
|
|
(*DataMessage)(nil), // 24: buildx.controller.v1.DataMessage
|
|
(*InputResponse)(nil), // 25: buildx.controller.v1.InputResponse
|
|
(*Message)(nil), // 26: buildx.controller.v1.Message
|
|
(*InitMessage)(nil), // 27: buildx.controller.v1.InitMessage
|
|
(*InvokeConfig)(nil), // 28: buildx.controller.v1.InvokeConfig
|
|
(*FdMessage)(nil), // 29: buildx.controller.v1.FdMessage
|
|
(*ResizeMessage)(nil), // 30: buildx.controller.v1.ResizeMessage
|
|
(*SignalMessage)(nil), // 31: buildx.controller.v1.SignalMessage
|
|
(*StatusRequest)(nil), // 32: buildx.controller.v1.StatusRequest
|
|
(*StatusResponse)(nil), // 33: buildx.controller.v1.StatusResponse
|
|
(*InfoRequest)(nil), // 34: buildx.controller.v1.InfoRequest
|
|
(*InfoResponse)(nil), // 35: buildx.controller.v1.InfoResponse
|
|
(*BuildxVersion)(nil), // 36: buildx.controller.v1.BuildxVersion
|
|
nil, // 37: buildx.controller.v1.BuildOptions.NamedContextsEntry
|
|
nil, // 38: buildx.controller.v1.BuildOptions.BuildArgsEntry
|
|
nil, // 39: buildx.controller.v1.BuildOptions.LabelsEntry
|
|
nil, // 40: buildx.controller.v1.ExportEntry.AttrsEntry
|
|
nil, // 41: buildx.controller.v1.CacheOptionsEntry.AttrsEntry
|
|
nil, // 42: buildx.controller.v1.UlimitOpt.ValuesEntry
|
|
nil, // 43: buildx.controller.v1.BuildResponse.ExporterResponseEntry
|
|
(*pb.Policy)(nil), // 44: moby.buildkit.v1.sourcepolicy.Policy
|
|
(*control.Vertex)(nil), // 45: moby.buildkit.v1.Vertex
|
|
(*control.VertexStatus)(nil), // 46: moby.buildkit.v1.VertexStatus
|
|
(*control.VertexLog)(nil), // 47: moby.buildkit.v1.VertexLog
|
|
(*control.VertexWarning)(nil), // 48: moby.buildkit.v1.VertexWarning
|
|
}
|
|
var file_github_com_docker_buildx_controller_pb_controller_proto_depIdxs = []int32{
|
|
2, // 0: buildx.controller.v1.ListProcessesResponse.Infos:type_name -> buildx.controller.v1.ProcessInfo
|
|
28, // 1: buildx.controller.v1.ProcessInfo.InvokeConfig:type_name -> buildx.controller.v1.InvokeConfig
|
|
6, // 2: buildx.controller.v1.BuildRequest.Options:type_name -> buildx.controller.v1.BuildOptions
|
|
12, // 3: buildx.controller.v1.BuildOptions.CallFunc:type_name -> buildx.controller.v1.CallFunc
|
|
37, // 4: buildx.controller.v1.BuildOptions.NamedContexts:type_name -> buildx.controller.v1.BuildOptions.NamedContextsEntry
|
|
9, // 5: buildx.controller.v1.BuildOptions.Attests:type_name -> buildx.controller.v1.Attest
|
|
38, // 6: buildx.controller.v1.BuildOptions.BuildArgs:type_name -> buildx.controller.v1.BuildOptions.BuildArgsEntry
|
|
8, // 7: buildx.controller.v1.BuildOptions.CacheFrom:type_name -> buildx.controller.v1.CacheOptionsEntry
|
|
8, // 8: buildx.controller.v1.BuildOptions.CacheTo:type_name -> buildx.controller.v1.CacheOptionsEntry
|
|
7, // 9: buildx.controller.v1.BuildOptions.Exports:type_name -> buildx.controller.v1.ExportEntry
|
|
39, // 10: buildx.controller.v1.BuildOptions.Labels:type_name -> buildx.controller.v1.BuildOptions.LabelsEntry
|
|
11, // 11: buildx.controller.v1.BuildOptions.Secrets:type_name -> buildx.controller.v1.Secret
|
|
10, // 12: buildx.controller.v1.BuildOptions.SSH:type_name -> buildx.controller.v1.SSH
|
|
15, // 13: buildx.controller.v1.BuildOptions.Ulimits:type_name -> buildx.controller.v1.UlimitOpt
|
|
44, // 14: buildx.controller.v1.BuildOptions.SourcePolicy:type_name -> moby.buildkit.v1.sourcepolicy.Policy
|
|
40, // 15: buildx.controller.v1.ExportEntry.Attrs:type_name -> buildx.controller.v1.ExportEntry.AttrsEntry
|
|
41, // 16: buildx.controller.v1.CacheOptionsEntry.Attrs:type_name -> buildx.controller.v1.CacheOptionsEntry.AttrsEntry
|
|
6, // 17: buildx.controller.v1.InspectResponse.Options:type_name -> buildx.controller.v1.BuildOptions
|
|
42, // 18: buildx.controller.v1.UlimitOpt.values:type_name -> buildx.controller.v1.UlimitOpt.ValuesEntry
|
|
43, // 19: buildx.controller.v1.BuildResponse.ExporterResponse:type_name -> buildx.controller.v1.BuildResponse.ExporterResponseEntry
|
|
23, // 20: buildx.controller.v1.InputMessage.Init:type_name -> buildx.controller.v1.InputInitMessage
|
|
24, // 21: buildx.controller.v1.InputMessage.Data:type_name -> buildx.controller.v1.DataMessage
|
|
27, // 22: buildx.controller.v1.Message.Init:type_name -> buildx.controller.v1.InitMessage
|
|
29, // 23: buildx.controller.v1.Message.File:type_name -> buildx.controller.v1.FdMessage
|
|
30, // 24: buildx.controller.v1.Message.Resize:type_name -> buildx.controller.v1.ResizeMessage
|
|
31, // 25: buildx.controller.v1.Message.Signal:type_name -> buildx.controller.v1.SignalMessage
|
|
28, // 26: buildx.controller.v1.InitMessage.InvokeConfig:type_name -> buildx.controller.v1.InvokeConfig
|
|
45, // 27: buildx.controller.v1.StatusResponse.vertexes:type_name -> moby.buildkit.v1.Vertex
|
|
46, // 28: buildx.controller.v1.StatusResponse.statuses:type_name -> moby.buildkit.v1.VertexStatus
|
|
47, // 29: buildx.controller.v1.StatusResponse.logs:type_name -> moby.buildkit.v1.VertexLog
|
|
48, // 30: buildx.controller.v1.StatusResponse.warnings:type_name -> moby.buildkit.v1.VertexWarning
|
|
36, // 31: buildx.controller.v1.InfoResponse.buildxVersion:type_name -> buildx.controller.v1.BuildxVersion
|
|
16, // 32: buildx.controller.v1.UlimitOpt.ValuesEntry.value:type_name -> buildx.controller.v1.Ulimit
|
|
5, // 33: buildx.controller.v1.Controller.Build:input_type -> buildx.controller.v1.BuildRequest
|
|
13, // 34: buildx.controller.v1.Controller.Inspect:input_type -> buildx.controller.v1.InspectRequest
|
|
32, // 35: buildx.controller.v1.Controller.Status:input_type -> buildx.controller.v1.StatusRequest
|
|
22, // 36: buildx.controller.v1.Controller.Input:input_type -> buildx.controller.v1.InputMessage
|
|
26, // 37: buildx.controller.v1.Controller.Invoke:input_type -> buildx.controller.v1.Message
|
|
20, // 38: buildx.controller.v1.Controller.List:input_type -> buildx.controller.v1.ListRequest
|
|
18, // 39: buildx.controller.v1.Controller.Disconnect:input_type -> buildx.controller.v1.DisconnectRequest
|
|
34, // 40: buildx.controller.v1.Controller.Info:input_type -> buildx.controller.v1.InfoRequest
|
|
0, // 41: buildx.controller.v1.Controller.ListProcesses:input_type -> buildx.controller.v1.ListProcessesRequest
|
|
3, // 42: buildx.controller.v1.Controller.DisconnectProcess:input_type -> buildx.controller.v1.DisconnectProcessRequest
|
|
17, // 43: buildx.controller.v1.Controller.Build:output_type -> buildx.controller.v1.BuildResponse
|
|
14, // 44: buildx.controller.v1.Controller.Inspect:output_type -> buildx.controller.v1.InspectResponse
|
|
33, // 45: buildx.controller.v1.Controller.Status:output_type -> buildx.controller.v1.StatusResponse
|
|
25, // 46: buildx.controller.v1.Controller.Input:output_type -> buildx.controller.v1.InputResponse
|
|
26, // 47: buildx.controller.v1.Controller.Invoke:output_type -> buildx.controller.v1.Message
|
|
21, // 48: buildx.controller.v1.Controller.List:output_type -> buildx.controller.v1.ListResponse
|
|
19, // 49: buildx.controller.v1.Controller.Disconnect:output_type -> buildx.controller.v1.DisconnectResponse
|
|
35, // 50: buildx.controller.v1.Controller.Info:output_type -> buildx.controller.v1.InfoResponse
|
|
1, // 51: buildx.controller.v1.Controller.ListProcesses:output_type -> buildx.controller.v1.ListProcessesResponse
|
|
4, // 52: buildx.controller.v1.Controller.DisconnectProcess:output_type -> buildx.controller.v1.DisconnectProcessResponse
|
|
43, // [43:53] is the sub-list for method output_type
|
|
33, // [33:43] is the sub-list for method input_type
|
|
33, // [33:33] is the sub-list for extension type_name
|
|
33, // [33:33] is the sub-list for extension extendee
|
|
0, // [0:33] is the sub-list for field type_name
|
|
}
|
|
|
|
func init() { file_github_com_docker_buildx_controller_pb_controller_proto_init() }
|
|
func file_github_com_docker_buildx_controller_pb_controller_proto_init() {
|
|
if File_github_com_docker_buildx_controller_pb_controller_proto != nil {
|
|
return
|
|
}
|
|
if !protoimpl.UnsafeEnabled {
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[0].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*ListProcessesRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[1].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*ListProcessesResponse); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[2].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*ProcessInfo); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[3].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*DisconnectProcessRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[4].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*DisconnectProcessResponse); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[5].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*BuildRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[6].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*BuildOptions); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[7].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*ExportEntry); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[8].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*CacheOptionsEntry); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[9].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*Attest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[10].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*SSH); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[11].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*Secret); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[12].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*CallFunc); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[13].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*InspectRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[14].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*InspectResponse); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[15].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*UlimitOpt); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[16].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*Ulimit); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[17].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*BuildResponse); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[18].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*DisconnectRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[19].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*DisconnectResponse); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[20].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*ListRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[21].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*ListResponse); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[22].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*InputMessage); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[23].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*InputInitMessage); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[24].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*DataMessage); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[25].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*InputResponse); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[26].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*Message); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[27].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*InitMessage); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[28].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*InvokeConfig); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[29].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*FdMessage); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[30].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*ResizeMessage); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[31].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*SignalMessage); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[32].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*StatusRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[33].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*StatusResponse); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[34].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*InfoRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[35].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*InfoResponse); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[36].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*BuildxVersion); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[22].OneofWrappers = []interface{}{
|
|
(*InputMessage_Init)(nil),
|
|
(*InputMessage_Data)(nil),
|
|
}
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes[26].OneofWrappers = []interface{}{
|
|
(*Message_Init)(nil),
|
|
(*Message_File)(nil),
|
|
(*Message_Resize)(nil),
|
|
(*Message_Signal)(nil),
|
|
}
|
|
type x struct{}
|
|
out := protoimpl.TypeBuilder{
|
|
File: protoimpl.DescBuilder{
|
|
GoPackagePath: reflect.TypeOf(x{}).PkgPath(),
|
|
RawDescriptor: file_github_com_docker_buildx_controller_pb_controller_proto_rawDesc,
|
|
NumEnums: 0,
|
|
NumMessages: 44,
|
|
NumExtensions: 0,
|
|
NumServices: 1,
|
|
},
|
|
GoTypes: file_github_com_docker_buildx_controller_pb_controller_proto_goTypes,
|
|
DependencyIndexes: file_github_com_docker_buildx_controller_pb_controller_proto_depIdxs,
|
|
MessageInfos: file_github_com_docker_buildx_controller_pb_controller_proto_msgTypes,
|
|
}.Build()
|
|
File_github_com_docker_buildx_controller_pb_controller_proto = out.File
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_rawDesc = nil
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_goTypes = nil
|
|
file_github_com_docker_buildx_controller_pb_controller_proto_depIdxs = nil
|
|
}
|