mirror of
https://gitea.com/Lydanne/buildx.git
synced 2025-07-07 03:57:42 +08:00
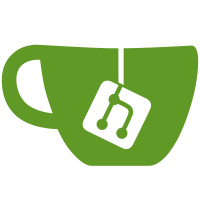
Removes gogo/protobuf from buildx and updates to a version of moby/buildkit where gogo is removed. This also changes how the proto files are generated. This is because newer versions of protobuf are more strict about name conflicts. If two files have the same name (even if they are relative paths) and are used in different protoc commands, they'll conflict in the registry. Since protobuf file generation doesn't work very well with `paths=source_relative`, this removes the `go:generate` expression and just relies on the dockerfile to perform the generation. Signed-off-by: Jonathan A. Sternberg <jonathan.sternberg@docker.com>
3026 lines
117 KiB
Go
3026 lines
117 KiB
Go
// Code generated by protoc-gen-go. DO NOT EDIT.
|
|
// versions:
|
|
// protoc-gen-go v1.34.1
|
|
// protoc v3.11.4
|
|
// source: control.proto
|
|
|
|
package moby_buildkit_v1
|
|
|
|
import (
|
|
timestamp "github.com/golang/protobuf/ptypes/timestamp"
|
|
types "github.com/moby/buildkit/api/types"
|
|
pb "github.com/moby/buildkit/solver/pb"
|
|
pb1 "github.com/moby/buildkit/sourcepolicy/pb"
|
|
status "google.golang.org/genproto/googleapis/rpc/status"
|
|
protoreflect "google.golang.org/protobuf/reflect/protoreflect"
|
|
protoimpl "google.golang.org/protobuf/runtime/protoimpl"
|
|
reflect "reflect"
|
|
sync "sync"
|
|
)
|
|
|
|
const (
|
|
// Verify that this generated code is sufficiently up-to-date.
|
|
_ = protoimpl.EnforceVersion(20 - protoimpl.MinVersion)
|
|
// Verify that runtime/protoimpl is sufficiently up-to-date.
|
|
_ = protoimpl.EnforceVersion(protoimpl.MaxVersion - 20)
|
|
)
|
|
|
|
type BuildHistoryEventType int32
|
|
|
|
const (
|
|
BuildHistoryEventType_STARTED BuildHistoryEventType = 0
|
|
BuildHistoryEventType_COMPLETE BuildHistoryEventType = 1
|
|
BuildHistoryEventType_DELETED BuildHistoryEventType = 2
|
|
)
|
|
|
|
// Enum value maps for BuildHistoryEventType.
|
|
var (
|
|
BuildHistoryEventType_name = map[int32]string{
|
|
0: "STARTED",
|
|
1: "COMPLETE",
|
|
2: "DELETED",
|
|
}
|
|
BuildHistoryEventType_value = map[string]int32{
|
|
"STARTED": 0,
|
|
"COMPLETE": 1,
|
|
"DELETED": 2,
|
|
}
|
|
)
|
|
|
|
func (x BuildHistoryEventType) Enum() *BuildHistoryEventType {
|
|
p := new(BuildHistoryEventType)
|
|
*p = x
|
|
return p
|
|
}
|
|
|
|
func (x BuildHistoryEventType) String() string {
|
|
return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x))
|
|
}
|
|
|
|
func (BuildHistoryEventType) Descriptor() protoreflect.EnumDescriptor {
|
|
return file_control_proto_enumTypes[0].Descriptor()
|
|
}
|
|
|
|
func (BuildHistoryEventType) Type() protoreflect.EnumType {
|
|
return &file_control_proto_enumTypes[0]
|
|
}
|
|
|
|
func (x BuildHistoryEventType) Number() protoreflect.EnumNumber {
|
|
return protoreflect.EnumNumber(x)
|
|
}
|
|
|
|
// Deprecated: Use BuildHistoryEventType.Descriptor instead.
|
|
func (BuildHistoryEventType) EnumDescriptor() ([]byte, []int) {
|
|
return file_control_proto_rawDescGZIP(), []int{0}
|
|
}
|
|
|
|
type PruneRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Filter []string `protobuf:"bytes,1,rep,name=filter,proto3" json:"filter,omitempty"`
|
|
All bool `protobuf:"varint,2,opt,name=all,proto3" json:"all,omitempty"`
|
|
KeepDuration int64 `protobuf:"varint,3,opt,name=keepDuration,proto3" json:"keepDuration,omitempty"`
|
|
MinStorage int64 `protobuf:"varint,5,opt,name=minStorage,proto3" json:"minStorage,omitempty"`
|
|
MaxStorage int64 `protobuf:"varint,4,opt,name=maxStorage,proto3" json:"maxStorage,omitempty"`
|
|
Free int64 `protobuf:"varint,6,opt,name=free,proto3" json:"free,omitempty"`
|
|
}
|
|
|
|
func (x *PruneRequest) Reset() {
|
|
*x = PruneRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_control_proto_msgTypes[0]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *PruneRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*PruneRequest) ProtoMessage() {}
|
|
|
|
func (x *PruneRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_control_proto_msgTypes[0]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use PruneRequest.ProtoReflect.Descriptor instead.
|
|
func (*PruneRequest) Descriptor() ([]byte, []int) {
|
|
return file_control_proto_rawDescGZIP(), []int{0}
|
|
}
|
|
|
|
func (x *PruneRequest) GetFilter() []string {
|
|
if x != nil {
|
|
return x.Filter
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *PruneRequest) GetAll() bool {
|
|
if x != nil {
|
|
return x.All
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *PruneRequest) GetKeepDuration() int64 {
|
|
if x != nil {
|
|
return x.KeepDuration
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *PruneRequest) GetMinStorage() int64 {
|
|
if x != nil {
|
|
return x.MinStorage
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *PruneRequest) GetMaxStorage() int64 {
|
|
if x != nil {
|
|
return x.MaxStorage
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *PruneRequest) GetFree() int64 {
|
|
if x != nil {
|
|
return x.Free
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type DiskUsageRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Filter []string `protobuf:"bytes,1,rep,name=filter,proto3" json:"filter,omitempty"`
|
|
}
|
|
|
|
func (x *DiskUsageRequest) Reset() {
|
|
*x = DiskUsageRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_control_proto_msgTypes[1]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *DiskUsageRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*DiskUsageRequest) ProtoMessage() {}
|
|
|
|
func (x *DiskUsageRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_control_proto_msgTypes[1]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use DiskUsageRequest.ProtoReflect.Descriptor instead.
|
|
func (*DiskUsageRequest) Descriptor() ([]byte, []int) {
|
|
return file_control_proto_rawDescGZIP(), []int{1}
|
|
}
|
|
|
|
func (x *DiskUsageRequest) GetFilter() []string {
|
|
if x != nil {
|
|
return x.Filter
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type DiskUsageResponse struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Record []*UsageRecord `protobuf:"bytes,1,rep,name=record,proto3" json:"record,omitempty"`
|
|
}
|
|
|
|
func (x *DiskUsageResponse) Reset() {
|
|
*x = DiskUsageResponse{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_control_proto_msgTypes[2]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *DiskUsageResponse) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*DiskUsageResponse) ProtoMessage() {}
|
|
|
|
func (x *DiskUsageResponse) ProtoReflect() protoreflect.Message {
|
|
mi := &file_control_proto_msgTypes[2]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use DiskUsageResponse.ProtoReflect.Descriptor instead.
|
|
func (*DiskUsageResponse) Descriptor() ([]byte, []int) {
|
|
return file_control_proto_rawDescGZIP(), []int{2}
|
|
}
|
|
|
|
func (x *DiskUsageResponse) GetRecord() []*UsageRecord {
|
|
if x != nil {
|
|
return x.Record
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type UsageRecord struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
ID string `protobuf:"bytes,1,opt,name=ID,proto3" json:"ID,omitempty"`
|
|
Mutable bool `protobuf:"varint,2,opt,name=Mutable,proto3" json:"Mutable,omitempty"`
|
|
InUse bool `protobuf:"varint,3,opt,name=InUse,proto3" json:"InUse,omitempty"`
|
|
Size int64 `protobuf:"varint,4,opt,name=Size,proto3" json:"Size,omitempty"`
|
|
// Deprecated: Marked as deprecated in control.proto.
|
|
Parent string `protobuf:"bytes,5,opt,name=Parent,proto3" json:"Parent,omitempty"`
|
|
CreatedAt *timestamp.Timestamp `protobuf:"bytes,6,opt,name=CreatedAt,proto3" json:"CreatedAt,omitempty"`
|
|
LastUsedAt *timestamp.Timestamp `protobuf:"bytes,7,opt,name=LastUsedAt,proto3" json:"LastUsedAt,omitempty"`
|
|
UsageCount int64 `protobuf:"varint,8,opt,name=UsageCount,proto3" json:"UsageCount,omitempty"`
|
|
Description string `protobuf:"bytes,9,opt,name=Description,proto3" json:"Description,omitempty"`
|
|
RecordType string `protobuf:"bytes,10,opt,name=RecordType,proto3" json:"RecordType,omitempty"`
|
|
Shared bool `protobuf:"varint,11,opt,name=Shared,proto3" json:"Shared,omitempty"`
|
|
Parents []string `protobuf:"bytes,12,rep,name=Parents,proto3" json:"Parents,omitempty"`
|
|
}
|
|
|
|
func (x *UsageRecord) Reset() {
|
|
*x = UsageRecord{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_control_proto_msgTypes[3]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *UsageRecord) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*UsageRecord) ProtoMessage() {}
|
|
|
|
func (x *UsageRecord) ProtoReflect() protoreflect.Message {
|
|
mi := &file_control_proto_msgTypes[3]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use UsageRecord.ProtoReflect.Descriptor instead.
|
|
func (*UsageRecord) Descriptor() ([]byte, []int) {
|
|
return file_control_proto_rawDescGZIP(), []int{3}
|
|
}
|
|
|
|
func (x *UsageRecord) GetID() string {
|
|
if x != nil {
|
|
return x.ID
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UsageRecord) GetMutable() bool {
|
|
if x != nil {
|
|
return x.Mutable
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *UsageRecord) GetInUse() bool {
|
|
if x != nil {
|
|
return x.InUse
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *UsageRecord) GetSize() int64 {
|
|
if x != nil {
|
|
return x.Size
|
|
}
|
|
return 0
|
|
}
|
|
|
|
// Deprecated: Marked as deprecated in control.proto.
|
|
func (x *UsageRecord) GetParent() string {
|
|
if x != nil {
|
|
return x.Parent
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UsageRecord) GetCreatedAt() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.CreatedAt
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *UsageRecord) GetLastUsedAt() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.LastUsedAt
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *UsageRecord) GetUsageCount() int64 {
|
|
if x != nil {
|
|
return x.UsageCount
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *UsageRecord) GetDescription() string {
|
|
if x != nil {
|
|
return x.Description
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UsageRecord) GetRecordType() string {
|
|
if x != nil {
|
|
return x.RecordType
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UsageRecord) GetShared() bool {
|
|
if x != nil {
|
|
return x.Shared
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *UsageRecord) GetParents() []string {
|
|
if x != nil {
|
|
return x.Parents
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type SolveRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Ref string `protobuf:"bytes,1,opt,name=Ref,proto3" json:"Ref,omitempty"`
|
|
Definition *pb.Definition `protobuf:"bytes,2,opt,name=Definition,proto3" json:"Definition,omitempty"`
|
|
// ExporterDeprecated and ExporterAttrsDeprecated are deprecated in favor
|
|
// of the new Exporters. If these fields are set, then they will be
|
|
// appended to the Exporters field if Exporters was not explicitly set.
|
|
ExporterDeprecated string `protobuf:"bytes,3,opt,name=ExporterDeprecated,proto3" json:"ExporterDeprecated,omitempty"`
|
|
ExporterAttrsDeprecated map[string]string `protobuf:"bytes,4,rep,name=ExporterAttrsDeprecated,proto3" json:"ExporterAttrsDeprecated,omitempty" protobuf_key:"bytes,1,opt,name=key,proto3" protobuf_val:"bytes,2,opt,name=value,proto3"`
|
|
Session string `protobuf:"bytes,5,opt,name=Session,proto3" json:"Session,omitempty"`
|
|
Frontend string `protobuf:"bytes,6,opt,name=Frontend,proto3" json:"Frontend,omitempty"`
|
|
FrontendAttrs map[string]string `protobuf:"bytes,7,rep,name=FrontendAttrs,proto3" json:"FrontendAttrs,omitempty" protobuf_key:"bytes,1,opt,name=key,proto3" protobuf_val:"bytes,2,opt,name=value,proto3"`
|
|
Cache *CacheOptions `protobuf:"bytes,8,opt,name=Cache,proto3" json:"Cache,omitempty"`
|
|
Entitlements []string `protobuf:"bytes,9,rep,name=Entitlements,proto3" json:"Entitlements,omitempty"`
|
|
FrontendInputs map[string]*pb.Definition `protobuf:"bytes,10,rep,name=FrontendInputs,proto3" json:"FrontendInputs,omitempty" protobuf_key:"bytes,1,opt,name=key,proto3" protobuf_val:"bytes,2,opt,name=value,proto3"`
|
|
Internal bool `protobuf:"varint,11,opt,name=Internal,proto3" json:"Internal,omitempty"` // Internal builds are not recorded in build history
|
|
SourcePolicy *pb1.Policy `protobuf:"bytes,12,opt,name=SourcePolicy,proto3" json:"SourcePolicy,omitempty"`
|
|
Exporters []*Exporter `protobuf:"bytes,13,rep,name=Exporters,proto3" json:"Exporters,omitempty"`
|
|
}
|
|
|
|
func (x *SolveRequest) Reset() {
|
|
*x = SolveRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_control_proto_msgTypes[4]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *SolveRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*SolveRequest) ProtoMessage() {}
|
|
|
|
func (x *SolveRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_control_proto_msgTypes[4]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use SolveRequest.ProtoReflect.Descriptor instead.
|
|
func (*SolveRequest) Descriptor() ([]byte, []int) {
|
|
return file_control_proto_rawDescGZIP(), []int{4}
|
|
}
|
|
|
|
func (x *SolveRequest) GetRef() string {
|
|
if x != nil {
|
|
return x.Ref
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *SolveRequest) GetDefinition() *pb.Definition {
|
|
if x != nil {
|
|
return x.Definition
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *SolveRequest) GetExporterDeprecated() string {
|
|
if x != nil {
|
|
return x.ExporterDeprecated
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *SolveRequest) GetExporterAttrsDeprecated() map[string]string {
|
|
if x != nil {
|
|
return x.ExporterAttrsDeprecated
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *SolveRequest) GetSession() string {
|
|
if x != nil {
|
|
return x.Session
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *SolveRequest) GetFrontend() string {
|
|
if x != nil {
|
|
return x.Frontend
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *SolveRequest) GetFrontendAttrs() map[string]string {
|
|
if x != nil {
|
|
return x.FrontendAttrs
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *SolveRequest) GetCache() *CacheOptions {
|
|
if x != nil {
|
|
return x.Cache
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *SolveRequest) GetEntitlements() []string {
|
|
if x != nil {
|
|
return x.Entitlements
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *SolveRequest) GetFrontendInputs() map[string]*pb.Definition {
|
|
if x != nil {
|
|
return x.FrontendInputs
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *SolveRequest) GetInternal() bool {
|
|
if x != nil {
|
|
return x.Internal
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *SolveRequest) GetSourcePolicy() *pb1.Policy {
|
|
if x != nil {
|
|
return x.SourcePolicy
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *SolveRequest) GetExporters() []*Exporter {
|
|
if x != nil {
|
|
return x.Exporters
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type CacheOptions struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
// ExportRefDeprecated is deprecated in favor or the new Exports since BuildKit v0.4.0.
|
|
// When ExportRefDeprecated is set, the solver appends
|
|
// {.Type = "registry", .Attrs = ExportAttrs.add("ref", ExportRef)}
|
|
// to Exports for compatibility. (planned to be removed)
|
|
ExportRefDeprecated string `protobuf:"bytes,1,opt,name=ExportRefDeprecated,proto3" json:"ExportRefDeprecated,omitempty"`
|
|
// ImportRefsDeprecated is deprecated in favor or the new Imports since BuildKit v0.4.0.
|
|
// When ImportRefsDeprecated is set, the solver appends
|
|
// {.Type = "registry", .Attrs = {"ref": importRef}}
|
|
// for each of the ImportRefs entry to Imports for compatibility. (planned to be removed)
|
|
ImportRefsDeprecated []string `protobuf:"bytes,2,rep,name=ImportRefsDeprecated,proto3" json:"ImportRefsDeprecated,omitempty"`
|
|
// ExportAttrsDeprecated is deprecated since BuildKit v0.4.0.
|
|
// See the description of ExportRefDeprecated.
|
|
ExportAttrsDeprecated map[string]string `protobuf:"bytes,3,rep,name=ExportAttrsDeprecated,proto3" json:"ExportAttrsDeprecated,omitempty" protobuf_key:"bytes,1,opt,name=key,proto3" protobuf_val:"bytes,2,opt,name=value,proto3"`
|
|
// Exports was introduced in BuildKit v0.4.0.
|
|
Exports []*CacheOptionsEntry `protobuf:"bytes,4,rep,name=Exports,proto3" json:"Exports,omitempty"`
|
|
// Imports was introduced in BuildKit v0.4.0.
|
|
Imports []*CacheOptionsEntry `protobuf:"bytes,5,rep,name=Imports,proto3" json:"Imports,omitempty"`
|
|
}
|
|
|
|
func (x *CacheOptions) Reset() {
|
|
*x = CacheOptions{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_control_proto_msgTypes[5]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *CacheOptions) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*CacheOptions) ProtoMessage() {}
|
|
|
|
func (x *CacheOptions) ProtoReflect() protoreflect.Message {
|
|
mi := &file_control_proto_msgTypes[5]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use CacheOptions.ProtoReflect.Descriptor instead.
|
|
func (*CacheOptions) Descriptor() ([]byte, []int) {
|
|
return file_control_proto_rawDescGZIP(), []int{5}
|
|
}
|
|
|
|
func (x *CacheOptions) GetExportRefDeprecated() string {
|
|
if x != nil {
|
|
return x.ExportRefDeprecated
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *CacheOptions) GetImportRefsDeprecated() []string {
|
|
if x != nil {
|
|
return x.ImportRefsDeprecated
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *CacheOptions) GetExportAttrsDeprecated() map[string]string {
|
|
if x != nil {
|
|
return x.ExportAttrsDeprecated
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *CacheOptions) GetExports() []*CacheOptionsEntry {
|
|
if x != nil {
|
|
return x.Exports
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *CacheOptions) GetImports() []*CacheOptionsEntry {
|
|
if x != nil {
|
|
return x.Imports
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type CacheOptionsEntry struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
// Type is like "registry" or "local"
|
|
Type string `protobuf:"bytes,1,opt,name=Type,proto3" json:"Type,omitempty"`
|
|
// Attrs are like mode=(min,max), ref=example.com:5000/foo/bar .
|
|
// See cache importer/exporter implementations' documentation.
|
|
Attrs map[string]string `protobuf:"bytes,2,rep,name=Attrs,proto3" json:"Attrs,omitempty" protobuf_key:"bytes,1,opt,name=key,proto3" protobuf_val:"bytes,2,opt,name=value,proto3"`
|
|
}
|
|
|
|
func (x *CacheOptionsEntry) Reset() {
|
|
*x = CacheOptionsEntry{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_control_proto_msgTypes[6]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *CacheOptionsEntry) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*CacheOptionsEntry) ProtoMessage() {}
|
|
|
|
func (x *CacheOptionsEntry) ProtoReflect() protoreflect.Message {
|
|
mi := &file_control_proto_msgTypes[6]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use CacheOptionsEntry.ProtoReflect.Descriptor instead.
|
|
func (*CacheOptionsEntry) Descriptor() ([]byte, []int) {
|
|
return file_control_proto_rawDescGZIP(), []int{6}
|
|
}
|
|
|
|
func (x *CacheOptionsEntry) GetType() string {
|
|
if x != nil {
|
|
return x.Type
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *CacheOptionsEntry) GetAttrs() map[string]string {
|
|
if x != nil {
|
|
return x.Attrs
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type SolveResponse struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
ExporterResponse map[string]string `protobuf:"bytes,1,rep,name=ExporterResponse,proto3" json:"ExporterResponse,omitempty" protobuf_key:"bytes,1,opt,name=key,proto3" protobuf_val:"bytes,2,opt,name=value,proto3"`
|
|
}
|
|
|
|
func (x *SolveResponse) Reset() {
|
|
*x = SolveResponse{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_control_proto_msgTypes[7]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *SolveResponse) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*SolveResponse) ProtoMessage() {}
|
|
|
|
func (x *SolveResponse) ProtoReflect() protoreflect.Message {
|
|
mi := &file_control_proto_msgTypes[7]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use SolveResponse.ProtoReflect.Descriptor instead.
|
|
func (*SolveResponse) Descriptor() ([]byte, []int) {
|
|
return file_control_proto_rawDescGZIP(), []int{7}
|
|
}
|
|
|
|
func (x *SolveResponse) GetExporterResponse() map[string]string {
|
|
if x != nil {
|
|
return x.ExporterResponse
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type StatusRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Ref string `protobuf:"bytes,1,opt,name=Ref,proto3" json:"Ref,omitempty"`
|
|
}
|
|
|
|
func (x *StatusRequest) Reset() {
|
|
*x = StatusRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_control_proto_msgTypes[8]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *StatusRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*StatusRequest) ProtoMessage() {}
|
|
|
|
func (x *StatusRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_control_proto_msgTypes[8]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use StatusRequest.ProtoReflect.Descriptor instead.
|
|
func (*StatusRequest) Descriptor() ([]byte, []int) {
|
|
return file_control_proto_rawDescGZIP(), []int{8}
|
|
}
|
|
|
|
func (x *StatusRequest) GetRef() string {
|
|
if x != nil {
|
|
return x.Ref
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type StatusResponse struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Vertexes []*Vertex `protobuf:"bytes,1,rep,name=vertexes,proto3" json:"vertexes,omitempty"`
|
|
Statuses []*VertexStatus `protobuf:"bytes,2,rep,name=statuses,proto3" json:"statuses,omitempty"`
|
|
Logs []*VertexLog `protobuf:"bytes,3,rep,name=logs,proto3" json:"logs,omitempty"`
|
|
Warnings []*VertexWarning `protobuf:"bytes,4,rep,name=warnings,proto3" json:"warnings,omitempty"`
|
|
}
|
|
|
|
func (x *StatusResponse) Reset() {
|
|
*x = StatusResponse{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_control_proto_msgTypes[9]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *StatusResponse) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*StatusResponse) ProtoMessage() {}
|
|
|
|
func (x *StatusResponse) ProtoReflect() protoreflect.Message {
|
|
mi := &file_control_proto_msgTypes[9]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use StatusResponse.ProtoReflect.Descriptor instead.
|
|
func (*StatusResponse) Descriptor() ([]byte, []int) {
|
|
return file_control_proto_rawDescGZIP(), []int{9}
|
|
}
|
|
|
|
func (x *StatusResponse) GetVertexes() []*Vertex {
|
|
if x != nil {
|
|
return x.Vertexes
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *StatusResponse) GetStatuses() []*VertexStatus {
|
|
if x != nil {
|
|
return x.Statuses
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *StatusResponse) GetLogs() []*VertexLog {
|
|
if x != nil {
|
|
return x.Logs
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *StatusResponse) GetWarnings() []*VertexWarning {
|
|
if x != nil {
|
|
return x.Warnings
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type Vertex struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Digest string `protobuf:"bytes,1,opt,name=digest,proto3" json:"digest,omitempty"`
|
|
Inputs []string `protobuf:"bytes,2,rep,name=inputs,proto3" json:"inputs,omitempty"`
|
|
Name string `protobuf:"bytes,3,opt,name=name,proto3" json:"name,omitempty"`
|
|
Cached bool `protobuf:"varint,4,opt,name=cached,proto3" json:"cached,omitempty"`
|
|
Started *timestamp.Timestamp `protobuf:"bytes,5,opt,name=started,proto3" json:"started,omitempty"`
|
|
Completed *timestamp.Timestamp `protobuf:"bytes,6,opt,name=completed,proto3" json:"completed,omitempty"`
|
|
Error string `protobuf:"bytes,7,opt,name=error,proto3" json:"error,omitempty"` // typed errors?
|
|
ProgressGroup *pb.ProgressGroup `protobuf:"bytes,8,opt,name=progressGroup,proto3" json:"progressGroup,omitempty"`
|
|
}
|
|
|
|
func (x *Vertex) Reset() {
|
|
*x = Vertex{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_control_proto_msgTypes[10]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *Vertex) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*Vertex) ProtoMessage() {}
|
|
|
|
func (x *Vertex) ProtoReflect() protoreflect.Message {
|
|
mi := &file_control_proto_msgTypes[10]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use Vertex.ProtoReflect.Descriptor instead.
|
|
func (*Vertex) Descriptor() ([]byte, []int) {
|
|
return file_control_proto_rawDescGZIP(), []int{10}
|
|
}
|
|
|
|
func (x *Vertex) GetDigest() string {
|
|
if x != nil {
|
|
return x.Digest
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *Vertex) GetInputs() []string {
|
|
if x != nil {
|
|
return x.Inputs
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *Vertex) GetName() string {
|
|
if x != nil {
|
|
return x.Name
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *Vertex) GetCached() bool {
|
|
if x != nil {
|
|
return x.Cached
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *Vertex) GetStarted() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.Started
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *Vertex) GetCompleted() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.Completed
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *Vertex) GetError() string {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *Vertex) GetProgressGroup() *pb.ProgressGroup {
|
|
if x != nil {
|
|
return x.ProgressGroup
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type VertexStatus struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
ID string `protobuf:"bytes,1,opt,name=ID,proto3" json:"ID,omitempty"`
|
|
Vertex string `protobuf:"bytes,2,opt,name=vertex,proto3" json:"vertex,omitempty"`
|
|
Name string `protobuf:"bytes,3,opt,name=name,proto3" json:"name,omitempty"`
|
|
Current int64 `protobuf:"varint,4,opt,name=current,proto3" json:"current,omitempty"`
|
|
Total int64 `protobuf:"varint,5,opt,name=total,proto3" json:"total,omitempty"`
|
|
Timestamp *timestamp.Timestamp `protobuf:"bytes,6,opt,name=timestamp,proto3" json:"timestamp,omitempty"`
|
|
Started *timestamp.Timestamp `protobuf:"bytes,7,opt,name=started,proto3" json:"started,omitempty"`
|
|
Completed *timestamp.Timestamp `protobuf:"bytes,8,opt,name=completed,proto3" json:"completed,omitempty"`
|
|
}
|
|
|
|
func (x *VertexStatus) Reset() {
|
|
*x = VertexStatus{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_control_proto_msgTypes[11]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *VertexStatus) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*VertexStatus) ProtoMessage() {}
|
|
|
|
func (x *VertexStatus) ProtoReflect() protoreflect.Message {
|
|
mi := &file_control_proto_msgTypes[11]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use VertexStatus.ProtoReflect.Descriptor instead.
|
|
func (*VertexStatus) Descriptor() ([]byte, []int) {
|
|
return file_control_proto_rawDescGZIP(), []int{11}
|
|
}
|
|
|
|
func (x *VertexStatus) GetID() string {
|
|
if x != nil {
|
|
return x.ID
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *VertexStatus) GetVertex() string {
|
|
if x != nil {
|
|
return x.Vertex
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *VertexStatus) GetName() string {
|
|
if x != nil {
|
|
return x.Name
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *VertexStatus) GetCurrent() int64 {
|
|
if x != nil {
|
|
return x.Current
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *VertexStatus) GetTotal() int64 {
|
|
if x != nil {
|
|
return x.Total
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *VertexStatus) GetTimestamp() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.Timestamp
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *VertexStatus) GetStarted() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.Started
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *VertexStatus) GetCompleted() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.Completed
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type VertexLog struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Vertex string `protobuf:"bytes,1,opt,name=vertex,proto3" json:"vertex,omitempty"`
|
|
Timestamp *timestamp.Timestamp `protobuf:"bytes,2,opt,name=timestamp,proto3" json:"timestamp,omitempty"`
|
|
Stream int64 `protobuf:"varint,3,opt,name=stream,proto3" json:"stream,omitempty"`
|
|
Msg []byte `protobuf:"bytes,4,opt,name=msg,proto3" json:"msg,omitempty"`
|
|
}
|
|
|
|
func (x *VertexLog) Reset() {
|
|
*x = VertexLog{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_control_proto_msgTypes[12]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *VertexLog) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*VertexLog) ProtoMessage() {}
|
|
|
|
func (x *VertexLog) ProtoReflect() protoreflect.Message {
|
|
mi := &file_control_proto_msgTypes[12]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use VertexLog.ProtoReflect.Descriptor instead.
|
|
func (*VertexLog) Descriptor() ([]byte, []int) {
|
|
return file_control_proto_rawDescGZIP(), []int{12}
|
|
}
|
|
|
|
func (x *VertexLog) GetVertex() string {
|
|
if x != nil {
|
|
return x.Vertex
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *VertexLog) GetTimestamp() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.Timestamp
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *VertexLog) GetStream() int64 {
|
|
if x != nil {
|
|
return x.Stream
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *VertexLog) GetMsg() []byte {
|
|
if x != nil {
|
|
return x.Msg
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type VertexWarning struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Vertex string `protobuf:"bytes,1,opt,name=vertex,proto3" json:"vertex,omitempty"`
|
|
Level int64 `protobuf:"varint,2,opt,name=level,proto3" json:"level,omitempty"`
|
|
Short []byte `protobuf:"bytes,3,opt,name=short,proto3" json:"short,omitempty"`
|
|
Detail [][]byte `protobuf:"bytes,4,rep,name=detail,proto3" json:"detail,omitempty"`
|
|
Url string `protobuf:"bytes,5,opt,name=url,proto3" json:"url,omitempty"`
|
|
Info *pb.SourceInfo `protobuf:"bytes,6,opt,name=info,proto3" json:"info,omitempty"`
|
|
Ranges []*pb.Range `protobuf:"bytes,7,rep,name=ranges,proto3" json:"ranges,omitempty"`
|
|
}
|
|
|
|
func (x *VertexWarning) Reset() {
|
|
*x = VertexWarning{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_control_proto_msgTypes[13]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *VertexWarning) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*VertexWarning) ProtoMessage() {}
|
|
|
|
func (x *VertexWarning) ProtoReflect() protoreflect.Message {
|
|
mi := &file_control_proto_msgTypes[13]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use VertexWarning.ProtoReflect.Descriptor instead.
|
|
func (*VertexWarning) Descriptor() ([]byte, []int) {
|
|
return file_control_proto_rawDescGZIP(), []int{13}
|
|
}
|
|
|
|
func (x *VertexWarning) GetVertex() string {
|
|
if x != nil {
|
|
return x.Vertex
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *VertexWarning) GetLevel() int64 {
|
|
if x != nil {
|
|
return x.Level
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *VertexWarning) GetShort() []byte {
|
|
if x != nil {
|
|
return x.Short
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *VertexWarning) GetDetail() [][]byte {
|
|
if x != nil {
|
|
return x.Detail
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *VertexWarning) GetUrl() string {
|
|
if x != nil {
|
|
return x.Url
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *VertexWarning) GetInfo() *pb.SourceInfo {
|
|
if x != nil {
|
|
return x.Info
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *VertexWarning) GetRanges() []*pb.Range {
|
|
if x != nil {
|
|
return x.Ranges
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type BytesMessage struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Data []byte `protobuf:"bytes,1,opt,name=data,proto3" json:"data,omitempty"`
|
|
}
|
|
|
|
func (x *BytesMessage) Reset() {
|
|
*x = BytesMessage{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_control_proto_msgTypes[14]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *BytesMessage) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*BytesMessage) ProtoMessage() {}
|
|
|
|
func (x *BytesMessage) ProtoReflect() protoreflect.Message {
|
|
mi := &file_control_proto_msgTypes[14]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use BytesMessage.ProtoReflect.Descriptor instead.
|
|
func (*BytesMessage) Descriptor() ([]byte, []int) {
|
|
return file_control_proto_rawDescGZIP(), []int{14}
|
|
}
|
|
|
|
func (x *BytesMessage) GetData() []byte {
|
|
if x != nil {
|
|
return x.Data
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type ListWorkersRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Filter []string `protobuf:"bytes,1,rep,name=filter,proto3" json:"filter,omitempty"` // containerd style
|
|
}
|
|
|
|
func (x *ListWorkersRequest) Reset() {
|
|
*x = ListWorkersRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_control_proto_msgTypes[15]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *ListWorkersRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*ListWorkersRequest) ProtoMessage() {}
|
|
|
|
func (x *ListWorkersRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_control_proto_msgTypes[15]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use ListWorkersRequest.ProtoReflect.Descriptor instead.
|
|
func (*ListWorkersRequest) Descriptor() ([]byte, []int) {
|
|
return file_control_proto_rawDescGZIP(), []int{15}
|
|
}
|
|
|
|
func (x *ListWorkersRequest) GetFilter() []string {
|
|
if x != nil {
|
|
return x.Filter
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type ListWorkersResponse struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Record []*types.WorkerRecord `protobuf:"bytes,1,rep,name=record,proto3" json:"record,omitempty"`
|
|
}
|
|
|
|
func (x *ListWorkersResponse) Reset() {
|
|
*x = ListWorkersResponse{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_control_proto_msgTypes[16]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *ListWorkersResponse) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*ListWorkersResponse) ProtoMessage() {}
|
|
|
|
func (x *ListWorkersResponse) ProtoReflect() protoreflect.Message {
|
|
mi := &file_control_proto_msgTypes[16]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use ListWorkersResponse.ProtoReflect.Descriptor instead.
|
|
func (*ListWorkersResponse) Descriptor() ([]byte, []int) {
|
|
return file_control_proto_rawDescGZIP(), []int{16}
|
|
}
|
|
|
|
func (x *ListWorkersResponse) GetRecord() []*types.WorkerRecord {
|
|
if x != nil {
|
|
return x.Record
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type InfoRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
}
|
|
|
|
func (x *InfoRequest) Reset() {
|
|
*x = InfoRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_control_proto_msgTypes[17]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *InfoRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*InfoRequest) ProtoMessage() {}
|
|
|
|
func (x *InfoRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_control_proto_msgTypes[17]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use InfoRequest.ProtoReflect.Descriptor instead.
|
|
func (*InfoRequest) Descriptor() ([]byte, []int) {
|
|
return file_control_proto_rawDescGZIP(), []int{17}
|
|
}
|
|
|
|
type InfoResponse struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
BuildkitVersion *types.BuildkitVersion `protobuf:"bytes,1,opt,name=buildkitVersion,proto3" json:"buildkitVersion,omitempty"`
|
|
}
|
|
|
|
func (x *InfoResponse) Reset() {
|
|
*x = InfoResponse{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_control_proto_msgTypes[18]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *InfoResponse) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*InfoResponse) ProtoMessage() {}
|
|
|
|
func (x *InfoResponse) ProtoReflect() protoreflect.Message {
|
|
mi := &file_control_proto_msgTypes[18]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use InfoResponse.ProtoReflect.Descriptor instead.
|
|
func (*InfoResponse) Descriptor() ([]byte, []int) {
|
|
return file_control_proto_rawDescGZIP(), []int{18}
|
|
}
|
|
|
|
func (x *InfoResponse) GetBuildkitVersion() *types.BuildkitVersion {
|
|
if x != nil {
|
|
return x.BuildkitVersion
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type BuildHistoryRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
ActiveOnly bool `protobuf:"varint,1,opt,name=ActiveOnly,proto3" json:"ActiveOnly,omitempty"`
|
|
Ref string `protobuf:"bytes,2,opt,name=Ref,proto3" json:"Ref,omitempty"`
|
|
EarlyExit bool `protobuf:"varint,3,opt,name=EarlyExit,proto3" json:"EarlyExit,omitempty"`
|
|
}
|
|
|
|
func (x *BuildHistoryRequest) Reset() {
|
|
*x = BuildHistoryRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_control_proto_msgTypes[19]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *BuildHistoryRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*BuildHistoryRequest) ProtoMessage() {}
|
|
|
|
func (x *BuildHistoryRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_control_proto_msgTypes[19]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use BuildHistoryRequest.ProtoReflect.Descriptor instead.
|
|
func (*BuildHistoryRequest) Descriptor() ([]byte, []int) {
|
|
return file_control_proto_rawDescGZIP(), []int{19}
|
|
}
|
|
|
|
func (x *BuildHistoryRequest) GetActiveOnly() bool {
|
|
if x != nil {
|
|
return x.ActiveOnly
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *BuildHistoryRequest) GetRef() string {
|
|
if x != nil {
|
|
return x.Ref
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *BuildHistoryRequest) GetEarlyExit() bool {
|
|
if x != nil {
|
|
return x.EarlyExit
|
|
}
|
|
return false
|
|
}
|
|
|
|
type BuildHistoryEvent struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Type BuildHistoryEventType `protobuf:"varint,1,opt,name=type,proto3,enum=moby.buildkit.v1.BuildHistoryEventType" json:"type,omitempty"`
|
|
Record *BuildHistoryRecord `protobuf:"bytes,2,opt,name=record,proto3" json:"record,omitempty"`
|
|
}
|
|
|
|
func (x *BuildHistoryEvent) Reset() {
|
|
*x = BuildHistoryEvent{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_control_proto_msgTypes[20]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *BuildHistoryEvent) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*BuildHistoryEvent) ProtoMessage() {}
|
|
|
|
func (x *BuildHistoryEvent) ProtoReflect() protoreflect.Message {
|
|
mi := &file_control_proto_msgTypes[20]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use BuildHistoryEvent.ProtoReflect.Descriptor instead.
|
|
func (*BuildHistoryEvent) Descriptor() ([]byte, []int) {
|
|
return file_control_proto_rawDescGZIP(), []int{20}
|
|
}
|
|
|
|
func (x *BuildHistoryEvent) GetType() BuildHistoryEventType {
|
|
if x != nil {
|
|
return x.Type
|
|
}
|
|
return BuildHistoryEventType_STARTED
|
|
}
|
|
|
|
func (x *BuildHistoryEvent) GetRecord() *BuildHistoryRecord {
|
|
if x != nil {
|
|
return x.Record
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type BuildHistoryRecord struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Ref string `protobuf:"bytes,1,opt,name=Ref,proto3" json:"Ref,omitempty"`
|
|
Frontend string `protobuf:"bytes,2,opt,name=Frontend,proto3" json:"Frontend,omitempty"`
|
|
FrontendAttrs map[string]string `protobuf:"bytes,3,rep,name=FrontendAttrs,proto3" json:"FrontendAttrs,omitempty" protobuf_key:"bytes,1,opt,name=key,proto3" protobuf_val:"bytes,2,opt,name=value,proto3"`
|
|
Exporters []*Exporter `protobuf:"bytes,4,rep,name=Exporters,proto3" json:"Exporters,omitempty"`
|
|
Error *status.Status `protobuf:"bytes,5,opt,name=error,proto3" json:"error,omitempty"`
|
|
CreatedAt *timestamp.Timestamp `protobuf:"bytes,6,opt,name=CreatedAt,proto3" json:"CreatedAt,omitempty"`
|
|
CompletedAt *timestamp.Timestamp `protobuf:"bytes,7,opt,name=CompletedAt,proto3" json:"CompletedAt,omitempty"`
|
|
Logs *Descriptor `protobuf:"bytes,8,opt,name=logs,proto3" json:"logs,omitempty"`
|
|
ExporterResponse map[string]string `protobuf:"bytes,9,rep,name=ExporterResponse,proto3" json:"ExporterResponse,omitempty" protobuf_key:"bytes,1,opt,name=key,proto3" protobuf_val:"bytes,2,opt,name=value,proto3"`
|
|
Result *BuildResultInfo `protobuf:"bytes,10,opt,name=Result,proto3" json:"Result,omitempty"`
|
|
Results map[string]*BuildResultInfo `protobuf:"bytes,11,rep,name=Results,proto3" json:"Results,omitempty" protobuf_key:"bytes,1,opt,name=key,proto3" protobuf_val:"bytes,2,opt,name=value,proto3"`
|
|
Generation int32 `protobuf:"varint,12,opt,name=Generation,proto3" json:"Generation,omitempty"`
|
|
Trace *Descriptor `protobuf:"bytes,13,opt,name=trace,proto3" json:"trace,omitempty"`
|
|
Pinned bool `protobuf:"varint,14,opt,name=pinned,proto3" json:"pinned,omitempty"`
|
|
NumCachedSteps int32 `protobuf:"varint,15,opt,name=numCachedSteps,proto3" json:"numCachedSteps,omitempty"`
|
|
NumTotalSteps int32 `protobuf:"varint,16,opt,name=numTotalSteps,proto3" json:"numTotalSteps,omitempty"`
|
|
NumCompletedSteps int32 `protobuf:"varint,17,opt,name=numCompletedSteps,proto3" json:"numCompletedSteps,omitempty"`
|
|
ExternalError *Descriptor `protobuf:"bytes,18,opt,name=externalError,proto3" json:"externalError,omitempty"`
|
|
NumWarnings int32 `protobuf:"varint,19,opt,name=numWarnings,proto3" json:"numWarnings,omitempty"`
|
|
}
|
|
|
|
func (x *BuildHistoryRecord) Reset() {
|
|
*x = BuildHistoryRecord{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_control_proto_msgTypes[21]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *BuildHistoryRecord) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*BuildHistoryRecord) ProtoMessage() {}
|
|
|
|
func (x *BuildHistoryRecord) ProtoReflect() protoreflect.Message {
|
|
mi := &file_control_proto_msgTypes[21]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use BuildHistoryRecord.ProtoReflect.Descriptor instead.
|
|
func (*BuildHistoryRecord) Descriptor() ([]byte, []int) {
|
|
return file_control_proto_rawDescGZIP(), []int{21}
|
|
}
|
|
|
|
func (x *BuildHistoryRecord) GetRef() string {
|
|
if x != nil {
|
|
return x.Ref
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *BuildHistoryRecord) GetFrontend() string {
|
|
if x != nil {
|
|
return x.Frontend
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *BuildHistoryRecord) GetFrontendAttrs() map[string]string {
|
|
if x != nil {
|
|
return x.FrontendAttrs
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BuildHistoryRecord) GetExporters() []*Exporter {
|
|
if x != nil {
|
|
return x.Exporters
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BuildHistoryRecord) GetError() *status.Status {
|
|
if x != nil {
|
|
return x.Error
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BuildHistoryRecord) GetCreatedAt() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.CreatedAt
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BuildHistoryRecord) GetCompletedAt() *timestamp.Timestamp {
|
|
if x != nil {
|
|
return x.CompletedAt
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BuildHistoryRecord) GetLogs() *Descriptor {
|
|
if x != nil {
|
|
return x.Logs
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BuildHistoryRecord) GetExporterResponse() map[string]string {
|
|
if x != nil {
|
|
return x.ExporterResponse
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BuildHistoryRecord) GetResult() *BuildResultInfo {
|
|
if x != nil {
|
|
return x.Result
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BuildHistoryRecord) GetResults() map[string]*BuildResultInfo {
|
|
if x != nil {
|
|
return x.Results
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BuildHistoryRecord) GetGeneration() int32 {
|
|
if x != nil {
|
|
return x.Generation
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *BuildHistoryRecord) GetTrace() *Descriptor {
|
|
if x != nil {
|
|
return x.Trace
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BuildHistoryRecord) GetPinned() bool {
|
|
if x != nil {
|
|
return x.Pinned
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *BuildHistoryRecord) GetNumCachedSteps() int32 {
|
|
if x != nil {
|
|
return x.NumCachedSteps
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *BuildHistoryRecord) GetNumTotalSteps() int32 {
|
|
if x != nil {
|
|
return x.NumTotalSteps
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *BuildHistoryRecord) GetNumCompletedSteps() int32 {
|
|
if x != nil {
|
|
return x.NumCompletedSteps
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *BuildHistoryRecord) GetExternalError() *Descriptor {
|
|
if x != nil {
|
|
return x.ExternalError
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BuildHistoryRecord) GetNumWarnings() int32 {
|
|
if x != nil {
|
|
return x.NumWarnings
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type UpdateBuildHistoryRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Ref string `protobuf:"bytes,1,opt,name=Ref,proto3" json:"Ref,omitempty"`
|
|
Pinned bool `protobuf:"varint,2,opt,name=Pinned,proto3" json:"Pinned,omitempty"`
|
|
Delete bool `protobuf:"varint,3,opt,name=Delete,proto3" json:"Delete,omitempty"`
|
|
Finalize bool `protobuf:"varint,4,opt,name=Finalize,proto3" json:"Finalize,omitempty"`
|
|
}
|
|
|
|
func (x *UpdateBuildHistoryRequest) Reset() {
|
|
*x = UpdateBuildHistoryRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_control_proto_msgTypes[22]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *UpdateBuildHistoryRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*UpdateBuildHistoryRequest) ProtoMessage() {}
|
|
|
|
func (x *UpdateBuildHistoryRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_control_proto_msgTypes[22]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use UpdateBuildHistoryRequest.ProtoReflect.Descriptor instead.
|
|
func (*UpdateBuildHistoryRequest) Descriptor() ([]byte, []int) {
|
|
return file_control_proto_rawDescGZIP(), []int{22}
|
|
}
|
|
|
|
func (x *UpdateBuildHistoryRequest) GetRef() string {
|
|
if x != nil {
|
|
return x.Ref
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *UpdateBuildHistoryRequest) GetPinned() bool {
|
|
if x != nil {
|
|
return x.Pinned
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *UpdateBuildHistoryRequest) GetDelete() bool {
|
|
if x != nil {
|
|
return x.Delete
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *UpdateBuildHistoryRequest) GetFinalize() bool {
|
|
if x != nil {
|
|
return x.Finalize
|
|
}
|
|
return false
|
|
}
|
|
|
|
type UpdateBuildHistoryResponse struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
}
|
|
|
|
func (x *UpdateBuildHistoryResponse) Reset() {
|
|
*x = UpdateBuildHistoryResponse{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_control_proto_msgTypes[23]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *UpdateBuildHistoryResponse) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*UpdateBuildHistoryResponse) ProtoMessage() {}
|
|
|
|
func (x *UpdateBuildHistoryResponse) ProtoReflect() protoreflect.Message {
|
|
mi := &file_control_proto_msgTypes[23]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use UpdateBuildHistoryResponse.ProtoReflect.Descriptor instead.
|
|
func (*UpdateBuildHistoryResponse) Descriptor() ([]byte, []int) {
|
|
return file_control_proto_rawDescGZIP(), []int{23}
|
|
}
|
|
|
|
type Descriptor struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
MediaType string `protobuf:"bytes,1,opt,name=media_type,json=mediaType,proto3" json:"media_type,omitempty"`
|
|
Digest string `protobuf:"bytes,2,opt,name=digest,proto3" json:"digest,omitempty"`
|
|
Size int64 `protobuf:"varint,3,opt,name=size,proto3" json:"size,omitempty"`
|
|
Annotations map[string]string `protobuf:"bytes,5,rep,name=annotations,proto3" json:"annotations,omitempty" protobuf_key:"bytes,1,opt,name=key,proto3" protobuf_val:"bytes,2,opt,name=value,proto3"`
|
|
}
|
|
|
|
func (x *Descriptor) Reset() {
|
|
*x = Descriptor{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_control_proto_msgTypes[24]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *Descriptor) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*Descriptor) ProtoMessage() {}
|
|
|
|
func (x *Descriptor) ProtoReflect() protoreflect.Message {
|
|
mi := &file_control_proto_msgTypes[24]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use Descriptor.ProtoReflect.Descriptor instead.
|
|
func (*Descriptor) Descriptor() ([]byte, []int) {
|
|
return file_control_proto_rawDescGZIP(), []int{24}
|
|
}
|
|
|
|
func (x *Descriptor) GetMediaType() string {
|
|
if x != nil {
|
|
return x.MediaType
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *Descriptor) GetDigest() string {
|
|
if x != nil {
|
|
return x.Digest
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *Descriptor) GetSize() int64 {
|
|
if x != nil {
|
|
return x.Size
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *Descriptor) GetAnnotations() map[string]string {
|
|
if x != nil {
|
|
return x.Annotations
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type BuildResultInfo struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
ResultDeprecated *Descriptor `protobuf:"bytes,1,opt,name=ResultDeprecated,proto3" json:"ResultDeprecated,omitempty"`
|
|
Attestations []*Descriptor `protobuf:"bytes,2,rep,name=Attestations,proto3" json:"Attestations,omitempty"`
|
|
Results map[int64]*Descriptor `protobuf:"bytes,3,rep,name=Results,proto3" json:"Results,omitempty" protobuf_key:"varint,1,opt,name=key,proto3" protobuf_val:"bytes,2,opt,name=value,proto3"`
|
|
}
|
|
|
|
func (x *BuildResultInfo) Reset() {
|
|
*x = BuildResultInfo{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_control_proto_msgTypes[25]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *BuildResultInfo) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*BuildResultInfo) ProtoMessage() {}
|
|
|
|
func (x *BuildResultInfo) ProtoReflect() protoreflect.Message {
|
|
mi := &file_control_proto_msgTypes[25]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use BuildResultInfo.ProtoReflect.Descriptor instead.
|
|
func (*BuildResultInfo) Descriptor() ([]byte, []int) {
|
|
return file_control_proto_rawDescGZIP(), []int{25}
|
|
}
|
|
|
|
func (x *BuildResultInfo) GetResultDeprecated() *Descriptor {
|
|
if x != nil {
|
|
return x.ResultDeprecated
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BuildResultInfo) GetAttestations() []*Descriptor {
|
|
if x != nil {
|
|
return x.Attestations
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BuildResultInfo) GetResults() map[int64]*Descriptor {
|
|
if x != nil {
|
|
return x.Results
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// Exporter describes the output exporter
|
|
type Exporter struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
// Type identifies the exporter
|
|
Type string `protobuf:"bytes,1,opt,name=Type,proto3" json:"Type,omitempty"`
|
|
// Attrs specifies exporter configuration
|
|
Attrs map[string]string `protobuf:"bytes,2,rep,name=Attrs,proto3" json:"Attrs,omitempty" protobuf_key:"bytes,1,opt,name=key,proto3" protobuf_val:"bytes,2,opt,name=value,proto3"`
|
|
}
|
|
|
|
func (x *Exporter) Reset() {
|
|
*x = Exporter{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_control_proto_msgTypes[26]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *Exporter) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*Exporter) ProtoMessage() {}
|
|
|
|
func (x *Exporter) ProtoReflect() protoreflect.Message {
|
|
mi := &file_control_proto_msgTypes[26]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use Exporter.ProtoReflect.Descriptor instead.
|
|
func (*Exporter) Descriptor() ([]byte, []int) {
|
|
return file_control_proto_rawDescGZIP(), []int{26}
|
|
}
|
|
|
|
func (x *Exporter) GetType() string {
|
|
if x != nil {
|
|
return x.Type
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *Exporter) GetAttrs() map[string]string {
|
|
if x != nil {
|
|
return x.Attrs
|
|
}
|
|
return nil
|
|
}
|
|
|
|
var File_control_proto protoreflect.FileDescriptor
|
|
|
|
var file_control_proto_rawDesc = []byte{
|
|
0x0a, 0x0d, 0x63, 0x6f, 0x6e, 0x74, 0x72, 0x6f, 0x6c, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x12,
|
|
0x10, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76,
|
|
0x31, 0x1a, 0x2f, 0x67, 0x69, 0x74, 0x68, 0x75, 0x62, 0x2e, 0x63, 0x6f, 0x6d, 0x2f, 0x6d, 0x6f,
|
|
0x62, 0x79, 0x2f, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2f, 0x61, 0x70, 0x69, 0x2f,
|
|
0x74, 0x79, 0x70, 0x65, 0x73, 0x2f, 0x77, 0x6f, 0x72, 0x6b, 0x65, 0x72, 0x2e, 0x70, 0x72, 0x6f,
|
|
0x74, 0x6f, 0x1a, 0x2c, 0x67, 0x69, 0x74, 0x68, 0x75, 0x62, 0x2e, 0x63, 0x6f, 0x6d, 0x2f, 0x6d,
|
|
0x6f, 0x62, 0x79, 0x2f, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2f, 0x73, 0x6f, 0x6c,
|
|
0x76, 0x65, 0x72, 0x2f, 0x70, 0x62, 0x2f, 0x6f, 0x70, 0x73, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f,
|
|
0x1a, 0x35, 0x67, 0x69, 0x74, 0x68, 0x75, 0x62, 0x2e, 0x63, 0x6f, 0x6d, 0x2f, 0x6d, 0x6f, 0x62,
|
|
0x79, 0x2f, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2f, 0x73, 0x6f, 0x75, 0x72, 0x63,
|
|
0x65, 0x70, 0x6f, 0x6c, 0x69, 0x63, 0x79, 0x2f, 0x70, 0x62, 0x2f, 0x70, 0x6f, 0x6c, 0x69, 0x63,
|
|
0x79, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x1a, 0x1f, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2f,
|
|
0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2f, 0x74, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61,
|
|
0x6d, 0x70, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x1a, 0x17, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65,
|
|
0x2f, 0x72, 0x70, 0x63, 0x2f, 0x73, 0x74, 0x61, 0x74, 0x75, 0x73, 0x2e, 0x70, 0x72, 0x6f, 0x74,
|
|
0x6f, 0x22, 0xb0, 0x01, 0x0a, 0x0c, 0x50, 0x72, 0x75, 0x6e, 0x65, 0x52, 0x65, 0x71, 0x75, 0x65,
|
|
0x73, 0x74, 0x12, 0x16, 0x0a, 0x06, 0x66, 0x69, 0x6c, 0x74, 0x65, 0x72, 0x18, 0x01, 0x20, 0x03,
|
|
0x28, 0x09, 0x52, 0x06, 0x66, 0x69, 0x6c, 0x74, 0x65, 0x72, 0x12, 0x10, 0x0a, 0x03, 0x61, 0x6c,
|
|
0x6c, 0x18, 0x02, 0x20, 0x01, 0x28, 0x08, 0x52, 0x03, 0x61, 0x6c, 0x6c, 0x12, 0x22, 0x0a, 0x0c,
|
|
0x6b, 0x65, 0x65, 0x70, 0x44, 0x75, 0x72, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x18, 0x03, 0x20, 0x01,
|
|
0x28, 0x03, 0x52, 0x0c, 0x6b, 0x65, 0x65, 0x70, 0x44, 0x75, 0x72, 0x61, 0x74, 0x69, 0x6f, 0x6e,
|
|
0x12, 0x1e, 0x0a, 0x0a, 0x6d, 0x69, 0x6e, 0x53, 0x74, 0x6f, 0x72, 0x61, 0x67, 0x65, 0x18, 0x05,
|
|
0x20, 0x01, 0x28, 0x03, 0x52, 0x0a, 0x6d, 0x69, 0x6e, 0x53, 0x74, 0x6f, 0x72, 0x61, 0x67, 0x65,
|
|
0x12, 0x1e, 0x0a, 0x0a, 0x6d, 0x61, 0x78, 0x53, 0x74, 0x6f, 0x72, 0x61, 0x67, 0x65, 0x18, 0x04,
|
|
0x20, 0x01, 0x28, 0x03, 0x52, 0x0a, 0x6d, 0x61, 0x78, 0x53, 0x74, 0x6f, 0x72, 0x61, 0x67, 0x65,
|
|
0x12, 0x12, 0x0a, 0x04, 0x66, 0x72, 0x65, 0x65, 0x18, 0x06, 0x20, 0x01, 0x28, 0x03, 0x52, 0x04,
|
|
0x66, 0x72, 0x65, 0x65, 0x22, 0x2a, 0x0a, 0x10, 0x44, 0x69, 0x73, 0x6b, 0x55, 0x73, 0x61, 0x67,
|
|
0x65, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x12, 0x16, 0x0a, 0x06, 0x66, 0x69, 0x6c, 0x74,
|
|
0x65, 0x72, 0x18, 0x01, 0x20, 0x03, 0x28, 0x09, 0x52, 0x06, 0x66, 0x69, 0x6c, 0x74, 0x65, 0x72,
|
|
0x22, 0x4a, 0x0a, 0x11, 0x44, 0x69, 0x73, 0x6b, 0x55, 0x73, 0x61, 0x67, 0x65, 0x52, 0x65, 0x73,
|
|
0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x35, 0x0a, 0x06, 0x72, 0x65, 0x63, 0x6f, 0x72, 0x64, 0x18,
|
|
0x01, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x1d, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69,
|
|
0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x55, 0x73, 0x61, 0x67, 0x65, 0x52, 0x65,
|
|
0x63, 0x6f, 0x72, 0x64, 0x52, 0x06, 0x72, 0x65, 0x63, 0x6f, 0x72, 0x64, 0x22, 0x87, 0x03, 0x0a,
|
|
0x0b, 0x55, 0x73, 0x61, 0x67, 0x65, 0x52, 0x65, 0x63, 0x6f, 0x72, 0x64, 0x12, 0x0e, 0x0a, 0x02,
|
|
0x49, 0x44, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x02, 0x49, 0x44, 0x12, 0x18, 0x0a, 0x07,
|
|
0x4d, 0x75, 0x74, 0x61, 0x62, 0x6c, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x08, 0x52, 0x07, 0x4d,
|
|
0x75, 0x74, 0x61, 0x62, 0x6c, 0x65, 0x12, 0x14, 0x0a, 0x05, 0x49, 0x6e, 0x55, 0x73, 0x65, 0x18,
|
|
0x03, 0x20, 0x01, 0x28, 0x08, 0x52, 0x05, 0x49, 0x6e, 0x55, 0x73, 0x65, 0x12, 0x12, 0x0a, 0x04,
|
|
0x53, 0x69, 0x7a, 0x65, 0x18, 0x04, 0x20, 0x01, 0x28, 0x03, 0x52, 0x04, 0x53, 0x69, 0x7a, 0x65,
|
|
0x12, 0x1a, 0x0a, 0x06, 0x50, 0x61, 0x72, 0x65, 0x6e, 0x74, 0x18, 0x05, 0x20, 0x01, 0x28, 0x09,
|
|
0x42, 0x02, 0x18, 0x01, 0x52, 0x06, 0x50, 0x61, 0x72, 0x65, 0x6e, 0x74, 0x12, 0x38, 0x0a, 0x09,
|
|
0x43, 0x72, 0x65, 0x61, 0x74, 0x65, 0x64, 0x41, 0x74, 0x18, 0x06, 0x20, 0x01, 0x28, 0x0b, 0x32,
|
|
0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75,
|
|
0x66, 0x2e, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x52, 0x09, 0x43, 0x72, 0x65,
|
|
0x61, 0x74, 0x65, 0x64, 0x41, 0x74, 0x12, 0x3a, 0x0a, 0x0a, 0x4c, 0x61, 0x73, 0x74, 0x55, 0x73,
|
|
0x65, 0x64, 0x41, 0x74, 0x18, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x67, 0x6f, 0x6f,
|
|
0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x54, 0x69, 0x6d,
|
|
0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x52, 0x0a, 0x4c, 0x61, 0x73, 0x74, 0x55, 0x73, 0x65, 0x64,
|
|
0x41, 0x74, 0x12, 0x1e, 0x0a, 0x0a, 0x55, 0x73, 0x61, 0x67, 0x65, 0x43, 0x6f, 0x75, 0x6e, 0x74,
|
|
0x18, 0x08, 0x20, 0x01, 0x28, 0x03, 0x52, 0x0a, 0x55, 0x73, 0x61, 0x67, 0x65, 0x43, 0x6f, 0x75,
|
|
0x6e, 0x74, 0x12, 0x20, 0x0a, 0x0b, 0x44, 0x65, 0x73, 0x63, 0x72, 0x69, 0x70, 0x74, 0x69, 0x6f,
|
|
0x6e, 0x18, 0x09, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0b, 0x44, 0x65, 0x73, 0x63, 0x72, 0x69, 0x70,
|
|
0x74, 0x69, 0x6f, 0x6e, 0x12, 0x1e, 0x0a, 0x0a, 0x52, 0x65, 0x63, 0x6f, 0x72, 0x64, 0x54, 0x79,
|
|
0x70, 0x65, 0x18, 0x0a, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0a, 0x52, 0x65, 0x63, 0x6f, 0x72, 0x64,
|
|
0x54, 0x79, 0x70, 0x65, 0x12, 0x16, 0x0a, 0x06, 0x53, 0x68, 0x61, 0x72, 0x65, 0x64, 0x18, 0x0b,
|
|
0x20, 0x01, 0x28, 0x08, 0x52, 0x06, 0x53, 0x68, 0x61, 0x72, 0x65, 0x64, 0x12, 0x18, 0x0a, 0x07,
|
|
0x50, 0x61, 0x72, 0x65, 0x6e, 0x74, 0x73, 0x18, 0x0c, 0x20, 0x03, 0x28, 0x09, 0x52, 0x07, 0x50,
|
|
0x61, 0x72, 0x65, 0x6e, 0x74, 0x73, 0x22, 0xbe, 0x07, 0x0a, 0x0c, 0x53, 0x6f, 0x6c, 0x76, 0x65,
|
|
0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x12, 0x10, 0x0a, 0x03, 0x52, 0x65, 0x66, 0x18, 0x01,
|
|
0x20, 0x01, 0x28, 0x09, 0x52, 0x03, 0x52, 0x65, 0x66, 0x12, 0x2e, 0x0a, 0x0a, 0x44, 0x65, 0x66,
|
|
0x69, 0x6e, 0x69, 0x74, 0x69, 0x6f, 0x6e, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x0e, 0x2e,
|
|
0x70, 0x62, 0x2e, 0x44, 0x65, 0x66, 0x69, 0x6e, 0x69, 0x74, 0x69, 0x6f, 0x6e, 0x52, 0x0a, 0x44,
|
|
0x65, 0x66, 0x69, 0x6e, 0x69, 0x74, 0x69, 0x6f, 0x6e, 0x12, 0x2e, 0x0a, 0x12, 0x45, 0x78, 0x70,
|
|
0x6f, 0x72, 0x74, 0x65, 0x72, 0x44, 0x65, 0x70, 0x72, 0x65, 0x63, 0x61, 0x74, 0x65, 0x64, 0x18,
|
|
0x03, 0x20, 0x01, 0x28, 0x09, 0x52, 0x12, 0x45, 0x78, 0x70, 0x6f, 0x72, 0x74, 0x65, 0x72, 0x44,
|
|
0x65, 0x70, 0x72, 0x65, 0x63, 0x61, 0x74, 0x65, 0x64, 0x12, 0x75, 0x0a, 0x17, 0x45, 0x78, 0x70,
|
|
0x6f, 0x72, 0x74, 0x65, 0x72, 0x41, 0x74, 0x74, 0x72, 0x73, 0x44, 0x65, 0x70, 0x72, 0x65, 0x63,
|
|
0x61, 0x74, 0x65, 0x64, 0x18, 0x04, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x3b, 0x2e, 0x6d, 0x6f, 0x62,
|
|
0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x53, 0x6f,
|
|
0x6c, 0x76, 0x65, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x2e, 0x45, 0x78, 0x70, 0x6f, 0x72,
|
|
0x74, 0x65, 0x72, 0x41, 0x74, 0x74, 0x72, 0x73, 0x44, 0x65, 0x70, 0x72, 0x65, 0x63, 0x61, 0x74,
|
|
0x65, 0x64, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x52, 0x17, 0x45, 0x78, 0x70, 0x6f, 0x72, 0x74, 0x65,
|
|
0x72, 0x41, 0x74, 0x74, 0x72, 0x73, 0x44, 0x65, 0x70, 0x72, 0x65, 0x63, 0x61, 0x74, 0x65, 0x64,
|
|
0x12, 0x18, 0x0a, 0x07, 0x53, 0x65, 0x73, 0x73, 0x69, 0x6f, 0x6e, 0x18, 0x05, 0x20, 0x01, 0x28,
|
|
0x09, 0x52, 0x07, 0x53, 0x65, 0x73, 0x73, 0x69, 0x6f, 0x6e, 0x12, 0x1a, 0x0a, 0x08, 0x46, 0x72,
|
|
0x6f, 0x6e, 0x74, 0x65, 0x6e, 0x64, 0x18, 0x06, 0x20, 0x01, 0x28, 0x09, 0x52, 0x08, 0x46, 0x72,
|
|
0x6f, 0x6e, 0x74, 0x65, 0x6e, 0x64, 0x12, 0x57, 0x0a, 0x0d, 0x46, 0x72, 0x6f, 0x6e, 0x74, 0x65,
|
|
0x6e, 0x64, 0x41, 0x74, 0x74, 0x72, 0x73, 0x18, 0x07, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x31, 0x2e,
|
|
0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31,
|
|
0x2e, 0x53, 0x6f, 0x6c, 0x76, 0x65, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x2e, 0x46, 0x72,
|
|
0x6f, 0x6e, 0x74, 0x65, 0x6e, 0x64, 0x41, 0x74, 0x74, 0x72, 0x73, 0x45, 0x6e, 0x74, 0x72, 0x79,
|
|
0x52, 0x0d, 0x46, 0x72, 0x6f, 0x6e, 0x74, 0x65, 0x6e, 0x64, 0x41, 0x74, 0x74, 0x72, 0x73, 0x12,
|
|
0x34, 0x0a, 0x05, 0x43, 0x61, 0x63, 0x68, 0x65, 0x18, 0x08, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1e,
|
|
0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76,
|
|
0x31, 0x2e, 0x43, 0x61, 0x63, 0x68, 0x65, 0x4f, 0x70, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x52, 0x05,
|
|
0x43, 0x61, 0x63, 0x68, 0x65, 0x12, 0x22, 0x0a, 0x0c, 0x45, 0x6e, 0x74, 0x69, 0x74, 0x6c, 0x65,
|
|
0x6d, 0x65, 0x6e, 0x74, 0x73, 0x18, 0x09, 0x20, 0x03, 0x28, 0x09, 0x52, 0x0c, 0x45, 0x6e, 0x74,
|
|
0x69, 0x74, 0x6c, 0x65, 0x6d, 0x65, 0x6e, 0x74, 0x73, 0x12, 0x5a, 0x0a, 0x0e, 0x46, 0x72, 0x6f,
|
|
0x6e, 0x74, 0x65, 0x6e, 0x64, 0x49, 0x6e, 0x70, 0x75, 0x74, 0x73, 0x18, 0x0a, 0x20, 0x03, 0x28,
|
|
0x0b, 0x32, 0x32, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69,
|
|
0x74, 0x2e, 0x76, 0x31, 0x2e, 0x53, 0x6f, 0x6c, 0x76, 0x65, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73,
|
|
0x74, 0x2e, 0x46, 0x72, 0x6f, 0x6e, 0x74, 0x65, 0x6e, 0x64, 0x49, 0x6e, 0x70, 0x75, 0x74, 0x73,
|
|
0x45, 0x6e, 0x74, 0x72, 0x79, 0x52, 0x0e, 0x46, 0x72, 0x6f, 0x6e, 0x74, 0x65, 0x6e, 0x64, 0x49,
|
|
0x6e, 0x70, 0x75, 0x74, 0x73, 0x12, 0x1a, 0x0a, 0x08, 0x49, 0x6e, 0x74, 0x65, 0x72, 0x6e, 0x61,
|
|
0x6c, 0x18, 0x0b, 0x20, 0x01, 0x28, 0x08, 0x52, 0x08, 0x49, 0x6e, 0x74, 0x65, 0x72, 0x6e, 0x61,
|
|
0x6c, 0x12, 0x49, 0x0a, 0x0c, 0x53, 0x6f, 0x75, 0x72, 0x63, 0x65, 0x50, 0x6f, 0x6c, 0x69, 0x63,
|
|
0x79, 0x18, 0x0c, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x25, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62,
|
|
0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x73, 0x6f, 0x75, 0x72, 0x63,
|
|
0x65, 0x70, 0x6f, 0x6c, 0x69, 0x63, 0x79, 0x2e, 0x50, 0x6f, 0x6c, 0x69, 0x63, 0x79, 0x52, 0x0c,
|
|
0x53, 0x6f, 0x75, 0x72, 0x63, 0x65, 0x50, 0x6f, 0x6c, 0x69, 0x63, 0x79, 0x12, 0x38, 0x0a, 0x09,
|
|
0x45, 0x78, 0x70, 0x6f, 0x72, 0x74, 0x65, 0x72, 0x73, 0x18, 0x0d, 0x20, 0x03, 0x28, 0x0b, 0x32,
|
|
0x1a, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e,
|
|
0x76, 0x31, 0x2e, 0x45, 0x78, 0x70, 0x6f, 0x72, 0x74, 0x65, 0x72, 0x52, 0x09, 0x45, 0x78, 0x70,
|
|
0x6f, 0x72, 0x74, 0x65, 0x72, 0x73, 0x1a, 0x4a, 0x0a, 0x1c, 0x45, 0x78, 0x70, 0x6f, 0x72, 0x74,
|
|
0x65, 0x72, 0x41, 0x74, 0x74, 0x72, 0x73, 0x44, 0x65, 0x70, 0x72, 0x65, 0x63, 0x61, 0x74, 0x65,
|
|
0x64, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x12, 0x10, 0x0a, 0x03, 0x6b, 0x65, 0x79, 0x18, 0x01, 0x20,
|
|
0x01, 0x28, 0x09, 0x52, 0x03, 0x6b, 0x65, 0x79, 0x12, 0x14, 0x0a, 0x05, 0x76, 0x61, 0x6c, 0x75,
|
|
0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x3a, 0x02,
|
|
0x38, 0x01, 0x1a, 0x40, 0x0a, 0x12, 0x46, 0x72, 0x6f, 0x6e, 0x74, 0x65, 0x6e, 0x64, 0x41, 0x74,
|
|
0x74, 0x72, 0x73, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x12, 0x10, 0x0a, 0x03, 0x6b, 0x65, 0x79, 0x18,
|
|
0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x03, 0x6b, 0x65, 0x79, 0x12, 0x14, 0x0a, 0x05, 0x76, 0x61,
|
|
0x6c, 0x75, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x05, 0x76, 0x61, 0x6c, 0x75, 0x65,
|
|
0x3a, 0x02, 0x38, 0x01, 0x1a, 0x51, 0x0a, 0x13, 0x46, 0x72, 0x6f, 0x6e, 0x74, 0x65, 0x6e, 0x64,
|
|
0x49, 0x6e, 0x70, 0x75, 0x74, 0x73, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x12, 0x10, 0x0a, 0x03, 0x6b,
|
|
0x65, 0x79, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x03, 0x6b, 0x65, 0x79, 0x12, 0x24, 0x0a,
|
|
0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x0e, 0x2e, 0x70,
|
|
0x62, 0x2e, 0x44, 0x65, 0x66, 0x69, 0x6e, 0x69, 0x74, 0x69, 0x6f, 0x6e, 0x52, 0x05, 0x76, 0x61,
|
|
0x6c, 0x75, 0x65, 0x3a, 0x02, 0x38, 0x01, 0x22, 0xad, 0x03, 0x0a, 0x0c, 0x43, 0x61, 0x63, 0x68,
|
|
0x65, 0x4f, 0x70, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x12, 0x30, 0x0a, 0x13, 0x45, 0x78, 0x70, 0x6f,
|
|
0x72, 0x74, 0x52, 0x65, 0x66, 0x44, 0x65, 0x70, 0x72, 0x65, 0x63, 0x61, 0x74, 0x65, 0x64, 0x18,
|
|
0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x13, 0x45, 0x78, 0x70, 0x6f, 0x72, 0x74, 0x52, 0x65, 0x66,
|
|
0x44, 0x65, 0x70, 0x72, 0x65, 0x63, 0x61, 0x74, 0x65, 0x64, 0x12, 0x32, 0x0a, 0x14, 0x49, 0x6d,
|
|
0x70, 0x6f, 0x72, 0x74, 0x52, 0x65, 0x66, 0x73, 0x44, 0x65, 0x70, 0x72, 0x65, 0x63, 0x61, 0x74,
|
|
0x65, 0x64, 0x18, 0x02, 0x20, 0x03, 0x28, 0x09, 0x52, 0x14, 0x49, 0x6d, 0x70, 0x6f, 0x72, 0x74,
|
|
0x52, 0x65, 0x66, 0x73, 0x44, 0x65, 0x70, 0x72, 0x65, 0x63, 0x61, 0x74, 0x65, 0x64, 0x12, 0x6f,
|
|
0x0a, 0x15, 0x45, 0x78, 0x70, 0x6f, 0x72, 0x74, 0x41, 0x74, 0x74, 0x72, 0x73, 0x44, 0x65, 0x70,
|
|
0x72, 0x65, 0x63, 0x61, 0x74, 0x65, 0x64, 0x18, 0x03, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x39, 0x2e,
|
|
0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31,
|
|
0x2e, 0x43, 0x61, 0x63, 0x68, 0x65, 0x4f, 0x70, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x2e, 0x45, 0x78,
|
|
0x70, 0x6f, 0x72, 0x74, 0x41, 0x74, 0x74, 0x72, 0x73, 0x44, 0x65, 0x70, 0x72, 0x65, 0x63, 0x61,
|
|
0x74, 0x65, 0x64, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x52, 0x15, 0x45, 0x78, 0x70, 0x6f, 0x72, 0x74,
|
|
0x41, 0x74, 0x74, 0x72, 0x73, 0x44, 0x65, 0x70, 0x72, 0x65, 0x63, 0x61, 0x74, 0x65, 0x64, 0x12,
|
|
0x3d, 0x0a, 0x07, 0x45, 0x78, 0x70, 0x6f, 0x72, 0x74, 0x73, 0x18, 0x04, 0x20, 0x03, 0x28, 0x0b,
|
|
0x32, 0x23, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74,
|
|
0x2e, 0x76, 0x31, 0x2e, 0x43, 0x61, 0x63, 0x68, 0x65, 0x4f, 0x70, 0x74, 0x69, 0x6f, 0x6e, 0x73,
|
|
0x45, 0x6e, 0x74, 0x72, 0x79, 0x52, 0x07, 0x45, 0x78, 0x70, 0x6f, 0x72, 0x74, 0x73, 0x12, 0x3d,
|
|
0x0a, 0x07, 0x49, 0x6d, 0x70, 0x6f, 0x72, 0x74, 0x73, 0x18, 0x05, 0x20, 0x03, 0x28, 0x0b, 0x32,
|
|
0x23, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e,
|
|
0x76, 0x31, 0x2e, 0x43, 0x61, 0x63, 0x68, 0x65, 0x4f, 0x70, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x45,
|
|
0x6e, 0x74, 0x72, 0x79, 0x52, 0x07, 0x49, 0x6d, 0x70, 0x6f, 0x72, 0x74, 0x73, 0x1a, 0x48, 0x0a,
|
|
0x1a, 0x45, 0x78, 0x70, 0x6f, 0x72, 0x74, 0x41, 0x74, 0x74, 0x72, 0x73, 0x44, 0x65, 0x70, 0x72,
|
|
0x65, 0x63, 0x61, 0x74, 0x65, 0x64, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x12, 0x10, 0x0a, 0x03, 0x6b,
|
|
0x65, 0x79, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x03, 0x6b, 0x65, 0x79, 0x12, 0x14, 0x0a,
|
|
0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x05, 0x76, 0x61,
|
|
0x6c, 0x75, 0x65, 0x3a, 0x02, 0x38, 0x01, 0x22, 0xa7, 0x01, 0x0a, 0x11, 0x43, 0x61, 0x63, 0x68,
|
|
0x65, 0x4f, 0x70, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x12, 0x12, 0x0a,
|
|
0x04, 0x54, 0x79, 0x70, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x04, 0x54, 0x79, 0x70,
|
|
0x65, 0x12, 0x44, 0x0a, 0x05, 0x41, 0x74, 0x74, 0x72, 0x73, 0x18, 0x02, 0x20, 0x03, 0x28, 0x0b,
|
|
0x32, 0x2e, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74,
|
|
0x2e, 0x76, 0x31, 0x2e, 0x43, 0x61, 0x63, 0x68, 0x65, 0x4f, 0x70, 0x74, 0x69, 0x6f, 0x6e, 0x73,
|
|
0x45, 0x6e, 0x74, 0x72, 0x79, 0x2e, 0x41, 0x74, 0x74, 0x72, 0x73, 0x45, 0x6e, 0x74, 0x72, 0x79,
|
|
0x52, 0x05, 0x41, 0x74, 0x74, 0x72, 0x73, 0x1a, 0x38, 0x0a, 0x0a, 0x41, 0x74, 0x74, 0x72, 0x73,
|
|
0x45, 0x6e, 0x74, 0x72, 0x79, 0x12, 0x10, 0x0a, 0x03, 0x6b, 0x65, 0x79, 0x18, 0x01, 0x20, 0x01,
|
|
0x28, 0x09, 0x52, 0x03, 0x6b, 0x65, 0x79, 0x12, 0x14, 0x0a, 0x05, 0x76, 0x61, 0x6c, 0x75, 0x65,
|
|
0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x3a, 0x02, 0x38,
|
|
0x01, 0x22, 0xb7, 0x01, 0x0a, 0x0d, 0x53, 0x6f, 0x6c, 0x76, 0x65, 0x52, 0x65, 0x73, 0x70, 0x6f,
|
|
0x6e, 0x73, 0x65, 0x12, 0x61, 0x0a, 0x10, 0x45, 0x78, 0x70, 0x6f, 0x72, 0x74, 0x65, 0x72, 0x52,
|
|
0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x18, 0x01, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x35, 0x2e,
|
|
0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31,
|
|
0x2e, 0x53, 0x6f, 0x6c, 0x76, 0x65, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x2e, 0x45,
|
|
0x78, 0x70, 0x6f, 0x72, 0x74, 0x65, 0x72, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x45,
|
|
0x6e, 0x74, 0x72, 0x79, 0x52, 0x10, 0x45, 0x78, 0x70, 0x6f, 0x72, 0x74, 0x65, 0x72, 0x52, 0x65,
|
|
0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x1a, 0x43, 0x0a, 0x15, 0x45, 0x78, 0x70, 0x6f, 0x72, 0x74,
|
|
0x65, 0x72, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x12,
|
|
0x10, 0x0a, 0x03, 0x6b, 0x65, 0x79, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x03, 0x6b, 0x65,
|
|
0x79, 0x12, 0x14, 0x0a, 0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09,
|
|
0x52, 0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x3a, 0x02, 0x38, 0x01, 0x22, 0x21, 0x0a, 0x0d, 0x53,
|
|
0x74, 0x61, 0x74, 0x75, 0x73, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x12, 0x10, 0x0a, 0x03,
|
|
0x52, 0x65, 0x66, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x03, 0x52, 0x65, 0x66, 0x22, 0xf0,
|
|
0x01, 0x0a, 0x0e, 0x53, 0x74, 0x61, 0x74, 0x75, 0x73, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73,
|
|
0x65, 0x12, 0x34, 0x0a, 0x08, 0x76, 0x65, 0x72, 0x74, 0x65, 0x78, 0x65, 0x73, 0x18, 0x01, 0x20,
|
|
0x03, 0x28, 0x0b, 0x32, 0x18, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64,
|
|
0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x56, 0x65, 0x72, 0x74, 0x65, 0x78, 0x52, 0x08, 0x76,
|
|
0x65, 0x72, 0x74, 0x65, 0x78, 0x65, 0x73, 0x12, 0x3a, 0x0a, 0x08, 0x73, 0x74, 0x61, 0x74, 0x75,
|
|
0x73, 0x65, 0x73, 0x18, 0x02, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x1e, 0x2e, 0x6d, 0x6f, 0x62, 0x79,
|
|
0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x56, 0x65, 0x72,
|
|
0x74, 0x65, 0x78, 0x53, 0x74, 0x61, 0x74, 0x75, 0x73, 0x52, 0x08, 0x73, 0x74, 0x61, 0x74, 0x75,
|
|
0x73, 0x65, 0x73, 0x12, 0x2f, 0x0a, 0x04, 0x6c, 0x6f, 0x67, 0x73, 0x18, 0x03, 0x20, 0x03, 0x28,
|
|
0x0b, 0x32, 0x1b, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69,
|
|
0x74, 0x2e, 0x76, 0x31, 0x2e, 0x56, 0x65, 0x72, 0x74, 0x65, 0x78, 0x4c, 0x6f, 0x67, 0x52, 0x04,
|
|
0x6c, 0x6f, 0x67, 0x73, 0x12, 0x3b, 0x0a, 0x08, 0x77, 0x61, 0x72, 0x6e, 0x69, 0x6e, 0x67, 0x73,
|
|
0x18, 0x04, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x1f, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75,
|
|
0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x56, 0x65, 0x72, 0x74, 0x65, 0x78,
|
|
0x57, 0x61, 0x72, 0x6e, 0x69, 0x6e, 0x67, 0x52, 0x08, 0x77, 0x61, 0x72, 0x6e, 0x69, 0x6e, 0x67,
|
|
0x73, 0x22, 0xa3, 0x02, 0x0a, 0x06, 0x56, 0x65, 0x72, 0x74, 0x65, 0x78, 0x12, 0x16, 0x0a, 0x06,
|
|
0x64, 0x69, 0x67, 0x65, 0x73, 0x74, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x06, 0x64, 0x69,
|
|
0x67, 0x65, 0x73, 0x74, 0x12, 0x16, 0x0a, 0x06, 0x69, 0x6e, 0x70, 0x75, 0x74, 0x73, 0x18, 0x02,
|
|
0x20, 0x03, 0x28, 0x09, 0x52, 0x06, 0x69, 0x6e, 0x70, 0x75, 0x74, 0x73, 0x12, 0x12, 0x0a, 0x04,
|
|
0x6e, 0x61, 0x6d, 0x65, 0x18, 0x03, 0x20, 0x01, 0x28, 0x09, 0x52, 0x04, 0x6e, 0x61, 0x6d, 0x65,
|
|
0x12, 0x16, 0x0a, 0x06, 0x63, 0x61, 0x63, 0x68, 0x65, 0x64, 0x18, 0x04, 0x20, 0x01, 0x28, 0x08,
|
|
0x52, 0x06, 0x63, 0x61, 0x63, 0x68, 0x65, 0x64, 0x12, 0x34, 0x0a, 0x07, 0x73, 0x74, 0x61, 0x72,
|
|
0x74, 0x65, 0x64, 0x18, 0x05, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67,
|
|
0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x54, 0x69, 0x6d, 0x65,
|
|
0x73, 0x74, 0x61, 0x6d, 0x70, 0x52, 0x07, 0x73, 0x74, 0x61, 0x72, 0x74, 0x65, 0x64, 0x12, 0x38,
|
|
0x0a, 0x09, 0x63, 0x6f, 0x6d, 0x70, 0x6c, 0x65, 0x74, 0x65, 0x64, 0x18, 0x06, 0x20, 0x01, 0x28,
|
|
0x0b, 0x32, 0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f,
|
|
0x62, 0x75, 0x66, 0x2e, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x52, 0x09, 0x63,
|
|
0x6f, 0x6d, 0x70, 0x6c, 0x65, 0x74, 0x65, 0x64, 0x12, 0x14, 0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f,
|
|
0x72, 0x18, 0x07, 0x20, 0x01, 0x28, 0x09, 0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x12, 0x37,
|
|
0x0a, 0x0d, 0x70, 0x72, 0x6f, 0x67, 0x72, 0x65, 0x73, 0x73, 0x47, 0x72, 0x6f, 0x75, 0x70, 0x18,
|
|
0x08, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x11, 0x2e, 0x70, 0x62, 0x2e, 0x50, 0x72, 0x6f, 0x67, 0x72,
|
|
0x65, 0x73, 0x73, 0x47, 0x72, 0x6f, 0x75, 0x70, 0x52, 0x0d, 0x70, 0x72, 0x6f, 0x67, 0x72, 0x65,
|
|
0x73, 0x73, 0x47, 0x72, 0x6f, 0x75, 0x70, 0x22, 0xa4, 0x02, 0x0a, 0x0c, 0x56, 0x65, 0x72, 0x74,
|
|
0x65, 0x78, 0x53, 0x74, 0x61, 0x74, 0x75, 0x73, 0x12, 0x0e, 0x0a, 0x02, 0x49, 0x44, 0x18, 0x01,
|
|
0x20, 0x01, 0x28, 0x09, 0x52, 0x02, 0x49, 0x44, 0x12, 0x16, 0x0a, 0x06, 0x76, 0x65, 0x72, 0x74,
|
|
0x65, 0x78, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x06, 0x76, 0x65, 0x72, 0x74, 0x65, 0x78,
|
|
0x12, 0x12, 0x0a, 0x04, 0x6e, 0x61, 0x6d, 0x65, 0x18, 0x03, 0x20, 0x01, 0x28, 0x09, 0x52, 0x04,
|
|
0x6e, 0x61, 0x6d, 0x65, 0x12, 0x18, 0x0a, 0x07, 0x63, 0x75, 0x72, 0x72, 0x65, 0x6e, 0x74, 0x18,
|
|
0x04, 0x20, 0x01, 0x28, 0x03, 0x52, 0x07, 0x63, 0x75, 0x72, 0x72, 0x65, 0x6e, 0x74, 0x12, 0x14,
|
|
0x0a, 0x05, 0x74, 0x6f, 0x74, 0x61, 0x6c, 0x18, 0x05, 0x20, 0x01, 0x28, 0x03, 0x52, 0x05, 0x74,
|
|
0x6f, 0x74, 0x61, 0x6c, 0x12, 0x38, 0x0a, 0x09, 0x74, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d,
|
|
0x70, 0x18, 0x06, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65,
|
|
0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74,
|
|
0x61, 0x6d, 0x70, 0x52, 0x09, 0x74, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x12, 0x34,
|
|
0x0a, 0x07, 0x73, 0x74, 0x61, 0x72, 0x74, 0x65, 0x64, 0x18, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32,
|
|
0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75,
|
|
0x66, 0x2e, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x52, 0x07, 0x73, 0x74, 0x61,
|
|
0x72, 0x74, 0x65, 0x64, 0x12, 0x38, 0x0a, 0x09, 0x63, 0x6f, 0x6d, 0x70, 0x6c, 0x65, 0x74, 0x65,
|
|
0x64, 0x18, 0x08, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65,
|
|
0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74,
|
|
0x61, 0x6d, 0x70, 0x52, 0x09, 0x63, 0x6f, 0x6d, 0x70, 0x6c, 0x65, 0x74, 0x65, 0x64, 0x22, 0x87,
|
|
0x01, 0x0a, 0x09, 0x56, 0x65, 0x72, 0x74, 0x65, 0x78, 0x4c, 0x6f, 0x67, 0x12, 0x16, 0x0a, 0x06,
|
|
0x76, 0x65, 0x72, 0x74, 0x65, 0x78, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x06, 0x76, 0x65,
|
|
0x72, 0x74, 0x65, 0x78, 0x12, 0x38, 0x0a, 0x09, 0x74, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d,
|
|
0x70, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65,
|
|
0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74,
|
|
0x61, 0x6d, 0x70, 0x52, 0x09, 0x74, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x12, 0x16,
|
|
0x0a, 0x06, 0x73, 0x74, 0x72, 0x65, 0x61, 0x6d, 0x18, 0x03, 0x20, 0x01, 0x28, 0x03, 0x52, 0x06,
|
|
0x73, 0x74, 0x72, 0x65, 0x61, 0x6d, 0x12, 0x10, 0x0a, 0x03, 0x6d, 0x73, 0x67, 0x18, 0x04, 0x20,
|
|
0x01, 0x28, 0x0c, 0x52, 0x03, 0x6d, 0x73, 0x67, 0x22, 0xc4, 0x01, 0x0a, 0x0d, 0x56, 0x65, 0x72,
|
|
0x74, 0x65, 0x78, 0x57, 0x61, 0x72, 0x6e, 0x69, 0x6e, 0x67, 0x12, 0x16, 0x0a, 0x06, 0x76, 0x65,
|
|
0x72, 0x74, 0x65, 0x78, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x06, 0x76, 0x65, 0x72, 0x74,
|
|
0x65, 0x78, 0x12, 0x14, 0x0a, 0x05, 0x6c, 0x65, 0x76, 0x65, 0x6c, 0x18, 0x02, 0x20, 0x01, 0x28,
|
|
0x03, 0x52, 0x05, 0x6c, 0x65, 0x76, 0x65, 0x6c, 0x12, 0x14, 0x0a, 0x05, 0x73, 0x68, 0x6f, 0x72,
|
|
0x74, 0x18, 0x03, 0x20, 0x01, 0x28, 0x0c, 0x52, 0x05, 0x73, 0x68, 0x6f, 0x72, 0x74, 0x12, 0x16,
|
|
0x0a, 0x06, 0x64, 0x65, 0x74, 0x61, 0x69, 0x6c, 0x18, 0x04, 0x20, 0x03, 0x28, 0x0c, 0x52, 0x06,
|
|
0x64, 0x65, 0x74, 0x61, 0x69, 0x6c, 0x12, 0x10, 0x0a, 0x03, 0x75, 0x72, 0x6c, 0x18, 0x05, 0x20,
|
|
0x01, 0x28, 0x09, 0x52, 0x03, 0x75, 0x72, 0x6c, 0x12, 0x22, 0x0a, 0x04, 0x69, 0x6e, 0x66, 0x6f,
|
|
0x18, 0x06, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x0e, 0x2e, 0x70, 0x62, 0x2e, 0x53, 0x6f, 0x75, 0x72,
|
|
0x63, 0x65, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x04, 0x69, 0x6e, 0x66, 0x6f, 0x12, 0x21, 0x0a, 0x06,
|
|
0x72, 0x61, 0x6e, 0x67, 0x65, 0x73, 0x18, 0x07, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x09, 0x2e, 0x70,
|
|
0x62, 0x2e, 0x52, 0x61, 0x6e, 0x67, 0x65, 0x52, 0x06, 0x72, 0x61, 0x6e, 0x67, 0x65, 0x73, 0x22,
|
|
0x22, 0x0a, 0x0c, 0x42, 0x79, 0x74, 0x65, 0x73, 0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x12,
|
|
0x12, 0x0a, 0x04, 0x64, 0x61, 0x74, 0x61, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0c, 0x52, 0x04, 0x64,
|
|
0x61, 0x74, 0x61, 0x22, 0x2c, 0x0a, 0x12, 0x4c, 0x69, 0x73, 0x74, 0x57, 0x6f, 0x72, 0x6b, 0x65,
|
|
0x72, 0x73, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x12, 0x16, 0x0a, 0x06, 0x66, 0x69, 0x6c,
|
|
0x74, 0x65, 0x72, 0x18, 0x01, 0x20, 0x03, 0x28, 0x09, 0x52, 0x06, 0x66, 0x69, 0x6c, 0x74, 0x65,
|
|
0x72, 0x22, 0x53, 0x0a, 0x13, 0x4c, 0x69, 0x73, 0x74, 0x57, 0x6f, 0x72, 0x6b, 0x65, 0x72, 0x73,
|
|
0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x3c, 0x0a, 0x06, 0x72, 0x65, 0x63, 0x6f,
|
|
0x72, 0x64, 0x18, 0x01, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x24, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e,
|
|
0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x74, 0x79, 0x70, 0x65,
|
|
0x73, 0x2e, 0x57, 0x6f, 0x72, 0x6b, 0x65, 0x72, 0x52, 0x65, 0x63, 0x6f, 0x72, 0x64, 0x52, 0x06,
|
|
0x72, 0x65, 0x63, 0x6f, 0x72, 0x64, 0x22, 0x0d, 0x0a, 0x0b, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65,
|
|
0x71, 0x75, 0x65, 0x73, 0x74, 0x22, 0x61, 0x0a, 0x0c, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x73,
|
|
0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x51, 0x0a, 0x0f, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69,
|
|
0x74, 0x56, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x27,
|
|
0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76,
|
|
0x31, 0x2e, 0x74, 0x79, 0x70, 0x65, 0x73, 0x2e, 0x42, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74,
|
|
0x56, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x52, 0x0f, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69,
|
|
0x74, 0x56, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x22, 0x65, 0x0a, 0x13, 0x42, 0x75, 0x69, 0x6c,
|
|
0x64, 0x48, 0x69, 0x73, 0x74, 0x6f, 0x72, 0x79, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x12,
|
|
0x1e, 0x0a, 0x0a, 0x41, 0x63, 0x74, 0x69, 0x76, 0x65, 0x4f, 0x6e, 0x6c, 0x79, 0x18, 0x01, 0x20,
|
|
0x01, 0x28, 0x08, 0x52, 0x0a, 0x41, 0x63, 0x74, 0x69, 0x76, 0x65, 0x4f, 0x6e, 0x6c, 0x79, 0x12,
|
|
0x10, 0x0a, 0x03, 0x52, 0x65, 0x66, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x03, 0x52, 0x65,
|
|
0x66, 0x12, 0x1c, 0x0a, 0x09, 0x45, 0x61, 0x72, 0x6c, 0x79, 0x45, 0x78, 0x69, 0x74, 0x18, 0x03,
|
|
0x20, 0x01, 0x28, 0x08, 0x52, 0x09, 0x45, 0x61, 0x72, 0x6c, 0x79, 0x45, 0x78, 0x69, 0x74, 0x22,
|
|
0x8e, 0x01, 0x0a, 0x11, 0x42, 0x75, 0x69, 0x6c, 0x64, 0x48, 0x69, 0x73, 0x74, 0x6f, 0x72, 0x79,
|
|
0x45, 0x76, 0x65, 0x6e, 0x74, 0x12, 0x3b, 0x0a, 0x04, 0x74, 0x79, 0x70, 0x65, 0x18, 0x01, 0x20,
|
|
0x01, 0x28, 0x0e, 0x32, 0x27, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64,
|
|
0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x42, 0x75, 0x69, 0x6c, 0x64, 0x48, 0x69, 0x73, 0x74,
|
|
0x6f, 0x72, 0x79, 0x45, 0x76, 0x65, 0x6e, 0x74, 0x54, 0x79, 0x70, 0x65, 0x52, 0x04, 0x74, 0x79,
|
|
0x70, 0x65, 0x12, 0x3c, 0x0a, 0x06, 0x72, 0x65, 0x63, 0x6f, 0x72, 0x64, 0x18, 0x02, 0x20, 0x01,
|
|
0x28, 0x0b, 0x32, 0x24, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b,
|
|
0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x42, 0x75, 0x69, 0x6c, 0x64, 0x48, 0x69, 0x73, 0x74, 0x6f,
|
|
0x72, 0x79, 0x52, 0x65, 0x63, 0x6f, 0x72, 0x64, 0x52, 0x06, 0x72, 0x65, 0x63, 0x6f, 0x72, 0x64,
|
|
0x22, 0xd3, 0x09, 0x0a, 0x12, 0x42, 0x75, 0x69, 0x6c, 0x64, 0x48, 0x69, 0x73, 0x74, 0x6f, 0x72,
|
|
0x79, 0x52, 0x65, 0x63, 0x6f, 0x72, 0x64, 0x12, 0x10, 0x0a, 0x03, 0x52, 0x65, 0x66, 0x18, 0x01,
|
|
0x20, 0x01, 0x28, 0x09, 0x52, 0x03, 0x52, 0x65, 0x66, 0x12, 0x1a, 0x0a, 0x08, 0x46, 0x72, 0x6f,
|
|
0x6e, 0x74, 0x65, 0x6e, 0x64, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x08, 0x46, 0x72, 0x6f,
|
|
0x6e, 0x74, 0x65, 0x6e, 0x64, 0x12, 0x5d, 0x0a, 0x0d, 0x46, 0x72, 0x6f, 0x6e, 0x74, 0x65, 0x6e,
|
|
0x64, 0x41, 0x74, 0x74, 0x72, 0x73, 0x18, 0x03, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x37, 0x2e, 0x6d,
|
|
0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e,
|
|
0x42, 0x75, 0x69, 0x6c, 0x64, 0x48, 0x69, 0x73, 0x74, 0x6f, 0x72, 0x79, 0x52, 0x65, 0x63, 0x6f,
|
|
0x72, 0x64, 0x2e, 0x46, 0x72, 0x6f, 0x6e, 0x74, 0x65, 0x6e, 0x64, 0x41, 0x74, 0x74, 0x72, 0x73,
|
|
0x45, 0x6e, 0x74, 0x72, 0x79, 0x52, 0x0d, 0x46, 0x72, 0x6f, 0x6e, 0x74, 0x65, 0x6e, 0x64, 0x41,
|
|
0x74, 0x74, 0x72, 0x73, 0x12, 0x38, 0x0a, 0x09, 0x45, 0x78, 0x70, 0x6f, 0x72, 0x74, 0x65, 0x72,
|
|
0x73, 0x18, 0x04, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62,
|
|
0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x45, 0x78, 0x70, 0x6f, 0x72,
|
|
0x74, 0x65, 0x72, 0x52, 0x09, 0x45, 0x78, 0x70, 0x6f, 0x72, 0x74, 0x65, 0x72, 0x73, 0x12, 0x28,
|
|
0x0a, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x18, 0x05, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x12, 0x2e,
|
|
0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x72, 0x70, 0x63, 0x2e, 0x53, 0x74, 0x61, 0x74, 0x75,
|
|
0x73, 0x52, 0x05, 0x65, 0x72, 0x72, 0x6f, 0x72, 0x12, 0x38, 0x0a, 0x09, 0x43, 0x72, 0x65, 0x61,
|
|
0x74, 0x65, 0x64, 0x41, 0x74, 0x18, 0x06, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x67, 0x6f,
|
|
0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x54, 0x69,
|
|
0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d, 0x70, 0x52, 0x09, 0x43, 0x72, 0x65, 0x61, 0x74, 0x65, 0x64,
|
|
0x41, 0x74, 0x12, 0x3c, 0x0a, 0x0b, 0x43, 0x6f, 0x6d, 0x70, 0x6c, 0x65, 0x74, 0x65, 0x64, 0x41,
|
|
0x74, 0x18, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1a, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65,
|
|
0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62, 0x75, 0x66, 0x2e, 0x54, 0x69, 0x6d, 0x65, 0x73, 0x74,
|
|
0x61, 0x6d, 0x70, 0x52, 0x0b, 0x43, 0x6f, 0x6d, 0x70, 0x6c, 0x65, 0x74, 0x65, 0x64, 0x41, 0x74,
|
|
0x12, 0x30, 0x0a, 0x04, 0x6c, 0x6f, 0x67, 0x73, 0x18, 0x08, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1c,
|
|
0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76,
|
|
0x31, 0x2e, 0x44, 0x65, 0x73, 0x63, 0x72, 0x69, 0x70, 0x74, 0x6f, 0x72, 0x52, 0x04, 0x6c, 0x6f,
|
|
0x67, 0x73, 0x12, 0x66, 0x0a, 0x10, 0x45, 0x78, 0x70, 0x6f, 0x72, 0x74, 0x65, 0x72, 0x52, 0x65,
|
|
0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x18, 0x09, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x3a, 0x2e, 0x6d,
|
|
0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e,
|
|
0x42, 0x75, 0x69, 0x6c, 0x64, 0x48, 0x69, 0x73, 0x74, 0x6f, 0x72, 0x79, 0x52, 0x65, 0x63, 0x6f,
|
|
0x72, 0x64, 0x2e, 0x45, 0x78, 0x70, 0x6f, 0x72, 0x74, 0x65, 0x72, 0x52, 0x65, 0x73, 0x70, 0x6f,
|
|
0x6e, 0x73, 0x65, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x52, 0x10, 0x45, 0x78, 0x70, 0x6f, 0x72, 0x74,
|
|
0x65, 0x72, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x39, 0x0a, 0x06, 0x52, 0x65,
|
|
0x73, 0x75, 0x6c, 0x74, 0x18, 0x0a, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x21, 0x2e, 0x6d, 0x6f, 0x62,
|
|
0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x42, 0x75,
|
|
0x69, 0x6c, 0x64, 0x52, 0x65, 0x73, 0x75, 0x6c, 0x74, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x06, 0x52,
|
|
0x65, 0x73, 0x75, 0x6c, 0x74, 0x12, 0x4b, 0x0a, 0x07, 0x52, 0x65, 0x73, 0x75, 0x6c, 0x74, 0x73,
|
|
0x18, 0x0b, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x31, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75,
|
|
0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x42, 0x75, 0x69, 0x6c, 0x64, 0x48,
|
|
0x69, 0x73, 0x74, 0x6f, 0x72, 0x79, 0x52, 0x65, 0x63, 0x6f, 0x72, 0x64, 0x2e, 0x52, 0x65, 0x73,
|
|
0x75, 0x6c, 0x74, 0x73, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x52, 0x07, 0x52, 0x65, 0x73, 0x75, 0x6c,
|
|
0x74, 0x73, 0x12, 0x1e, 0x0a, 0x0a, 0x47, 0x65, 0x6e, 0x65, 0x72, 0x61, 0x74, 0x69, 0x6f, 0x6e,
|
|
0x18, 0x0c, 0x20, 0x01, 0x28, 0x05, 0x52, 0x0a, 0x47, 0x65, 0x6e, 0x65, 0x72, 0x61, 0x74, 0x69,
|
|
0x6f, 0x6e, 0x12, 0x32, 0x0a, 0x05, 0x74, 0x72, 0x61, 0x63, 0x65, 0x18, 0x0d, 0x20, 0x01, 0x28,
|
|
0x0b, 0x32, 0x1c, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69,
|
|
0x74, 0x2e, 0x76, 0x31, 0x2e, 0x44, 0x65, 0x73, 0x63, 0x72, 0x69, 0x70, 0x74, 0x6f, 0x72, 0x52,
|
|
0x05, 0x74, 0x72, 0x61, 0x63, 0x65, 0x12, 0x16, 0x0a, 0x06, 0x70, 0x69, 0x6e, 0x6e, 0x65, 0x64,
|
|
0x18, 0x0e, 0x20, 0x01, 0x28, 0x08, 0x52, 0x06, 0x70, 0x69, 0x6e, 0x6e, 0x65, 0x64, 0x12, 0x26,
|
|
0x0a, 0x0e, 0x6e, 0x75, 0x6d, 0x43, 0x61, 0x63, 0x68, 0x65, 0x64, 0x53, 0x74, 0x65, 0x70, 0x73,
|
|
0x18, 0x0f, 0x20, 0x01, 0x28, 0x05, 0x52, 0x0e, 0x6e, 0x75, 0x6d, 0x43, 0x61, 0x63, 0x68, 0x65,
|
|
0x64, 0x53, 0x74, 0x65, 0x70, 0x73, 0x12, 0x24, 0x0a, 0x0d, 0x6e, 0x75, 0x6d, 0x54, 0x6f, 0x74,
|
|
0x61, 0x6c, 0x53, 0x74, 0x65, 0x70, 0x73, 0x18, 0x10, 0x20, 0x01, 0x28, 0x05, 0x52, 0x0d, 0x6e,
|
|
0x75, 0x6d, 0x54, 0x6f, 0x74, 0x61, 0x6c, 0x53, 0x74, 0x65, 0x70, 0x73, 0x12, 0x2c, 0x0a, 0x11,
|
|
0x6e, 0x75, 0x6d, 0x43, 0x6f, 0x6d, 0x70, 0x6c, 0x65, 0x74, 0x65, 0x64, 0x53, 0x74, 0x65, 0x70,
|
|
0x73, 0x18, 0x11, 0x20, 0x01, 0x28, 0x05, 0x52, 0x11, 0x6e, 0x75, 0x6d, 0x43, 0x6f, 0x6d, 0x70,
|
|
0x6c, 0x65, 0x74, 0x65, 0x64, 0x53, 0x74, 0x65, 0x70, 0x73, 0x12, 0x42, 0x0a, 0x0d, 0x65, 0x78,
|
|
0x74, 0x65, 0x72, 0x6e, 0x61, 0x6c, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x18, 0x12, 0x20, 0x01, 0x28,
|
|
0x0b, 0x32, 0x1c, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69,
|
|
0x74, 0x2e, 0x76, 0x31, 0x2e, 0x44, 0x65, 0x73, 0x63, 0x72, 0x69, 0x70, 0x74, 0x6f, 0x72, 0x52,
|
|
0x0d, 0x65, 0x78, 0x74, 0x65, 0x72, 0x6e, 0x61, 0x6c, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x12, 0x20,
|
|
0x0a, 0x0b, 0x6e, 0x75, 0x6d, 0x57, 0x61, 0x72, 0x6e, 0x69, 0x6e, 0x67, 0x73, 0x18, 0x13, 0x20,
|
|
0x01, 0x28, 0x05, 0x52, 0x0b, 0x6e, 0x75, 0x6d, 0x57, 0x61, 0x72, 0x6e, 0x69, 0x6e, 0x67, 0x73,
|
|
0x1a, 0x40, 0x0a, 0x12, 0x46, 0x72, 0x6f, 0x6e, 0x74, 0x65, 0x6e, 0x64, 0x41, 0x74, 0x74, 0x72,
|
|
0x73, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x12, 0x10, 0x0a, 0x03, 0x6b, 0x65, 0x79, 0x18, 0x01, 0x20,
|
|
0x01, 0x28, 0x09, 0x52, 0x03, 0x6b, 0x65, 0x79, 0x12, 0x14, 0x0a, 0x05, 0x76, 0x61, 0x6c, 0x75,
|
|
0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x3a, 0x02,
|
|
0x38, 0x01, 0x1a, 0x43, 0x0a, 0x15, 0x45, 0x78, 0x70, 0x6f, 0x72, 0x74, 0x65, 0x72, 0x52, 0x65,
|
|
0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x12, 0x10, 0x0a, 0x03, 0x6b,
|
|
0x65, 0x79, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x03, 0x6b, 0x65, 0x79, 0x12, 0x14, 0x0a,
|
|
0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x05, 0x76, 0x61,
|
|
0x6c, 0x75, 0x65, 0x3a, 0x02, 0x38, 0x01, 0x1a, 0x5d, 0x0a, 0x0c, 0x52, 0x65, 0x73, 0x75, 0x6c,
|
|
0x74, 0x73, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x12, 0x10, 0x0a, 0x03, 0x6b, 0x65, 0x79, 0x18, 0x01,
|
|
0x20, 0x01, 0x28, 0x09, 0x52, 0x03, 0x6b, 0x65, 0x79, 0x12, 0x37, 0x0a, 0x05, 0x76, 0x61, 0x6c,
|
|
0x75, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x21, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e,
|
|
0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x42, 0x75, 0x69, 0x6c,
|
|
0x64, 0x52, 0x65, 0x73, 0x75, 0x6c, 0x74, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x05, 0x76, 0x61, 0x6c,
|
|
0x75, 0x65, 0x3a, 0x02, 0x38, 0x01, 0x22, 0x79, 0x0a, 0x19, 0x55, 0x70, 0x64, 0x61, 0x74, 0x65,
|
|
0x42, 0x75, 0x69, 0x6c, 0x64, 0x48, 0x69, 0x73, 0x74, 0x6f, 0x72, 0x79, 0x52, 0x65, 0x71, 0x75,
|
|
0x65, 0x73, 0x74, 0x12, 0x10, 0x0a, 0x03, 0x52, 0x65, 0x66, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09,
|
|
0x52, 0x03, 0x52, 0x65, 0x66, 0x12, 0x16, 0x0a, 0x06, 0x50, 0x69, 0x6e, 0x6e, 0x65, 0x64, 0x18,
|
|
0x02, 0x20, 0x01, 0x28, 0x08, 0x52, 0x06, 0x50, 0x69, 0x6e, 0x6e, 0x65, 0x64, 0x12, 0x16, 0x0a,
|
|
0x06, 0x44, 0x65, 0x6c, 0x65, 0x74, 0x65, 0x18, 0x03, 0x20, 0x01, 0x28, 0x08, 0x52, 0x06, 0x44,
|
|
0x65, 0x6c, 0x65, 0x74, 0x65, 0x12, 0x1a, 0x0a, 0x08, 0x46, 0x69, 0x6e, 0x61, 0x6c, 0x69, 0x7a,
|
|
0x65, 0x18, 0x04, 0x20, 0x01, 0x28, 0x08, 0x52, 0x08, 0x46, 0x69, 0x6e, 0x61, 0x6c, 0x69, 0x7a,
|
|
0x65, 0x22, 0x1c, 0x0a, 0x1a, 0x55, 0x70, 0x64, 0x61, 0x74, 0x65, 0x42, 0x75, 0x69, 0x6c, 0x64,
|
|
0x48, 0x69, 0x73, 0x74, 0x6f, 0x72, 0x79, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x22,
|
|
0xe8, 0x01, 0x0a, 0x0a, 0x44, 0x65, 0x73, 0x63, 0x72, 0x69, 0x70, 0x74, 0x6f, 0x72, 0x12, 0x1d,
|
|
0x0a, 0x0a, 0x6d, 0x65, 0x64, 0x69, 0x61, 0x5f, 0x74, 0x79, 0x70, 0x65, 0x18, 0x01, 0x20, 0x01,
|
|
0x28, 0x09, 0x52, 0x09, 0x6d, 0x65, 0x64, 0x69, 0x61, 0x54, 0x79, 0x70, 0x65, 0x12, 0x16, 0x0a,
|
|
0x06, 0x64, 0x69, 0x67, 0x65, 0x73, 0x74, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x06, 0x64,
|
|
0x69, 0x67, 0x65, 0x73, 0x74, 0x12, 0x12, 0x0a, 0x04, 0x73, 0x69, 0x7a, 0x65, 0x18, 0x03, 0x20,
|
|
0x01, 0x28, 0x03, 0x52, 0x04, 0x73, 0x69, 0x7a, 0x65, 0x12, 0x4f, 0x0a, 0x0b, 0x61, 0x6e, 0x6e,
|
|
0x6f, 0x74, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x18, 0x05, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x2d,
|
|
0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76,
|
|
0x31, 0x2e, 0x44, 0x65, 0x73, 0x63, 0x72, 0x69, 0x70, 0x74, 0x6f, 0x72, 0x2e, 0x41, 0x6e, 0x6e,
|
|
0x6f, 0x74, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x52, 0x0b, 0x61,
|
|
0x6e, 0x6e, 0x6f, 0x74, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x1a, 0x3e, 0x0a, 0x10, 0x41, 0x6e,
|
|
0x6e, 0x6f, 0x74, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x12, 0x10,
|
|
0x0a, 0x03, 0x6b, 0x65, 0x79, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x03, 0x6b, 0x65, 0x79,
|
|
0x12, 0x14, 0x0a, 0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52,
|
|
0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x3a, 0x02, 0x38, 0x01, 0x22, 0xc1, 0x02, 0x0a, 0x0f, 0x42,
|
|
0x75, 0x69, 0x6c, 0x64, 0x52, 0x65, 0x73, 0x75, 0x6c, 0x74, 0x49, 0x6e, 0x66, 0x6f, 0x12, 0x48,
|
|
0x0a, 0x10, 0x52, 0x65, 0x73, 0x75, 0x6c, 0x74, 0x44, 0x65, 0x70, 0x72, 0x65, 0x63, 0x61, 0x74,
|
|
0x65, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1c, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e,
|
|
0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x44, 0x65, 0x73, 0x63,
|
|
0x72, 0x69, 0x70, 0x74, 0x6f, 0x72, 0x52, 0x10, 0x52, 0x65, 0x73, 0x75, 0x6c, 0x74, 0x44, 0x65,
|
|
0x70, 0x72, 0x65, 0x63, 0x61, 0x74, 0x65, 0x64, 0x12, 0x40, 0x0a, 0x0c, 0x41, 0x74, 0x74, 0x65,
|
|
0x73, 0x74, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x18, 0x02, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x1c,
|
|
0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76,
|
|
0x31, 0x2e, 0x44, 0x65, 0x73, 0x63, 0x72, 0x69, 0x70, 0x74, 0x6f, 0x72, 0x52, 0x0c, 0x41, 0x74,
|
|
0x74, 0x65, 0x73, 0x74, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x73, 0x12, 0x48, 0x0a, 0x07, 0x52, 0x65,
|
|
0x73, 0x75, 0x6c, 0x74, 0x73, 0x18, 0x03, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x2e, 0x2e, 0x6d, 0x6f,
|
|
0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x42,
|
|
0x75, 0x69, 0x6c, 0x64, 0x52, 0x65, 0x73, 0x75, 0x6c, 0x74, 0x49, 0x6e, 0x66, 0x6f, 0x2e, 0x52,
|
|
0x65, 0x73, 0x75, 0x6c, 0x74, 0x73, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x52, 0x07, 0x52, 0x65, 0x73,
|
|
0x75, 0x6c, 0x74, 0x73, 0x1a, 0x58, 0x0a, 0x0c, 0x52, 0x65, 0x73, 0x75, 0x6c, 0x74, 0x73, 0x45,
|
|
0x6e, 0x74, 0x72, 0x79, 0x12, 0x10, 0x0a, 0x03, 0x6b, 0x65, 0x79, 0x18, 0x01, 0x20, 0x01, 0x28,
|
|
0x03, 0x52, 0x03, 0x6b, 0x65, 0x79, 0x12, 0x32, 0x0a, 0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x18,
|
|
0x02, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1c, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69,
|
|
0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x44, 0x65, 0x73, 0x63, 0x72, 0x69, 0x70,
|
|
0x74, 0x6f, 0x72, 0x52, 0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x3a, 0x02, 0x38, 0x01, 0x22, 0x95,
|
|
0x01, 0x0a, 0x08, 0x45, 0x78, 0x70, 0x6f, 0x72, 0x74, 0x65, 0x72, 0x12, 0x12, 0x0a, 0x04, 0x54,
|
|
0x79, 0x70, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x04, 0x54, 0x79, 0x70, 0x65, 0x12,
|
|
0x3b, 0x0a, 0x05, 0x41, 0x74, 0x74, 0x72, 0x73, 0x18, 0x02, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x25,
|
|
0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76,
|
|
0x31, 0x2e, 0x45, 0x78, 0x70, 0x6f, 0x72, 0x74, 0x65, 0x72, 0x2e, 0x41, 0x74, 0x74, 0x72, 0x73,
|
|
0x45, 0x6e, 0x74, 0x72, 0x79, 0x52, 0x05, 0x41, 0x74, 0x74, 0x72, 0x73, 0x1a, 0x38, 0x0a, 0x0a,
|
|
0x41, 0x74, 0x74, 0x72, 0x73, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x12, 0x10, 0x0a, 0x03, 0x6b, 0x65,
|
|
0x79, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x03, 0x6b, 0x65, 0x79, 0x12, 0x14, 0x0a, 0x05,
|
|
0x76, 0x61, 0x6c, 0x75, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x05, 0x76, 0x61, 0x6c,
|
|
0x75, 0x65, 0x3a, 0x02, 0x38, 0x01, 0x2a, 0x3f, 0x0a, 0x15, 0x42, 0x75, 0x69, 0x6c, 0x64, 0x48,
|
|
0x69, 0x73, 0x74, 0x6f, 0x72, 0x79, 0x45, 0x76, 0x65, 0x6e, 0x74, 0x54, 0x79, 0x70, 0x65, 0x12,
|
|
0x0b, 0x0a, 0x07, 0x53, 0x54, 0x41, 0x52, 0x54, 0x45, 0x44, 0x10, 0x00, 0x12, 0x0c, 0x0a, 0x08,
|
|
0x43, 0x4f, 0x4d, 0x50, 0x4c, 0x45, 0x54, 0x45, 0x10, 0x01, 0x12, 0x0b, 0x0a, 0x07, 0x44, 0x45,
|
|
0x4c, 0x45, 0x54, 0x45, 0x44, 0x10, 0x02, 0x32, 0x89, 0x06, 0x0a, 0x07, 0x43, 0x6f, 0x6e, 0x74,
|
|
0x72, 0x6f, 0x6c, 0x12, 0x54, 0x0a, 0x09, 0x44, 0x69, 0x73, 0x6b, 0x55, 0x73, 0x61, 0x67, 0x65,
|
|
0x12, 0x22, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74,
|
|
0x2e, 0x76, 0x31, 0x2e, 0x44, 0x69, 0x73, 0x6b, 0x55, 0x73, 0x61, 0x67, 0x65, 0x52, 0x65, 0x71,
|
|
0x75, 0x65, 0x73, 0x74, 0x1a, 0x23, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c,
|
|
0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x44, 0x69, 0x73, 0x6b, 0x55, 0x73, 0x61, 0x67,
|
|
0x65, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x48, 0x0a, 0x05, 0x50, 0x72, 0x75,
|
|
0x6e, 0x65, 0x12, 0x1e, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b,
|
|
0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x50, 0x72, 0x75, 0x6e, 0x65, 0x52, 0x65, 0x71, 0x75, 0x65,
|
|
0x73, 0x74, 0x1a, 0x1d, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b,
|
|
0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x55, 0x73, 0x61, 0x67, 0x65, 0x52, 0x65, 0x63, 0x6f, 0x72,
|
|
0x64, 0x30, 0x01, 0x12, 0x48, 0x0a, 0x05, 0x53, 0x6f, 0x6c, 0x76, 0x65, 0x12, 0x1e, 0x2e, 0x6d,
|
|
0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e,
|
|
0x53, 0x6f, 0x6c, 0x76, 0x65, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x1f, 0x2e, 0x6d,
|
|
0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e,
|
|
0x53, 0x6f, 0x6c, 0x76, 0x65, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x4d, 0x0a,
|
|
0x06, 0x53, 0x74, 0x61, 0x74, 0x75, 0x73, 0x12, 0x1f, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62,
|
|
0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x53, 0x74, 0x61, 0x74, 0x75,
|
|
0x73, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x20, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e,
|
|
0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x53, 0x74, 0x61, 0x74,
|
|
0x75, 0x73, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x30, 0x01, 0x12, 0x4d, 0x0a, 0x07,
|
|
0x53, 0x65, 0x73, 0x73, 0x69, 0x6f, 0x6e, 0x12, 0x1e, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62,
|
|
0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x42, 0x79, 0x74, 0x65, 0x73,
|
|
0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x1a, 0x1e, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62,
|
|
0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x42, 0x79, 0x74, 0x65, 0x73,
|
|
0x4d, 0x65, 0x73, 0x73, 0x61, 0x67, 0x65, 0x28, 0x01, 0x30, 0x01, 0x12, 0x5a, 0x0a, 0x0b, 0x4c,
|
|
0x69, 0x73, 0x74, 0x57, 0x6f, 0x72, 0x6b, 0x65, 0x72, 0x73, 0x12, 0x24, 0x2e, 0x6d, 0x6f, 0x62,
|
|
0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x4c, 0x69,
|
|
0x73, 0x74, 0x57, 0x6f, 0x72, 0x6b, 0x65, 0x72, 0x73, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74,
|
|
0x1a, 0x25, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74,
|
|
0x2e, 0x76, 0x31, 0x2e, 0x4c, 0x69, 0x73, 0x74, 0x57, 0x6f, 0x72, 0x6b, 0x65, 0x72, 0x73, 0x52,
|
|
0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x45, 0x0a, 0x04, 0x49, 0x6e, 0x66, 0x6f, 0x12,
|
|
0x1d, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e,
|
|
0x76, 0x31, 0x2e, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x1e,
|
|
0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76,
|
|
0x31, 0x2e, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x73, 0x70, 0x6f, 0x6e, 0x73, 0x65, 0x12, 0x62,
|
|
0x0a, 0x12, 0x4c, 0x69, 0x73, 0x74, 0x65, 0x6e, 0x42, 0x75, 0x69, 0x6c, 0x64, 0x48, 0x69, 0x73,
|
|
0x74, 0x6f, 0x72, 0x79, 0x12, 0x25, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c,
|
|
0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x42, 0x75, 0x69, 0x6c, 0x64, 0x48, 0x69, 0x73,
|
|
0x74, 0x6f, 0x72, 0x79, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x23, 0x2e, 0x6d, 0x6f,
|
|
0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x42,
|
|
0x75, 0x69, 0x6c, 0x64, 0x48, 0x69, 0x73, 0x74, 0x6f, 0x72, 0x79, 0x45, 0x76, 0x65, 0x6e, 0x74,
|
|
0x30, 0x01, 0x12, 0x6f, 0x0a, 0x12, 0x55, 0x70, 0x64, 0x61, 0x74, 0x65, 0x42, 0x75, 0x69, 0x6c,
|
|
0x64, 0x48, 0x69, 0x73, 0x74, 0x6f, 0x72, 0x79, 0x12, 0x2b, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e,
|
|
0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x55, 0x70, 0x64, 0x61,
|
|
0x74, 0x65, 0x42, 0x75, 0x69, 0x6c, 0x64, 0x48, 0x69, 0x73, 0x74, 0x6f, 0x72, 0x79, 0x52, 0x65,
|
|
0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x2c, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69,
|
|
0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x55, 0x70, 0x64, 0x61, 0x74, 0x65, 0x42,
|
|
0x75, 0x69, 0x6c, 0x64, 0x48, 0x69, 0x73, 0x74, 0x6f, 0x72, 0x79, 0x52, 0x65, 0x73, 0x70, 0x6f,
|
|
0x6e, 0x73, 0x65, 0x42, 0x40, 0x5a, 0x3e, 0x67, 0x69, 0x74, 0x68, 0x75, 0x62, 0x2e, 0x63, 0x6f,
|
|
0x6d, 0x2f, 0x6d, 0x6f, 0x62, 0x79, 0x2f, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2f,
|
|
0x61, 0x70, 0x69, 0x2f, 0x73, 0x65, 0x72, 0x76, 0x69, 0x63, 0x65, 0x73, 0x2f, 0x63, 0x6f, 0x6e,
|
|
0x74, 0x72, 0x6f, 0x6c, 0x3b, 0x6d, 0x6f, 0x62, 0x79, 0x5f, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b,
|
|
0x69, 0x74, 0x5f, 0x76, 0x31, 0x62, 0x06, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x33,
|
|
}
|
|
|
|
var (
|
|
file_control_proto_rawDescOnce sync.Once
|
|
file_control_proto_rawDescData = file_control_proto_rawDesc
|
|
)
|
|
|
|
func file_control_proto_rawDescGZIP() []byte {
|
|
file_control_proto_rawDescOnce.Do(func() {
|
|
file_control_proto_rawDescData = protoimpl.X.CompressGZIP(file_control_proto_rawDescData)
|
|
})
|
|
return file_control_proto_rawDescData
|
|
}
|
|
|
|
var file_control_proto_enumTypes = make([]protoimpl.EnumInfo, 1)
|
|
var file_control_proto_msgTypes = make([]protoimpl.MessageInfo, 39)
|
|
var file_control_proto_goTypes = []interface{}{
|
|
(BuildHistoryEventType)(0), // 0: moby.buildkit.v1.BuildHistoryEventType
|
|
(*PruneRequest)(nil), // 1: moby.buildkit.v1.PruneRequest
|
|
(*DiskUsageRequest)(nil), // 2: moby.buildkit.v1.DiskUsageRequest
|
|
(*DiskUsageResponse)(nil), // 3: moby.buildkit.v1.DiskUsageResponse
|
|
(*UsageRecord)(nil), // 4: moby.buildkit.v1.UsageRecord
|
|
(*SolveRequest)(nil), // 5: moby.buildkit.v1.SolveRequest
|
|
(*CacheOptions)(nil), // 6: moby.buildkit.v1.CacheOptions
|
|
(*CacheOptionsEntry)(nil), // 7: moby.buildkit.v1.CacheOptionsEntry
|
|
(*SolveResponse)(nil), // 8: moby.buildkit.v1.SolveResponse
|
|
(*StatusRequest)(nil), // 9: moby.buildkit.v1.StatusRequest
|
|
(*StatusResponse)(nil), // 10: moby.buildkit.v1.StatusResponse
|
|
(*Vertex)(nil), // 11: moby.buildkit.v1.Vertex
|
|
(*VertexStatus)(nil), // 12: moby.buildkit.v1.VertexStatus
|
|
(*VertexLog)(nil), // 13: moby.buildkit.v1.VertexLog
|
|
(*VertexWarning)(nil), // 14: moby.buildkit.v1.VertexWarning
|
|
(*BytesMessage)(nil), // 15: moby.buildkit.v1.BytesMessage
|
|
(*ListWorkersRequest)(nil), // 16: moby.buildkit.v1.ListWorkersRequest
|
|
(*ListWorkersResponse)(nil), // 17: moby.buildkit.v1.ListWorkersResponse
|
|
(*InfoRequest)(nil), // 18: moby.buildkit.v1.InfoRequest
|
|
(*InfoResponse)(nil), // 19: moby.buildkit.v1.InfoResponse
|
|
(*BuildHistoryRequest)(nil), // 20: moby.buildkit.v1.BuildHistoryRequest
|
|
(*BuildHistoryEvent)(nil), // 21: moby.buildkit.v1.BuildHistoryEvent
|
|
(*BuildHistoryRecord)(nil), // 22: moby.buildkit.v1.BuildHistoryRecord
|
|
(*UpdateBuildHistoryRequest)(nil), // 23: moby.buildkit.v1.UpdateBuildHistoryRequest
|
|
(*UpdateBuildHistoryResponse)(nil), // 24: moby.buildkit.v1.UpdateBuildHistoryResponse
|
|
(*Descriptor)(nil), // 25: moby.buildkit.v1.Descriptor
|
|
(*BuildResultInfo)(nil), // 26: moby.buildkit.v1.BuildResultInfo
|
|
(*Exporter)(nil), // 27: moby.buildkit.v1.Exporter
|
|
nil, // 28: moby.buildkit.v1.SolveRequest.ExporterAttrsDeprecatedEntry
|
|
nil, // 29: moby.buildkit.v1.SolveRequest.FrontendAttrsEntry
|
|
nil, // 30: moby.buildkit.v1.SolveRequest.FrontendInputsEntry
|
|
nil, // 31: moby.buildkit.v1.CacheOptions.ExportAttrsDeprecatedEntry
|
|
nil, // 32: moby.buildkit.v1.CacheOptionsEntry.AttrsEntry
|
|
nil, // 33: moby.buildkit.v1.SolveResponse.ExporterResponseEntry
|
|
nil, // 34: moby.buildkit.v1.BuildHistoryRecord.FrontendAttrsEntry
|
|
nil, // 35: moby.buildkit.v1.BuildHistoryRecord.ExporterResponseEntry
|
|
nil, // 36: moby.buildkit.v1.BuildHistoryRecord.ResultsEntry
|
|
nil, // 37: moby.buildkit.v1.Descriptor.AnnotationsEntry
|
|
nil, // 38: moby.buildkit.v1.BuildResultInfo.ResultsEntry
|
|
nil, // 39: moby.buildkit.v1.Exporter.AttrsEntry
|
|
(*timestamp.Timestamp)(nil), // 40: google.protobuf.Timestamp
|
|
(*pb.Definition)(nil), // 41: pb.Definition
|
|
(*pb1.Policy)(nil), // 42: moby.buildkit.v1.sourcepolicy.Policy
|
|
(*pb.ProgressGroup)(nil), // 43: pb.ProgressGroup
|
|
(*pb.SourceInfo)(nil), // 44: pb.SourceInfo
|
|
(*pb.Range)(nil), // 45: pb.Range
|
|
(*types.WorkerRecord)(nil), // 46: moby.buildkit.v1.types.WorkerRecord
|
|
(*types.BuildkitVersion)(nil), // 47: moby.buildkit.v1.types.BuildkitVersion
|
|
(*status.Status)(nil), // 48: google.rpc.Status
|
|
}
|
|
var file_control_proto_depIdxs = []int32{
|
|
4, // 0: moby.buildkit.v1.DiskUsageResponse.record:type_name -> moby.buildkit.v1.UsageRecord
|
|
40, // 1: moby.buildkit.v1.UsageRecord.CreatedAt:type_name -> google.protobuf.Timestamp
|
|
40, // 2: moby.buildkit.v1.UsageRecord.LastUsedAt:type_name -> google.protobuf.Timestamp
|
|
41, // 3: moby.buildkit.v1.SolveRequest.Definition:type_name -> pb.Definition
|
|
28, // 4: moby.buildkit.v1.SolveRequest.ExporterAttrsDeprecated:type_name -> moby.buildkit.v1.SolveRequest.ExporterAttrsDeprecatedEntry
|
|
29, // 5: moby.buildkit.v1.SolveRequest.FrontendAttrs:type_name -> moby.buildkit.v1.SolveRequest.FrontendAttrsEntry
|
|
6, // 6: moby.buildkit.v1.SolveRequest.Cache:type_name -> moby.buildkit.v1.CacheOptions
|
|
30, // 7: moby.buildkit.v1.SolveRequest.FrontendInputs:type_name -> moby.buildkit.v1.SolveRequest.FrontendInputsEntry
|
|
42, // 8: moby.buildkit.v1.SolveRequest.SourcePolicy:type_name -> moby.buildkit.v1.sourcepolicy.Policy
|
|
27, // 9: moby.buildkit.v1.SolveRequest.Exporters:type_name -> moby.buildkit.v1.Exporter
|
|
31, // 10: moby.buildkit.v1.CacheOptions.ExportAttrsDeprecated:type_name -> moby.buildkit.v1.CacheOptions.ExportAttrsDeprecatedEntry
|
|
7, // 11: moby.buildkit.v1.CacheOptions.Exports:type_name -> moby.buildkit.v1.CacheOptionsEntry
|
|
7, // 12: moby.buildkit.v1.CacheOptions.Imports:type_name -> moby.buildkit.v1.CacheOptionsEntry
|
|
32, // 13: moby.buildkit.v1.CacheOptionsEntry.Attrs:type_name -> moby.buildkit.v1.CacheOptionsEntry.AttrsEntry
|
|
33, // 14: moby.buildkit.v1.SolveResponse.ExporterResponse:type_name -> moby.buildkit.v1.SolveResponse.ExporterResponseEntry
|
|
11, // 15: moby.buildkit.v1.StatusResponse.vertexes:type_name -> moby.buildkit.v1.Vertex
|
|
12, // 16: moby.buildkit.v1.StatusResponse.statuses:type_name -> moby.buildkit.v1.VertexStatus
|
|
13, // 17: moby.buildkit.v1.StatusResponse.logs:type_name -> moby.buildkit.v1.VertexLog
|
|
14, // 18: moby.buildkit.v1.StatusResponse.warnings:type_name -> moby.buildkit.v1.VertexWarning
|
|
40, // 19: moby.buildkit.v1.Vertex.started:type_name -> google.protobuf.Timestamp
|
|
40, // 20: moby.buildkit.v1.Vertex.completed:type_name -> google.protobuf.Timestamp
|
|
43, // 21: moby.buildkit.v1.Vertex.progressGroup:type_name -> pb.ProgressGroup
|
|
40, // 22: moby.buildkit.v1.VertexStatus.timestamp:type_name -> google.protobuf.Timestamp
|
|
40, // 23: moby.buildkit.v1.VertexStatus.started:type_name -> google.protobuf.Timestamp
|
|
40, // 24: moby.buildkit.v1.VertexStatus.completed:type_name -> google.protobuf.Timestamp
|
|
40, // 25: moby.buildkit.v1.VertexLog.timestamp:type_name -> google.protobuf.Timestamp
|
|
44, // 26: moby.buildkit.v1.VertexWarning.info:type_name -> pb.SourceInfo
|
|
45, // 27: moby.buildkit.v1.VertexWarning.ranges:type_name -> pb.Range
|
|
46, // 28: moby.buildkit.v1.ListWorkersResponse.record:type_name -> moby.buildkit.v1.types.WorkerRecord
|
|
47, // 29: moby.buildkit.v1.InfoResponse.buildkitVersion:type_name -> moby.buildkit.v1.types.BuildkitVersion
|
|
0, // 30: moby.buildkit.v1.BuildHistoryEvent.type:type_name -> moby.buildkit.v1.BuildHistoryEventType
|
|
22, // 31: moby.buildkit.v1.BuildHistoryEvent.record:type_name -> moby.buildkit.v1.BuildHistoryRecord
|
|
34, // 32: moby.buildkit.v1.BuildHistoryRecord.FrontendAttrs:type_name -> moby.buildkit.v1.BuildHistoryRecord.FrontendAttrsEntry
|
|
27, // 33: moby.buildkit.v1.BuildHistoryRecord.Exporters:type_name -> moby.buildkit.v1.Exporter
|
|
48, // 34: moby.buildkit.v1.BuildHistoryRecord.error:type_name -> google.rpc.Status
|
|
40, // 35: moby.buildkit.v1.BuildHistoryRecord.CreatedAt:type_name -> google.protobuf.Timestamp
|
|
40, // 36: moby.buildkit.v1.BuildHistoryRecord.CompletedAt:type_name -> google.protobuf.Timestamp
|
|
25, // 37: moby.buildkit.v1.BuildHistoryRecord.logs:type_name -> moby.buildkit.v1.Descriptor
|
|
35, // 38: moby.buildkit.v1.BuildHistoryRecord.ExporterResponse:type_name -> moby.buildkit.v1.BuildHistoryRecord.ExporterResponseEntry
|
|
26, // 39: moby.buildkit.v1.BuildHistoryRecord.Result:type_name -> moby.buildkit.v1.BuildResultInfo
|
|
36, // 40: moby.buildkit.v1.BuildHistoryRecord.Results:type_name -> moby.buildkit.v1.BuildHistoryRecord.ResultsEntry
|
|
25, // 41: moby.buildkit.v1.BuildHistoryRecord.trace:type_name -> moby.buildkit.v1.Descriptor
|
|
25, // 42: moby.buildkit.v1.BuildHistoryRecord.externalError:type_name -> moby.buildkit.v1.Descriptor
|
|
37, // 43: moby.buildkit.v1.Descriptor.annotations:type_name -> moby.buildkit.v1.Descriptor.AnnotationsEntry
|
|
25, // 44: moby.buildkit.v1.BuildResultInfo.ResultDeprecated:type_name -> moby.buildkit.v1.Descriptor
|
|
25, // 45: moby.buildkit.v1.BuildResultInfo.Attestations:type_name -> moby.buildkit.v1.Descriptor
|
|
38, // 46: moby.buildkit.v1.BuildResultInfo.Results:type_name -> moby.buildkit.v1.BuildResultInfo.ResultsEntry
|
|
39, // 47: moby.buildkit.v1.Exporter.Attrs:type_name -> moby.buildkit.v1.Exporter.AttrsEntry
|
|
41, // 48: moby.buildkit.v1.SolveRequest.FrontendInputsEntry.value:type_name -> pb.Definition
|
|
26, // 49: moby.buildkit.v1.BuildHistoryRecord.ResultsEntry.value:type_name -> moby.buildkit.v1.BuildResultInfo
|
|
25, // 50: moby.buildkit.v1.BuildResultInfo.ResultsEntry.value:type_name -> moby.buildkit.v1.Descriptor
|
|
2, // 51: moby.buildkit.v1.Control.DiskUsage:input_type -> moby.buildkit.v1.DiskUsageRequest
|
|
1, // 52: moby.buildkit.v1.Control.Prune:input_type -> moby.buildkit.v1.PruneRequest
|
|
5, // 53: moby.buildkit.v1.Control.Solve:input_type -> moby.buildkit.v1.SolveRequest
|
|
9, // 54: moby.buildkit.v1.Control.Status:input_type -> moby.buildkit.v1.StatusRequest
|
|
15, // 55: moby.buildkit.v1.Control.Session:input_type -> moby.buildkit.v1.BytesMessage
|
|
16, // 56: moby.buildkit.v1.Control.ListWorkers:input_type -> moby.buildkit.v1.ListWorkersRequest
|
|
18, // 57: moby.buildkit.v1.Control.Info:input_type -> moby.buildkit.v1.InfoRequest
|
|
20, // 58: moby.buildkit.v1.Control.ListenBuildHistory:input_type -> moby.buildkit.v1.BuildHistoryRequest
|
|
23, // 59: moby.buildkit.v1.Control.UpdateBuildHistory:input_type -> moby.buildkit.v1.UpdateBuildHistoryRequest
|
|
3, // 60: moby.buildkit.v1.Control.DiskUsage:output_type -> moby.buildkit.v1.DiskUsageResponse
|
|
4, // 61: moby.buildkit.v1.Control.Prune:output_type -> moby.buildkit.v1.UsageRecord
|
|
8, // 62: moby.buildkit.v1.Control.Solve:output_type -> moby.buildkit.v1.SolveResponse
|
|
10, // 63: moby.buildkit.v1.Control.Status:output_type -> moby.buildkit.v1.StatusResponse
|
|
15, // 64: moby.buildkit.v1.Control.Session:output_type -> moby.buildkit.v1.BytesMessage
|
|
17, // 65: moby.buildkit.v1.Control.ListWorkers:output_type -> moby.buildkit.v1.ListWorkersResponse
|
|
19, // 66: moby.buildkit.v1.Control.Info:output_type -> moby.buildkit.v1.InfoResponse
|
|
21, // 67: moby.buildkit.v1.Control.ListenBuildHistory:output_type -> moby.buildkit.v1.BuildHistoryEvent
|
|
24, // 68: moby.buildkit.v1.Control.UpdateBuildHistory:output_type -> moby.buildkit.v1.UpdateBuildHistoryResponse
|
|
60, // [60:69] is the sub-list for method output_type
|
|
51, // [51:60] is the sub-list for method input_type
|
|
51, // [51:51] is the sub-list for extension type_name
|
|
51, // [51:51] is the sub-list for extension extendee
|
|
0, // [0:51] is the sub-list for field type_name
|
|
}
|
|
|
|
func init() { file_control_proto_init() }
|
|
func file_control_proto_init() {
|
|
if File_control_proto != nil {
|
|
return
|
|
}
|
|
if !protoimpl.UnsafeEnabled {
|
|
file_control_proto_msgTypes[0].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*PruneRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_control_proto_msgTypes[1].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*DiskUsageRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_control_proto_msgTypes[2].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*DiskUsageResponse); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_control_proto_msgTypes[3].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*UsageRecord); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_control_proto_msgTypes[4].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*SolveRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_control_proto_msgTypes[5].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*CacheOptions); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_control_proto_msgTypes[6].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*CacheOptionsEntry); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_control_proto_msgTypes[7].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*SolveResponse); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_control_proto_msgTypes[8].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*StatusRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_control_proto_msgTypes[9].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*StatusResponse); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_control_proto_msgTypes[10].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*Vertex); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_control_proto_msgTypes[11].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*VertexStatus); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_control_proto_msgTypes[12].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*VertexLog); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_control_proto_msgTypes[13].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*VertexWarning); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_control_proto_msgTypes[14].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*BytesMessage); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_control_proto_msgTypes[15].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*ListWorkersRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_control_proto_msgTypes[16].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*ListWorkersResponse); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_control_proto_msgTypes[17].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*InfoRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_control_proto_msgTypes[18].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*InfoResponse); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_control_proto_msgTypes[19].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*BuildHistoryRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_control_proto_msgTypes[20].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*BuildHistoryEvent); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_control_proto_msgTypes[21].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*BuildHistoryRecord); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_control_proto_msgTypes[22].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*UpdateBuildHistoryRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_control_proto_msgTypes[23].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*UpdateBuildHistoryResponse); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_control_proto_msgTypes[24].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*Descriptor); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_control_proto_msgTypes[25].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*BuildResultInfo); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_control_proto_msgTypes[26].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*Exporter); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
}
|
|
type x struct{}
|
|
out := protoimpl.TypeBuilder{
|
|
File: protoimpl.DescBuilder{
|
|
GoPackagePath: reflect.TypeOf(x{}).PkgPath(),
|
|
RawDescriptor: file_control_proto_rawDesc,
|
|
NumEnums: 1,
|
|
NumMessages: 39,
|
|
NumExtensions: 0,
|
|
NumServices: 1,
|
|
},
|
|
GoTypes: file_control_proto_goTypes,
|
|
DependencyIndexes: file_control_proto_depIdxs,
|
|
EnumInfos: file_control_proto_enumTypes,
|
|
MessageInfos: file_control_proto_msgTypes,
|
|
}.Build()
|
|
File_control_proto = out.File
|
|
file_control_proto_rawDesc = nil
|
|
file_control_proto_goTypes = nil
|
|
file_control_proto_depIdxs = nil
|
|
}
|