mirror of
https://gitea.com/Lydanne/buildx.git
synced 2025-05-20 02:17:44 +08:00
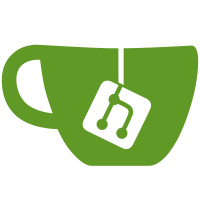
Removes gogo/protobuf from buildx and updates to a version of moby/buildkit where gogo is removed. This also changes how the proto files are generated. This is because newer versions of protobuf are more strict about name conflicts. If two files have the same name (even if they are relative paths) and are used in different protoc commands, they'll conflict in the registry. Since protobuf file generation doesn't work very well with `paths=source_relative`, this removes the `go:generate` expression and just relies on the dockerfile to perform the generation. Signed-off-by: Jonathan A. Sternberg <jonathan.sternberg@docker.com>
77 lines
2.9 KiB
Go
77 lines
2.9 KiB
Go
/*
|
|
*
|
|
* Copyright 2018 gRPC authors.
|
|
*
|
|
* Licensed under the Apache License, Version 2.0 (the "License");
|
|
* you may not use this file except in compliance with the License.
|
|
* You may obtain a copy of the License at
|
|
*
|
|
* http://www.apache.org/licenses/LICENSE-2.0
|
|
*
|
|
* Unless required by applicable law or agreed to in writing, software
|
|
* distributed under the License is distributed on an "AS IS" BASIS,
|
|
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
* See the License for the specific language governing permissions and
|
|
* limitations under the License.
|
|
*
|
|
*/
|
|
|
|
// Package envconfig contains grpc settings configured by environment variables.
|
|
package envconfig
|
|
|
|
import (
|
|
"os"
|
|
"strconv"
|
|
"strings"
|
|
)
|
|
|
|
var (
|
|
// TXTErrIgnore is set if TXT errors should be ignored ("GRPC_GO_IGNORE_TXT_ERRORS" is not "false").
|
|
TXTErrIgnore = boolFromEnv("GRPC_GO_IGNORE_TXT_ERRORS", true)
|
|
// RingHashCap indicates the maximum ring size which defaults to 4096
|
|
// entries but may be overridden by setting the environment variable
|
|
// "GRPC_RING_HASH_CAP". This does not override the default bounds
|
|
// checking which NACKs configs specifying ring sizes > 8*1024*1024 (~8M).
|
|
RingHashCap = uint64FromEnv("GRPC_RING_HASH_CAP", 4096, 1, 8*1024*1024)
|
|
// LeastRequestLB is set if we should support the least_request_experimental
|
|
// LB policy, which can be enabled by setting the environment variable
|
|
// "GRPC_EXPERIMENTAL_ENABLE_LEAST_REQUEST" to "true".
|
|
LeastRequestLB = boolFromEnv("GRPC_EXPERIMENTAL_ENABLE_LEAST_REQUEST", false)
|
|
// ALTSMaxConcurrentHandshakes is the maximum number of concurrent ALTS
|
|
// handshakes that can be performed.
|
|
ALTSMaxConcurrentHandshakes = uint64FromEnv("GRPC_ALTS_MAX_CONCURRENT_HANDSHAKES", 100, 1, 100)
|
|
// EnforceALPNEnabled is set if TLS connections to servers with ALPN disabled
|
|
// should be rejected. The HTTP/2 protocol requires ALPN to be enabled, this
|
|
// option is present for backward compatibility. This option may be overridden
|
|
// by setting the environment variable "GRPC_ENFORCE_ALPN_ENABLED" to "true"
|
|
// or "false".
|
|
EnforceALPNEnabled = boolFromEnv("GRPC_ENFORCE_ALPN_ENABLED", false)
|
|
// XDSFallbackSupport is the env variable that controls whether support for
|
|
// xDS fallback is turned on. If this is unset or is false, only the first
|
|
// xDS server in the list of server configs will be used.
|
|
XDSFallbackSupport = boolFromEnv("GRPC_EXPERIMENTAL_XDS_FALLBACK", false)
|
|
)
|
|
|
|
func boolFromEnv(envVar string, def bool) bool {
|
|
if def {
|
|
// The default is true; return true unless the variable is "false".
|
|
return !strings.EqualFold(os.Getenv(envVar), "false")
|
|
}
|
|
// The default is false; return false unless the variable is "true".
|
|
return strings.EqualFold(os.Getenv(envVar), "true")
|
|
}
|
|
|
|
func uint64FromEnv(envVar string, def, min, max uint64) uint64 {
|
|
v, err := strconv.ParseUint(os.Getenv(envVar), 10, 64)
|
|
if err != nil {
|
|
return def
|
|
}
|
|
if v < min {
|
|
return min
|
|
}
|
|
if v > max {
|
|
return max
|
|
}
|
|
return v
|
|
}
|