mirror of
https://gitea.com/Lydanne/buildx.git
synced 2025-07-06 03:27:41 +08:00
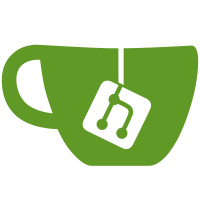
Integrates vtproto into buildx. The generated files dockerfile has been modified to copy the buildkit equivalent file to ensure files are laid out in the appropriate way for imports. An import has also been included to change the grpc codec to the version in buildkit that supports vtproto. This will allow buildx to utilize the speed and memory improvements from that. Also updates the gc control options for prune. Signed-off-by: Jonathan A. Sternberg <jonathan.sternberg@docker.com>
201 lines
4.4 KiB
Go
201 lines
4.4 KiB
Go
// Code generated by protoc-gen-go-vtproto. DO NOT EDIT.
|
|
// protoc-gen-go-vtproto version: v0.6.1-0.20240319094008-0393e58bdf10
|
|
// source: github.com/moby/buildkit/session/upload/upload.proto
|
|
|
|
package upload
|
|
|
|
import (
|
|
fmt "fmt"
|
|
protohelpers "github.com/planetscale/vtprotobuf/protohelpers"
|
|
proto "google.golang.org/protobuf/proto"
|
|
protoimpl "google.golang.org/protobuf/runtime/protoimpl"
|
|
io "io"
|
|
)
|
|
|
|
const (
|
|
// Verify that this generated code is sufficiently up-to-date.
|
|
_ = protoimpl.EnforceVersion(20 - protoimpl.MinVersion)
|
|
// Verify that runtime/protoimpl is sufficiently up-to-date.
|
|
_ = protoimpl.EnforceVersion(protoimpl.MaxVersion - 20)
|
|
)
|
|
|
|
func (m *BytesMessage) CloneVT() *BytesMessage {
|
|
if m == nil {
|
|
return (*BytesMessage)(nil)
|
|
}
|
|
r := new(BytesMessage)
|
|
if rhs := m.Data; rhs != nil {
|
|
tmpBytes := make([]byte, len(rhs))
|
|
copy(tmpBytes, rhs)
|
|
r.Data = tmpBytes
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *BytesMessage) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (this *BytesMessage) EqualVT(that *BytesMessage) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if string(this.Data) != string(that.Data) {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *BytesMessage) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*BytesMessage)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (m *BytesMessage) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *BytesMessage) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *BytesMessage) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.Data) > 0 {
|
|
i -= len(m.Data)
|
|
copy(dAtA[i:], m.Data)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Data)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *BytesMessage) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.Data)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *BytesMessage) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: BytesMessage: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: BytesMessage: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Data", wireType)
|
|
}
|
|
var byteLen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
byteLen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if byteLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + byteLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Data = append(m.Data[:0], dAtA[iNdEx:postIndex]...)
|
|
if m.Data == nil {
|
|
m.Data = []byte{}
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|