mirror of
https://gitea.com/Lydanne/buildx.git
synced 2025-05-18 09:17:49 +08:00
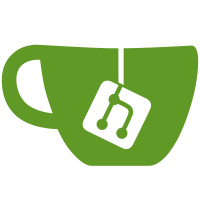
Integrates vtproto into buildx. The generated files dockerfile has been modified to copy the buildkit equivalent file to ensure files are laid out in the appropriate way for imports. An import has also been included to change the grpc codec to the version in buildkit that supports vtproto. This will allow buildx to utilize the speed and memory improvements from that. Also updates the gc control options for prune. Signed-off-by: Jonathan A. Sternberg <jonathan.sternberg@docker.com>
14051 lines
296 KiB
Go
14051 lines
296 KiB
Go
// Code generated by protoc-gen-go-vtproto. DO NOT EDIT.
|
|
// protoc-gen-go-vtproto version: v0.6.1-0.20240319094008-0393e58bdf10
|
|
// source: github.com/moby/buildkit/solver/pb/ops.proto
|
|
|
|
package pb
|
|
|
|
import (
|
|
fmt "fmt"
|
|
protohelpers "github.com/planetscale/vtprotobuf/protohelpers"
|
|
proto "google.golang.org/protobuf/proto"
|
|
protoimpl "google.golang.org/protobuf/runtime/protoimpl"
|
|
io "io"
|
|
)
|
|
|
|
const (
|
|
// Verify that this generated code is sufficiently up-to-date.
|
|
_ = protoimpl.EnforceVersion(20 - protoimpl.MinVersion)
|
|
// Verify that runtime/protoimpl is sufficiently up-to-date.
|
|
_ = protoimpl.EnforceVersion(protoimpl.MaxVersion - 20)
|
|
)
|
|
|
|
func (m *Op) CloneVT() *Op {
|
|
if m == nil {
|
|
return (*Op)(nil)
|
|
}
|
|
r := new(Op)
|
|
r.Platform = m.Platform.CloneVT()
|
|
r.Constraints = m.Constraints.CloneVT()
|
|
if rhs := m.Inputs; rhs != nil {
|
|
tmpContainer := make([]*Input, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpContainer[k] = v.CloneVT()
|
|
}
|
|
r.Inputs = tmpContainer
|
|
}
|
|
if m.Op != nil {
|
|
r.Op = m.Op.(interface{ CloneVT() isOp_Op }).CloneVT()
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *Op) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *Op_Exec) CloneVT() isOp_Op {
|
|
if m == nil {
|
|
return (*Op_Exec)(nil)
|
|
}
|
|
r := new(Op_Exec)
|
|
r.Exec = m.Exec.CloneVT()
|
|
return r
|
|
}
|
|
|
|
func (m *Op_Source) CloneVT() isOp_Op {
|
|
if m == nil {
|
|
return (*Op_Source)(nil)
|
|
}
|
|
r := new(Op_Source)
|
|
r.Source = m.Source.CloneVT()
|
|
return r
|
|
}
|
|
|
|
func (m *Op_File) CloneVT() isOp_Op {
|
|
if m == nil {
|
|
return (*Op_File)(nil)
|
|
}
|
|
r := new(Op_File)
|
|
r.File = m.File.CloneVT()
|
|
return r
|
|
}
|
|
|
|
func (m *Op_Build) CloneVT() isOp_Op {
|
|
if m == nil {
|
|
return (*Op_Build)(nil)
|
|
}
|
|
r := new(Op_Build)
|
|
r.Build = m.Build.CloneVT()
|
|
return r
|
|
}
|
|
|
|
func (m *Op_Merge) CloneVT() isOp_Op {
|
|
if m == nil {
|
|
return (*Op_Merge)(nil)
|
|
}
|
|
r := new(Op_Merge)
|
|
r.Merge = m.Merge.CloneVT()
|
|
return r
|
|
}
|
|
|
|
func (m *Op_Diff) CloneVT() isOp_Op {
|
|
if m == nil {
|
|
return (*Op_Diff)(nil)
|
|
}
|
|
r := new(Op_Diff)
|
|
r.Diff = m.Diff.CloneVT()
|
|
return r
|
|
}
|
|
|
|
func (m *Platform) CloneVT() *Platform {
|
|
if m == nil {
|
|
return (*Platform)(nil)
|
|
}
|
|
r := new(Platform)
|
|
r.Architecture = m.Architecture
|
|
r.OS = m.OS
|
|
r.Variant = m.Variant
|
|
r.OSVersion = m.OSVersion
|
|
if rhs := m.OSFeatures; rhs != nil {
|
|
tmpContainer := make([]string, len(rhs))
|
|
copy(tmpContainer, rhs)
|
|
r.OSFeatures = tmpContainer
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *Platform) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *Input) CloneVT() *Input {
|
|
if m == nil {
|
|
return (*Input)(nil)
|
|
}
|
|
r := new(Input)
|
|
r.Digest = m.Digest
|
|
r.Index = m.Index
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *Input) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *ExecOp) CloneVT() *ExecOp {
|
|
if m == nil {
|
|
return (*ExecOp)(nil)
|
|
}
|
|
r := new(ExecOp)
|
|
r.Meta = m.Meta.CloneVT()
|
|
r.Network = m.Network
|
|
r.Security = m.Security
|
|
if rhs := m.Mounts; rhs != nil {
|
|
tmpContainer := make([]*Mount, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpContainer[k] = v.CloneVT()
|
|
}
|
|
r.Mounts = tmpContainer
|
|
}
|
|
if rhs := m.Secretenv; rhs != nil {
|
|
tmpContainer := make([]*SecretEnv, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpContainer[k] = v.CloneVT()
|
|
}
|
|
r.Secretenv = tmpContainer
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *ExecOp) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *Meta) CloneVT() *Meta {
|
|
if m == nil {
|
|
return (*Meta)(nil)
|
|
}
|
|
r := new(Meta)
|
|
r.Cwd = m.Cwd
|
|
r.User = m.User
|
|
r.ProxyEnv = m.ProxyEnv.CloneVT()
|
|
r.Hostname = m.Hostname
|
|
r.CgroupParent = m.CgroupParent
|
|
r.RemoveMountStubsRecursive = m.RemoveMountStubsRecursive
|
|
if rhs := m.Args; rhs != nil {
|
|
tmpContainer := make([]string, len(rhs))
|
|
copy(tmpContainer, rhs)
|
|
r.Args = tmpContainer
|
|
}
|
|
if rhs := m.Env; rhs != nil {
|
|
tmpContainer := make([]string, len(rhs))
|
|
copy(tmpContainer, rhs)
|
|
r.Env = tmpContainer
|
|
}
|
|
if rhs := m.ExtraHosts; rhs != nil {
|
|
tmpContainer := make([]*HostIP, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpContainer[k] = v.CloneVT()
|
|
}
|
|
r.ExtraHosts = tmpContainer
|
|
}
|
|
if rhs := m.Ulimit; rhs != nil {
|
|
tmpContainer := make([]*Ulimit, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpContainer[k] = v.CloneVT()
|
|
}
|
|
r.Ulimit = tmpContainer
|
|
}
|
|
if rhs := m.ValidExitCodes; rhs != nil {
|
|
tmpContainer := make([]int32, len(rhs))
|
|
copy(tmpContainer, rhs)
|
|
r.ValidExitCodes = tmpContainer
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *Meta) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *HostIP) CloneVT() *HostIP {
|
|
if m == nil {
|
|
return (*HostIP)(nil)
|
|
}
|
|
r := new(HostIP)
|
|
r.Host = m.Host
|
|
r.IP = m.IP
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *HostIP) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *Ulimit) CloneVT() *Ulimit {
|
|
if m == nil {
|
|
return (*Ulimit)(nil)
|
|
}
|
|
r := new(Ulimit)
|
|
r.Name = m.Name
|
|
r.Soft = m.Soft
|
|
r.Hard = m.Hard
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *Ulimit) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *SecretEnv) CloneVT() *SecretEnv {
|
|
if m == nil {
|
|
return (*SecretEnv)(nil)
|
|
}
|
|
r := new(SecretEnv)
|
|
r.ID = m.ID
|
|
r.Name = m.Name
|
|
r.Optional = m.Optional
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *SecretEnv) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *Mount) CloneVT() *Mount {
|
|
if m == nil {
|
|
return (*Mount)(nil)
|
|
}
|
|
r := new(Mount)
|
|
r.Input = m.Input
|
|
r.Selector = m.Selector
|
|
r.Dest = m.Dest
|
|
r.Output = m.Output
|
|
r.Readonly = m.Readonly
|
|
r.MountType = m.MountType
|
|
r.TmpfsOpt = m.TmpfsOpt.CloneVT()
|
|
r.CacheOpt = m.CacheOpt.CloneVT()
|
|
r.SecretOpt = m.SecretOpt.CloneVT()
|
|
r.SSHOpt = m.SSHOpt.CloneVT()
|
|
r.ResultID = m.ResultID
|
|
r.ContentCache = m.ContentCache
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *Mount) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *TmpfsOpt) CloneVT() *TmpfsOpt {
|
|
if m == nil {
|
|
return (*TmpfsOpt)(nil)
|
|
}
|
|
r := new(TmpfsOpt)
|
|
r.Size = m.Size
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *TmpfsOpt) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *CacheOpt) CloneVT() *CacheOpt {
|
|
if m == nil {
|
|
return (*CacheOpt)(nil)
|
|
}
|
|
r := new(CacheOpt)
|
|
r.ID = m.ID
|
|
r.Sharing = m.Sharing
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *CacheOpt) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *SecretOpt) CloneVT() *SecretOpt {
|
|
if m == nil {
|
|
return (*SecretOpt)(nil)
|
|
}
|
|
r := new(SecretOpt)
|
|
r.ID = m.ID
|
|
r.Uid = m.Uid
|
|
r.Gid = m.Gid
|
|
r.Mode = m.Mode
|
|
r.Optional = m.Optional
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *SecretOpt) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *SSHOpt) CloneVT() *SSHOpt {
|
|
if m == nil {
|
|
return (*SSHOpt)(nil)
|
|
}
|
|
r := new(SSHOpt)
|
|
r.ID = m.ID
|
|
r.Uid = m.Uid
|
|
r.Gid = m.Gid
|
|
r.Mode = m.Mode
|
|
r.Optional = m.Optional
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *SSHOpt) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *SourceOp) CloneVT() *SourceOp {
|
|
if m == nil {
|
|
return (*SourceOp)(nil)
|
|
}
|
|
r := new(SourceOp)
|
|
r.Identifier = m.Identifier
|
|
if rhs := m.Attrs; rhs != nil {
|
|
tmpContainer := make(map[string]string, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpContainer[k] = v
|
|
}
|
|
r.Attrs = tmpContainer
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *SourceOp) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *BuildOp) CloneVT() *BuildOp {
|
|
if m == nil {
|
|
return (*BuildOp)(nil)
|
|
}
|
|
r := new(BuildOp)
|
|
r.Builder = m.Builder
|
|
r.Def = m.Def.CloneVT()
|
|
if rhs := m.Inputs; rhs != nil {
|
|
tmpContainer := make(map[string]*BuildInput, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpContainer[k] = v.CloneVT()
|
|
}
|
|
r.Inputs = tmpContainer
|
|
}
|
|
if rhs := m.Attrs; rhs != nil {
|
|
tmpContainer := make(map[string]string, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpContainer[k] = v
|
|
}
|
|
r.Attrs = tmpContainer
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *BuildOp) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *BuildInput) CloneVT() *BuildInput {
|
|
if m == nil {
|
|
return (*BuildInput)(nil)
|
|
}
|
|
r := new(BuildInput)
|
|
r.Input = m.Input
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *BuildInput) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *OpMetadata) CloneVT() *OpMetadata {
|
|
if m == nil {
|
|
return (*OpMetadata)(nil)
|
|
}
|
|
r := new(OpMetadata)
|
|
r.IgnoreCache = m.IgnoreCache
|
|
r.ExportCache = m.ExportCache.CloneVT()
|
|
r.ProgressGroup = m.ProgressGroup.CloneVT()
|
|
if rhs := m.Description; rhs != nil {
|
|
tmpContainer := make(map[string]string, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpContainer[k] = v
|
|
}
|
|
r.Description = tmpContainer
|
|
}
|
|
if rhs := m.Caps; rhs != nil {
|
|
tmpContainer := make(map[string]bool, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpContainer[k] = v
|
|
}
|
|
r.Caps = tmpContainer
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *OpMetadata) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *Source) CloneVT() *Source {
|
|
if m == nil {
|
|
return (*Source)(nil)
|
|
}
|
|
r := new(Source)
|
|
if rhs := m.Locations; rhs != nil {
|
|
tmpContainer := make(map[string]*Locations, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpContainer[k] = v.CloneVT()
|
|
}
|
|
r.Locations = tmpContainer
|
|
}
|
|
if rhs := m.Infos; rhs != nil {
|
|
tmpContainer := make([]*SourceInfo, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpContainer[k] = v.CloneVT()
|
|
}
|
|
r.Infos = tmpContainer
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *Source) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *Locations) CloneVT() *Locations {
|
|
if m == nil {
|
|
return (*Locations)(nil)
|
|
}
|
|
r := new(Locations)
|
|
if rhs := m.Locations; rhs != nil {
|
|
tmpContainer := make([]*Location, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpContainer[k] = v.CloneVT()
|
|
}
|
|
r.Locations = tmpContainer
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *Locations) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *SourceInfo) CloneVT() *SourceInfo {
|
|
if m == nil {
|
|
return (*SourceInfo)(nil)
|
|
}
|
|
r := new(SourceInfo)
|
|
r.Filename = m.Filename
|
|
r.Definition = m.Definition.CloneVT()
|
|
r.Language = m.Language
|
|
if rhs := m.Data; rhs != nil {
|
|
tmpBytes := make([]byte, len(rhs))
|
|
copy(tmpBytes, rhs)
|
|
r.Data = tmpBytes
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *SourceInfo) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *Location) CloneVT() *Location {
|
|
if m == nil {
|
|
return (*Location)(nil)
|
|
}
|
|
r := new(Location)
|
|
r.SourceIndex = m.SourceIndex
|
|
if rhs := m.Ranges; rhs != nil {
|
|
tmpContainer := make([]*Range, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpContainer[k] = v.CloneVT()
|
|
}
|
|
r.Ranges = tmpContainer
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *Location) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *Range) CloneVT() *Range {
|
|
if m == nil {
|
|
return (*Range)(nil)
|
|
}
|
|
r := new(Range)
|
|
r.Start = m.Start.CloneVT()
|
|
r.End = m.End.CloneVT()
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *Range) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *Position) CloneVT() *Position {
|
|
if m == nil {
|
|
return (*Position)(nil)
|
|
}
|
|
r := new(Position)
|
|
r.Line = m.Line
|
|
r.Character = m.Character
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *Position) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *ExportCache) CloneVT() *ExportCache {
|
|
if m == nil {
|
|
return (*ExportCache)(nil)
|
|
}
|
|
r := new(ExportCache)
|
|
r.Value = m.Value
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *ExportCache) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *ProgressGroup) CloneVT() *ProgressGroup {
|
|
if m == nil {
|
|
return (*ProgressGroup)(nil)
|
|
}
|
|
r := new(ProgressGroup)
|
|
r.Id = m.Id
|
|
r.Name = m.Name
|
|
r.Weak = m.Weak
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *ProgressGroup) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *ProxyEnv) CloneVT() *ProxyEnv {
|
|
if m == nil {
|
|
return (*ProxyEnv)(nil)
|
|
}
|
|
r := new(ProxyEnv)
|
|
r.HttpProxy = m.HttpProxy
|
|
r.HttpsProxy = m.HttpsProxy
|
|
r.FtpProxy = m.FtpProxy
|
|
r.NoProxy = m.NoProxy
|
|
r.AllProxy = m.AllProxy
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *ProxyEnv) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *WorkerConstraints) CloneVT() *WorkerConstraints {
|
|
if m == nil {
|
|
return (*WorkerConstraints)(nil)
|
|
}
|
|
r := new(WorkerConstraints)
|
|
if rhs := m.Filter; rhs != nil {
|
|
tmpContainer := make([]string, len(rhs))
|
|
copy(tmpContainer, rhs)
|
|
r.Filter = tmpContainer
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *WorkerConstraints) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *Definition) CloneVT() *Definition {
|
|
if m == nil {
|
|
return (*Definition)(nil)
|
|
}
|
|
r := new(Definition)
|
|
r.Source = m.Source.CloneVT()
|
|
if rhs := m.Def; rhs != nil {
|
|
tmpContainer := make([][]byte, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpBytes := make([]byte, len(v))
|
|
copy(tmpBytes, v)
|
|
tmpContainer[k] = tmpBytes
|
|
}
|
|
r.Def = tmpContainer
|
|
}
|
|
if rhs := m.Metadata; rhs != nil {
|
|
tmpContainer := make(map[string]*OpMetadata, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpContainer[k] = v.CloneVT()
|
|
}
|
|
r.Metadata = tmpContainer
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *Definition) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *FileOp) CloneVT() *FileOp {
|
|
if m == nil {
|
|
return (*FileOp)(nil)
|
|
}
|
|
r := new(FileOp)
|
|
if rhs := m.Actions; rhs != nil {
|
|
tmpContainer := make([]*FileAction, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpContainer[k] = v.CloneVT()
|
|
}
|
|
r.Actions = tmpContainer
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *FileOp) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *FileAction) CloneVT() *FileAction {
|
|
if m == nil {
|
|
return (*FileAction)(nil)
|
|
}
|
|
r := new(FileAction)
|
|
r.Input = m.Input
|
|
r.SecondaryInput = m.SecondaryInput
|
|
r.Output = m.Output
|
|
if m.Action != nil {
|
|
r.Action = m.Action.(interface{ CloneVT() isFileAction_Action }).CloneVT()
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *FileAction) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *FileAction_Copy) CloneVT() isFileAction_Action {
|
|
if m == nil {
|
|
return (*FileAction_Copy)(nil)
|
|
}
|
|
r := new(FileAction_Copy)
|
|
r.Copy = m.Copy.CloneVT()
|
|
return r
|
|
}
|
|
|
|
func (m *FileAction_Mkfile) CloneVT() isFileAction_Action {
|
|
if m == nil {
|
|
return (*FileAction_Mkfile)(nil)
|
|
}
|
|
r := new(FileAction_Mkfile)
|
|
r.Mkfile = m.Mkfile.CloneVT()
|
|
return r
|
|
}
|
|
|
|
func (m *FileAction_Mkdir) CloneVT() isFileAction_Action {
|
|
if m == nil {
|
|
return (*FileAction_Mkdir)(nil)
|
|
}
|
|
r := new(FileAction_Mkdir)
|
|
r.Mkdir = m.Mkdir.CloneVT()
|
|
return r
|
|
}
|
|
|
|
func (m *FileAction_Rm) CloneVT() isFileAction_Action {
|
|
if m == nil {
|
|
return (*FileAction_Rm)(nil)
|
|
}
|
|
r := new(FileAction_Rm)
|
|
r.Rm = m.Rm.CloneVT()
|
|
return r
|
|
}
|
|
|
|
func (m *FileActionCopy) CloneVT() *FileActionCopy {
|
|
if m == nil {
|
|
return (*FileActionCopy)(nil)
|
|
}
|
|
r := new(FileActionCopy)
|
|
r.Src = m.Src
|
|
r.Dest = m.Dest
|
|
r.Owner = m.Owner.CloneVT()
|
|
r.Mode = m.Mode
|
|
r.FollowSymlink = m.FollowSymlink
|
|
r.DirCopyContents = m.DirCopyContents
|
|
r.AttemptUnpackDockerCompatibility = m.AttemptUnpackDockerCompatibility
|
|
r.CreateDestPath = m.CreateDestPath
|
|
r.AllowWildcard = m.AllowWildcard
|
|
r.AllowEmptyWildcard = m.AllowEmptyWildcard
|
|
r.Timestamp = m.Timestamp
|
|
r.AlwaysReplaceExistingDestPaths = m.AlwaysReplaceExistingDestPaths
|
|
r.ModeStr = m.ModeStr
|
|
if rhs := m.IncludePatterns; rhs != nil {
|
|
tmpContainer := make([]string, len(rhs))
|
|
copy(tmpContainer, rhs)
|
|
r.IncludePatterns = tmpContainer
|
|
}
|
|
if rhs := m.ExcludePatterns; rhs != nil {
|
|
tmpContainer := make([]string, len(rhs))
|
|
copy(tmpContainer, rhs)
|
|
r.ExcludePatterns = tmpContainer
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *FileActionCopy) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *FileActionMkFile) CloneVT() *FileActionMkFile {
|
|
if m == nil {
|
|
return (*FileActionMkFile)(nil)
|
|
}
|
|
r := new(FileActionMkFile)
|
|
r.Path = m.Path
|
|
r.Mode = m.Mode
|
|
r.Owner = m.Owner.CloneVT()
|
|
r.Timestamp = m.Timestamp
|
|
if rhs := m.Data; rhs != nil {
|
|
tmpBytes := make([]byte, len(rhs))
|
|
copy(tmpBytes, rhs)
|
|
r.Data = tmpBytes
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *FileActionMkFile) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *FileActionMkDir) CloneVT() *FileActionMkDir {
|
|
if m == nil {
|
|
return (*FileActionMkDir)(nil)
|
|
}
|
|
r := new(FileActionMkDir)
|
|
r.Path = m.Path
|
|
r.Mode = m.Mode
|
|
r.MakeParents = m.MakeParents
|
|
r.Owner = m.Owner.CloneVT()
|
|
r.Timestamp = m.Timestamp
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *FileActionMkDir) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *FileActionRm) CloneVT() *FileActionRm {
|
|
if m == nil {
|
|
return (*FileActionRm)(nil)
|
|
}
|
|
r := new(FileActionRm)
|
|
r.Path = m.Path
|
|
r.AllowNotFound = m.AllowNotFound
|
|
r.AllowWildcard = m.AllowWildcard
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *FileActionRm) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *ChownOpt) CloneVT() *ChownOpt {
|
|
if m == nil {
|
|
return (*ChownOpt)(nil)
|
|
}
|
|
r := new(ChownOpt)
|
|
r.User = m.User.CloneVT()
|
|
r.Group = m.Group.CloneVT()
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *ChownOpt) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *UserOpt) CloneVT() *UserOpt {
|
|
if m == nil {
|
|
return (*UserOpt)(nil)
|
|
}
|
|
r := new(UserOpt)
|
|
if m.User != nil {
|
|
r.User = m.User.(interface{ CloneVT() isUserOpt_User }).CloneVT()
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *UserOpt) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *UserOpt_ByName) CloneVT() isUserOpt_User {
|
|
if m == nil {
|
|
return (*UserOpt_ByName)(nil)
|
|
}
|
|
r := new(UserOpt_ByName)
|
|
r.ByName = m.ByName.CloneVT()
|
|
return r
|
|
}
|
|
|
|
func (m *UserOpt_ByID) CloneVT() isUserOpt_User {
|
|
if m == nil {
|
|
return (*UserOpt_ByID)(nil)
|
|
}
|
|
r := new(UserOpt_ByID)
|
|
r.ByID = m.ByID
|
|
return r
|
|
}
|
|
|
|
func (m *NamedUserOpt) CloneVT() *NamedUserOpt {
|
|
if m == nil {
|
|
return (*NamedUserOpt)(nil)
|
|
}
|
|
r := new(NamedUserOpt)
|
|
r.Name = m.Name
|
|
r.Input = m.Input
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *NamedUserOpt) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *MergeInput) CloneVT() *MergeInput {
|
|
if m == nil {
|
|
return (*MergeInput)(nil)
|
|
}
|
|
r := new(MergeInput)
|
|
r.Input = m.Input
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *MergeInput) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *MergeOp) CloneVT() *MergeOp {
|
|
if m == nil {
|
|
return (*MergeOp)(nil)
|
|
}
|
|
r := new(MergeOp)
|
|
if rhs := m.Inputs; rhs != nil {
|
|
tmpContainer := make([]*MergeInput, len(rhs))
|
|
for k, v := range rhs {
|
|
tmpContainer[k] = v.CloneVT()
|
|
}
|
|
r.Inputs = tmpContainer
|
|
}
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *MergeOp) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *LowerDiffInput) CloneVT() *LowerDiffInput {
|
|
if m == nil {
|
|
return (*LowerDiffInput)(nil)
|
|
}
|
|
r := new(LowerDiffInput)
|
|
r.Input = m.Input
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *LowerDiffInput) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *UpperDiffInput) CloneVT() *UpperDiffInput {
|
|
if m == nil {
|
|
return (*UpperDiffInput)(nil)
|
|
}
|
|
r := new(UpperDiffInput)
|
|
r.Input = m.Input
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *UpperDiffInput) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (m *DiffOp) CloneVT() *DiffOp {
|
|
if m == nil {
|
|
return (*DiffOp)(nil)
|
|
}
|
|
r := new(DiffOp)
|
|
r.Lower = m.Lower.CloneVT()
|
|
r.Upper = m.Upper.CloneVT()
|
|
if len(m.unknownFields) > 0 {
|
|
r.unknownFields = make([]byte, len(m.unknownFields))
|
|
copy(r.unknownFields, m.unknownFields)
|
|
}
|
|
return r
|
|
}
|
|
|
|
func (m *DiffOp) CloneMessageVT() proto.Message {
|
|
return m.CloneVT()
|
|
}
|
|
|
|
func (this *Op) EqualVT(that *Op) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Op == nil && that.Op != nil {
|
|
return false
|
|
} else if this.Op != nil {
|
|
if that.Op == nil {
|
|
return false
|
|
}
|
|
if !this.Op.(interface{ EqualVT(isOp_Op) bool }).EqualVT(that.Op) {
|
|
return false
|
|
}
|
|
}
|
|
if len(this.Inputs) != len(that.Inputs) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Inputs {
|
|
vy := that.Inputs[i]
|
|
if p, q := vx, vy; p != q {
|
|
if p == nil {
|
|
p = &Input{}
|
|
}
|
|
if q == nil {
|
|
q = &Input{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
}
|
|
if !this.Platform.EqualVT(that.Platform) {
|
|
return false
|
|
}
|
|
if !this.Constraints.EqualVT(that.Constraints) {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *Op) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*Op)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *Op_Exec) EqualVT(thatIface isOp_Op) bool {
|
|
that, ok := thatIface.(*Op_Exec)
|
|
if !ok {
|
|
return false
|
|
}
|
|
if this == that {
|
|
return true
|
|
}
|
|
if this == nil && that != nil || this != nil && that == nil {
|
|
return false
|
|
}
|
|
if p, q := this.Exec, that.Exec; p != q {
|
|
if p == nil {
|
|
p = &ExecOp{}
|
|
}
|
|
if q == nil {
|
|
q = &ExecOp{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (this *Op_Source) EqualVT(thatIface isOp_Op) bool {
|
|
that, ok := thatIface.(*Op_Source)
|
|
if !ok {
|
|
return false
|
|
}
|
|
if this == that {
|
|
return true
|
|
}
|
|
if this == nil && that != nil || this != nil && that == nil {
|
|
return false
|
|
}
|
|
if p, q := this.Source, that.Source; p != q {
|
|
if p == nil {
|
|
p = &SourceOp{}
|
|
}
|
|
if q == nil {
|
|
q = &SourceOp{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (this *Op_File) EqualVT(thatIface isOp_Op) bool {
|
|
that, ok := thatIface.(*Op_File)
|
|
if !ok {
|
|
return false
|
|
}
|
|
if this == that {
|
|
return true
|
|
}
|
|
if this == nil && that != nil || this != nil && that == nil {
|
|
return false
|
|
}
|
|
if p, q := this.File, that.File; p != q {
|
|
if p == nil {
|
|
p = &FileOp{}
|
|
}
|
|
if q == nil {
|
|
q = &FileOp{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (this *Op_Build) EqualVT(thatIface isOp_Op) bool {
|
|
that, ok := thatIface.(*Op_Build)
|
|
if !ok {
|
|
return false
|
|
}
|
|
if this == that {
|
|
return true
|
|
}
|
|
if this == nil && that != nil || this != nil && that == nil {
|
|
return false
|
|
}
|
|
if p, q := this.Build, that.Build; p != q {
|
|
if p == nil {
|
|
p = &BuildOp{}
|
|
}
|
|
if q == nil {
|
|
q = &BuildOp{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (this *Op_Merge) EqualVT(thatIface isOp_Op) bool {
|
|
that, ok := thatIface.(*Op_Merge)
|
|
if !ok {
|
|
return false
|
|
}
|
|
if this == that {
|
|
return true
|
|
}
|
|
if this == nil && that != nil || this != nil && that == nil {
|
|
return false
|
|
}
|
|
if p, q := this.Merge, that.Merge; p != q {
|
|
if p == nil {
|
|
p = &MergeOp{}
|
|
}
|
|
if q == nil {
|
|
q = &MergeOp{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (this *Op_Diff) EqualVT(thatIface isOp_Op) bool {
|
|
that, ok := thatIface.(*Op_Diff)
|
|
if !ok {
|
|
return false
|
|
}
|
|
if this == that {
|
|
return true
|
|
}
|
|
if this == nil && that != nil || this != nil && that == nil {
|
|
return false
|
|
}
|
|
if p, q := this.Diff, that.Diff; p != q {
|
|
if p == nil {
|
|
p = &DiffOp{}
|
|
}
|
|
if q == nil {
|
|
q = &DiffOp{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (this *Platform) EqualVT(that *Platform) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Architecture != that.Architecture {
|
|
return false
|
|
}
|
|
if this.OS != that.OS {
|
|
return false
|
|
}
|
|
if this.Variant != that.Variant {
|
|
return false
|
|
}
|
|
if this.OSVersion != that.OSVersion {
|
|
return false
|
|
}
|
|
if len(this.OSFeatures) != len(that.OSFeatures) {
|
|
return false
|
|
}
|
|
for i, vx := range this.OSFeatures {
|
|
vy := that.OSFeatures[i]
|
|
if vx != vy {
|
|
return false
|
|
}
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *Platform) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*Platform)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *Input) EqualVT(that *Input) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Digest != that.Digest {
|
|
return false
|
|
}
|
|
if this.Index != that.Index {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *Input) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*Input)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *ExecOp) EqualVT(that *ExecOp) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if !this.Meta.EqualVT(that.Meta) {
|
|
return false
|
|
}
|
|
if len(this.Mounts) != len(that.Mounts) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Mounts {
|
|
vy := that.Mounts[i]
|
|
if p, q := vx, vy; p != q {
|
|
if p == nil {
|
|
p = &Mount{}
|
|
}
|
|
if q == nil {
|
|
q = &Mount{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
}
|
|
if this.Network != that.Network {
|
|
return false
|
|
}
|
|
if this.Security != that.Security {
|
|
return false
|
|
}
|
|
if len(this.Secretenv) != len(that.Secretenv) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Secretenv {
|
|
vy := that.Secretenv[i]
|
|
if p, q := vx, vy; p != q {
|
|
if p == nil {
|
|
p = &SecretEnv{}
|
|
}
|
|
if q == nil {
|
|
q = &SecretEnv{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *ExecOp) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*ExecOp)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *Meta) EqualVT(that *Meta) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if len(this.Args) != len(that.Args) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Args {
|
|
vy := that.Args[i]
|
|
if vx != vy {
|
|
return false
|
|
}
|
|
}
|
|
if len(this.Env) != len(that.Env) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Env {
|
|
vy := that.Env[i]
|
|
if vx != vy {
|
|
return false
|
|
}
|
|
}
|
|
if this.Cwd != that.Cwd {
|
|
return false
|
|
}
|
|
if this.User != that.User {
|
|
return false
|
|
}
|
|
if !this.ProxyEnv.EqualVT(that.ProxyEnv) {
|
|
return false
|
|
}
|
|
if len(this.ExtraHosts) != len(that.ExtraHosts) {
|
|
return false
|
|
}
|
|
for i, vx := range this.ExtraHosts {
|
|
vy := that.ExtraHosts[i]
|
|
if p, q := vx, vy; p != q {
|
|
if p == nil {
|
|
p = &HostIP{}
|
|
}
|
|
if q == nil {
|
|
q = &HostIP{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
}
|
|
if this.Hostname != that.Hostname {
|
|
return false
|
|
}
|
|
if len(this.Ulimit) != len(that.Ulimit) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Ulimit {
|
|
vy := that.Ulimit[i]
|
|
if p, q := vx, vy; p != q {
|
|
if p == nil {
|
|
p = &Ulimit{}
|
|
}
|
|
if q == nil {
|
|
q = &Ulimit{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
}
|
|
if this.CgroupParent != that.CgroupParent {
|
|
return false
|
|
}
|
|
if this.RemoveMountStubsRecursive != that.RemoveMountStubsRecursive {
|
|
return false
|
|
}
|
|
if len(this.ValidExitCodes) != len(that.ValidExitCodes) {
|
|
return false
|
|
}
|
|
for i, vx := range this.ValidExitCodes {
|
|
vy := that.ValidExitCodes[i]
|
|
if vx != vy {
|
|
return false
|
|
}
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *Meta) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*Meta)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *HostIP) EqualVT(that *HostIP) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Host != that.Host {
|
|
return false
|
|
}
|
|
if this.IP != that.IP {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *HostIP) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*HostIP)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *Ulimit) EqualVT(that *Ulimit) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Name != that.Name {
|
|
return false
|
|
}
|
|
if this.Soft != that.Soft {
|
|
return false
|
|
}
|
|
if this.Hard != that.Hard {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *Ulimit) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*Ulimit)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *SecretEnv) EqualVT(that *SecretEnv) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.ID != that.ID {
|
|
return false
|
|
}
|
|
if this.Name != that.Name {
|
|
return false
|
|
}
|
|
if this.Optional != that.Optional {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *SecretEnv) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*SecretEnv)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *Mount) EqualVT(that *Mount) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Input != that.Input {
|
|
return false
|
|
}
|
|
if this.Selector != that.Selector {
|
|
return false
|
|
}
|
|
if this.Dest != that.Dest {
|
|
return false
|
|
}
|
|
if this.Output != that.Output {
|
|
return false
|
|
}
|
|
if this.Readonly != that.Readonly {
|
|
return false
|
|
}
|
|
if this.MountType != that.MountType {
|
|
return false
|
|
}
|
|
if !this.TmpfsOpt.EqualVT(that.TmpfsOpt) {
|
|
return false
|
|
}
|
|
if !this.CacheOpt.EqualVT(that.CacheOpt) {
|
|
return false
|
|
}
|
|
if !this.SecretOpt.EqualVT(that.SecretOpt) {
|
|
return false
|
|
}
|
|
if !this.SSHOpt.EqualVT(that.SSHOpt) {
|
|
return false
|
|
}
|
|
if this.ResultID != that.ResultID {
|
|
return false
|
|
}
|
|
if this.ContentCache != that.ContentCache {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *Mount) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*Mount)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *TmpfsOpt) EqualVT(that *TmpfsOpt) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Size != that.Size {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *TmpfsOpt) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*TmpfsOpt)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *CacheOpt) EqualVT(that *CacheOpt) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.ID != that.ID {
|
|
return false
|
|
}
|
|
if this.Sharing != that.Sharing {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *CacheOpt) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*CacheOpt)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *SecretOpt) EqualVT(that *SecretOpt) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.ID != that.ID {
|
|
return false
|
|
}
|
|
if this.Uid != that.Uid {
|
|
return false
|
|
}
|
|
if this.Gid != that.Gid {
|
|
return false
|
|
}
|
|
if this.Mode != that.Mode {
|
|
return false
|
|
}
|
|
if this.Optional != that.Optional {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *SecretOpt) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*SecretOpt)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *SSHOpt) EqualVT(that *SSHOpt) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.ID != that.ID {
|
|
return false
|
|
}
|
|
if this.Uid != that.Uid {
|
|
return false
|
|
}
|
|
if this.Gid != that.Gid {
|
|
return false
|
|
}
|
|
if this.Mode != that.Mode {
|
|
return false
|
|
}
|
|
if this.Optional != that.Optional {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *SSHOpt) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*SSHOpt)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *SourceOp) EqualVT(that *SourceOp) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Identifier != that.Identifier {
|
|
return false
|
|
}
|
|
if len(this.Attrs) != len(that.Attrs) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Attrs {
|
|
vy, ok := that.Attrs[i]
|
|
if !ok {
|
|
return false
|
|
}
|
|
if vx != vy {
|
|
return false
|
|
}
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *SourceOp) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*SourceOp)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *BuildOp) EqualVT(that *BuildOp) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Builder != that.Builder {
|
|
return false
|
|
}
|
|
if len(this.Inputs) != len(that.Inputs) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Inputs {
|
|
vy, ok := that.Inputs[i]
|
|
if !ok {
|
|
return false
|
|
}
|
|
if p, q := vx, vy; p != q {
|
|
if p == nil {
|
|
p = &BuildInput{}
|
|
}
|
|
if q == nil {
|
|
q = &BuildInput{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
}
|
|
if !this.Def.EqualVT(that.Def) {
|
|
return false
|
|
}
|
|
if len(this.Attrs) != len(that.Attrs) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Attrs {
|
|
vy, ok := that.Attrs[i]
|
|
if !ok {
|
|
return false
|
|
}
|
|
if vx != vy {
|
|
return false
|
|
}
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *BuildOp) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*BuildOp)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *BuildInput) EqualVT(that *BuildInput) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Input != that.Input {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *BuildInput) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*BuildInput)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *OpMetadata) EqualVT(that *OpMetadata) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.IgnoreCache != that.IgnoreCache {
|
|
return false
|
|
}
|
|
if len(this.Description) != len(that.Description) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Description {
|
|
vy, ok := that.Description[i]
|
|
if !ok {
|
|
return false
|
|
}
|
|
if vx != vy {
|
|
return false
|
|
}
|
|
}
|
|
if !this.ExportCache.EqualVT(that.ExportCache) {
|
|
return false
|
|
}
|
|
if len(this.Caps) != len(that.Caps) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Caps {
|
|
vy, ok := that.Caps[i]
|
|
if !ok {
|
|
return false
|
|
}
|
|
if vx != vy {
|
|
return false
|
|
}
|
|
}
|
|
if !this.ProgressGroup.EqualVT(that.ProgressGroup) {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *OpMetadata) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*OpMetadata)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *Source) EqualVT(that *Source) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if len(this.Locations) != len(that.Locations) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Locations {
|
|
vy, ok := that.Locations[i]
|
|
if !ok {
|
|
return false
|
|
}
|
|
if p, q := vx, vy; p != q {
|
|
if p == nil {
|
|
p = &Locations{}
|
|
}
|
|
if q == nil {
|
|
q = &Locations{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
}
|
|
if len(this.Infos) != len(that.Infos) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Infos {
|
|
vy := that.Infos[i]
|
|
if p, q := vx, vy; p != q {
|
|
if p == nil {
|
|
p = &SourceInfo{}
|
|
}
|
|
if q == nil {
|
|
q = &SourceInfo{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *Source) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*Source)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *Locations) EqualVT(that *Locations) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if len(this.Locations) != len(that.Locations) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Locations {
|
|
vy := that.Locations[i]
|
|
if p, q := vx, vy; p != q {
|
|
if p == nil {
|
|
p = &Location{}
|
|
}
|
|
if q == nil {
|
|
q = &Location{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *Locations) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*Locations)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *SourceInfo) EqualVT(that *SourceInfo) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Filename != that.Filename {
|
|
return false
|
|
}
|
|
if string(this.Data) != string(that.Data) {
|
|
return false
|
|
}
|
|
if !this.Definition.EqualVT(that.Definition) {
|
|
return false
|
|
}
|
|
if this.Language != that.Language {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *SourceInfo) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*SourceInfo)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *Location) EqualVT(that *Location) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.SourceIndex != that.SourceIndex {
|
|
return false
|
|
}
|
|
if len(this.Ranges) != len(that.Ranges) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Ranges {
|
|
vy := that.Ranges[i]
|
|
if p, q := vx, vy; p != q {
|
|
if p == nil {
|
|
p = &Range{}
|
|
}
|
|
if q == nil {
|
|
q = &Range{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *Location) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*Location)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *Range) EqualVT(that *Range) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if !this.Start.EqualVT(that.Start) {
|
|
return false
|
|
}
|
|
if !this.End.EqualVT(that.End) {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *Range) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*Range)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *Position) EqualVT(that *Position) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Line != that.Line {
|
|
return false
|
|
}
|
|
if this.Character != that.Character {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *Position) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*Position)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *ExportCache) EqualVT(that *ExportCache) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Value != that.Value {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *ExportCache) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*ExportCache)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *ProgressGroup) EqualVT(that *ProgressGroup) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Id != that.Id {
|
|
return false
|
|
}
|
|
if this.Name != that.Name {
|
|
return false
|
|
}
|
|
if this.Weak != that.Weak {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *ProgressGroup) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*ProgressGroup)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *ProxyEnv) EqualVT(that *ProxyEnv) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.HttpProxy != that.HttpProxy {
|
|
return false
|
|
}
|
|
if this.HttpsProxy != that.HttpsProxy {
|
|
return false
|
|
}
|
|
if this.FtpProxy != that.FtpProxy {
|
|
return false
|
|
}
|
|
if this.NoProxy != that.NoProxy {
|
|
return false
|
|
}
|
|
if this.AllProxy != that.AllProxy {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *ProxyEnv) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*ProxyEnv)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *WorkerConstraints) EqualVT(that *WorkerConstraints) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if len(this.Filter) != len(that.Filter) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Filter {
|
|
vy := that.Filter[i]
|
|
if vx != vy {
|
|
return false
|
|
}
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *WorkerConstraints) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*WorkerConstraints)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *Definition) EqualVT(that *Definition) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if len(this.Def) != len(that.Def) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Def {
|
|
vy := that.Def[i]
|
|
if string(vx) != string(vy) {
|
|
return false
|
|
}
|
|
}
|
|
if len(this.Metadata) != len(that.Metadata) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Metadata {
|
|
vy, ok := that.Metadata[i]
|
|
if !ok {
|
|
return false
|
|
}
|
|
if p, q := vx, vy; p != q {
|
|
if p == nil {
|
|
p = &OpMetadata{}
|
|
}
|
|
if q == nil {
|
|
q = &OpMetadata{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
}
|
|
if !this.Source.EqualVT(that.Source) {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *Definition) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*Definition)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *FileOp) EqualVT(that *FileOp) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if len(this.Actions) != len(that.Actions) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Actions {
|
|
vy := that.Actions[i]
|
|
if p, q := vx, vy; p != q {
|
|
if p == nil {
|
|
p = &FileAction{}
|
|
}
|
|
if q == nil {
|
|
q = &FileAction{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *FileOp) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*FileOp)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *FileAction) EqualVT(that *FileAction) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Action == nil && that.Action != nil {
|
|
return false
|
|
} else if this.Action != nil {
|
|
if that.Action == nil {
|
|
return false
|
|
}
|
|
if !this.Action.(interface {
|
|
EqualVT(isFileAction_Action) bool
|
|
}).EqualVT(that.Action) {
|
|
return false
|
|
}
|
|
}
|
|
if this.Input != that.Input {
|
|
return false
|
|
}
|
|
if this.SecondaryInput != that.SecondaryInput {
|
|
return false
|
|
}
|
|
if this.Output != that.Output {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *FileAction) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*FileAction)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *FileAction_Copy) EqualVT(thatIface isFileAction_Action) bool {
|
|
that, ok := thatIface.(*FileAction_Copy)
|
|
if !ok {
|
|
return false
|
|
}
|
|
if this == that {
|
|
return true
|
|
}
|
|
if this == nil && that != nil || this != nil && that == nil {
|
|
return false
|
|
}
|
|
if p, q := this.Copy, that.Copy; p != q {
|
|
if p == nil {
|
|
p = &FileActionCopy{}
|
|
}
|
|
if q == nil {
|
|
q = &FileActionCopy{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (this *FileAction_Mkfile) EqualVT(thatIface isFileAction_Action) bool {
|
|
that, ok := thatIface.(*FileAction_Mkfile)
|
|
if !ok {
|
|
return false
|
|
}
|
|
if this == that {
|
|
return true
|
|
}
|
|
if this == nil && that != nil || this != nil && that == nil {
|
|
return false
|
|
}
|
|
if p, q := this.Mkfile, that.Mkfile; p != q {
|
|
if p == nil {
|
|
p = &FileActionMkFile{}
|
|
}
|
|
if q == nil {
|
|
q = &FileActionMkFile{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (this *FileAction_Mkdir) EqualVT(thatIface isFileAction_Action) bool {
|
|
that, ok := thatIface.(*FileAction_Mkdir)
|
|
if !ok {
|
|
return false
|
|
}
|
|
if this == that {
|
|
return true
|
|
}
|
|
if this == nil && that != nil || this != nil && that == nil {
|
|
return false
|
|
}
|
|
if p, q := this.Mkdir, that.Mkdir; p != q {
|
|
if p == nil {
|
|
p = &FileActionMkDir{}
|
|
}
|
|
if q == nil {
|
|
q = &FileActionMkDir{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (this *FileAction_Rm) EqualVT(thatIface isFileAction_Action) bool {
|
|
that, ok := thatIface.(*FileAction_Rm)
|
|
if !ok {
|
|
return false
|
|
}
|
|
if this == that {
|
|
return true
|
|
}
|
|
if this == nil && that != nil || this != nil && that == nil {
|
|
return false
|
|
}
|
|
if p, q := this.Rm, that.Rm; p != q {
|
|
if p == nil {
|
|
p = &FileActionRm{}
|
|
}
|
|
if q == nil {
|
|
q = &FileActionRm{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (this *FileActionCopy) EqualVT(that *FileActionCopy) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Src != that.Src {
|
|
return false
|
|
}
|
|
if this.Dest != that.Dest {
|
|
return false
|
|
}
|
|
if !this.Owner.EqualVT(that.Owner) {
|
|
return false
|
|
}
|
|
if this.Mode != that.Mode {
|
|
return false
|
|
}
|
|
if this.FollowSymlink != that.FollowSymlink {
|
|
return false
|
|
}
|
|
if this.DirCopyContents != that.DirCopyContents {
|
|
return false
|
|
}
|
|
if this.AttemptUnpackDockerCompatibility != that.AttemptUnpackDockerCompatibility {
|
|
return false
|
|
}
|
|
if this.CreateDestPath != that.CreateDestPath {
|
|
return false
|
|
}
|
|
if this.AllowWildcard != that.AllowWildcard {
|
|
return false
|
|
}
|
|
if this.AllowEmptyWildcard != that.AllowEmptyWildcard {
|
|
return false
|
|
}
|
|
if this.Timestamp != that.Timestamp {
|
|
return false
|
|
}
|
|
if len(this.IncludePatterns) != len(that.IncludePatterns) {
|
|
return false
|
|
}
|
|
for i, vx := range this.IncludePatterns {
|
|
vy := that.IncludePatterns[i]
|
|
if vx != vy {
|
|
return false
|
|
}
|
|
}
|
|
if len(this.ExcludePatterns) != len(that.ExcludePatterns) {
|
|
return false
|
|
}
|
|
for i, vx := range this.ExcludePatterns {
|
|
vy := that.ExcludePatterns[i]
|
|
if vx != vy {
|
|
return false
|
|
}
|
|
}
|
|
if this.AlwaysReplaceExistingDestPaths != that.AlwaysReplaceExistingDestPaths {
|
|
return false
|
|
}
|
|
if this.ModeStr != that.ModeStr {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *FileActionCopy) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*FileActionCopy)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *FileActionMkFile) EqualVT(that *FileActionMkFile) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Path != that.Path {
|
|
return false
|
|
}
|
|
if this.Mode != that.Mode {
|
|
return false
|
|
}
|
|
if string(this.Data) != string(that.Data) {
|
|
return false
|
|
}
|
|
if !this.Owner.EqualVT(that.Owner) {
|
|
return false
|
|
}
|
|
if this.Timestamp != that.Timestamp {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *FileActionMkFile) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*FileActionMkFile)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *FileActionMkDir) EqualVT(that *FileActionMkDir) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Path != that.Path {
|
|
return false
|
|
}
|
|
if this.Mode != that.Mode {
|
|
return false
|
|
}
|
|
if this.MakeParents != that.MakeParents {
|
|
return false
|
|
}
|
|
if !this.Owner.EqualVT(that.Owner) {
|
|
return false
|
|
}
|
|
if this.Timestamp != that.Timestamp {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *FileActionMkDir) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*FileActionMkDir)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *FileActionRm) EqualVT(that *FileActionRm) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Path != that.Path {
|
|
return false
|
|
}
|
|
if this.AllowNotFound != that.AllowNotFound {
|
|
return false
|
|
}
|
|
if this.AllowWildcard != that.AllowWildcard {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *FileActionRm) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*FileActionRm)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *ChownOpt) EqualVT(that *ChownOpt) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if !this.User.EqualVT(that.User) {
|
|
return false
|
|
}
|
|
if !this.Group.EqualVT(that.Group) {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *ChownOpt) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*ChownOpt)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *UserOpt) EqualVT(that *UserOpt) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.User == nil && that.User != nil {
|
|
return false
|
|
} else if this.User != nil {
|
|
if that.User == nil {
|
|
return false
|
|
}
|
|
if !this.User.(interface{ EqualVT(isUserOpt_User) bool }).EqualVT(that.User) {
|
|
return false
|
|
}
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *UserOpt) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*UserOpt)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *UserOpt_ByName) EqualVT(thatIface isUserOpt_User) bool {
|
|
that, ok := thatIface.(*UserOpt_ByName)
|
|
if !ok {
|
|
return false
|
|
}
|
|
if this == that {
|
|
return true
|
|
}
|
|
if this == nil && that != nil || this != nil && that == nil {
|
|
return false
|
|
}
|
|
if p, q := this.ByName, that.ByName; p != q {
|
|
if p == nil {
|
|
p = &NamedUserOpt{}
|
|
}
|
|
if q == nil {
|
|
q = &NamedUserOpt{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (this *UserOpt_ByID) EqualVT(thatIface isUserOpt_User) bool {
|
|
that, ok := thatIface.(*UserOpt_ByID)
|
|
if !ok {
|
|
return false
|
|
}
|
|
if this == that {
|
|
return true
|
|
}
|
|
if this == nil && that != nil || this != nil && that == nil {
|
|
return false
|
|
}
|
|
if this.ByID != that.ByID {
|
|
return false
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (this *NamedUserOpt) EqualVT(that *NamedUserOpt) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Name != that.Name {
|
|
return false
|
|
}
|
|
if this.Input != that.Input {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *NamedUserOpt) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*NamedUserOpt)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *MergeInput) EqualVT(that *MergeInput) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Input != that.Input {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *MergeInput) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*MergeInput)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *MergeOp) EqualVT(that *MergeOp) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if len(this.Inputs) != len(that.Inputs) {
|
|
return false
|
|
}
|
|
for i, vx := range this.Inputs {
|
|
vy := that.Inputs[i]
|
|
if p, q := vx, vy; p != q {
|
|
if p == nil {
|
|
p = &MergeInput{}
|
|
}
|
|
if q == nil {
|
|
q = &MergeInput{}
|
|
}
|
|
if !p.EqualVT(q) {
|
|
return false
|
|
}
|
|
}
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *MergeOp) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*MergeOp)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *LowerDiffInput) EqualVT(that *LowerDiffInput) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Input != that.Input {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *LowerDiffInput) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*LowerDiffInput)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *UpperDiffInput) EqualVT(that *UpperDiffInput) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if this.Input != that.Input {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *UpperDiffInput) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*UpperDiffInput)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (this *DiffOp) EqualVT(that *DiffOp) bool {
|
|
if this == that {
|
|
return true
|
|
} else if this == nil || that == nil {
|
|
return false
|
|
}
|
|
if !this.Lower.EqualVT(that.Lower) {
|
|
return false
|
|
}
|
|
if !this.Upper.EqualVT(that.Upper) {
|
|
return false
|
|
}
|
|
return string(this.unknownFields) == string(that.unknownFields)
|
|
}
|
|
|
|
func (this *DiffOp) EqualMessageVT(thatMsg proto.Message) bool {
|
|
that, ok := thatMsg.(*DiffOp)
|
|
if !ok {
|
|
return false
|
|
}
|
|
return this.EqualVT(that)
|
|
}
|
|
func (m *Op) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *Op) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *Op) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if vtmsg, ok := m.Op.(interface {
|
|
MarshalToSizedBufferVT([]byte) (int, error)
|
|
}); ok {
|
|
size, err := vtmsg.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
}
|
|
if m.Constraints != nil {
|
|
size, err := m.Constraints.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x5a
|
|
}
|
|
if m.Platform != nil {
|
|
size, err := m.Platform.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x52
|
|
}
|
|
if len(m.Inputs) > 0 {
|
|
for iNdEx := len(m.Inputs) - 1; iNdEx >= 0; iNdEx-- {
|
|
size, err := m.Inputs[iNdEx].MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *Op_Exec) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *Op_Exec) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
i := len(dAtA)
|
|
if m.Exec != nil {
|
|
size, err := m.Exec.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
} else {
|
|
i = protohelpers.EncodeVarint(dAtA, i, 0)
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
func (m *Op_Source) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *Op_Source) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
i := len(dAtA)
|
|
if m.Source != nil {
|
|
size, err := m.Source.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x1a
|
|
} else {
|
|
i = protohelpers.EncodeVarint(dAtA, i, 0)
|
|
i--
|
|
dAtA[i] = 0x1a
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
func (m *Op_File) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *Op_File) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
i := len(dAtA)
|
|
if m.File != nil {
|
|
size, err := m.File.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x22
|
|
} else {
|
|
i = protohelpers.EncodeVarint(dAtA, i, 0)
|
|
i--
|
|
dAtA[i] = 0x22
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
func (m *Op_Build) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *Op_Build) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
i := len(dAtA)
|
|
if m.Build != nil {
|
|
size, err := m.Build.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x2a
|
|
} else {
|
|
i = protohelpers.EncodeVarint(dAtA, i, 0)
|
|
i--
|
|
dAtA[i] = 0x2a
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
func (m *Op_Merge) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *Op_Merge) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
i := len(dAtA)
|
|
if m.Merge != nil {
|
|
size, err := m.Merge.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x32
|
|
} else {
|
|
i = protohelpers.EncodeVarint(dAtA, i, 0)
|
|
i--
|
|
dAtA[i] = 0x32
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
func (m *Op_Diff) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *Op_Diff) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
i := len(dAtA)
|
|
if m.Diff != nil {
|
|
size, err := m.Diff.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x3a
|
|
} else {
|
|
i = protohelpers.EncodeVarint(dAtA, i, 0)
|
|
i--
|
|
dAtA[i] = 0x3a
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
func (m *Platform) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *Platform) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *Platform) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.OSFeatures) > 0 {
|
|
for iNdEx := len(m.OSFeatures) - 1; iNdEx >= 0; iNdEx-- {
|
|
i -= len(m.OSFeatures[iNdEx])
|
|
copy(dAtA[i:], m.OSFeatures[iNdEx])
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.OSFeatures[iNdEx])))
|
|
i--
|
|
dAtA[i] = 0x2a
|
|
}
|
|
}
|
|
if len(m.OSVersion) > 0 {
|
|
i -= len(m.OSVersion)
|
|
copy(dAtA[i:], m.OSVersion)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.OSVersion)))
|
|
i--
|
|
dAtA[i] = 0x22
|
|
}
|
|
if len(m.Variant) > 0 {
|
|
i -= len(m.Variant)
|
|
copy(dAtA[i:], m.Variant)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Variant)))
|
|
i--
|
|
dAtA[i] = 0x1a
|
|
}
|
|
if len(m.OS) > 0 {
|
|
i -= len(m.OS)
|
|
copy(dAtA[i:], m.OS)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.OS)))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
if len(m.Architecture) > 0 {
|
|
i -= len(m.Architecture)
|
|
copy(dAtA[i:], m.Architecture)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Architecture)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *Input) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *Input) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *Input) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.Index != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Index))
|
|
i--
|
|
dAtA[i] = 0x10
|
|
}
|
|
if len(m.Digest) > 0 {
|
|
i -= len(m.Digest)
|
|
copy(dAtA[i:], m.Digest)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Digest)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *ExecOp) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *ExecOp) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *ExecOp) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.Secretenv) > 0 {
|
|
for iNdEx := len(m.Secretenv) - 1; iNdEx >= 0; iNdEx-- {
|
|
size, err := m.Secretenv[iNdEx].MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x2a
|
|
}
|
|
}
|
|
if m.Security != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Security))
|
|
i--
|
|
dAtA[i] = 0x20
|
|
}
|
|
if m.Network != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Network))
|
|
i--
|
|
dAtA[i] = 0x18
|
|
}
|
|
if len(m.Mounts) > 0 {
|
|
for iNdEx := len(m.Mounts) - 1; iNdEx >= 0; iNdEx-- {
|
|
size, err := m.Mounts[iNdEx].MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
}
|
|
if m.Meta != nil {
|
|
size, err := m.Meta.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *Meta) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *Meta) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *Meta) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.ValidExitCodes) > 0 {
|
|
var pksize2 int
|
|
for _, num := range m.ValidExitCodes {
|
|
pksize2 += protohelpers.SizeOfVarint(uint64(num))
|
|
}
|
|
i -= pksize2
|
|
j1 := i
|
|
for _, num1 := range m.ValidExitCodes {
|
|
num := uint64(num1)
|
|
for num >= 1<<7 {
|
|
dAtA[j1] = uint8(uint64(num)&0x7f | 0x80)
|
|
num >>= 7
|
|
j1++
|
|
}
|
|
dAtA[j1] = uint8(num)
|
|
j1++
|
|
}
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(pksize2))
|
|
i--
|
|
dAtA[i] = 0x62
|
|
}
|
|
if m.RemoveMountStubsRecursive {
|
|
i--
|
|
if m.RemoveMountStubsRecursive {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x58
|
|
}
|
|
if len(m.CgroupParent) > 0 {
|
|
i -= len(m.CgroupParent)
|
|
copy(dAtA[i:], m.CgroupParent)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.CgroupParent)))
|
|
i--
|
|
dAtA[i] = 0x52
|
|
}
|
|
if len(m.Ulimit) > 0 {
|
|
for iNdEx := len(m.Ulimit) - 1; iNdEx >= 0; iNdEx-- {
|
|
size, err := m.Ulimit[iNdEx].MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x4a
|
|
}
|
|
}
|
|
if len(m.Hostname) > 0 {
|
|
i -= len(m.Hostname)
|
|
copy(dAtA[i:], m.Hostname)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Hostname)))
|
|
i--
|
|
dAtA[i] = 0x3a
|
|
}
|
|
if len(m.ExtraHosts) > 0 {
|
|
for iNdEx := len(m.ExtraHosts) - 1; iNdEx >= 0; iNdEx-- {
|
|
size, err := m.ExtraHosts[iNdEx].MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x32
|
|
}
|
|
}
|
|
if m.ProxyEnv != nil {
|
|
size, err := m.ProxyEnv.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x2a
|
|
}
|
|
if len(m.User) > 0 {
|
|
i -= len(m.User)
|
|
copy(dAtA[i:], m.User)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.User)))
|
|
i--
|
|
dAtA[i] = 0x22
|
|
}
|
|
if len(m.Cwd) > 0 {
|
|
i -= len(m.Cwd)
|
|
copy(dAtA[i:], m.Cwd)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Cwd)))
|
|
i--
|
|
dAtA[i] = 0x1a
|
|
}
|
|
if len(m.Env) > 0 {
|
|
for iNdEx := len(m.Env) - 1; iNdEx >= 0; iNdEx-- {
|
|
i -= len(m.Env[iNdEx])
|
|
copy(dAtA[i:], m.Env[iNdEx])
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Env[iNdEx])))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
}
|
|
if len(m.Args) > 0 {
|
|
for iNdEx := len(m.Args) - 1; iNdEx >= 0; iNdEx-- {
|
|
i -= len(m.Args[iNdEx])
|
|
copy(dAtA[i:], m.Args[iNdEx])
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Args[iNdEx])))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *HostIP) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *HostIP) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *HostIP) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.IP) > 0 {
|
|
i -= len(m.IP)
|
|
copy(dAtA[i:], m.IP)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.IP)))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
if len(m.Host) > 0 {
|
|
i -= len(m.Host)
|
|
copy(dAtA[i:], m.Host)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Host)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *Ulimit) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *Ulimit) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *Ulimit) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.Hard != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Hard))
|
|
i--
|
|
dAtA[i] = 0x18
|
|
}
|
|
if m.Soft != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Soft))
|
|
i--
|
|
dAtA[i] = 0x10
|
|
}
|
|
if len(m.Name) > 0 {
|
|
i -= len(m.Name)
|
|
copy(dAtA[i:], m.Name)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Name)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *SecretEnv) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *SecretEnv) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *SecretEnv) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.Optional {
|
|
i--
|
|
if m.Optional {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x18
|
|
}
|
|
if len(m.Name) > 0 {
|
|
i -= len(m.Name)
|
|
copy(dAtA[i:], m.Name)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Name)))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
if len(m.ID) > 0 {
|
|
i -= len(m.ID)
|
|
copy(dAtA[i:], m.ID)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.ID)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *Mount) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *Mount) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *Mount) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.ContentCache != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.ContentCache))
|
|
i--
|
|
dAtA[i] = 0x1
|
|
i--
|
|
dAtA[i] = 0xc0
|
|
}
|
|
if len(m.ResultID) > 0 {
|
|
i -= len(m.ResultID)
|
|
copy(dAtA[i:], m.ResultID)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.ResultID)))
|
|
i--
|
|
dAtA[i] = 0x1
|
|
i--
|
|
dAtA[i] = 0xba
|
|
}
|
|
if m.SSHOpt != nil {
|
|
size, err := m.SSHOpt.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x1
|
|
i--
|
|
dAtA[i] = 0xb2
|
|
}
|
|
if m.SecretOpt != nil {
|
|
size, err := m.SecretOpt.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x1
|
|
i--
|
|
dAtA[i] = 0xaa
|
|
}
|
|
if m.CacheOpt != nil {
|
|
size, err := m.CacheOpt.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x1
|
|
i--
|
|
dAtA[i] = 0xa2
|
|
}
|
|
if m.TmpfsOpt != nil {
|
|
size, err := m.TmpfsOpt.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x1
|
|
i--
|
|
dAtA[i] = 0x9a
|
|
}
|
|
if m.MountType != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.MountType))
|
|
i--
|
|
dAtA[i] = 0x30
|
|
}
|
|
if m.Readonly {
|
|
i--
|
|
if m.Readonly {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x28
|
|
}
|
|
if m.Output != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Output))
|
|
i--
|
|
dAtA[i] = 0x20
|
|
}
|
|
if len(m.Dest) > 0 {
|
|
i -= len(m.Dest)
|
|
copy(dAtA[i:], m.Dest)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Dest)))
|
|
i--
|
|
dAtA[i] = 0x1a
|
|
}
|
|
if len(m.Selector) > 0 {
|
|
i -= len(m.Selector)
|
|
copy(dAtA[i:], m.Selector)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Selector)))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
if m.Input != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Input))
|
|
i--
|
|
dAtA[i] = 0x8
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *TmpfsOpt) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *TmpfsOpt) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *TmpfsOpt) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.Size != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Size))
|
|
i--
|
|
dAtA[i] = 0x8
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *CacheOpt) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *CacheOpt) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *CacheOpt) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.Sharing != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Sharing))
|
|
i--
|
|
dAtA[i] = 0x10
|
|
}
|
|
if len(m.ID) > 0 {
|
|
i -= len(m.ID)
|
|
copy(dAtA[i:], m.ID)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.ID)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *SecretOpt) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *SecretOpt) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *SecretOpt) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.Optional {
|
|
i--
|
|
if m.Optional {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x28
|
|
}
|
|
if m.Mode != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Mode))
|
|
i--
|
|
dAtA[i] = 0x20
|
|
}
|
|
if m.Gid != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Gid))
|
|
i--
|
|
dAtA[i] = 0x18
|
|
}
|
|
if m.Uid != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Uid))
|
|
i--
|
|
dAtA[i] = 0x10
|
|
}
|
|
if len(m.ID) > 0 {
|
|
i -= len(m.ID)
|
|
copy(dAtA[i:], m.ID)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.ID)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *SSHOpt) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *SSHOpt) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *SSHOpt) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.Optional {
|
|
i--
|
|
if m.Optional {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x28
|
|
}
|
|
if m.Mode != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Mode))
|
|
i--
|
|
dAtA[i] = 0x20
|
|
}
|
|
if m.Gid != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Gid))
|
|
i--
|
|
dAtA[i] = 0x18
|
|
}
|
|
if m.Uid != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Uid))
|
|
i--
|
|
dAtA[i] = 0x10
|
|
}
|
|
if len(m.ID) > 0 {
|
|
i -= len(m.ID)
|
|
copy(dAtA[i:], m.ID)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.ID)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *SourceOp) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *SourceOp) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *SourceOp) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.Attrs) > 0 {
|
|
for k := range m.Attrs {
|
|
v := m.Attrs[k]
|
|
baseI := i
|
|
i -= len(v)
|
|
copy(dAtA[i:], v)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(v)))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
i -= len(k)
|
|
copy(dAtA[i:], k)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(k)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(baseI-i))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
}
|
|
if len(m.Identifier) > 0 {
|
|
i -= len(m.Identifier)
|
|
copy(dAtA[i:], m.Identifier)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Identifier)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *BuildOp) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *BuildOp) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *BuildOp) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.Attrs) > 0 {
|
|
for k := range m.Attrs {
|
|
v := m.Attrs[k]
|
|
baseI := i
|
|
i -= len(v)
|
|
copy(dAtA[i:], v)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(v)))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
i -= len(k)
|
|
copy(dAtA[i:], k)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(k)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(baseI-i))
|
|
i--
|
|
dAtA[i] = 0x22
|
|
}
|
|
}
|
|
if m.Def != nil {
|
|
size, err := m.Def.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x1a
|
|
}
|
|
if len(m.Inputs) > 0 {
|
|
for k := range m.Inputs {
|
|
v := m.Inputs[k]
|
|
baseI := i
|
|
size, err := v.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
i -= len(k)
|
|
copy(dAtA[i:], k)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(k)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(baseI-i))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
}
|
|
if m.Builder != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Builder))
|
|
i--
|
|
dAtA[i] = 0x8
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *BuildInput) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *BuildInput) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *BuildInput) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.Input != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Input))
|
|
i--
|
|
dAtA[i] = 0x8
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *OpMetadata) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *OpMetadata) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *OpMetadata) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.ProgressGroup != nil {
|
|
size, err := m.ProgressGroup.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x32
|
|
}
|
|
if len(m.Caps) > 0 {
|
|
for k := range m.Caps {
|
|
v := m.Caps[k]
|
|
baseI := i
|
|
i--
|
|
if v {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x10
|
|
i -= len(k)
|
|
copy(dAtA[i:], k)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(k)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(baseI-i))
|
|
i--
|
|
dAtA[i] = 0x2a
|
|
}
|
|
}
|
|
if m.ExportCache != nil {
|
|
size, err := m.ExportCache.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x22
|
|
}
|
|
if len(m.Description) > 0 {
|
|
for k := range m.Description {
|
|
v := m.Description[k]
|
|
baseI := i
|
|
i -= len(v)
|
|
copy(dAtA[i:], v)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(v)))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
i -= len(k)
|
|
copy(dAtA[i:], k)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(k)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(baseI-i))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
}
|
|
if m.IgnoreCache {
|
|
i--
|
|
if m.IgnoreCache {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x8
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *Source) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *Source) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *Source) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.Infos) > 0 {
|
|
for iNdEx := len(m.Infos) - 1; iNdEx >= 0; iNdEx-- {
|
|
size, err := m.Infos[iNdEx].MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
}
|
|
if len(m.Locations) > 0 {
|
|
for k := range m.Locations {
|
|
v := m.Locations[k]
|
|
baseI := i
|
|
size, err := v.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
i -= len(k)
|
|
copy(dAtA[i:], k)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(k)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(baseI-i))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *Locations) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *Locations) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *Locations) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.Locations) > 0 {
|
|
for iNdEx := len(m.Locations) - 1; iNdEx >= 0; iNdEx-- {
|
|
size, err := m.Locations[iNdEx].MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *SourceInfo) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *SourceInfo) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *SourceInfo) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.Language) > 0 {
|
|
i -= len(m.Language)
|
|
copy(dAtA[i:], m.Language)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Language)))
|
|
i--
|
|
dAtA[i] = 0x22
|
|
}
|
|
if m.Definition != nil {
|
|
size, err := m.Definition.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x1a
|
|
}
|
|
if len(m.Data) > 0 {
|
|
i -= len(m.Data)
|
|
copy(dAtA[i:], m.Data)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Data)))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
if len(m.Filename) > 0 {
|
|
i -= len(m.Filename)
|
|
copy(dAtA[i:], m.Filename)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Filename)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *Location) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *Location) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *Location) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.Ranges) > 0 {
|
|
for iNdEx := len(m.Ranges) - 1; iNdEx >= 0; iNdEx-- {
|
|
size, err := m.Ranges[iNdEx].MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
}
|
|
if m.SourceIndex != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.SourceIndex))
|
|
i--
|
|
dAtA[i] = 0x8
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *Range) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *Range) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *Range) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.End != nil {
|
|
size, err := m.End.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
if m.Start != nil {
|
|
size, err := m.Start.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *Position) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *Position) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *Position) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.Character != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Character))
|
|
i--
|
|
dAtA[i] = 0x10
|
|
}
|
|
if m.Line != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Line))
|
|
i--
|
|
dAtA[i] = 0x8
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *ExportCache) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *ExportCache) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *ExportCache) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.Value {
|
|
i--
|
|
if m.Value {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x8
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *ProgressGroup) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *ProgressGroup) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *ProgressGroup) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.Weak {
|
|
i--
|
|
if m.Weak {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x18
|
|
}
|
|
if len(m.Name) > 0 {
|
|
i -= len(m.Name)
|
|
copy(dAtA[i:], m.Name)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Name)))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
if len(m.Id) > 0 {
|
|
i -= len(m.Id)
|
|
copy(dAtA[i:], m.Id)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Id)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *ProxyEnv) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *ProxyEnv) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *ProxyEnv) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.AllProxy) > 0 {
|
|
i -= len(m.AllProxy)
|
|
copy(dAtA[i:], m.AllProxy)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.AllProxy)))
|
|
i--
|
|
dAtA[i] = 0x2a
|
|
}
|
|
if len(m.NoProxy) > 0 {
|
|
i -= len(m.NoProxy)
|
|
copy(dAtA[i:], m.NoProxy)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.NoProxy)))
|
|
i--
|
|
dAtA[i] = 0x22
|
|
}
|
|
if len(m.FtpProxy) > 0 {
|
|
i -= len(m.FtpProxy)
|
|
copy(dAtA[i:], m.FtpProxy)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.FtpProxy)))
|
|
i--
|
|
dAtA[i] = 0x1a
|
|
}
|
|
if len(m.HttpsProxy) > 0 {
|
|
i -= len(m.HttpsProxy)
|
|
copy(dAtA[i:], m.HttpsProxy)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.HttpsProxy)))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
if len(m.HttpProxy) > 0 {
|
|
i -= len(m.HttpProxy)
|
|
copy(dAtA[i:], m.HttpProxy)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.HttpProxy)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *WorkerConstraints) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *WorkerConstraints) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *WorkerConstraints) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.Filter) > 0 {
|
|
for iNdEx := len(m.Filter) - 1; iNdEx >= 0; iNdEx-- {
|
|
i -= len(m.Filter[iNdEx])
|
|
copy(dAtA[i:], m.Filter[iNdEx])
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Filter[iNdEx])))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *Definition) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *Definition) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *Definition) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.Source != nil {
|
|
size, err := m.Source.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x1a
|
|
}
|
|
if len(m.Metadata) > 0 {
|
|
for k := range m.Metadata {
|
|
v := m.Metadata[k]
|
|
baseI := i
|
|
size, err := v.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
i -= len(k)
|
|
copy(dAtA[i:], k)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(k)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(baseI-i))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
}
|
|
if len(m.Def) > 0 {
|
|
for iNdEx := len(m.Def) - 1; iNdEx >= 0; iNdEx-- {
|
|
i -= len(m.Def[iNdEx])
|
|
copy(dAtA[i:], m.Def[iNdEx])
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Def[iNdEx])))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *FileOp) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *FileOp) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *FileOp) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.Actions) > 0 {
|
|
for iNdEx := len(m.Actions) - 1; iNdEx >= 0; iNdEx-- {
|
|
size, err := m.Actions[iNdEx].MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *FileAction) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *FileAction) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *FileAction) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if vtmsg, ok := m.Action.(interface {
|
|
MarshalToSizedBufferVT([]byte) (int, error)
|
|
}); ok {
|
|
size, err := vtmsg.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
}
|
|
if m.Output != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Output))
|
|
i--
|
|
dAtA[i] = 0x18
|
|
}
|
|
if m.SecondaryInput != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.SecondaryInput))
|
|
i--
|
|
dAtA[i] = 0x10
|
|
}
|
|
if m.Input != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Input))
|
|
i--
|
|
dAtA[i] = 0x8
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *FileAction_Copy) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *FileAction_Copy) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
i := len(dAtA)
|
|
if m.Copy != nil {
|
|
size, err := m.Copy.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x22
|
|
} else {
|
|
i = protohelpers.EncodeVarint(dAtA, i, 0)
|
|
i--
|
|
dAtA[i] = 0x22
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
func (m *FileAction_Mkfile) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *FileAction_Mkfile) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
i := len(dAtA)
|
|
if m.Mkfile != nil {
|
|
size, err := m.Mkfile.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x2a
|
|
} else {
|
|
i = protohelpers.EncodeVarint(dAtA, i, 0)
|
|
i--
|
|
dAtA[i] = 0x2a
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
func (m *FileAction_Mkdir) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *FileAction_Mkdir) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
i := len(dAtA)
|
|
if m.Mkdir != nil {
|
|
size, err := m.Mkdir.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x32
|
|
} else {
|
|
i = protohelpers.EncodeVarint(dAtA, i, 0)
|
|
i--
|
|
dAtA[i] = 0x32
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
func (m *FileAction_Rm) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *FileAction_Rm) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
i := len(dAtA)
|
|
if m.Rm != nil {
|
|
size, err := m.Rm.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x3a
|
|
} else {
|
|
i = protohelpers.EncodeVarint(dAtA, i, 0)
|
|
i--
|
|
dAtA[i] = 0x3a
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
func (m *FileActionCopy) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *FileActionCopy) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *FileActionCopy) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.ModeStr) > 0 {
|
|
i -= len(m.ModeStr)
|
|
copy(dAtA[i:], m.ModeStr)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.ModeStr)))
|
|
i--
|
|
dAtA[i] = 0x7a
|
|
}
|
|
if m.AlwaysReplaceExistingDestPaths {
|
|
i--
|
|
if m.AlwaysReplaceExistingDestPaths {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x70
|
|
}
|
|
if len(m.ExcludePatterns) > 0 {
|
|
for iNdEx := len(m.ExcludePatterns) - 1; iNdEx >= 0; iNdEx-- {
|
|
i -= len(m.ExcludePatterns[iNdEx])
|
|
copy(dAtA[i:], m.ExcludePatterns[iNdEx])
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.ExcludePatterns[iNdEx])))
|
|
i--
|
|
dAtA[i] = 0x6a
|
|
}
|
|
}
|
|
if len(m.IncludePatterns) > 0 {
|
|
for iNdEx := len(m.IncludePatterns) - 1; iNdEx >= 0; iNdEx-- {
|
|
i -= len(m.IncludePatterns[iNdEx])
|
|
copy(dAtA[i:], m.IncludePatterns[iNdEx])
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.IncludePatterns[iNdEx])))
|
|
i--
|
|
dAtA[i] = 0x62
|
|
}
|
|
}
|
|
if m.Timestamp != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Timestamp))
|
|
i--
|
|
dAtA[i] = 0x58
|
|
}
|
|
if m.AllowEmptyWildcard {
|
|
i--
|
|
if m.AllowEmptyWildcard {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x50
|
|
}
|
|
if m.AllowWildcard {
|
|
i--
|
|
if m.AllowWildcard {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x48
|
|
}
|
|
if m.CreateDestPath {
|
|
i--
|
|
if m.CreateDestPath {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x40
|
|
}
|
|
if m.AttemptUnpackDockerCompatibility {
|
|
i--
|
|
if m.AttemptUnpackDockerCompatibility {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x38
|
|
}
|
|
if m.DirCopyContents {
|
|
i--
|
|
if m.DirCopyContents {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x30
|
|
}
|
|
if m.FollowSymlink {
|
|
i--
|
|
if m.FollowSymlink {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x28
|
|
}
|
|
if m.Mode != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Mode))
|
|
i--
|
|
dAtA[i] = 0x20
|
|
}
|
|
if m.Owner != nil {
|
|
size, err := m.Owner.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x1a
|
|
}
|
|
if len(m.Dest) > 0 {
|
|
i -= len(m.Dest)
|
|
copy(dAtA[i:], m.Dest)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Dest)))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
if len(m.Src) > 0 {
|
|
i -= len(m.Src)
|
|
copy(dAtA[i:], m.Src)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Src)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *FileActionMkFile) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *FileActionMkFile) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *FileActionMkFile) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.Timestamp != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Timestamp))
|
|
i--
|
|
dAtA[i] = 0x28
|
|
}
|
|
if m.Owner != nil {
|
|
size, err := m.Owner.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x22
|
|
}
|
|
if len(m.Data) > 0 {
|
|
i -= len(m.Data)
|
|
copy(dAtA[i:], m.Data)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Data)))
|
|
i--
|
|
dAtA[i] = 0x1a
|
|
}
|
|
if m.Mode != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Mode))
|
|
i--
|
|
dAtA[i] = 0x10
|
|
}
|
|
if len(m.Path) > 0 {
|
|
i -= len(m.Path)
|
|
copy(dAtA[i:], m.Path)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Path)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *FileActionMkDir) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *FileActionMkDir) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *FileActionMkDir) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.Timestamp != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Timestamp))
|
|
i--
|
|
dAtA[i] = 0x28
|
|
}
|
|
if m.Owner != nil {
|
|
size, err := m.Owner.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x22
|
|
}
|
|
if m.MakeParents {
|
|
i--
|
|
if m.MakeParents {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x18
|
|
}
|
|
if m.Mode != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Mode))
|
|
i--
|
|
dAtA[i] = 0x10
|
|
}
|
|
if len(m.Path) > 0 {
|
|
i -= len(m.Path)
|
|
copy(dAtA[i:], m.Path)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Path)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *FileActionRm) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *FileActionRm) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *FileActionRm) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.AllowWildcard {
|
|
i--
|
|
if m.AllowWildcard {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x18
|
|
}
|
|
if m.AllowNotFound {
|
|
i--
|
|
if m.AllowNotFound {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i--
|
|
dAtA[i] = 0x10
|
|
}
|
|
if len(m.Path) > 0 {
|
|
i -= len(m.Path)
|
|
copy(dAtA[i:], m.Path)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Path)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *ChownOpt) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *ChownOpt) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *ChownOpt) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.Group != nil {
|
|
size, err := m.Group.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
if m.User != nil {
|
|
size, err := m.User.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *UserOpt) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *UserOpt) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *UserOpt) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if vtmsg, ok := m.User.(interface {
|
|
MarshalToSizedBufferVT([]byte) (int, error)
|
|
}); ok {
|
|
size, err := vtmsg.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *UserOpt_ByName) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *UserOpt_ByName) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
i := len(dAtA)
|
|
if m.ByName != nil {
|
|
size, err := m.ByName.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
} else {
|
|
i = protohelpers.EncodeVarint(dAtA, i, 0)
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
func (m *UserOpt_ByID) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *UserOpt_ByID) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
i := len(dAtA)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.ByID))
|
|
i--
|
|
dAtA[i] = 0x10
|
|
return len(dAtA) - i, nil
|
|
}
|
|
func (m *NamedUserOpt) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *NamedUserOpt) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *NamedUserOpt) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.Input != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Input))
|
|
i--
|
|
dAtA[i] = 0x10
|
|
}
|
|
if len(m.Name) > 0 {
|
|
i -= len(m.Name)
|
|
copy(dAtA[i:], m.Name)
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(len(m.Name)))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *MergeInput) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *MergeInput) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *MergeInput) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.Input != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Input))
|
|
i--
|
|
dAtA[i] = 0x8
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *MergeOp) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *MergeOp) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *MergeOp) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if len(m.Inputs) > 0 {
|
|
for iNdEx := len(m.Inputs) - 1; iNdEx >= 0; iNdEx-- {
|
|
size, err := m.Inputs[iNdEx].MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *LowerDiffInput) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *LowerDiffInput) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *LowerDiffInput) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.Input != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Input))
|
|
i--
|
|
dAtA[i] = 0x8
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *UpperDiffInput) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *UpperDiffInput) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *UpperDiffInput) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.Input != 0 {
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(m.Input))
|
|
i--
|
|
dAtA[i] = 0x8
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *DiffOp) MarshalVT() (dAtA []byte, err error) {
|
|
if m == nil {
|
|
return nil, nil
|
|
}
|
|
size := m.SizeVT()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalToSizedBufferVT(dAtA[:size])
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *DiffOp) MarshalToVT(dAtA []byte) (int, error) {
|
|
size := m.SizeVT()
|
|
return m.MarshalToSizedBufferVT(dAtA[:size])
|
|
}
|
|
|
|
func (m *DiffOp) MarshalToSizedBufferVT(dAtA []byte) (int, error) {
|
|
if m == nil {
|
|
return 0, nil
|
|
}
|
|
i := len(dAtA)
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.unknownFields != nil {
|
|
i -= len(m.unknownFields)
|
|
copy(dAtA[i:], m.unknownFields)
|
|
}
|
|
if m.Upper != nil {
|
|
size, err := m.Upper.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0x12
|
|
}
|
|
if m.Lower != nil {
|
|
size, err := m.Lower.MarshalToSizedBufferVT(dAtA[:i])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i -= size
|
|
i = protohelpers.EncodeVarint(dAtA, i, uint64(size))
|
|
i--
|
|
dAtA[i] = 0xa
|
|
}
|
|
return len(dAtA) - i, nil
|
|
}
|
|
|
|
func (m *Op) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if len(m.Inputs) > 0 {
|
|
for _, e := range m.Inputs {
|
|
l = e.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
if vtmsg, ok := m.Op.(interface{ SizeVT() int }); ok {
|
|
n += vtmsg.SizeVT()
|
|
}
|
|
if m.Platform != nil {
|
|
l = m.Platform.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.Constraints != nil {
|
|
l = m.Constraints.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *Op_Exec) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.Exec != nil {
|
|
l = m.Exec.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
} else {
|
|
n += 3
|
|
}
|
|
return n
|
|
}
|
|
func (m *Op_Source) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.Source != nil {
|
|
l = m.Source.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
} else {
|
|
n += 3
|
|
}
|
|
return n
|
|
}
|
|
func (m *Op_File) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.File != nil {
|
|
l = m.File.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
} else {
|
|
n += 3
|
|
}
|
|
return n
|
|
}
|
|
func (m *Op_Build) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.Build != nil {
|
|
l = m.Build.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
} else {
|
|
n += 3
|
|
}
|
|
return n
|
|
}
|
|
func (m *Op_Merge) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.Merge != nil {
|
|
l = m.Merge.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
} else {
|
|
n += 3
|
|
}
|
|
return n
|
|
}
|
|
func (m *Op_Diff) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.Diff != nil {
|
|
l = m.Diff.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
} else {
|
|
n += 3
|
|
}
|
|
return n
|
|
}
|
|
func (m *Platform) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.Architecture)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
l = len(m.OS)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
l = len(m.Variant)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
l = len(m.OSVersion)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if len(m.OSFeatures) > 0 {
|
|
for _, s := range m.OSFeatures {
|
|
l = len(s)
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *Input) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.Digest)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.Index != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Index))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *ExecOp) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.Meta != nil {
|
|
l = m.Meta.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if len(m.Mounts) > 0 {
|
|
for _, e := range m.Mounts {
|
|
l = e.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
if m.Network != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Network))
|
|
}
|
|
if m.Security != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Security))
|
|
}
|
|
if len(m.Secretenv) > 0 {
|
|
for _, e := range m.Secretenv {
|
|
l = e.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *Meta) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if len(m.Args) > 0 {
|
|
for _, s := range m.Args {
|
|
l = len(s)
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
if len(m.Env) > 0 {
|
|
for _, s := range m.Env {
|
|
l = len(s)
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
l = len(m.Cwd)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
l = len(m.User)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.ProxyEnv != nil {
|
|
l = m.ProxyEnv.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if len(m.ExtraHosts) > 0 {
|
|
for _, e := range m.ExtraHosts {
|
|
l = e.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
l = len(m.Hostname)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if len(m.Ulimit) > 0 {
|
|
for _, e := range m.Ulimit {
|
|
l = e.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
l = len(m.CgroupParent)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.RemoveMountStubsRecursive {
|
|
n += 2
|
|
}
|
|
if len(m.ValidExitCodes) > 0 {
|
|
l = 0
|
|
for _, e := range m.ValidExitCodes {
|
|
l += protohelpers.SizeOfVarint(uint64(e))
|
|
}
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(l)) + l
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *HostIP) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.Host)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
l = len(m.IP)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *Ulimit) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.Name)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.Soft != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Soft))
|
|
}
|
|
if m.Hard != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Hard))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *SecretEnv) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.ID)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
l = len(m.Name)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.Optional {
|
|
n += 2
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *Mount) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.Input != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Input))
|
|
}
|
|
l = len(m.Selector)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
l = len(m.Dest)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.Output != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Output))
|
|
}
|
|
if m.Readonly {
|
|
n += 2
|
|
}
|
|
if m.MountType != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.MountType))
|
|
}
|
|
if m.TmpfsOpt != nil {
|
|
l = m.TmpfsOpt.SizeVT()
|
|
n += 2 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.CacheOpt != nil {
|
|
l = m.CacheOpt.SizeVT()
|
|
n += 2 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.SecretOpt != nil {
|
|
l = m.SecretOpt.SizeVT()
|
|
n += 2 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.SSHOpt != nil {
|
|
l = m.SSHOpt.SizeVT()
|
|
n += 2 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
l = len(m.ResultID)
|
|
if l > 0 {
|
|
n += 2 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.ContentCache != 0 {
|
|
n += 2 + protohelpers.SizeOfVarint(uint64(m.ContentCache))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *TmpfsOpt) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.Size != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Size))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *CacheOpt) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.ID)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.Sharing != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Sharing))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *SecretOpt) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.ID)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.Uid != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Uid))
|
|
}
|
|
if m.Gid != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Gid))
|
|
}
|
|
if m.Mode != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Mode))
|
|
}
|
|
if m.Optional {
|
|
n += 2
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *SSHOpt) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.ID)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.Uid != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Uid))
|
|
}
|
|
if m.Gid != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Gid))
|
|
}
|
|
if m.Mode != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Mode))
|
|
}
|
|
if m.Optional {
|
|
n += 2
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *SourceOp) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.Identifier)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if len(m.Attrs) > 0 {
|
|
for k, v := range m.Attrs {
|
|
_ = k
|
|
_ = v
|
|
mapEntrySize := 1 + len(k) + protohelpers.SizeOfVarint(uint64(len(k))) + 1 + len(v) + protohelpers.SizeOfVarint(uint64(len(v)))
|
|
n += mapEntrySize + 1 + protohelpers.SizeOfVarint(uint64(mapEntrySize))
|
|
}
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *BuildOp) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.Builder != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Builder))
|
|
}
|
|
if len(m.Inputs) > 0 {
|
|
for k, v := range m.Inputs {
|
|
_ = k
|
|
_ = v
|
|
l = 0
|
|
if v != nil {
|
|
l = v.SizeVT()
|
|
}
|
|
l += 1 + protohelpers.SizeOfVarint(uint64(l))
|
|
mapEntrySize := 1 + len(k) + protohelpers.SizeOfVarint(uint64(len(k))) + l
|
|
n += mapEntrySize + 1 + protohelpers.SizeOfVarint(uint64(mapEntrySize))
|
|
}
|
|
}
|
|
if m.Def != nil {
|
|
l = m.Def.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if len(m.Attrs) > 0 {
|
|
for k, v := range m.Attrs {
|
|
_ = k
|
|
_ = v
|
|
mapEntrySize := 1 + len(k) + protohelpers.SizeOfVarint(uint64(len(k))) + 1 + len(v) + protohelpers.SizeOfVarint(uint64(len(v)))
|
|
n += mapEntrySize + 1 + protohelpers.SizeOfVarint(uint64(mapEntrySize))
|
|
}
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *BuildInput) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.Input != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Input))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *OpMetadata) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.IgnoreCache {
|
|
n += 2
|
|
}
|
|
if len(m.Description) > 0 {
|
|
for k, v := range m.Description {
|
|
_ = k
|
|
_ = v
|
|
mapEntrySize := 1 + len(k) + protohelpers.SizeOfVarint(uint64(len(k))) + 1 + len(v) + protohelpers.SizeOfVarint(uint64(len(v)))
|
|
n += mapEntrySize + 1 + protohelpers.SizeOfVarint(uint64(mapEntrySize))
|
|
}
|
|
}
|
|
if m.ExportCache != nil {
|
|
l = m.ExportCache.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if len(m.Caps) > 0 {
|
|
for k, v := range m.Caps {
|
|
_ = k
|
|
_ = v
|
|
mapEntrySize := 1 + len(k) + protohelpers.SizeOfVarint(uint64(len(k))) + 1 + 1
|
|
n += mapEntrySize + 1 + protohelpers.SizeOfVarint(uint64(mapEntrySize))
|
|
}
|
|
}
|
|
if m.ProgressGroup != nil {
|
|
l = m.ProgressGroup.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *Source) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if len(m.Locations) > 0 {
|
|
for k, v := range m.Locations {
|
|
_ = k
|
|
_ = v
|
|
l = 0
|
|
if v != nil {
|
|
l = v.SizeVT()
|
|
}
|
|
l += 1 + protohelpers.SizeOfVarint(uint64(l))
|
|
mapEntrySize := 1 + len(k) + protohelpers.SizeOfVarint(uint64(len(k))) + l
|
|
n += mapEntrySize + 1 + protohelpers.SizeOfVarint(uint64(mapEntrySize))
|
|
}
|
|
}
|
|
if len(m.Infos) > 0 {
|
|
for _, e := range m.Infos {
|
|
l = e.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *Locations) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if len(m.Locations) > 0 {
|
|
for _, e := range m.Locations {
|
|
l = e.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *SourceInfo) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.Filename)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
l = len(m.Data)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.Definition != nil {
|
|
l = m.Definition.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
l = len(m.Language)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *Location) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.SourceIndex != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.SourceIndex))
|
|
}
|
|
if len(m.Ranges) > 0 {
|
|
for _, e := range m.Ranges {
|
|
l = e.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *Range) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.Start != nil {
|
|
l = m.Start.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.End != nil {
|
|
l = m.End.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *Position) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.Line != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Line))
|
|
}
|
|
if m.Character != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Character))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *ExportCache) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.Value {
|
|
n += 2
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *ProgressGroup) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.Id)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
l = len(m.Name)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.Weak {
|
|
n += 2
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *ProxyEnv) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.HttpProxy)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
l = len(m.HttpsProxy)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
l = len(m.FtpProxy)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
l = len(m.NoProxy)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
l = len(m.AllProxy)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *WorkerConstraints) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if len(m.Filter) > 0 {
|
|
for _, s := range m.Filter {
|
|
l = len(s)
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *Definition) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if len(m.Def) > 0 {
|
|
for _, b := range m.Def {
|
|
l = len(b)
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
if len(m.Metadata) > 0 {
|
|
for k, v := range m.Metadata {
|
|
_ = k
|
|
_ = v
|
|
l = 0
|
|
if v != nil {
|
|
l = v.SizeVT()
|
|
}
|
|
l += 1 + protohelpers.SizeOfVarint(uint64(l))
|
|
mapEntrySize := 1 + len(k) + protohelpers.SizeOfVarint(uint64(len(k))) + l
|
|
n += mapEntrySize + 1 + protohelpers.SizeOfVarint(uint64(mapEntrySize))
|
|
}
|
|
}
|
|
if m.Source != nil {
|
|
l = m.Source.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *FileOp) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if len(m.Actions) > 0 {
|
|
for _, e := range m.Actions {
|
|
l = e.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *FileAction) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.Input != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Input))
|
|
}
|
|
if m.SecondaryInput != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.SecondaryInput))
|
|
}
|
|
if m.Output != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Output))
|
|
}
|
|
if vtmsg, ok := m.Action.(interface{ SizeVT() int }); ok {
|
|
n += vtmsg.SizeVT()
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *FileAction_Copy) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.Copy != nil {
|
|
l = m.Copy.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
} else {
|
|
n += 3
|
|
}
|
|
return n
|
|
}
|
|
func (m *FileAction_Mkfile) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.Mkfile != nil {
|
|
l = m.Mkfile.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
} else {
|
|
n += 3
|
|
}
|
|
return n
|
|
}
|
|
func (m *FileAction_Mkdir) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.Mkdir != nil {
|
|
l = m.Mkdir.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
} else {
|
|
n += 3
|
|
}
|
|
return n
|
|
}
|
|
func (m *FileAction_Rm) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.Rm != nil {
|
|
l = m.Rm.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
} else {
|
|
n += 3
|
|
}
|
|
return n
|
|
}
|
|
func (m *FileActionCopy) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.Src)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
l = len(m.Dest)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.Owner != nil {
|
|
l = m.Owner.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.Mode != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Mode))
|
|
}
|
|
if m.FollowSymlink {
|
|
n += 2
|
|
}
|
|
if m.DirCopyContents {
|
|
n += 2
|
|
}
|
|
if m.AttemptUnpackDockerCompatibility {
|
|
n += 2
|
|
}
|
|
if m.CreateDestPath {
|
|
n += 2
|
|
}
|
|
if m.AllowWildcard {
|
|
n += 2
|
|
}
|
|
if m.AllowEmptyWildcard {
|
|
n += 2
|
|
}
|
|
if m.Timestamp != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Timestamp))
|
|
}
|
|
if len(m.IncludePatterns) > 0 {
|
|
for _, s := range m.IncludePatterns {
|
|
l = len(s)
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
if len(m.ExcludePatterns) > 0 {
|
|
for _, s := range m.ExcludePatterns {
|
|
l = len(s)
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
if m.AlwaysReplaceExistingDestPaths {
|
|
n += 2
|
|
}
|
|
l = len(m.ModeStr)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *FileActionMkFile) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.Path)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.Mode != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Mode))
|
|
}
|
|
l = len(m.Data)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.Owner != nil {
|
|
l = m.Owner.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.Timestamp != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Timestamp))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *FileActionMkDir) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.Path)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.Mode != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Mode))
|
|
}
|
|
if m.MakeParents {
|
|
n += 2
|
|
}
|
|
if m.Owner != nil {
|
|
l = m.Owner.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.Timestamp != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Timestamp))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *FileActionRm) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.Path)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.AllowNotFound {
|
|
n += 2
|
|
}
|
|
if m.AllowWildcard {
|
|
n += 2
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *ChownOpt) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.User != nil {
|
|
l = m.User.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.Group != nil {
|
|
l = m.Group.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *UserOpt) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if vtmsg, ok := m.User.(interface{ SizeVT() int }); ok {
|
|
n += vtmsg.SizeVT()
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *UserOpt_ByName) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.ByName != nil {
|
|
l = m.ByName.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
} else {
|
|
n += 3
|
|
}
|
|
return n
|
|
}
|
|
func (m *UserOpt_ByID) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.ByID))
|
|
return n
|
|
}
|
|
func (m *NamedUserOpt) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
l = len(m.Name)
|
|
if l > 0 {
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.Input != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Input))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *MergeInput) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.Input != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Input))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *MergeOp) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if len(m.Inputs) > 0 {
|
|
for _, e := range m.Inputs {
|
|
l = e.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *LowerDiffInput) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.Input != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Input))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *UpperDiffInput) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.Input != 0 {
|
|
n += 1 + protohelpers.SizeOfVarint(uint64(m.Input))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *DiffOp) SizeVT() (n int) {
|
|
if m == nil {
|
|
return 0
|
|
}
|
|
var l int
|
|
_ = l
|
|
if m.Lower != nil {
|
|
l = m.Lower.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
if m.Upper != nil {
|
|
l = m.Upper.SizeVT()
|
|
n += 1 + l + protohelpers.SizeOfVarint(uint64(l))
|
|
}
|
|
n += len(m.unknownFields)
|
|
return n
|
|
}
|
|
|
|
func (m *Op) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Op: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Op: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Inputs", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Inputs = append(m.Inputs, &Input{})
|
|
if err := m.Inputs[len(m.Inputs)-1].UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Exec", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if oneof, ok := m.Op.(*Op_Exec); ok {
|
|
if err := oneof.Exec.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
} else {
|
|
v := &ExecOp{}
|
|
if err := v.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
m.Op = &Op_Exec{Exec: v}
|
|
}
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Source", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if oneof, ok := m.Op.(*Op_Source); ok {
|
|
if err := oneof.Source.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
} else {
|
|
v := &SourceOp{}
|
|
if err := v.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
m.Op = &Op_Source{Source: v}
|
|
}
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field File", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if oneof, ok := m.Op.(*Op_File); ok {
|
|
if err := oneof.File.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
} else {
|
|
v := &FileOp{}
|
|
if err := v.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
m.Op = &Op_File{File: v}
|
|
}
|
|
iNdEx = postIndex
|
|
case 5:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Build", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if oneof, ok := m.Op.(*Op_Build); ok {
|
|
if err := oneof.Build.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
} else {
|
|
v := &BuildOp{}
|
|
if err := v.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
m.Op = &Op_Build{Build: v}
|
|
}
|
|
iNdEx = postIndex
|
|
case 6:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Merge", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if oneof, ok := m.Op.(*Op_Merge); ok {
|
|
if err := oneof.Merge.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
} else {
|
|
v := &MergeOp{}
|
|
if err := v.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
m.Op = &Op_Merge{Merge: v}
|
|
}
|
|
iNdEx = postIndex
|
|
case 7:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Diff", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if oneof, ok := m.Op.(*Op_Diff); ok {
|
|
if err := oneof.Diff.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
} else {
|
|
v := &DiffOp{}
|
|
if err := v.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
m.Op = &Op_Diff{Diff: v}
|
|
}
|
|
iNdEx = postIndex
|
|
case 10:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Platform", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Platform == nil {
|
|
m.Platform = &Platform{}
|
|
}
|
|
if err := m.Platform.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 11:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Constraints", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Constraints == nil {
|
|
m.Constraints = &WorkerConstraints{}
|
|
}
|
|
if err := m.Constraints.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Platform) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Platform: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Platform: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Architecture", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Architecture = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field OS", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.OS = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Variant", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Variant = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field OSVersion", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.OSVersion = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 5:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field OSFeatures", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.OSFeatures = append(m.OSFeatures, string(dAtA[iNdEx:postIndex]))
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Input) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Input: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Input: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Digest", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Digest = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Index", wireType)
|
|
}
|
|
m.Index = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Index |= int64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *ExecOp) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: ExecOp: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: ExecOp: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Meta", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Meta == nil {
|
|
m.Meta = &Meta{}
|
|
}
|
|
if err := m.Meta.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Mounts", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Mounts = append(m.Mounts, &Mount{})
|
|
if err := m.Mounts[len(m.Mounts)-1].UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Network", wireType)
|
|
}
|
|
m.Network = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Network |= NetMode(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 4:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Security", wireType)
|
|
}
|
|
m.Security = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Security |= SecurityMode(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 5:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Secretenv", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Secretenv = append(m.Secretenv, &SecretEnv{})
|
|
if err := m.Secretenv[len(m.Secretenv)-1].UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Meta) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Meta: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Meta: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Args", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Args = append(m.Args, string(dAtA[iNdEx:postIndex]))
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Env", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Env = append(m.Env, string(dAtA[iNdEx:postIndex]))
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Cwd", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Cwd = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field User", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.User = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 5:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ProxyEnv", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.ProxyEnv == nil {
|
|
m.ProxyEnv = &ProxyEnv{}
|
|
}
|
|
if err := m.ProxyEnv.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 6:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ExtraHosts", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ExtraHosts = append(m.ExtraHosts, &HostIP{})
|
|
if err := m.ExtraHosts[len(m.ExtraHosts)-1].UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 7:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Hostname", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Hostname = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 9:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Ulimit", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Ulimit = append(m.Ulimit, &Ulimit{})
|
|
if err := m.Ulimit[len(m.Ulimit)-1].UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 10:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field CgroupParent", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.CgroupParent = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 11:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field RemoveMountStubsRecursive", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.RemoveMountStubsRecursive = bool(v != 0)
|
|
case 12:
|
|
if wireType == 0 {
|
|
var v int32
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int32(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.ValidExitCodes = append(m.ValidExitCodes, v)
|
|
} else if wireType == 2 {
|
|
var packedLen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
packedLen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if packedLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + packedLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
var elementCount int
|
|
var count int
|
|
for _, integer := range dAtA[iNdEx:postIndex] {
|
|
if integer < 128 {
|
|
count++
|
|
}
|
|
}
|
|
elementCount = count
|
|
if elementCount != 0 && len(m.ValidExitCodes) == 0 {
|
|
m.ValidExitCodes = make([]int32, 0, elementCount)
|
|
}
|
|
for iNdEx < postIndex {
|
|
var v int32
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int32(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.ValidExitCodes = append(m.ValidExitCodes, v)
|
|
}
|
|
} else {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ValidExitCodes", wireType)
|
|
}
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *HostIP) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: HostIP: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: HostIP: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Host", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Host = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field IP", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.IP = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Ulimit) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Ulimit: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Ulimit: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Name", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Name = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Soft", wireType)
|
|
}
|
|
m.Soft = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Soft |= int64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 3:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Hard", wireType)
|
|
}
|
|
m.Hard = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Hard |= int64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *SecretEnv) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: SecretEnv: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: SecretEnv: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ID = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Name", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Name = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Optional", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.Optional = bool(v != 0)
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Mount) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Mount: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Mount: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Input", wireType)
|
|
}
|
|
m.Input = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Input |= int64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Selector", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Selector = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Dest", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Dest = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Output", wireType)
|
|
}
|
|
m.Output = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Output |= int64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 5:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Readonly", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.Readonly = bool(v != 0)
|
|
case 6:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field MountType", wireType)
|
|
}
|
|
m.MountType = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.MountType |= MountType(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 19:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field TmpfsOpt", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.TmpfsOpt == nil {
|
|
m.TmpfsOpt = &TmpfsOpt{}
|
|
}
|
|
if err := m.TmpfsOpt.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 20:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field CacheOpt", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.CacheOpt == nil {
|
|
m.CacheOpt = &CacheOpt{}
|
|
}
|
|
if err := m.CacheOpt.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 21:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field SecretOpt", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.SecretOpt == nil {
|
|
m.SecretOpt = &SecretOpt{}
|
|
}
|
|
if err := m.SecretOpt.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 22:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field SSHOpt", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.SSHOpt == nil {
|
|
m.SSHOpt = &SSHOpt{}
|
|
}
|
|
if err := m.SSHOpt.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 23:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ResultID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ResultID = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 24:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ContentCache", wireType)
|
|
}
|
|
m.ContentCache = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.ContentCache |= MountContentCache(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *TmpfsOpt) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: TmpfsOpt: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: TmpfsOpt: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Size", wireType)
|
|
}
|
|
m.Size = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Size |= int64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *CacheOpt) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: CacheOpt: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: CacheOpt: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ID = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Sharing", wireType)
|
|
}
|
|
m.Sharing = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Sharing |= CacheSharingOpt(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *SecretOpt) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: SecretOpt: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: SecretOpt: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ID = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Uid", wireType)
|
|
}
|
|
m.Uid = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Uid |= uint32(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 3:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Gid", wireType)
|
|
}
|
|
m.Gid = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Gid |= uint32(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 4:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Mode", wireType)
|
|
}
|
|
m.Mode = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Mode |= uint32(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 5:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Optional", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.Optional = bool(v != 0)
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *SSHOpt) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: SSHOpt: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: SSHOpt: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ID = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Uid", wireType)
|
|
}
|
|
m.Uid = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Uid |= uint32(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 3:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Gid", wireType)
|
|
}
|
|
m.Gid = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Gid |= uint32(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 4:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Mode", wireType)
|
|
}
|
|
m.Mode = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Mode |= uint32(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 5:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Optional", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.Optional = bool(v != 0)
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *SourceOp) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: SourceOp: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: SourceOp: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Identifier", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Identifier = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Attrs", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Attrs == nil {
|
|
m.Attrs = make(map[string]string)
|
|
}
|
|
var mapkey string
|
|
var mapvalue string
|
|
for iNdEx < postIndex {
|
|
entryPreIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
if fieldNum == 1 {
|
|
var stringLenmapkey uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLenmapkey |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLenmapkey := int(stringLenmapkey)
|
|
if intStringLenmapkey < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postStringIndexmapkey := iNdEx + intStringLenmapkey
|
|
if postStringIndexmapkey < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postStringIndexmapkey > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapkey = string(dAtA[iNdEx:postStringIndexmapkey])
|
|
iNdEx = postStringIndexmapkey
|
|
} else if fieldNum == 2 {
|
|
var stringLenmapvalue uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLenmapvalue |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLenmapvalue := int(stringLenmapvalue)
|
|
if intStringLenmapvalue < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postStringIndexmapvalue := iNdEx + intStringLenmapvalue
|
|
if postStringIndexmapvalue < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postStringIndexmapvalue > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapvalue = string(dAtA[iNdEx:postStringIndexmapvalue])
|
|
iNdEx = postStringIndexmapvalue
|
|
} else {
|
|
iNdEx = entryPreIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > postIndex {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
m.Attrs[mapkey] = mapvalue
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *BuildOp) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: BuildOp: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: BuildOp: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Builder", wireType)
|
|
}
|
|
m.Builder = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Builder |= int64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Inputs", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Inputs == nil {
|
|
m.Inputs = make(map[string]*BuildInput)
|
|
}
|
|
var mapkey string
|
|
var mapvalue *BuildInput
|
|
for iNdEx < postIndex {
|
|
entryPreIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
if fieldNum == 1 {
|
|
var stringLenmapkey uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLenmapkey |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLenmapkey := int(stringLenmapkey)
|
|
if intStringLenmapkey < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postStringIndexmapkey := iNdEx + intStringLenmapkey
|
|
if postStringIndexmapkey < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postStringIndexmapkey > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapkey = string(dAtA[iNdEx:postStringIndexmapkey])
|
|
iNdEx = postStringIndexmapkey
|
|
} else if fieldNum == 2 {
|
|
var mapmsglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
mapmsglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if mapmsglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postmsgIndex := iNdEx + mapmsglen
|
|
if postmsgIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postmsgIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapvalue = &BuildInput{}
|
|
if err := mapvalue.UnmarshalVT(dAtA[iNdEx:postmsgIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postmsgIndex
|
|
} else {
|
|
iNdEx = entryPreIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > postIndex {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
m.Inputs[mapkey] = mapvalue
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Def", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Def == nil {
|
|
m.Def = &Definition{}
|
|
}
|
|
if err := m.Def.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Attrs", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Attrs == nil {
|
|
m.Attrs = make(map[string]string)
|
|
}
|
|
var mapkey string
|
|
var mapvalue string
|
|
for iNdEx < postIndex {
|
|
entryPreIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
if fieldNum == 1 {
|
|
var stringLenmapkey uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLenmapkey |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLenmapkey := int(stringLenmapkey)
|
|
if intStringLenmapkey < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postStringIndexmapkey := iNdEx + intStringLenmapkey
|
|
if postStringIndexmapkey < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postStringIndexmapkey > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapkey = string(dAtA[iNdEx:postStringIndexmapkey])
|
|
iNdEx = postStringIndexmapkey
|
|
} else if fieldNum == 2 {
|
|
var stringLenmapvalue uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLenmapvalue |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLenmapvalue := int(stringLenmapvalue)
|
|
if intStringLenmapvalue < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postStringIndexmapvalue := iNdEx + intStringLenmapvalue
|
|
if postStringIndexmapvalue < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postStringIndexmapvalue > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapvalue = string(dAtA[iNdEx:postStringIndexmapvalue])
|
|
iNdEx = postStringIndexmapvalue
|
|
} else {
|
|
iNdEx = entryPreIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > postIndex {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
m.Attrs[mapkey] = mapvalue
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *BuildInput) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: BuildInput: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: BuildInput: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Input", wireType)
|
|
}
|
|
m.Input = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Input |= int64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *OpMetadata) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: OpMetadata: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: OpMetadata: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field IgnoreCache", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.IgnoreCache = bool(v != 0)
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Description", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Description == nil {
|
|
m.Description = make(map[string]string)
|
|
}
|
|
var mapkey string
|
|
var mapvalue string
|
|
for iNdEx < postIndex {
|
|
entryPreIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
if fieldNum == 1 {
|
|
var stringLenmapkey uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLenmapkey |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLenmapkey := int(stringLenmapkey)
|
|
if intStringLenmapkey < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postStringIndexmapkey := iNdEx + intStringLenmapkey
|
|
if postStringIndexmapkey < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postStringIndexmapkey > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapkey = string(dAtA[iNdEx:postStringIndexmapkey])
|
|
iNdEx = postStringIndexmapkey
|
|
} else if fieldNum == 2 {
|
|
var stringLenmapvalue uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLenmapvalue |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLenmapvalue := int(stringLenmapvalue)
|
|
if intStringLenmapvalue < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postStringIndexmapvalue := iNdEx + intStringLenmapvalue
|
|
if postStringIndexmapvalue < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postStringIndexmapvalue > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapvalue = string(dAtA[iNdEx:postStringIndexmapvalue])
|
|
iNdEx = postStringIndexmapvalue
|
|
} else {
|
|
iNdEx = entryPreIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > postIndex {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
m.Description[mapkey] = mapvalue
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ExportCache", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.ExportCache == nil {
|
|
m.ExportCache = &ExportCache{}
|
|
}
|
|
if err := m.ExportCache.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 5:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Caps", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Caps == nil {
|
|
m.Caps = make(map[string]bool)
|
|
}
|
|
var mapkey string
|
|
var mapvalue bool
|
|
for iNdEx < postIndex {
|
|
entryPreIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
if fieldNum == 1 {
|
|
var stringLenmapkey uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLenmapkey |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLenmapkey := int(stringLenmapkey)
|
|
if intStringLenmapkey < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postStringIndexmapkey := iNdEx + intStringLenmapkey
|
|
if postStringIndexmapkey < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postStringIndexmapkey > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapkey = string(dAtA[iNdEx:postStringIndexmapkey])
|
|
iNdEx = postStringIndexmapkey
|
|
} else if fieldNum == 2 {
|
|
var mapvaluetemp int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
mapvaluetemp |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
mapvalue = bool(mapvaluetemp != 0)
|
|
} else {
|
|
iNdEx = entryPreIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > postIndex {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
m.Caps[mapkey] = mapvalue
|
|
iNdEx = postIndex
|
|
case 6:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ProgressGroup", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.ProgressGroup == nil {
|
|
m.ProgressGroup = &ProgressGroup{}
|
|
}
|
|
if err := m.ProgressGroup.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Source) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Source: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Source: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Locations", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Locations == nil {
|
|
m.Locations = make(map[string]*Locations)
|
|
}
|
|
var mapkey string
|
|
var mapvalue *Locations
|
|
for iNdEx < postIndex {
|
|
entryPreIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
if fieldNum == 1 {
|
|
var stringLenmapkey uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLenmapkey |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLenmapkey := int(stringLenmapkey)
|
|
if intStringLenmapkey < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postStringIndexmapkey := iNdEx + intStringLenmapkey
|
|
if postStringIndexmapkey < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postStringIndexmapkey > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapkey = string(dAtA[iNdEx:postStringIndexmapkey])
|
|
iNdEx = postStringIndexmapkey
|
|
} else if fieldNum == 2 {
|
|
var mapmsglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
mapmsglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if mapmsglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postmsgIndex := iNdEx + mapmsglen
|
|
if postmsgIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postmsgIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapvalue = &Locations{}
|
|
if err := mapvalue.UnmarshalVT(dAtA[iNdEx:postmsgIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postmsgIndex
|
|
} else {
|
|
iNdEx = entryPreIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > postIndex {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
m.Locations[mapkey] = mapvalue
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Infos", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Infos = append(m.Infos, &SourceInfo{})
|
|
if err := m.Infos[len(m.Infos)-1].UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Locations) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Locations: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Locations: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Locations", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Locations = append(m.Locations, &Location{})
|
|
if err := m.Locations[len(m.Locations)-1].UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *SourceInfo) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: SourceInfo: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: SourceInfo: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Filename", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Filename = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Data", wireType)
|
|
}
|
|
var byteLen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
byteLen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if byteLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + byteLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Data = append(m.Data[:0], dAtA[iNdEx:postIndex]...)
|
|
if m.Data == nil {
|
|
m.Data = []byte{}
|
|
}
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Definition", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Definition == nil {
|
|
m.Definition = &Definition{}
|
|
}
|
|
if err := m.Definition.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Language", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Language = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Location) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Location: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Location: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field SourceIndex", wireType)
|
|
}
|
|
m.SourceIndex = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.SourceIndex |= int32(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Ranges", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Ranges = append(m.Ranges, &Range{})
|
|
if err := m.Ranges[len(m.Ranges)-1].UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Range) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Range: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Range: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Start", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Start == nil {
|
|
m.Start = &Position{}
|
|
}
|
|
if err := m.Start.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field End", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.End == nil {
|
|
m.End = &Position{}
|
|
}
|
|
if err := m.End.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Position) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Position: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Position: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Line", wireType)
|
|
}
|
|
m.Line = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Line |= int32(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 2:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Character", wireType)
|
|
}
|
|
m.Character = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Character |= int32(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *ExportCache) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: ExportCache: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: ExportCache: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Value", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.Value = bool(v != 0)
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *ProgressGroup) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: ProgressGroup: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: ProgressGroup: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Id", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Id = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Name", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Name = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Weak", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.Weak = bool(v != 0)
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *ProxyEnv) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: ProxyEnv: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: ProxyEnv: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field HttpProxy", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.HttpProxy = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field HttpsProxy", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.HttpsProxy = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field FtpProxy", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.FtpProxy = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field NoProxy", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.NoProxy = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 5:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field AllProxy", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.AllProxy = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *WorkerConstraints) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: WorkerConstraints: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: WorkerConstraints: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Filter", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Filter = append(m.Filter, string(dAtA[iNdEx:postIndex]))
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Definition) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Definition: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Definition: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Def", wireType)
|
|
}
|
|
var byteLen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
byteLen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if byteLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + byteLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Def = append(m.Def, make([]byte, postIndex-iNdEx))
|
|
copy(m.Def[len(m.Def)-1], dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Metadata", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Metadata == nil {
|
|
m.Metadata = make(map[string]*OpMetadata)
|
|
}
|
|
var mapkey string
|
|
var mapvalue *OpMetadata
|
|
for iNdEx < postIndex {
|
|
entryPreIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
if fieldNum == 1 {
|
|
var stringLenmapkey uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLenmapkey |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLenmapkey := int(stringLenmapkey)
|
|
if intStringLenmapkey < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postStringIndexmapkey := iNdEx + intStringLenmapkey
|
|
if postStringIndexmapkey < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postStringIndexmapkey > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapkey = string(dAtA[iNdEx:postStringIndexmapkey])
|
|
iNdEx = postStringIndexmapkey
|
|
} else if fieldNum == 2 {
|
|
var mapmsglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
mapmsglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if mapmsglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postmsgIndex := iNdEx + mapmsglen
|
|
if postmsgIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postmsgIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapvalue = &OpMetadata{}
|
|
if err := mapvalue.UnmarshalVT(dAtA[iNdEx:postmsgIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postmsgIndex
|
|
} else {
|
|
iNdEx = entryPreIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > postIndex {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
m.Metadata[mapkey] = mapvalue
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Source", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Source == nil {
|
|
m.Source = &Source{}
|
|
}
|
|
if err := m.Source.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *FileOp) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: FileOp: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: FileOp: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Actions", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Actions = append(m.Actions, &FileAction{})
|
|
if err := m.Actions[len(m.Actions)-1].UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *FileAction) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: FileAction: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: FileAction: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Input", wireType)
|
|
}
|
|
m.Input = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Input |= int64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 2:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field SecondaryInput", wireType)
|
|
}
|
|
m.SecondaryInput = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.SecondaryInput |= int64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 3:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Output", wireType)
|
|
}
|
|
m.Output = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Output |= int64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Copy", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if oneof, ok := m.Action.(*FileAction_Copy); ok {
|
|
if err := oneof.Copy.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
} else {
|
|
v := &FileActionCopy{}
|
|
if err := v.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
m.Action = &FileAction_Copy{Copy: v}
|
|
}
|
|
iNdEx = postIndex
|
|
case 5:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Mkfile", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if oneof, ok := m.Action.(*FileAction_Mkfile); ok {
|
|
if err := oneof.Mkfile.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
} else {
|
|
v := &FileActionMkFile{}
|
|
if err := v.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
m.Action = &FileAction_Mkfile{Mkfile: v}
|
|
}
|
|
iNdEx = postIndex
|
|
case 6:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Mkdir", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if oneof, ok := m.Action.(*FileAction_Mkdir); ok {
|
|
if err := oneof.Mkdir.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
} else {
|
|
v := &FileActionMkDir{}
|
|
if err := v.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
m.Action = &FileAction_Mkdir{Mkdir: v}
|
|
}
|
|
iNdEx = postIndex
|
|
case 7:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Rm", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if oneof, ok := m.Action.(*FileAction_Rm); ok {
|
|
if err := oneof.Rm.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
} else {
|
|
v := &FileActionRm{}
|
|
if err := v.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
m.Action = &FileAction_Rm{Rm: v}
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *FileActionCopy) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: FileActionCopy: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: FileActionCopy: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Src", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Src = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Dest", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Dest = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Owner", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Owner == nil {
|
|
m.Owner = &ChownOpt{}
|
|
}
|
|
if err := m.Owner.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Mode", wireType)
|
|
}
|
|
m.Mode = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Mode |= int32(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 5:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field FollowSymlink", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.FollowSymlink = bool(v != 0)
|
|
case 6:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field DirCopyContents", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.DirCopyContents = bool(v != 0)
|
|
case 7:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field AttemptUnpackDockerCompatibility", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.AttemptUnpackDockerCompatibility = bool(v != 0)
|
|
case 8:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field CreateDestPath", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.CreateDestPath = bool(v != 0)
|
|
case 9:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field AllowWildcard", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.AllowWildcard = bool(v != 0)
|
|
case 10:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field AllowEmptyWildcard", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.AllowEmptyWildcard = bool(v != 0)
|
|
case 11:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Timestamp", wireType)
|
|
}
|
|
m.Timestamp = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Timestamp |= int64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 12:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field IncludePatterns", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.IncludePatterns = append(m.IncludePatterns, string(dAtA[iNdEx:postIndex]))
|
|
iNdEx = postIndex
|
|
case 13:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ExcludePatterns", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ExcludePatterns = append(m.ExcludePatterns, string(dAtA[iNdEx:postIndex]))
|
|
iNdEx = postIndex
|
|
case 14:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field AlwaysReplaceExistingDestPaths", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.AlwaysReplaceExistingDestPaths = bool(v != 0)
|
|
case 15:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ModeStr", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ModeStr = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *FileActionMkFile) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: FileActionMkFile: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: FileActionMkFile: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Path", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Path = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Mode", wireType)
|
|
}
|
|
m.Mode = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Mode |= int32(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Data", wireType)
|
|
}
|
|
var byteLen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
byteLen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if byteLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + byteLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Data = append(m.Data[:0], dAtA[iNdEx:postIndex]...)
|
|
if m.Data == nil {
|
|
m.Data = []byte{}
|
|
}
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Owner", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Owner == nil {
|
|
m.Owner = &ChownOpt{}
|
|
}
|
|
if err := m.Owner.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 5:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Timestamp", wireType)
|
|
}
|
|
m.Timestamp = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Timestamp |= int64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *FileActionMkDir) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: FileActionMkDir: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: FileActionMkDir: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Path", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Path = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Mode", wireType)
|
|
}
|
|
m.Mode = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Mode |= int32(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 3:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field MakeParents", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.MakeParents = bool(v != 0)
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Owner", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Owner == nil {
|
|
m.Owner = &ChownOpt{}
|
|
}
|
|
if err := m.Owner.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 5:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Timestamp", wireType)
|
|
}
|
|
m.Timestamp = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Timestamp |= int64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *FileActionRm) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: FileActionRm: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: FileActionRm: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Path", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Path = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field AllowNotFound", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.AllowNotFound = bool(v != 0)
|
|
case 3:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field AllowWildcard", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.AllowWildcard = bool(v != 0)
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *ChownOpt) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: ChownOpt: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: ChownOpt: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field User", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.User == nil {
|
|
m.User = &UserOpt{}
|
|
}
|
|
if err := m.User.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Group", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Group == nil {
|
|
m.Group = &UserOpt{}
|
|
}
|
|
if err := m.Group.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *UserOpt) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: UserOpt: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: UserOpt: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ByName", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if oneof, ok := m.User.(*UserOpt_ByName); ok {
|
|
if err := oneof.ByName.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
} else {
|
|
v := &NamedUserOpt{}
|
|
if err := v.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
m.User = &UserOpt_ByName{ByName: v}
|
|
}
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ByID", wireType)
|
|
}
|
|
var v uint32
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= uint32(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.User = &UserOpt_ByID{ByID: v}
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *NamedUserOpt) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: NamedUserOpt: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: NamedUserOpt: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Name", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Name = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Input", wireType)
|
|
}
|
|
m.Input = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Input |= int64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *MergeInput) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: MergeInput: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: MergeInput: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Input", wireType)
|
|
}
|
|
m.Input = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Input |= int64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *MergeOp) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: MergeOp: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: MergeOp: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Inputs", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Inputs = append(m.Inputs, &MergeInput{})
|
|
if err := m.Inputs[len(m.Inputs)-1].UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *LowerDiffInput) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: LowerDiffInput: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: LowerDiffInput: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Input", wireType)
|
|
}
|
|
m.Input = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Input |= int64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *UpperDiffInput) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: UpperDiffInput: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: UpperDiffInput: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Input", wireType)
|
|
}
|
|
m.Input = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Input |= int64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *DiffOp) UnmarshalVT(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= uint64(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: DiffOp: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: DiffOp: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Lower", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Lower == nil {
|
|
m.Lower = &LowerDiffInput{}
|
|
}
|
|
if err := m.Lower.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Upper", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return protohelpers.ErrIntOverflow
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= int(b&0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Upper == nil {
|
|
m.Upper = &UpperDiffInput{}
|
|
}
|
|
if err := m.Upper.UnmarshalVT(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := protohelpers.Skip(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if (skippy < 0) || (iNdEx+skippy) < 0 {
|
|
return protohelpers.ErrInvalidLength
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.unknownFields = append(m.unknownFields, dAtA[iNdEx:iNdEx+skippy]...)
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|