mirror of
https://gitea.com/Lydanne/buildx.git
synced 2025-07-04 10:27:43 +08:00
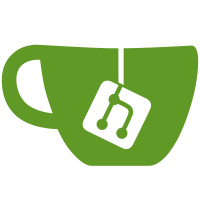
Integrates vtproto into buildx. The generated files dockerfile has been modified to copy the buildkit equivalent file to ensure files are laid out in the appropriate way for imports. An import has also been included to change the grpc codec to the version in buildkit that supports vtproto. This will allow buildx to utilize the speed and memory improvements from that. Also updates the gc control options for prune. Signed-off-by: Jonathan A. Sternberg <jonathan.sternberg@docker.com>
688 lines
25 KiB
Go
688 lines
25 KiB
Go
// Code generated by protoc-gen-go. DO NOT EDIT.
|
|
// versions:
|
|
// protoc-gen-go v1.34.1
|
|
// protoc v3.11.4
|
|
// source: github.com/moby/buildkit/sourcepolicy/pb/policy.proto
|
|
|
|
package moby_buildkit_v1_sourcepolicy
|
|
|
|
import (
|
|
protoreflect "google.golang.org/protobuf/reflect/protoreflect"
|
|
protoimpl "google.golang.org/protobuf/runtime/protoimpl"
|
|
reflect "reflect"
|
|
sync "sync"
|
|
)
|
|
|
|
const (
|
|
// Verify that this generated code is sufficiently up-to-date.
|
|
_ = protoimpl.EnforceVersion(20 - protoimpl.MinVersion)
|
|
// Verify that runtime/protoimpl is sufficiently up-to-date.
|
|
_ = protoimpl.EnforceVersion(protoimpl.MaxVersion - 20)
|
|
)
|
|
|
|
// PolicyAction defines the action to take when a source is matched
|
|
type PolicyAction int32
|
|
|
|
const (
|
|
PolicyAction_ALLOW PolicyAction = 0
|
|
PolicyAction_DENY PolicyAction = 1
|
|
PolicyAction_CONVERT PolicyAction = 2
|
|
)
|
|
|
|
// Enum value maps for PolicyAction.
|
|
var (
|
|
PolicyAction_name = map[int32]string{
|
|
0: "ALLOW",
|
|
1: "DENY",
|
|
2: "CONVERT",
|
|
}
|
|
PolicyAction_value = map[string]int32{
|
|
"ALLOW": 0,
|
|
"DENY": 1,
|
|
"CONVERT": 2,
|
|
}
|
|
)
|
|
|
|
func (x PolicyAction) Enum() *PolicyAction {
|
|
p := new(PolicyAction)
|
|
*p = x
|
|
return p
|
|
}
|
|
|
|
func (x PolicyAction) String() string {
|
|
return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x))
|
|
}
|
|
|
|
func (PolicyAction) Descriptor() protoreflect.EnumDescriptor {
|
|
return file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_enumTypes[0].Descriptor()
|
|
}
|
|
|
|
func (PolicyAction) Type() protoreflect.EnumType {
|
|
return &file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_enumTypes[0]
|
|
}
|
|
|
|
func (x PolicyAction) Number() protoreflect.EnumNumber {
|
|
return protoreflect.EnumNumber(x)
|
|
}
|
|
|
|
// Deprecated: Use PolicyAction.Descriptor instead.
|
|
func (PolicyAction) EnumDescriptor() ([]byte, []int) {
|
|
return file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_rawDescGZIP(), []int{0}
|
|
}
|
|
|
|
// AttrMatch defines the condition to match a source attribute
|
|
type AttrMatch int32
|
|
|
|
const (
|
|
AttrMatch_EQUAL AttrMatch = 0
|
|
AttrMatch_NOTEQUAL AttrMatch = 1
|
|
AttrMatch_MATCHES AttrMatch = 2
|
|
)
|
|
|
|
// Enum value maps for AttrMatch.
|
|
var (
|
|
AttrMatch_name = map[int32]string{
|
|
0: "EQUAL",
|
|
1: "NOTEQUAL",
|
|
2: "MATCHES",
|
|
}
|
|
AttrMatch_value = map[string]int32{
|
|
"EQUAL": 0,
|
|
"NOTEQUAL": 1,
|
|
"MATCHES": 2,
|
|
}
|
|
)
|
|
|
|
func (x AttrMatch) Enum() *AttrMatch {
|
|
p := new(AttrMatch)
|
|
*p = x
|
|
return p
|
|
}
|
|
|
|
func (x AttrMatch) String() string {
|
|
return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x))
|
|
}
|
|
|
|
func (AttrMatch) Descriptor() protoreflect.EnumDescriptor {
|
|
return file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_enumTypes[1].Descriptor()
|
|
}
|
|
|
|
func (AttrMatch) Type() protoreflect.EnumType {
|
|
return &file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_enumTypes[1]
|
|
}
|
|
|
|
func (x AttrMatch) Number() protoreflect.EnumNumber {
|
|
return protoreflect.EnumNumber(x)
|
|
}
|
|
|
|
// Deprecated: Use AttrMatch.Descriptor instead.
|
|
func (AttrMatch) EnumDescriptor() ([]byte, []int) {
|
|
return file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_rawDescGZIP(), []int{1}
|
|
}
|
|
|
|
// Match type is used to determine how a rule source is matched
|
|
type MatchType int32
|
|
|
|
const (
|
|
// WILDCARD is the default matching type.
|
|
// It may first attempt to due an exact match but will follow up with a wildcard match
|
|
// For something more powerful, use REGEX
|
|
MatchType_WILDCARD MatchType = 0
|
|
// EXACT treats the source identifier as a litteral string match
|
|
MatchType_EXACT MatchType = 1
|
|
// REGEX treats the source identifier as a regular expression
|
|
// With regex matching you can also use match groups to replace values in the destination identifier
|
|
MatchType_REGEX MatchType = 2
|
|
)
|
|
|
|
// Enum value maps for MatchType.
|
|
var (
|
|
MatchType_name = map[int32]string{
|
|
0: "WILDCARD",
|
|
1: "EXACT",
|
|
2: "REGEX",
|
|
}
|
|
MatchType_value = map[string]int32{
|
|
"WILDCARD": 0,
|
|
"EXACT": 1,
|
|
"REGEX": 2,
|
|
}
|
|
)
|
|
|
|
func (x MatchType) Enum() *MatchType {
|
|
p := new(MatchType)
|
|
*p = x
|
|
return p
|
|
}
|
|
|
|
func (x MatchType) String() string {
|
|
return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x))
|
|
}
|
|
|
|
func (MatchType) Descriptor() protoreflect.EnumDescriptor {
|
|
return file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_enumTypes[2].Descriptor()
|
|
}
|
|
|
|
func (MatchType) Type() protoreflect.EnumType {
|
|
return &file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_enumTypes[2]
|
|
}
|
|
|
|
func (x MatchType) Number() protoreflect.EnumNumber {
|
|
return protoreflect.EnumNumber(x)
|
|
}
|
|
|
|
// Deprecated: Use MatchType.Descriptor instead.
|
|
func (MatchType) EnumDescriptor() ([]byte, []int) {
|
|
return file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_rawDescGZIP(), []int{2}
|
|
}
|
|
|
|
// Rule defines the action(s) to take when a source is matched
|
|
type Rule struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Action PolicyAction `protobuf:"varint,1,opt,name=action,proto3,enum=moby.buildkit.v1.sourcepolicy.PolicyAction" json:"action,omitempty"`
|
|
Selector *Selector `protobuf:"bytes,2,opt,name=selector,proto3" json:"selector,omitempty"`
|
|
Updates *Update `protobuf:"bytes,3,opt,name=updates,proto3" json:"updates,omitempty"`
|
|
}
|
|
|
|
func (x *Rule) Reset() {
|
|
*x = Rule{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_msgTypes[0]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *Rule) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*Rule) ProtoMessage() {}
|
|
|
|
func (x *Rule) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_msgTypes[0]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use Rule.ProtoReflect.Descriptor instead.
|
|
func (*Rule) Descriptor() ([]byte, []int) {
|
|
return file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_rawDescGZIP(), []int{0}
|
|
}
|
|
|
|
func (x *Rule) GetAction() PolicyAction {
|
|
if x != nil {
|
|
return x.Action
|
|
}
|
|
return PolicyAction_ALLOW
|
|
}
|
|
|
|
func (x *Rule) GetSelector() *Selector {
|
|
if x != nil {
|
|
return x.Selector
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *Rule) GetUpdates() *Update {
|
|
if x != nil {
|
|
return x.Updates
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// Update contains updates to the matched build step after rule is applied
|
|
type Update struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Identifier string `protobuf:"bytes,1,opt,name=identifier,proto3" json:"identifier,omitempty"`
|
|
Attrs map[string]string `protobuf:"bytes,2,rep,name=attrs,proto3" json:"attrs,omitempty" protobuf_key:"bytes,1,opt,name=key,proto3" protobuf_val:"bytes,2,opt,name=value,proto3"`
|
|
}
|
|
|
|
func (x *Update) Reset() {
|
|
*x = Update{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_msgTypes[1]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *Update) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*Update) ProtoMessage() {}
|
|
|
|
func (x *Update) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_msgTypes[1]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use Update.ProtoReflect.Descriptor instead.
|
|
func (*Update) Descriptor() ([]byte, []int) {
|
|
return file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_rawDescGZIP(), []int{1}
|
|
}
|
|
|
|
func (x *Update) GetIdentifier() string {
|
|
if x != nil {
|
|
return x.Identifier
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *Update) GetAttrs() map[string]string {
|
|
if x != nil {
|
|
return x.Attrs
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// Selector identifies a source to match a policy to
|
|
type Selector struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Identifier string `protobuf:"bytes,1,opt,name=identifier,proto3" json:"identifier,omitempty"`
|
|
// MatchType is the type of match to perform on the source identifier
|
|
MatchType MatchType `protobuf:"varint,2,opt,name=match_type,json=matchType,proto3,enum=moby.buildkit.v1.sourcepolicy.MatchType" json:"match_type,omitempty"`
|
|
Constraints []*AttrConstraint `protobuf:"bytes,3,rep,name=constraints,proto3" json:"constraints,omitempty"`
|
|
}
|
|
|
|
func (x *Selector) Reset() {
|
|
*x = Selector{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_msgTypes[2]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *Selector) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*Selector) ProtoMessage() {}
|
|
|
|
func (x *Selector) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_msgTypes[2]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use Selector.ProtoReflect.Descriptor instead.
|
|
func (*Selector) Descriptor() ([]byte, []int) {
|
|
return file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_rawDescGZIP(), []int{2}
|
|
}
|
|
|
|
func (x *Selector) GetIdentifier() string {
|
|
if x != nil {
|
|
return x.Identifier
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *Selector) GetMatchType() MatchType {
|
|
if x != nil {
|
|
return x.MatchType
|
|
}
|
|
return MatchType_WILDCARD
|
|
}
|
|
|
|
func (x *Selector) GetConstraints() []*AttrConstraint {
|
|
if x != nil {
|
|
return x.Constraints
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// AttrConstraint defines a constraint on a source attribute
|
|
type AttrConstraint struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Key string `protobuf:"bytes,1,opt,name=key,proto3" json:"key,omitempty"`
|
|
Value string `protobuf:"bytes,2,opt,name=value,proto3" json:"value,omitempty"`
|
|
Condition AttrMatch `protobuf:"varint,3,opt,name=condition,proto3,enum=moby.buildkit.v1.sourcepolicy.AttrMatch" json:"condition,omitempty"`
|
|
}
|
|
|
|
func (x *AttrConstraint) Reset() {
|
|
*x = AttrConstraint{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_msgTypes[3]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *AttrConstraint) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*AttrConstraint) ProtoMessage() {}
|
|
|
|
func (x *AttrConstraint) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_msgTypes[3]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use AttrConstraint.ProtoReflect.Descriptor instead.
|
|
func (*AttrConstraint) Descriptor() ([]byte, []int) {
|
|
return file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_rawDescGZIP(), []int{3}
|
|
}
|
|
|
|
func (x *AttrConstraint) GetKey() string {
|
|
if x != nil {
|
|
return x.Key
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *AttrConstraint) GetValue() string {
|
|
if x != nil {
|
|
return x.Value
|
|
}
|
|
return ""
|
|
}
|
|
|
|
func (x *AttrConstraint) GetCondition() AttrMatch {
|
|
if x != nil {
|
|
return x.Condition
|
|
}
|
|
return AttrMatch_EQUAL
|
|
}
|
|
|
|
// Policy is the list of rules the policy engine will perform
|
|
type Policy struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Version int64 `protobuf:"varint,1,opt,name=version,proto3" json:"version,omitempty"` // Currently 1
|
|
Rules []*Rule `protobuf:"bytes,2,rep,name=rules,proto3" json:"rules,omitempty"`
|
|
}
|
|
|
|
func (x *Policy) Reset() {
|
|
*x = Policy{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_msgTypes[4]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *Policy) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*Policy) ProtoMessage() {}
|
|
|
|
func (x *Policy) ProtoReflect() protoreflect.Message {
|
|
mi := &file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_msgTypes[4]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use Policy.ProtoReflect.Descriptor instead.
|
|
func (*Policy) Descriptor() ([]byte, []int) {
|
|
return file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_rawDescGZIP(), []int{4}
|
|
}
|
|
|
|
func (x *Policy) GetVersion() int64 {
|
|
if x != nil {
|
|
return x.Version
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *Policy) GetRules() []*Rule {
|
|
if x != nil {
|
|
return x.Rules
|
|
}
|
|
return nil
|
|
}
|
|
|
|
var File_github_com_moby_buildkit_sourcepolicy_pb_policy_proto protoreflect.FileDescriptor
|
|
|
|
var file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_rawDesc = []byte{
|
|
0x0a, 0x35, 0x67, 0x69, 0x74, 0x68, 0x75, 0x62, 0x2e, 0x63, 0x6f, 0x6d, 0x2f, 0x6d, 0x6f, 0x62,
|
|
0x79, 0x2f, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2f, 0x73, 0x6f, 0x75, 0x72, 0x63,
|
|
0x65, 0x70, 0x6f, 0x6c, 0x69, 0x63, 0x79, 0x2f, 0x70, 0x62, 0x2f, 0x70, 0x6f, 0x6c, 0x69, 0x63,
|
|
0x79, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x12, 0x1d, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75,
|
|
0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x73, 0x6f, 0x75, 0x72, 0x63, 0x65,
|
|
0x70, 0x6f, 0x6c, 0x69, 0x63, 0x79, 0x22, 0xd1, 0x01, 0x0a, 0x04, 0x52, 0x75, 0x6c, 0x65, 0x12,
|
|
0x43, 0x0a, 0x06, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0e, 0x32,
|
|
0x2b, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e,
|
|
0x76, 0x31, 0x2e, 0x73, 0x6f, 0x75, 0x72, 0x63, 0x65, 0x70, 0x6f, 0x6c, 0x69, 0x63, 0x79, 0x2e,
|
|
0x50, 0x6f, 0x6c, 0x69, 0x63, 0x79, 0x41, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x52, 0x06, 0x61, 0x63,
|
|
0x74, 0x69, 0x6f, 0x6e, 0x12, 0x43, 0x0a, 0x08, 0x73, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x6f, 0x72,
|
|
0x18, 0x02, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x27, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75,
|
|
0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x73, 0x6f, 0x75, 0x72, 0x63, 0x65,
|
|
0x70, 0x6f, 0x6c, 0x69, 0x63, 0x79, 0x2e, 0x53, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x6f, 0x72, 0x52,
|
|
0x08, 0x73, 0x65, 0x6c, 0x65, 0x63, 0x74, 0x6f, 0x72, 0x12, 0x3f, 0x0a, 0x07, 0x75, 0x70, 0x64,
|
|
0x61, 0x74, 0x65, 0x73, 0x18, 0x03, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x25, 0x2e, 0x6d, 0x6f, 0x62,
|
|
0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x73, 0x6f,
|
|
0x75, 0x72, 0x63, 0x65, 0x70, 0x6f, 0x6c, 0x69, 0x63, 0x79, 0x2e, 0x55, 0x70, 0x64, 0x61, 0x74,
|
|
0x65, 0x52, 0x07, 0x75, 0x70, 0x64, 0x61, 0x74, 0x65, 0x73, 0x22, 0xaa, 0x01, 0x0a, 0x06, 0x55,
|
|
0x70, 0x64, 0x61, 0x74, 0x65, 0x12, 0x1e, 0x0a, 0x0a, 0x69, 0x64, 0x65, 0x6e, 0x74, 0x69, 0x66,
|
|
0x69, 0x65, 0x72, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0a, 0x69, 0x64, 0x65, 0x6e, 0x74,
|
|
0x69, 0x66, 0x69, 0x65, 0x72, 0x12, 0x46, 0x0a, 0x05, 0x61, 0x74, 0x74, 0x72, 0x73, 0x18, 0x02,
|
|
0x20, 0x03, 0x28, 0x0b, 0x32, 0x30, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c,
|
|
0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x73, 0x6f, 0x75, 0x72, 0x63, 0x65, 0x70, 0x6f,
|
|
0x6c, 0x69, 0x63, 0x79, 0x2e, 0x55, 0x70, 0x64, 0x61, 0x74, 0x65, 0x2e, 0x41, 0x74, 0x74, 0x72,
|
|
0x73, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x52, 0x05, 0x61, 0x74, 0x74, 0x72, 0x73, 0x1a, 0x38, 0x0a,
|
|
0x0a, 0x41, 0x74, 0x74, 0x72, 0x73, 0x45, 0x6e, 0x74, 0x72, 0x79, 0x12, 0x10, 0x0a, 0x03, 0x6b,
|
|
0x65, 0x79, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x03, 0x6b, 0x65, 0x79, 0x12, 0x14, 0x0a,
|
|
0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x09, 0x52, 0x05, 0x76, 0x61,
|
|
0x6c, 0x75, 0x65, 0x3a, 0x02, 0x38, 0x01, 0x22, 0xc4, 0x01, 0x0a, 0x08, 0x53, 0x65, 0x6c, 0x65,
|
|
0x63, 0x74, 0x6f, 0x72, 0x12, 0x1e, 0x0a, 0x0a, 0x69, 0x64, 0x65, 0x6e, 0x74, 0x69, 0x66, 0x69,
|
|
0x65, 0x72, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0a, 0x69, 0x64, 0x65, 0x6e, 0x74, 0x69,
|
|
0x66, 0x69, 0x65, 0x72, 0x12, 0x47, 0x0a, 0x0a, 0x6d, 0x61, 0x74, 0x63, 0x68, 0x5f, 0x74, 0x79,
|
|
0x70, 0x65, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0e, 0x32, 0x28, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e,
|
|
0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x73, 0x6f, 0x75, 0x72,
|
|
0x63, 0x65, 0x70, 0x6f, 0x6c, 0x69, 0x63, 0x79, 0x2e, 0x4d, 0x61, 0x74, 0x63, 0x68, 0x54, 0x79,
|
|
0x70, 0x65, 0x52, 0x09, 0x6d, 0x61, 0x74, 0x63, 0x68, 0x54, 0x79, 0x70, 0x65, 0x12, 0x4f, 0x0a,
|
|
0x0b, 0x63, 0x6f, 0x6e, 0x73, 0x74, 0x72, 0x61, 0x69, 0x6e, 0x74, 0x73, 0x18, 0x03, 0x20, 0x03,
|
|
0x28, 0x0b, 0x32, 0x2d, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b,
|
|
0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x73, 0x6f, 0x75, 0x72, 0x63, 0x65, 0x70, 0x6f, 0x6c, 0x69,
|
|
0x63, 0x79, 0x2e, 0x41, 0x74, 0x74, 0x72, 0x43, 0x6f, 0x6e, 0x73, 0x74, 0x72, 0x61, 0x69, 0x6e,
|
|
0x74, 0x52, 0x0b, 0x63, 0x6f, 0x6e, 0x73, 0x74, 0x72, 0x61, 0x69, 0x6e, 0x74, 0x73, 0x22, 0x80,
|
|
0x01, 0x0a, 0x0e, 0x41, 0x74, 0x74, 0x72, 0x43, 0x6f, 0x6e, 0x73, 0x74, 0x72, 0x61, 0x69, 0x6e,
|
|
0x74, 0x12, 0x10, 0x0a, 0x03, 0x6b, 0x65, 0x79, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x03,
|
|
0x6b, 0x65, 0x79, 0x12, 0x14, 0x0a, 0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x18, 0x02, 0x20, 0x01,
|
|
0x28, 0x09, 0x52, 0x05, 0x76, 0x61, 0x6c, 0x75, 0x65, 0x12, 0x46, 0x0a, 0x09, 0x63, 0x6f, 0x6e,
|
|
0x64, 0x69, 0x74, 0x69, 0x6f, 0x6e, 0x18, 0x03, 0x20, 0x01, 0x28, 0x0e, 0x32, 0x28, 0x2e, 0x6d,
|
|
0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e,
|
|
0x73, 0x6f, 0x75, 0x72, 0x63, 0x65, 0x70, 0x6f, 0x6c, 0x69, 0x63, 0x79, 0x2e, 0x41, 0x74, 0x74,
|
|
0x72, 0x4d, 0x61, 0x74, 0x63, 0x68, 0x52, 0x09, 0x63, 0x6f, 0x6e, 0x64, 0x69, 0x74, 0x69, 0x6f,
|
|
0x6e, 0x22, 0x5d, 0x0a, 0x06, 0x50, 0x6f, 0x6c, 0x69, 0x63, 0x79, 0x12, 0x18, 0x0a, 0x07, 0x76,
|
|
0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x18, 0x01, 0x20, 0x01, 0x28, 0x03, 0x52, 0x07, 0x76, 0x65,
|
|
0x72, 0x73, 0x69, 0x6f, 0x6e, 0x12, 0x39, 0x0a, 0x05, 0x72, 0x75, 0x6c, 0x65, 0x73, 0x18, 0x02,
|
|
0x20, 0x03, 0x28, 0x0b, 0x32, 0x23, 0x2e, 0x6d, 0x6f, 0x62, 0x79, 0x2e, 0x62, 0x75, 0x69, 0x6c,
|
|
0x64, 0x6b, 0x69, 0x74, 0x2e, 0x76, 0x31, 0x2e, 0x73, 0x6f, 0x75, 0x72, 0x63, 0x65, 0x70, 0x6f,
|
|
0x6c, 0x69, 0x63, 0x79, 0x2e, 0x52, 0x75, 0x6c, 0x65, 0x52, 0x05, 0x72, 0x75, 0x6c, 0x65, 0x73,
|
|
0x2a, 0x30, 0x0a, 0x0c, 0x50, 0x6f, 0x6c, 0x69, 0x63, 0x79, 0x41, 0x63, 0x74, 0x69, 0x6f, 0x6e,
|
|
0x12, 0x09, 0x0a, 0x05, 0x41, 0x4c, 0x4c, 0x4f, 0x57, 0x10, 0x00, 0x12, 0x08, 0x0a, 0x04, 0x44,
|
|
0x45, 0x4e, 0x59, 0x10, 0x01, 0x12, 0x0b, 0x0a, 0x07, 0x43, 0x4f, 0x4e, 0x56, 0x45, 0x52, 0x54,
|
|
0x10, 0x02, 0x2a, 0x31, 0x0a, 0x09, 0x41, 0x74, 0x74, 0x72, 0x4d, 0x61, 0x74, 0x63, 0x68, 0x12,
|
|
0x09, 0x0a, 0x05, 0x45, 0x51, 0x55, 0x41, 0x4c, 0x10, 0x00, 0x12, 0x0c, 0x0a, 0x08, 0x4e, 0x4f,
|
|
0x54, 0x45, 0x51, 0x55, 0x41, 0x4c, 0x10, 0x01, 0x12, 0x0b, 0x0a, 0x07, 0x4d, 0x41, 0x54, 0x43,
|
|
0x48, 0x45, 0x53, 0x10, 0x02, 0x2a, 0x2f, 0x0a, 0x09, 0x4d, 0x61, 0x74, 0x63, 0x68, 0x54, 0x79,
|
|
0x70, 0x65, 0x12, 0x0c, 0x0a, 0x08, 0x57, 0x49, 0x4c, 0x44, 0x43, 0x41, 0x52, 0x44, 0x10, 0x00,
|
|
0x12, 0x09, 0x0a, 0x05, 0x45, 0x58, 0x41, 0x43, 0x54, 0x10, 0x01, 0x12, 0x09, 0x0a, 0x05, 0x52,
|
|
0x45, 0x47, 0x45, 0x58, 0x10, 0x02, 0x42, 0x48, 0x5a, 0x46, 0x67, 0x69, 0x74, 0x68, 0x75, 0x62,
|
|
0x2e, 0x63, 0x6f, 0x6d, 0x2f, 0x6d, 0x6f, 0x62, 0x79, 0x2f, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b,
|
|
0x69, 0x74, 0x2f, 0x73, 0x6f, 0x75, 0x72, 0x63, 0x65, 0x70, 0x6f, 0x6c, 0x69, 0x63, 0x79, 0x2f,
|
|
0x70, 0x62, 0x3b, 0x6d, 0x6f, 0x62, 0x79, 0x5f, 0x62, 0x75, 0x69, 0x6c, 0x64, 0x6b, 0x69, 0x74,
|
|
0x5f, 0x76, 0x31, 0x5f, 0x73, 0x6f, 0x75, 0x72, 0x63, 0x65, 0x70, 0x6f, 0x6c, 0x69, 0x63, 0x79,
|
|
0x62, 0x06, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x33,
|
|
}
|
|
|
|
var (
|
|
file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_rawDescOnce sync.Once
|
|
file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_rawDescData = file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_rawDesc
|
|
)
|
|
|
|
func file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_rawDescGZIP() []byte {
|
|
file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_rawDescOnce.Do(func() {
|
|
file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_rawDescData = protoimpl.X.CompressGZIP(file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_rawDescData)
|
|
})
|
|
return file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_rawDescData
|
|
}
|
|
|
|
var file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_enumTypes = make([]protoimpl.EnumInfo, 3)
|
|
var file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_msgTypes = make([]protoimpl.MessageInfo, 6)
|
|
var file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_goTypes = []interface{}{
|
|
(PolicyAction)(0), // 0: moby.buildkit.v1.sourcepolicy.PolicyAction
|
|
(AttrMatch)(0), // 1: moby.buildkit.v1.sourcepolicy.AttrMatch
|
|
(MatchType)(0), // 2: moby.buildkit.v1.sourcepolicy.MatchType
|
|
(*Rule)(nil), // 3: moby.buildkit.v1.sourcepolicy.Rule
|
|
(*Update)(nil), // 4: moby.buildkit.v1.sourcepolicy.Update
|
|
(*Selector)(nil), // 5: moby.buildkit.v1.sourcepolicy.Selector
|
|
(*AttrConstraint)(nil), // 6: moby.buildkit.v1.sourcepolicy.AttrConstraint
|
|
(*Policy)(nil), // 7: moby.buildkit.v1.sourcepolicy.Policy
|
|
nil, // 8: moby.buildkit.v1.sourcepolicy.Update.AttrsEntry
|
|
}
|
|
var file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_depIdxs = []int32{
|
|
0, // 0: moby.buildkit.v1.sourcepolicy.Rule.action:type_name -> moby.buildkit.v1.sourcepolicy.PolicyAction
|
|
5, // 1: moby.buildkit.v1.sourcepolicy.Rule.selector:type_name -> moby.buildkit.v1.sourcepolicy.Selector
|
|
4, // 2: moby.buildkit.v1.sourcepolicy.Rule.updates:type_name -> moby.buildkit.v1.sourcepolicy.Update
|
|
8, // 3: moby.buildkit.v1.sourcepolicy.Update.attrs:type_name -> moby.buildkit.v1.sourcepolicy.Update.AttrsEntry
|
|
2, // 4: moby.buildkit.v1.sourcepolicy.Selector.match_type:type_name -> moby.buildkit.v1.sourcepolicy.MatchType
|
|
6, // 5: moby.buildkit.v1.sourcepolicy.Selector.constraints:type_name -> moby.buildkit.v1.sourcepolicy.AttrConstraint
|
|
1, // 6: moby.buildkit.v1.sourcepolicy.AttrConstraint.condition:type_name -> moby.buildkit.v1.sourcepolicy.AttrMatch
|
|
3, // 7: moby.buildkit.v1.sourcepolicy.Policy.rules:type_name -> moby.buildkit.v1.sourcepolicy.Rule
|
|
8, // [8:8] is the sub-list for method output_type
|
|
8, // [8:8] is the sub-list for method input_type
|
|
8, // [8:8] is the sub-list for extension type_name
|
|
8, // [8:8] is the sub-list for extension extendee
|
|
0, // [0:8] is the sub-list for field type_name
|
|
}
|
|
|
|
func init() { file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_init() }
|
|
func file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_init() {
|
|
if File_github_com_moby_buildkit_sourcepolicy_pb_policy_proto != nil {
|
|
return
|
|
}
|
|
if !protoimpl.UnsafeEnabled {
|
|
file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_msgTypes[0].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*Rule); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_msgTypes[1].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*Update); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_msgTypes[2].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*Selector); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_msgTypes[3].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*AttrConstraint); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_msgTypes[4].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*Policy); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
}
|
|
type x struct{}
|
|
out := protoimpl.TypeBuilder{
|
|
File: protoimpl.DescBuilder{
|
|
GoPackagePath: reflect.TypeOf(x{}).PkgPath(),
|
|
RawDescriptor: file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_rawDesc,
|
|
NumEnums: 3,
|
|
NumMessages: 6,
|
|
NumExtensions: 0,
|
|
NumServices: 0,
|
|
},
|
|
GoTypes: file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_goTypes,
|
|
DependencyIndexes: file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_depIdxs,
|
|
EnumInfos: file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_enumTypes,
|
|
MessageInfos: file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_msgTypes,
|
|
}.Build()
|
|
File_github_com_moby_buildkit_sourcepolicy_pb_policy_proto = out.File
|
|
file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_rawDesc = nil
|
|
file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_goTypes = nil
|
|
file_github_com_moby_buildkit_sourcepolicy_pb_policy_proto_depIdxs = nil
|
|
}
|