mirror of
https://gitea.com/Lydanne/buildx.git
synced 2025-05-18 00:47:48 +08:00
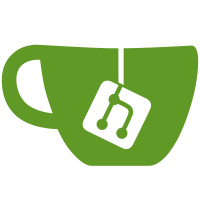
Removes gogo/protobuf from buildx and updates to a version of moby/buildkit where gogo is removed. This also changes how the proto files are generated. This is because newer versions of protobuf are more strict about name conflicts. If two files have the same name (even if they are relative paths) and are used in different protoc commands, they'll conflict in the registry. Since protobuf file generation doesn't work very well with `paths=source_relative`, this removes the `go:generate` expression and just relies on the dockerfile to perform the generation. Signed-off-by: Jonathan A. Sternberg <jonathan.sternberg@docker.com>
79 lines
1.6 KiB
Go
79 lines
1.6 KiB
Go
package bake
|
|
|
|
import (
|
|
"strings"
|
|
|
|
"github.com/hashicorp/hcl/v2"
|
|
"github.com/hashicorp/hcl/v2/hclparse"
|
|
"github.com/moby/buildkit/solver/errdefs"
|
|
"github.com/moby/buildkit/solver/pb"
|
|
)
|
|
|
|
func ParseHCLFile(dt []byte, fn string) (*hcl.File, bool, error) {
|
|
var err error
|
|
if strings.HasSuffix(fn, ".json") {
|
|
f, diags := hclparse.NewParser().ParseJSON(dt, fn)
|
|
if diags.HasErrors() {
|
|
err = diags
|
|
}
|
|
return f, true, err
|
|
}
|
|
if strings.HasSuffix(fn, ".hcl") {
|
|
f, diags := hclparse.NewParser().ParseHCL(dt, fn)
|
|
if diags.HasErrors() {
|
|
err = diags
|
|
}
|
|
return f, true, err
|
|
}
|
|
f, diags := hclparse.NewParser().ParseHCL(dt, fn+".hcl")
|
|
if diags.HasErrors() {
|
|
f, diags2 := hclparse.NewParser().ParseJSON(dt, fn+".json")
|
|
if !diags2.HasErrors() {
|
|
return f, true, nil
|
|
}
|
|
return nil, false, diags
|
|
}
|
|
return f, true, nil
|
|
}
|
|
|
|
func formatHCLError(err error, files []File) error {
|
|
if err == nil {
|
|
return nil
|
|
}
|
|
diags, ok := err.(hcl.Diagnostics)
|
|
if !ok {
|
|
return err
|
|
}
|
|
for _, d := range diags {
|
|
if d.Severity != hcl.DiagError {
|
|
continue
|
|
}
|
|
if d.Subject != nil {
|
|
var dt []byte
|
|
for _, f := range files {
|
|
if d.Subject.Filename == f.Name {
|
|
dt = f.Data
|
|
break
|
|
}
|
|
}
|
|
src := &errdefs.Source{
|
|
Info: &pb.SourceInfo{
|
|
Filename: d.Subject.Filename,
|
|
Data: dt,
|
|
},
|
|
Ranges: []*pb.Range{toErrRange(d.Subject)},
|
|
}
|
|
err = errdefs.WithSource(err, src)
|
|
break
|
|
}
|
|
}
|
|
return err
|
|
}
|
|
|
|
func toErrRange(in *hcl.Range) *pb.Range {
|
|
return &pb.Range{
|
|
Start: &pb.Position{Line: int32(in.Start.Line), Character: int32(in.Start.Column)},
|
|
End: &pb.Position{Line: int32(in.End.Line), Character: int32(in.End.Column)},
|
|
}
|
|
}
|